The Known [Problem] with decimals and fractions || Python
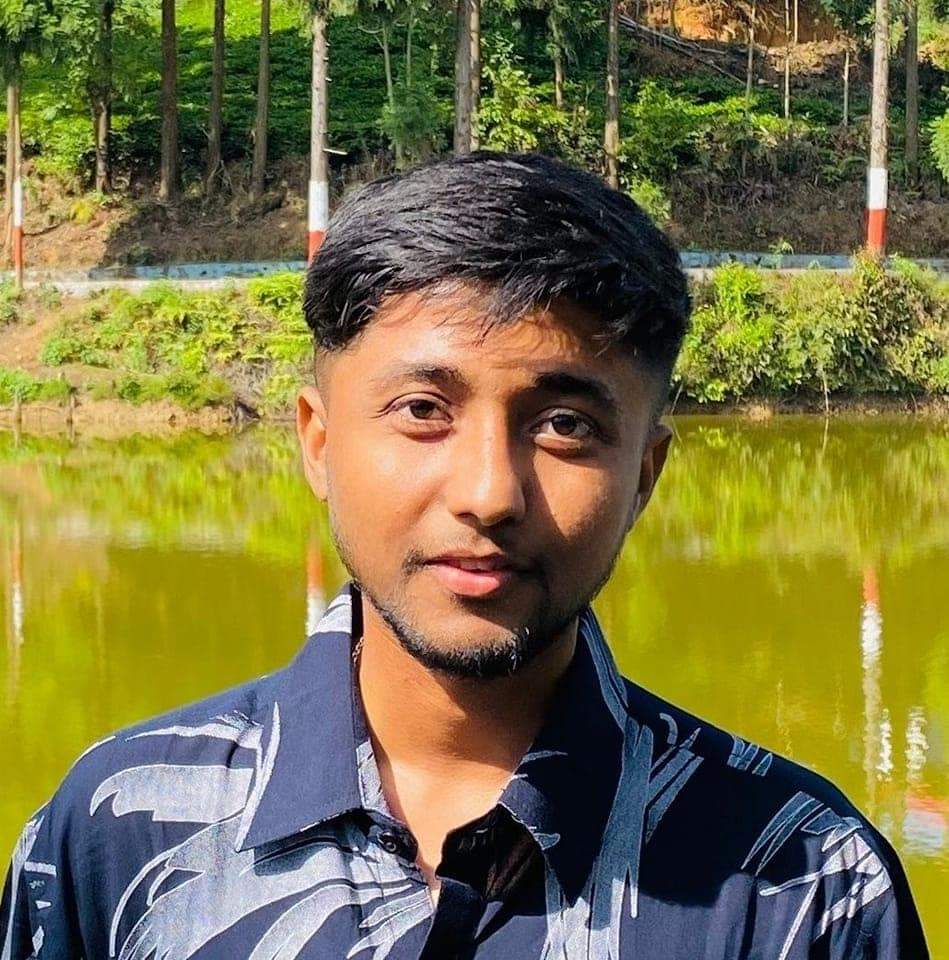
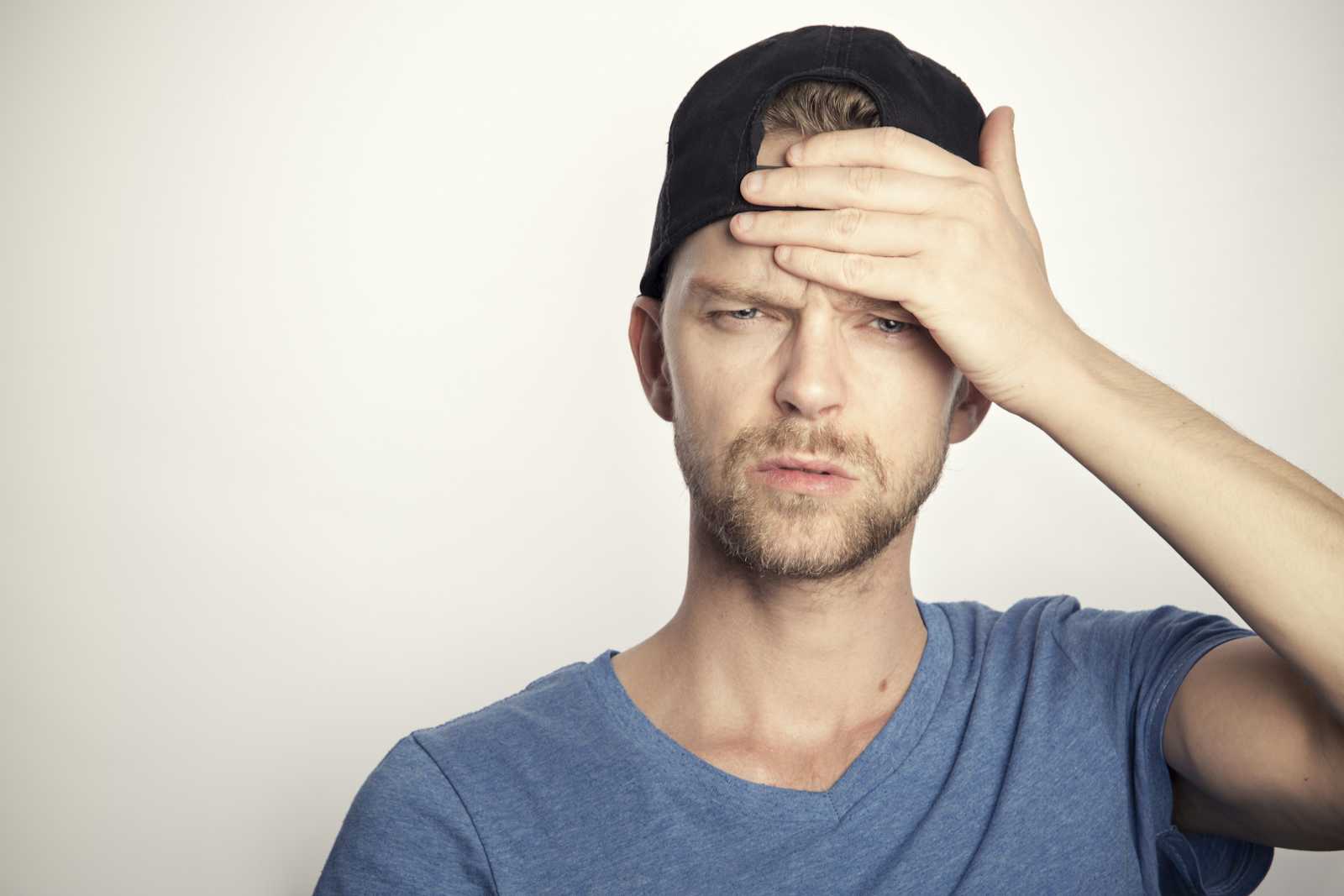
In Python, working with decimals and fractions may lead to precision issues due to the way floating-point numbers are represented in computers. This is not unique to Python but is a general concern in computing due to the limitations of representing real numbers in a finite amount of memory.
When dealing with decimal addition or fraction operations, it's often recommended to use the decimal
module for precise decimal arithmetic and the fractions
module for exact fraction arithmetic.
Here's an example of using the decimal
module for decimal addition:
from decimal import Decimal
# Example decimal addition
num1 = Decimal('0.1')
num2 = Decimal('0.2')
result = num1 + num2
print(result) # Output: 0.3
And here's an example of using the fractions
module for fraction addition:
from fractions import Fraction
# Example fraction addition
frac1 = Fraction(1, 3)
frac2 = Fraction(1, 6)
result = frac1 + frac2
print(result) # Output: 1/2
Using these modules can help mitigate precision issues that might arise with regular floating-point arithmetic. Remember to use the appropriate module based on your specific needs (e.g., decimal
for decimal arithmetic and fractions
for exact fraction arithmetic).
Subscribe to my newsletter
Read articles from Aayush Niraula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
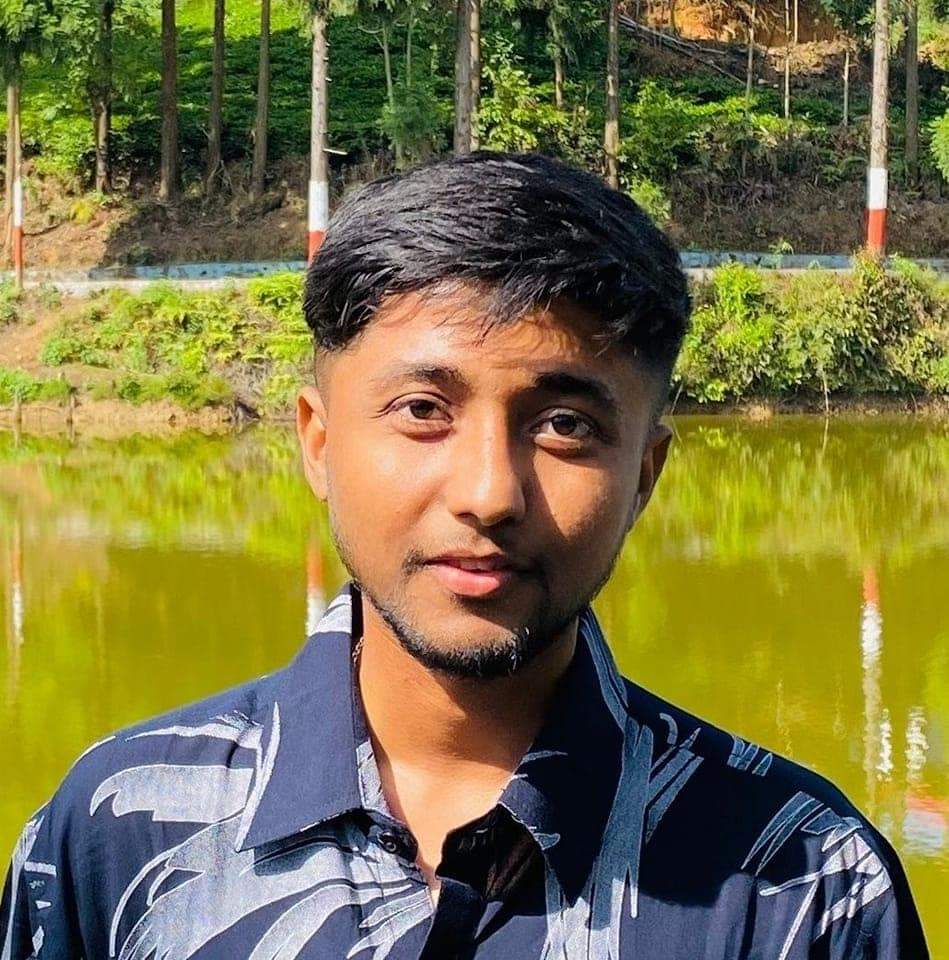
Aayush Niraula
Aayush Niraula
CS student