Understanding and Using the React useReducer Hook
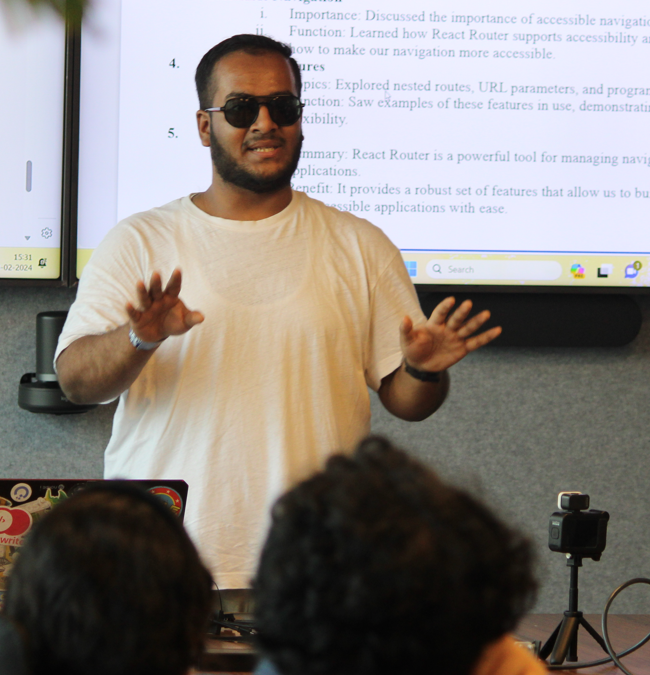
The useReducer
hook in React is a powerful tool for managing complex state logic. It allows you to handle state in a more structured and predictable way, especially when dealing with intricate component behavior. In this blog post, we’ll dive deep into the useReducer
hook, explore its syntax, discuss its optional parameters, provide real-world examples, and highlight best practices.
What is the useReducer
Hook?
Think of useReducer
as a sibling to the familiar useState
hook. While both manage component state, useReducer
offers a different approach. Instead of directly modifying state variables, you define a reducer function that handles state transitions based on actions. Let’s break it down:
Reducer Function: The reducer function takes two arguments: the current state and an action. It then computes the next state based on the action. Essentially, it’s like a state machine that processes actions and updates the state accordingly.
Initial State: When using
useReducer
, you provide an initial state. This state can be an object, an array, or any other data structure. For example,{ count: 0 }
represents an initial state with a counter set to zero.Dispatch Function: The
useReducer
hook returns two values: the state and a dispatch function. The dispatch function allows you to trigger state transitions by dispatching actions.
Now, let’s see some code examples to illustrate how useReducer
works:
Example 1: Simple Counter
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<>
Count: {state.count}
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
</>
);
}
In this example, we create a simple counter using useReducer
. The reducer handles actions like “increment” and “decrement,” updating the state accordingly.
Example 2: More Complex Scenario
Imagine a shopping cart where users can add, remove, or update items. useReducer
shines in such scenarios. Here’s a high-level overview:
Define your initial state (e.g., an empty cart).
Create a reducer that handles actions like “add item,” “remove item,” and “update quantity.”
Dispatch actions to modify the state.
// Simplified example
const initialState = { cartItems: [] };
function cartReducer(state, action) {
switch (action.type) {
case 'ADD_ITEM':
return { cartItems: [...state.cartItems, action.payload] };
case 'REMOVE_ITEM':
// Implement removal logic
return state;
// Other cases for updating quantities, etc.
default:
return state;
}
}
function ShoppingCart() {
const [state, dispatch] = useReducer(cartReducer, initialState);
// Render cart items and dispatch actions
// ...
}
Remember that useReducer
is particularly useful when managing more complex state transitions. It encourages a structured approach and helps prevent state-related bugs.
Subscribe to my newsletter
Read articles from Shashank Rustagi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
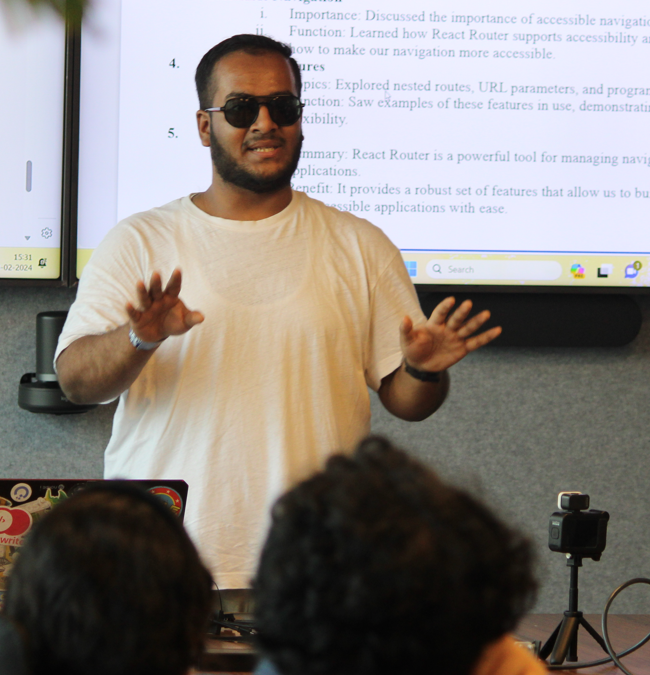
Shashank Rustagi
Shashank Rustagi
SDE2 @Delltech • Mtech CSE'23 @IIITDelhi • Views are personal and does not represent anyone • Travel Vlogger • multi instrumentalist • singer • content creator