Simplifying the ! [NOT] Operator in Javascript
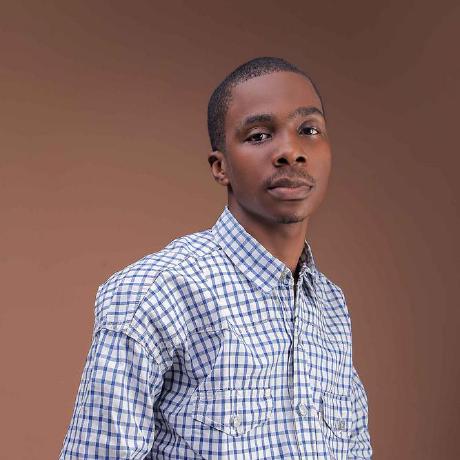
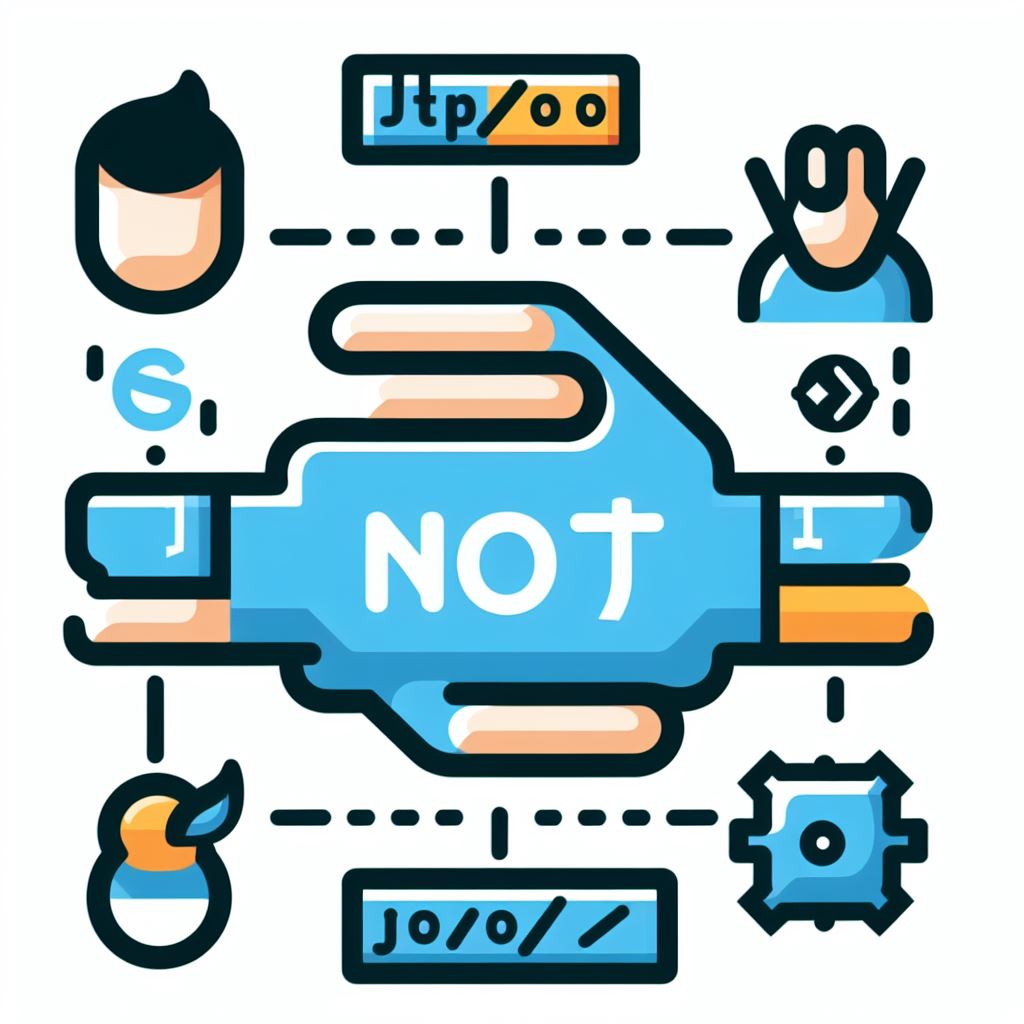
Introduction
The concept of a logical negation operator (for the purpose of this article let's call it NOT operator) was tricky for me to understand when I first encountered it in practical code, and it wasn't just understanding what it could do but how it worked was hard for me to grasp. Not anymore, I have figured both the "what" and "how" of the NOT operator and that's what we will be looking at in this article.
What NOT (!) Operator does?
The NOT operator denoted by an exclamation mark (!) is also known as the logical negation operator. After the AND(&&) and OR(||) operator, it is the third logical operator in Javascript. It's major objective is to negate a boolean operator i.e turn a value from truthy to falsey and vice versa. Just a reminder on truth-y and false-y values: there are values that when evaluated are either true or false in a boolean context (you can read more about truthy and falsey values).
let truthyValue = 11;
console.log(!truthyValue);
//output: false
let isTrue = true;
let isFalse = !true;
console.log(isTrue) //output: true
console.log(isFalse) //output: false
As seen with the examples above, when the ! sign is placed before a value or variable it negates that value from it's current boolean context to the opposite, i.e true to false and vice versa. The variable isTrue
still holds the value of true when referenced through the code. How does this happen?
How does the !NOT operator work?
Now that we know what the operator does, let's dive into how it works using a popular ! NOT operator example:
const isSunny = true;
const isRainy = !isSunny;
// isRainy is false
Let's break down the code above, it's important to note the NOT operator changes values in the boolean context and this means it has to check if the value is truthy or falsey, that's the first step in the conversion and when it does that it will then turn the value or assigned variable to the either truthy or falsey.
Using a step-by-step breakdown:
Convert the value to it's boolean context.
Turn the value which is now in it's boolean context to either true or false, basically negating the current value.
Pretty simple right? Let's crank it up a notch and dig deeper.
function formatAmount(amount) {
let zero = amount.toString().split('.');
let cents = zero[1];
if(!cents){
return (`$${amount}.00`)
} else if (cents.length === 1){
return (`$${amount}0`)
} else {
return (`$${amount}`)
}
}
console.log(formatAmount(5.5)) // output: $5.50
console.log(formatAmount(15)) // output: $15.00
In the example above, we have a function that takes a numeric value as it's argument and returns a formatted string with dollar
and cents
.
cents
has been assigned a value which is the index [1] of zero array[]. Our primary focus will be the first if block.
In the case of the first if statement, the ! NOT operator is negating the the value of cents (zero[1]) so it'll first evaluate the cents variable and convert to it's boolean context, if it's false-y value it will negate to true and the body of the code will execute else it'll check other conditions.
Conclusion
The important takeaway from this article is building that mental picture on how the operator works: which is negating a value in it's boolean context and understanding the how it negates this value, by first evaluating the value to it's boolean context either true or false and then negating it depending on the result of the value.
Remember that the ! operator doesn’t modify the original value; it only provides its negation in boolean expressions.
Subscribe to my newsletter
Read articles from Emmanuel Edem directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
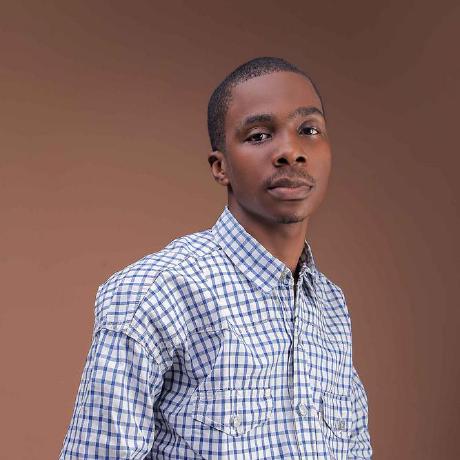