๐ณDay 21 - Docker Important interview Questions.
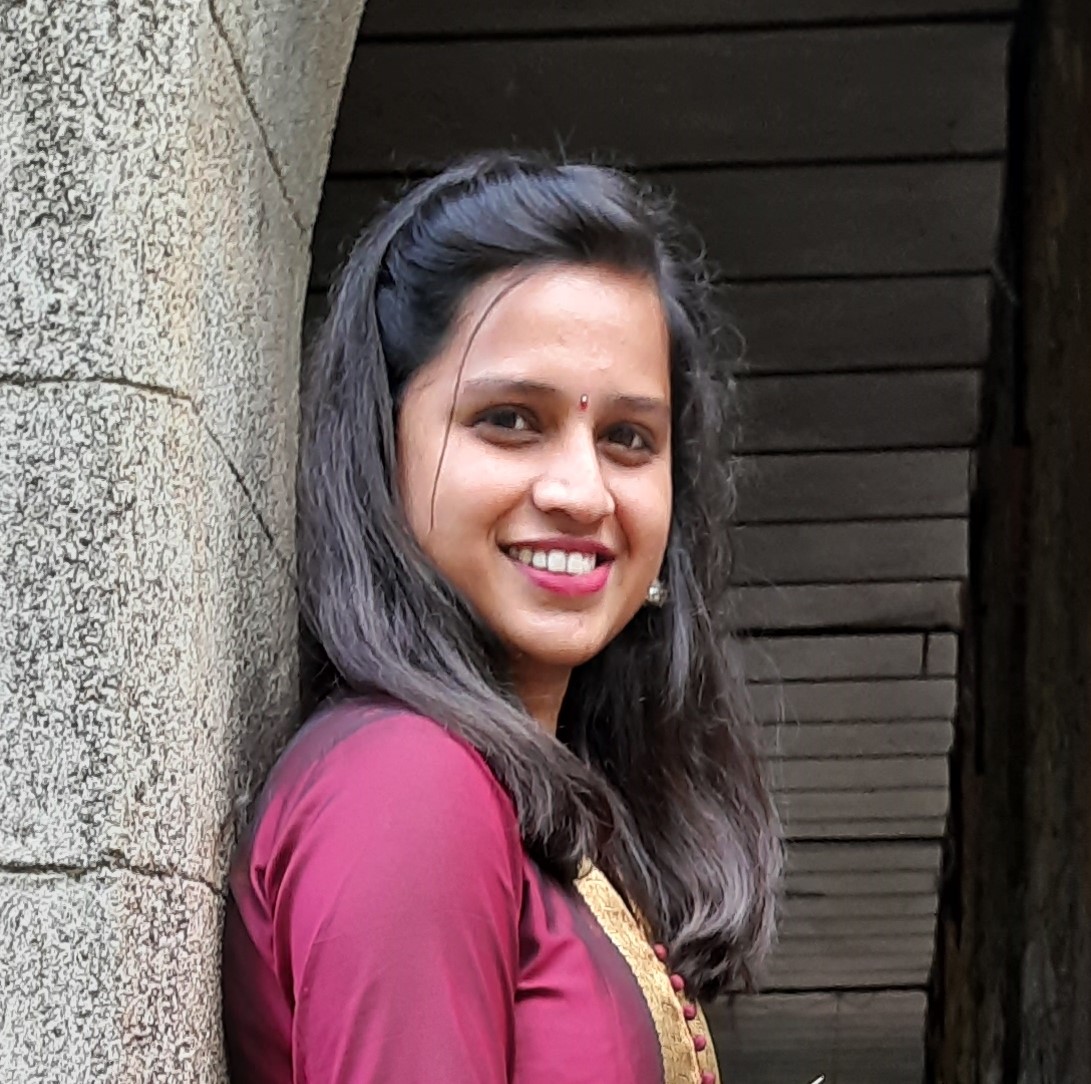
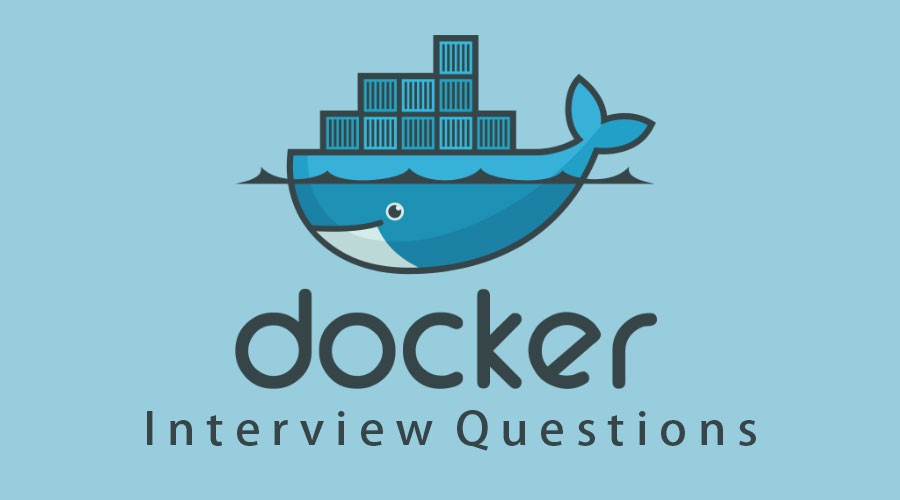
What is the Difference between an Image, Container and Engine?
In the context of Docker, an Image, Container, and Docker Engine are fundamental concepts that play distinct roles in the containerization process. Here's a brief explanation of each:
Docker Image:
An image is a lightweight, standalone, and executable package that includes everything needed to run a piece of software, including the code, runtime, libraries, and system tools.
It is essentially a snapshot of a file system and parameters needed to create and run a container.
Images are often built from a set of instructions called a Dockerfile, which specifies the configuration of the image.
Docker Container:
A container is a running instance of a Docker image.
It encapsulates the application and its dependencies in an isolated environment, ensuring consistency across different environments (development, testing, production).
Containers are lightweight and can be easily started, stopped, moved, and deleted.
Docker Engine:
The Docker Engine is the core component of Docker, responsible for building, running, and managing Docker containers.
It consists of a server and a REST API that interfaces with the host operating system and facilitates communication between containers and the host system.
The Docker Engine includes several components, such as a daemon, REST API, and a command-line interface (CLI) for interacting with Docker.
What is the Difference between the Docker command COPY vs ADD?
In Docker, both the
COPY
andADD
commands are used to copy files and directories from the host machine into a Docker image. However, there are some differences in their behavior:โ COPY
COPY
is a simpler and more straightforward command.It is used to copy files or directories from the host machine to the container filesystem.
The basic syntax is:
COPY <src> <dest>
<src>
can be a file or directory on the host machine, and<dest>
is the destination path inside the container.
Example:
COPY ./app /usr/src/app
โ ADD:
ADD
has additional features compared toCOPY
.In addition to copying files and directories, it can also handle URLs and automatically extract compressed files.
The syntax for
ADD
is similar toCOPY
:ADD <src> <dest>
<src>
can be a local file or directory, a URL, or an archive (tar, gzip, bzip2) that will be automatically extracted to the destination in the container.
Example:
ADD ./archive.tar.gz /usr/src/app
What is the Difference between the Docker command CMD vs RUN?
In Docker, the
CMD
andRUN
commands serve different purposes during the image building process, and they are used at different stages:โ RUN:
The
RUN
command is used to execute commands during the build process. These commands are run in a new layer on top of the current image, and the results are committed to the image.It is typically used for installing software, updating packages, or any other tasks required to set up the environment within the image.
The syntax for
RUN
is as follows:RUN <command>
Example:
RUN apt-get update && apt-get install -y nginx
โ CMD:
The
CMD
command is used to provide default arguments for the entry point of the container. It specifies the command and its arguments to be executed when the container starts.CMD
is often used to define the default behavior of the container, such as the main application or process to run when the container is launched.If a Docker image has multiple
CMD
instructions, only the last one takes effect.The syntax for
CMD
is as follows:CMD ["executable","param1","param2"]
or
CMD command param1 param2
Example:
CMD ["nginx", "-g", "daemon off;"]
Usage Scenario:
Use
RUN
for actions that are executed during the image build process to set up the environment, install dependencies, and configure the image.Use
CMD
to specify the default command that should be executed when a container is run based on the image.
How Will you reduce the size of the Docker image?
Reducing the size of a Docker image is crucial for optimizing performance, storage, and transfer times. Here are several strategies to minimize the size of your Docker images:
โ Use Minimal Base Images:
Start with a lightweight base image. Alpine Linux is a popular choice for its small size and security features.
Instead of using a general-purpose base image, choose one tailored to your application's specific needs.
Example with Alpine Linux:
FROM alpine:latest
โ Multi-Stage Builds:
Use multi-stage builds to separate the build environment from the runtime environment.
The final image only contains the necessary artifacts, reducing its overall size.
Example:
FROM builder as build
# Build stage commands
FROM alpine:latest
COPY --from=build /app /app
โ Minimize Layers:
Combine multiple commands into a single
RUN
instruction to reduce the number of layers.Clean up temporary files and cache within the same
RUN
command.
Example:
RUN apt-get update && \
apt-get install -y package && \
apt-get clean && \
rm -rf /var/lib/apt/lists/*
โ Use .dockerignore:
Exclude unnecessary files and directories from the build context using a
.dockerignore
file.This reduces the amount of data sent to the Docker daemon during the build.
Example .dockerignore
:
node_modules
.git
โ Remove Unnecessary Dependencies:
Remove unnecessary packages and dependencies after they are no longer needed.
This is especially important when installing development dependencies during the build process.
Example:
RUN npm install --production && \
npm prune
โ Optimize Dockerfile Instructions:
Be mindful of the order of instructions. Place frequently changing instructions later in the Dockerfile to maximize caching benefits.
Avoid unnecessary or redundant instructions.
Example:
# Bad: Frequent changes to code will invalidate the cache
COPY . /app
RUN npm install
# Good: Only copy package.json first (frequently changing) and install dependencies
COPY package.json /app
RUN npm install
COPY . /app
โ Use Smaller Package Variants:
- Choose smaller and more specialized variants of packages, libraries, and tools when available.
Example with Alpine Linux:
RUN apk add --no-cache openssl
โ Clean Up:
- Remove unnecessary or temporary files within the Dockerfile to reduce the final image size.
Example:
RUN apt-get purge -y --auto-remove
โ Compress Files and Layers:
Compress files within the image when applicable to reduce their size.
Use a tool like
docker-squash
to merge layers and compress the image further.
Example:
FROM alpine:latest as intermediate
# Build stage commands
FROM alpine:latest
COPY --from=intermediate /app /app
By employing these strategies, you can significantly reduce the size of your Docker images, making them more efficient and faster to deploy.
Why and when to use Docker?
Docker is a popular platform for containerization, providing a way to package, distribute, and run applications and their dependencies in isolated environments called containers. Here are some reasons why and scenarios when you might want to use Docker:
Why use Docker:
Consistency Across Environments:
- Docker containers encapsulate the application and its dependencies, ensuring consistency across different environments, from development to testing to production.
Isolation:
- Containers provide isolation for applications, avoiding conflicts between dependencies and ensuring that an application runs consistently regardless of the host system.
Portability:
- Docker containers can run on any system that supports Docker, providing excellent portability. This facilitates seamless deployment across various cloud providers, on-premises servers, and developer machines.
Resource Efficiency:
- Containers share the host system's kernel, which makes them lightweight compared to traditional virtual machines. This results in efficient resource utilization and faster startup times.
Microservices Architecture:
- Docker is well-suited for a microservices architecture, where applications are broken down into smaller, independent services that can be developed, deployed, and scaled independently.
Rapid Deployment:
- Containers can be started or stopped quickly, allowing for rapid deployment and scaling based on demand. This is especially beneficial in dynamic and auto-scaling environments.
Version Control:
- Docker images are versioned, making it easy to roll back to previous versions or deploy specific versions of an application. This enhances version control and reproducibility.
Continuous Integration/Continuous Deployment (CI/CD):
- Docker facilitates CI/CD pipelines by providing a consistent environment for building, testing, and deploying applications. Containers can be easily integrated into CI/CD workflows.
DevOps Practices:
- Docker aligns well with DevOps principles, enabling collaboration between development and operations teams. It promotes infrastructure as code and accelerates the development-to-production lifecycle.
Application Isolation:
- Docker containers isolate applications and their dependencies, reducing the risk of conflicts between different software components.
When to use Docker:
Multi-Platform Development:
- When working on projects that need to run consistently across different development machines, testing environments, and production servers.
Microservices Architecture:
- For applications designed as a collection of small, independent services that can be developed, deployed, and scaled independently.
Environment Standardization:
- When there is a need to standardize and reproduce development and deployment environments, reducing the "it works on my machine" problem.
Scaling Applications:
- When dealing with applications that require scaling horizontally to handle varying workloads or traffic spikes.
Resource Efficiency:
- In resource-constrained environments or when there's a need for efficient use of system resources.
Rapid Prototyping:
- For quickly setting up and tearing down development and testing environments, facilitating rapid prototyping and experimentation.
Legacy Application Modernization:
- When modernizing legacy applications by containerizing them, making them easier to maintain, deploy, and scale.
Continuous Integration/Continuous Deployment (CI/CD):
- In CI/CD pipelines to create reproducible and consistent build and deployment environments.
Collaboration Across Teams:
- When multiple teams or stakeholders are involved in the development, testing, and deployment of an application, Docker provides a common and consistent environment.
Application Isolation and Security:
- When there is a need for isolating applications and enhancing security by encapsulating dependencies within containers.
Docker is a versatile tool with a wide range of applications, and its usage can be beneficial in various scenarios depending on the specific needs and requirements of a project or organization.
Explain the Docker components and how they interact with each other.
The main components of Docker include:
Docker Daemon:
The Docker Daemon (dockerd) is a background process that manages Docker containers on a host system. It listens for Docker API requests and manages Docker objects, such as images, containers, networks, and volumes.
The Docker Daemon communicates with the Docker CLI (Command-Line Interface) and other Docker components to execute container-related commands.
Docker CLI:
The Docker CLI is the command-line interface that allows users to interact with the Docker Daemon. Users issue commands to the CLI to build, manage, and interact with containers and other Docker objects.
Common commands include
docker run
,docker build
,docker ps
, and many others.
Docker Images:
Docker Images are lightweight, standalone, and executable packages that include everything needed to run a piece of software, including the code, runtime, libraries, and system tools.
Images are typically created from a Dockerfile, which contains instructions for building the image layer by layer.
Docker Containers:
Docker Containers are running instances of Docker Images. They encapsulate the application and its dependencies, providing isolation from the host system and other containers.
Containers are created from images and can be started, stopped, moved, and deleted.
Docker Compose:
Docker Compose is a tool for defining and running multi-container Docker applications. It allows you to define a multi-container environment in a YAML file, specifying the services, networks, and volumes.
With a single command (
docker-compose up
), you can start and orchestrate multiple containers defined in the Docker Compose configuration.
Docker Registry:
A Docker Registry is a storage and distribution system for Docker images. It allows you to push and pull Docker images to and from a central repository.
Docker Hub is a public registry that is commonly used, but organizations may set up private registries for security and control.
Docker Network:
Docker Networks provide communication between containers running on the same host or across multiple hosts. It enables containers to discover and communicate with each other using DNS names or IP addresses.
Common network drivers include bridge, host, overlay, and macvlan.
Docker Volumes:
Docker Volumes are used to persist data generated by containers. They provide a way to share data between containers and between the host and containers.
Volumes are often used for databases, logs, and other data that needs to survive container restarts.
Here's how these components interact with each other:
The Docker Daemon runs as a background process on the host system and manages containers, images, networks, and volumes.
Users interact with the Docker Daemon using the Docker CLI, issuing commands to perform operations on containers and images.
Docker Images are built from Dockerfiles and stored in the host's local image cache. They can also be pushed to and pulled from Docker Registries.
Docker Containers are created from Docker Images and run on the host system. They can communicate with each other using Docker Networks.
Docker Compose allows the definition and orchestration of multi-container applications using a single configuration file.
Docker Volumes provide persistent storage for containers, allowing data to be shared and preserved across container restarts.
Explain the terminology: Docker Compose, Docker File, Docker Image, Docker Container?
Docker Compose:
Definition: Docker Compose is a tool that allows you to define and run multi-container Docker applications. It uses a YAML file to specify the services, networks, and volumes required for a complete application stack.
Purpose: Docker Compose simplifies the process of defining, configuring, and orchestrating multiple Docker containers that work together as a single application.
Example docker-compose.yml
file:
version: '3'
services:
web:
image: nginx:latest
database:
image: mysql:latest
Dockerfile:
Definition: A Dockerfile is a script that contains instructions for building a Docker image. It specifies the base image, environment variables, commands to run, and other configurations needed to create the image.
Purpose: Dockerfiles provide a reproducible and automated way to build Docker images, ensuring consistency across different environments and deployments.
Example Dockerfile
:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
CMD ["npm", "start"]
Docker Image:
Definition: A Docker image is a lightweight, standalone, and executable package that includes everything needed to run a piece of software, such as code, runtime, libraries, and system tools.
Purpose: Docker images provide a portable and consistent environment, ensuring that an application runs consistently across different systems and environments.
Example commands:
# Build an image from a Dockerfile
docker build -t myapp:latest .
# Pull an image from Docker Hub
docker pull nginx:latest
Docker Container:
Definition: A Docker container is a running instance of a Docker image. It encapsulates an application and its dependencies in an isolated environment, providing consistency and reproducibility.
Purpose: Docker containers enable the deployment, scaling, and isolation of applications. They can be started, stopped, moved, and deleted easily.
Example commands:
# Run a container from an image
docker run -d --name myapp myapp:latest
# List running containers
docker ps
# Stop and remove a container
docker stop myapp
docker rm myapp
In what real scenarios have you used Docker? Docker finds applications in various real-world scenarios:
Application Deployment: Docker simplifies application deployment by providing consistency across different environments and ensuring that dependencies are encapsulated within containers.
Microservices Architecture: In microservices-based architectures, Docker containers enable the deployment and scaling of individual microservices independently.
Continuous Integration/Continuous Deployment (CI/CD): Docker is integral to CI/CD pipelines, facilitating automated testing, building, and deployment of applications.
DevOps Practices: Docker aligns with DevOps practices, allowing teams to collaborate efficiently, automate workflows, and ensure smooth delivery of software.
Isolation for Development: Developers use Docker to create isolated development environments, ensuring that their applications run consistently across different stages of development.
Docker vs Hypervisor?
Docker and hypervisors are both technologies that provide virtualization, but they operate at different levels of the technology stack and serve different purposes.
Docker:
Containerization:
- Docker uses containerization, a lightweight form of virtualization, to package applications and their dependencies together. Containers share the host system's kernel, making them more lightweight and efficient compared to traditional virtualization.
Isolation:
- Containers provide process and file system isolation, allowing applications to run in isolated environments without the need for a full operating system virtualization.
Resource Efficiency:
- Docker containers are more resource-efficient compared to virtual machines (VMs) because they do not require a separate operating system for each instance. They share the host OS kernel, reducing overhead.
Portability:
- Docker containers are highly portable, allowing developers to package an application and its dependencies into a container, which can then run consistently across different environments.
Start-up Time:
- Containers start quickly since they don't need to boot an entire operating system. This makes them suitable for dynamic and scalable environments.
Hypervisor (Virtual Machine):
Virtualization:
- Hypervisors create and manage virtual machines (VMs) that run complete operating systems. Each VM is an independent instance with its own kernel, allowing it to run different operating systems on the same physical hardware.
Isolation:
- VMs provide strong isolation between different instances, as each VM runs its own operating system. This isolation is suitable for scenarios where stronger security boundaries are required.
Resource Overhead:
- VMs have higher resource overhead compared to containers because each VM includes a full operating system, contributing to increased memory and storage usage.
Portability:
- VMs are less portable than containers. While virtual machines can be moved between hypervisors that support the same virtualization technology (e.g., VMware to VMware), they are not as easily portable as Docker containers.
Start-up Time:
- VMs generally have longer start-up times compared to containers because they involve booting an entire operating system.
Use Cases:
Docker:
Ideal for lightweight, portable, and scalable applications.
Well-suited for microservices architectures.
Efficient for development, testing, and deployment in dynamic environments.
Hypervisor:
Suitable for scenarios requiring strong isolation between virtual machines.
Commonly used in traditional virtualization setups for running multiple operating systems on a single physical server.
Well-suited for scenarios with diverse operating system requirements.
What are the advantages and disadvantages of using docker?
Advantages:
Portability: Docker containers are highly portable, running consistently across different environments.
Isolation: Containers provide process and file system isolation, ensuring applications do not interfere with each other.
Efficiency: Docker containers are lightweight and share the host system's kernel, leading to efficient resource utilization.
Consistency Across Environments: Docker ensures consistency between development, testing, and production environments.
Scalability: Docker allows easy scaling by deploying multiple instances of containers.
Disadvantages:
Learning Curve: Docker has a learning curve, especially for those new to containerization concepts and Docker-specific commands.
Security Concerns: Improperly configured or insecure container images can pose security risks.
Persistence: Containers are typically designed to be ephemeral, and handling persistent storage can be challenging.
Resource Overhead: While more efficient than VMs, containers still introduce some resource overhead.
Networking Complexity: Configuring and managing networking between containers and external services can be complex.
Compatibility Issues: Some applications may not be suitable for containerization due to dependencies, licensing issues, or compatibility constraints.
Tooling Ecosystem: The rapid evolution of container orchestration tools can lead to compatibility challenges.
Limited Windows Support: While improving, Docker's roots in Linux mean that some features may be less mature on Windows.
What is a Docker namespace?
In Docker, a namespace is a feature of the Linux kernel that provides isolation for various system resources. Namespaces allow multiple processes to run on a system, each with its own isolated view of system resources. Docker leverages namespaces to provide containerization and isolation between containers.
Docker uses several types of namespaces to isolate different aspects of a container's runtime environment. Some key Docker namespaces include:
PID Namespace:
Purpose: Isolates the process IDs (PIDs) of containers, ensuring that processes within a container are unaware of processes in other containers or the host system.
Effect: Each container has its own PID namespace, and processes inside the container are assigned PIDs relative to the container's namespace.
Network Namespace:
Purpose: Isolates network interfaces, routing tables, and network-related resources.
Effect: Containers have their own network namespace, making them isolated from each other and from the host system. Each container has its own network stack, including its own network interfaces and IP addresses.
Mount Namespace:
Purpose: Isolates the file system mount points. Containers can have their own file system views without affecting other containers or the host.
Effect: Containers have their own mount namespace, allowing them to have a separate file system hierarchy. This enables the use of container images and the isolation of file systems between containers.
UTS Namespace:
Purpose: Isolates hostname and domain name identifiers.
Effect: Each container has its own UTS namespace, allowing it to have its own hostname and domain name. This isolation is useful for preventing naming conflicts between containers.
IPC Namespace:
Purpose: Isolates inter-process communication (IPC) resources, such as System V IPC objects (shared memory segments, semaphores, and message queues).
Effect: Containers have their own IPC namespace, preventing interference between processes in different containers.
What is a Docker registry?
A Docker registry is a centralized repository for storing and distributing Docker images. It serves as a place to host and share Docker images, allowing users to pull images from the registry to their local machines or push images to share with others. Docker images are typically versioned and can be easily retrieved and deployed from a registry.
Key points about Docker registries:
Public Registries:
- Public Docker registries are openly accessible to the public. Docker Hub is one of the most well-known public registries, providing a vast collection of pre-built Docker images for various applications and services.
Example pull command from Docker Hub:
docker pull ubuntu:latest
Private Registries:
- Organizations often use private Docker registries to host proprietary or sensitive images. Private registries provide controlled access and additional security for managing and distributing custom Docker images within an organization.
Example pull command from a private registry:
docker pull registry.example.com/myimage:latest
Docker Hub:
- Docker Hub is the default public registry maintained by Docker, Inc. It hosts a vast number of official images and community-contributed images for various software applications, operating systems, and development stacks.
Creating a Custom Registry:
- Organizations can set up their own custom Docker registry to host private images. Docker provides an official image called
registry
that can be used to run a simple, self-hosted registry.
- Organizations can set up their own custom Docker registry to host private images. Docker provides an official image called
Example of running a local registry:
docker run -d -p 5000:5000 --name myregistry registry:2
Pushing and Pulling Images:
- Docker images can be pushed to a registry to make them available for others or pulled from a registry to deploy on a local machine or another environment.
Example push command to a private registry:
docker push registry.example.com/myimage:latest
Example pull command from a private registry:
docker pull registry.example.com/myimage:latest
Image Tagging:
- Docker images are often tagged with a version or label, allowing users to specify a particular version of an image when pulling or pushing.
Example tagging and pushing an image:
docker tag myimage:latest registry.example.com/myimage:v1.0
docker push registry.example.com/myimage:v1.0
What is an entry point?
In the context of Docker, an "entry point" refers to the command or executable that is run when a container starts. It specifies the default command that should be executed when the container is launched. The entry point is defined in the Dockerfile using the
ENTRYPOINT
instruction.Here's the basic syntax for the
ENTRYPOINT
instruction in a Dockerfile:ENTRYPOINT ["executable", "param1", "param2", ...]
The
executable
is the command or program that will be run when the container starts.The optional parameters (
param1
,param2
, ...) are arguments passed to the executable.
For example, if you have a Dockerfile for a web server and you want the container to start the server when it launches, you might use ENTRYPOINT
like this:
FROM nginx:latest
# Copy configuration files, etc.
# Set the default command to start nginx
ENTRYPOINT ["nginx", "-g", "daemon off;"]
In this example, the nginx
executable is specified as the entry point, and it is given the command-line arguments to run in daemon mode (-g "daemon off;"
). When a container is started from this image, it will automatically run the specified nginx
command with the provided arguments.
It's important to note that the ENTRYPOINT
instruction is often used in conjunction with the CMD
instruction. If a Docker image includes both ENTRYPOINT
and CMD
, the command specified in CMD
will be passed as arguments to the command specified in ENTRYPOINT
.
FROM nginx:latest
# Copy configuration files, etc.
# Set the default command to start nginx
ENTRYPOINT ["nginx", "-g", "daemon off;"]
# Additional command-line arguments that can be overridden when running the container
CMD ["-c", "/etc/nginx/nginx.conf"]
When running a container from an image with an entry point, you can override the entry point and provide a different command by specifying it on the command line:
docker run mynginx-container -c /path/to/custom/nginx.conf
In this example, -c /path/to/custom/nginx.conf
becomes an argument passed to the nginx
command specified in the ENTRYPOINT
.
How to implement CI/CD in Docker?
Implementing Continuous Integration (CI) and Continuous Deployment (CD) with Docker involves automating the build, test, and deployment processes to ensure that changes in the codebase are efficiently and reliably delivered to production. Docker provides a containerized environment that is conducive to CI/CD practices. Here are the key steps to implement CI/CD in Docker:
Continuous Integration (CI):
Version Control System (VCS):
- Use a version control system like Git to manage the source code. CI starts with changes committed to the VCS.
Automated Builds with Dockerfile:
- Write a Dockerfile to define the application environment and dependencies. Set up automated builds to trigger when changes are pushed to the VCS. Services like Docker Hub, GitLab CI, or GitHub Actions can be used for this purpose.
Automated Tests:
- Include automated tests in the Docker image to ensure the reliability of the application. Tests can include unit tests, integration tests, and other types of checks depending on the application.
# Example Dockerfile with automated tests
FROM node:14
WORKDIR /app
COPY . .
# Run tests
RUN npm install && npm test
CI Server Integration:
- Use a CI server (e.g., Jenkins, GitLab CI, Travis CI, CircleCI) to orchestrate the CI pipeline. Configure the CI server to trigger builds on code commits and execute the defined build and test steps.
Continuous Deployment (CD):
Artifact Creation:
- Upon successful completion of CI, create a Docker image as an artifact. Tag the image with a version or commit hash for traceability.
docker build -t myapp:latest .
Docker Registry:
- Push the Docker image to a Docker registry. Docker Hub, AWS ECR, Google Container Registry (GCR), or a private registry can be used.
docker push myregistry/myapp:latest
Infrastructure as Code (IaC):
- Define infrastructure as code (e.g., using tools like Terraform, AWS CloudFormation) to manage the deployment environment. This ensures consistency and reproducibility across different environments.
Orchestration with Docker Compose or Kubernetes:
- Use Docker Compose for simpler deployments or Kubernetes for more complex orchestrations. Define deployment configurations to manage the deployment, scaling, and updating of containers.
CD Server Integration:
- Integrate a CD server (e.g., Jenkins, GitLab CI, Argo CD) to automate the deployment pipeline. Configure the CD server to trigger deployments when new artifacts are available.
Rolling Deployments:
- Implement rolling deployments to ensure zero-downtime updates. Strategies like blue-green deployments or canary releases can be employed based on the application requirements.
Monitoring and Rollback:
Monitoring:
- Implement monitoring and logging in the deployed containers. Tools like Prometheus, Grafana, ELK Stack, or cloud-native solutions can be used to gain insights into the application's performance.
Rollback Mechanism:
- Implement a rollback mechanism in case of deployment failures. This could involve versioning, automated testing of the deployment, and the ability to revert to a previous version quickly.
Will data on the container be lost when the docker container exits?
By default, data within a Docker container does not persist once the container exits. Docker containers are designed to be stateless, meaning that any changes made to the container's file system or data are not preserved when the container stops or is removed.
When a container exits, the changes made during its runtime, such as file modifications, database updates, or any other data written to the container's file system, are discarded. The container returns to its initial state, as defined by its Docker image.
To persist data between container runs, Docker provides several mechanisms:
Volumes:
- Docker volumes are the recommended way to persist data generated by a container. Volumes are separate from the container file system and can be mounted into one or more containers. Data stored in volumes persists even if the container is removed.
Example using a named volume:
docker run -d --name myapp -v mydata:/app/data myimage:latest
In this example, the /app/data
directory inside the container is mounted to the named volume mydata
.
Bind Mounts:
- Bind mounts allow you to mount a directory from the host machine into the container. Data written to the bind-mounted directory is persisted on the host.
Example using a bind mount:
docker run -d --name myapp -v /path/on/host:/app/data myimage:latest
In this example, the /path/on/host
directory on the host machine is mounted to the /app/data
directory inside the container.
Docker Compose Volumes:
- If you're using Docker Compose, you can define volumes in your
docker-compose.yml
file to persist data between container runs.
- If you're using Docker Compose, you can define volumes in your
Example using Docker Compose volumes:
version: '3'
services:
myapp:
image: myimage:latest
volumes:
- mydata:/app/data
volumes:
mydata:
Here, the named volume mydata
is defined in the volumes
section of the Docker Compose file and then mounted into the myapp
service.
What is a Docker swarm?
Docker Swarm is a native clustering and orchestration solution for Docker containers. It enables the creation and management of a swarm of Docker nodes, turning them into a single virtual Docker host. This allows for the deployment and scaling of containerized applications across multiple machines in a simplified and efficient manner.
Key features of Docker Swarm include:
Node Clustering:
- Docker Swarm allows multiple Docker hosts to be joined into a cluster, forming a swarm. Each host in the swarm is referred to as a "node." Nodes can be physical machines or virtual machines.
Service Deployment:
- Swarm provides a declarative service model for deploying and managing services. A service is a scalable and distributed application that runs on the swarm. It can be composed of multiple containers.
Load Balancing:
- Swarm automatically load-balances incoming requests across containers within a service. This ensures that the application is highly available and can handle increased traffic.
Scalability:
- Services can be scaled up or down by adjusting the desired number of replicas. Docker Swarm automatically distributes replicas across the available nodes in the swarm.
docker service scale myapp=5
Rolling Updates:
- Swarm supports rolling updates for services. This allows for updating a service to a new version without downtime by gradually replacing old containers with new ones.
docker service update --image newimage:latest myapp
Service Discovery:
- Swarm provides an integrated DNS-based service discovery mechanism. Each service is accessible via its service name, and the Swarm's internal DNS resolves the service name to the appropriate container IP address.
curl http://myapp:8080
Secrets Management:
- Swarm provides a secure way to manage sensitive information, such as API keys or passwords, using the secrets management feature. Secrets can be securely distributed to services.
Swarm Mode:
- Docker Swarm operates in "swarm mode," which was introduced in Docker 1.12. Swarm mode simplifies the setup and management of a swarm by integrating swarm capabilities directly into the Docker Engine.
Overlay Networking:
- Swarm supports overlay networking, allowing containers in the swarm to communicate with each other regardless of the host they are running on. This enables the creation of multi-node, multi-container applications.
Docker Swarm is an integrated part of the Docker ecosystem and provides a built-in solution for orchestrating and managing containerized applications at scale. While other orchestration tools like Kubernetes are widely used, Docker Swarm is a good choice for users who prefer a simpler and more lightweight solution that is tightly integrated with Docker.
What are the docker commands for the following:
Certainly! Here are the Docker commands for the tasks you've mentioned:
View Running Containers:
docker ps
- This command lists the currently running Docker containers, showing information such as container ID, names, status, ports, etc.
Run a Container Under a Specific Name:
docker run --name my_container_name my_image:tag
- Replace
my_container_name
with the desired name andmy_image:tag
with the image and tag you want to run.
Export a Docker Container:
docker export my_container > my_container.tar
- This command exports the file system of the specified container (
my_container
) to a tarball (my_container.tar
).
Import an Already Existing Docker Image:
docker import my_image.tar my_image:tag
- This command imports a previously exported tarball (
my_image.tar
) as a Docker image with the specified tag (my_image:tag
).
Delete a Container:
docker rm my_container
- This command removes a specific container (
my_container
). Add the-f
option to force removal even if the container is running.
Remove All Stopped Containers, Unused Networks, Build Caches, and Dangling Images:
docker system prune
- This command cleans up the Docker system by removing stopped containers, unused networks, dangling images, and build caches. It's a useful command for reclaiming disk space.
Caution: Be careful when using docker system prune
as it removes unused data, including stopped containers and unused images. Ensure you won't lose important data before executing this command.
Remember to adapt these commands based on your specific use case and requirements. Always replace placeholders like my_container
, my_image
, my_container_name
, and my_image:tag
with your actual container names and image details.
Subscribe to my newsletter
Read articles from Sarika Kamble directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
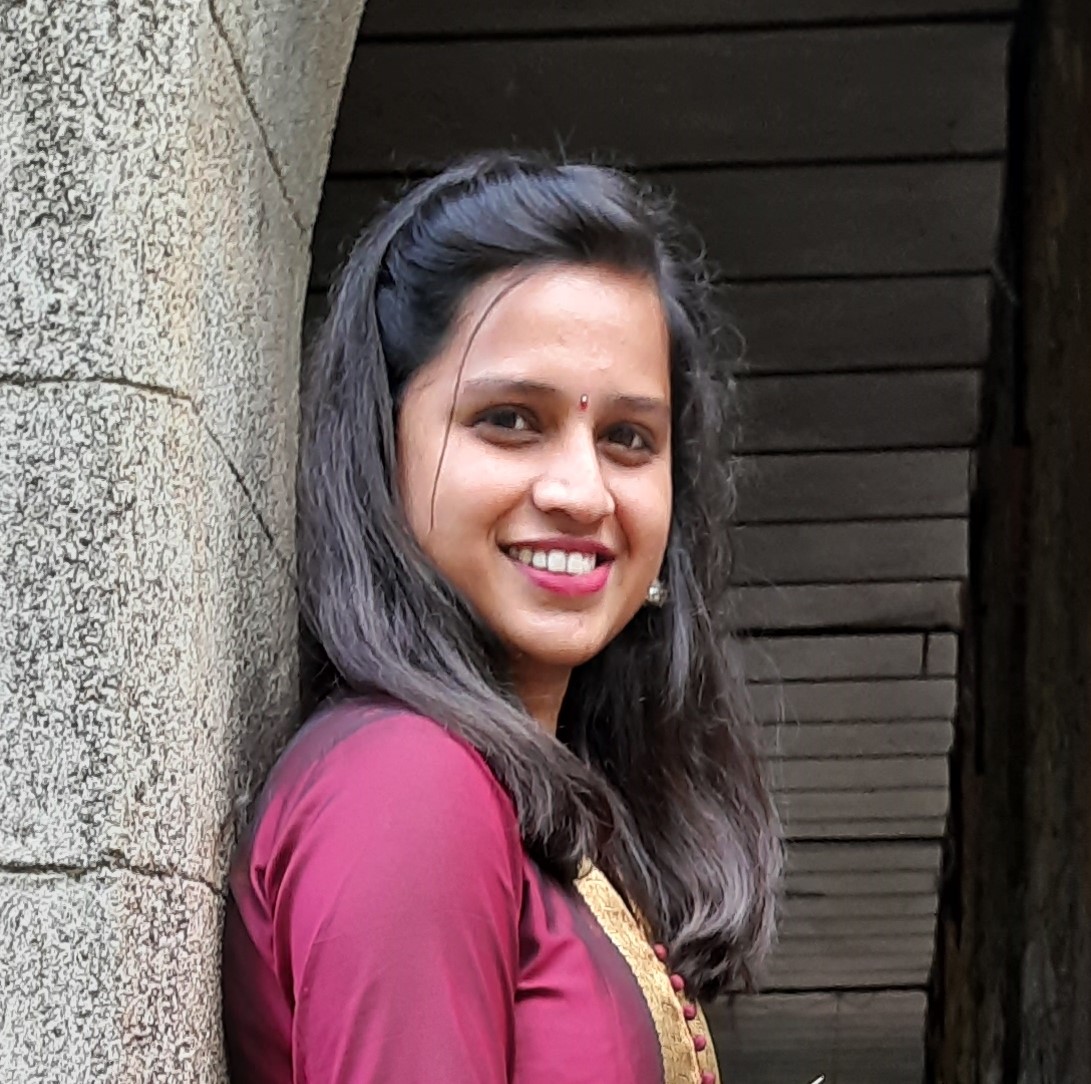
Sarika Kamble
Sarika Kamble
๐ Hello, and welcome to my DevOps journey! ๐ I'm Sarika Kamble, passionate about all things AWS DevOps Technology. Currently, on a learning adventure, I'm here to share my journey and Blogs in the world of cloud and DevOps. I'll be sharing my learnings, experiences, and adventures as I dive deep into the world of continuous integration, automation, and cloud technologies. โ๏ธโ๏ธ Let's connect, learn, and grow as a vibrant DevOps community. Follow my Hashnode blog, and let's embrace the DevOps adventure together! ๐ค๐ Follow me on LinkedIn: https://www.linkedin.com/in/sarika-kamble-3153b3218/