#20: Guarding Your Elixir Functions
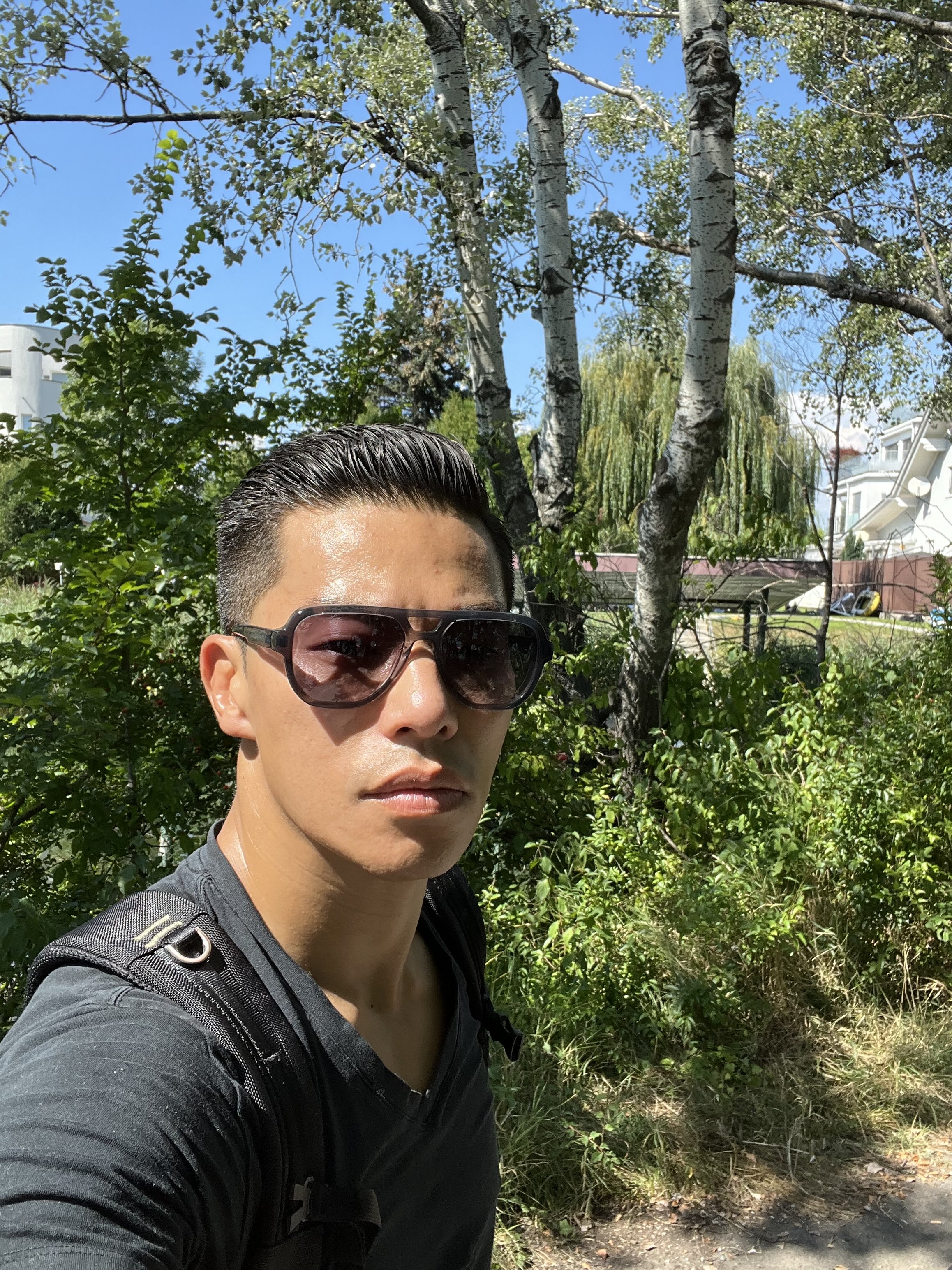
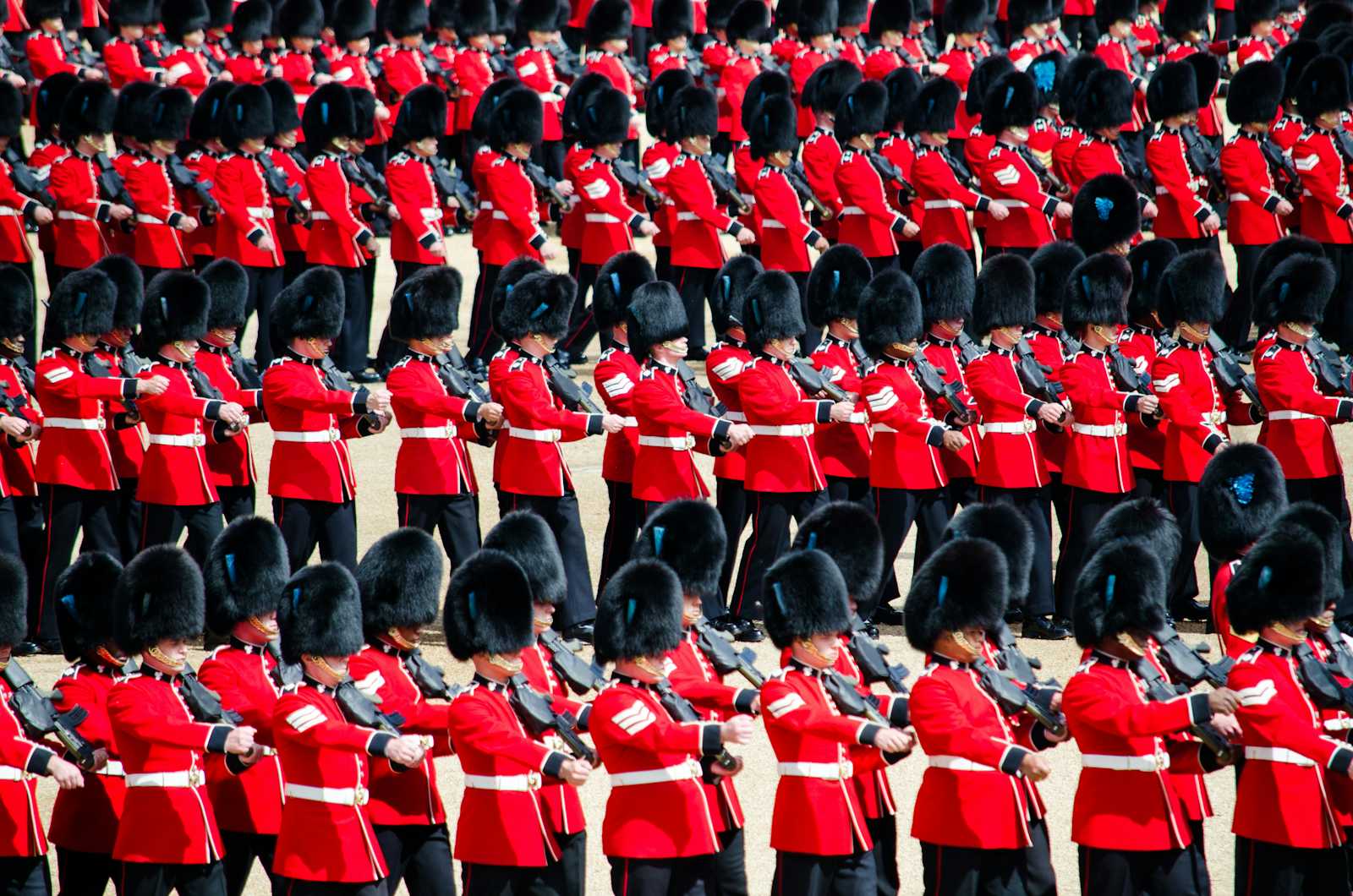
In Elixir, a guard in a function is a special clause that allows you to add additional conditions to the function definition. Guards are used to apply constraints or filters to the function arguments before the function is executed. Guards are denoted by the when
keyword followed by a boolean expression.
Here are two basic examples of a function with a guard in Elixir:
# Basic Example 1
defmodule Math do
def divide(a, b) when b != 0 do
a / b
end
def divide(_, 0) do
{:error, "Division by zero"}
end
end
#Basic Example 2
number = 121
case number do
n when n < 100 -> :low
n when n >= 100 and n < 1000 -> :medium
n when n >= 1000 -> :high
_ -> :unknown
end
In the divide/2
example, the guard when b != 0
ensures that the division operation is only performed when the divisor b
is not equal to zero. If b
is zero, the function pattern matching falls through to the second definition, which handles the case of division by zero.
In the basic example 2, the case
statement evaluates the value of number
and matches it against different patterns with guards. If number
is less than 100, it returns :low
, if it is between 100 and 1000 (inclusive), it returns :medium
, if it is 1000 or greater, it returns :high
. The _
wildcard pattern is used as a catch-all for any other value, returning :unknown
.
Guards can include various expressions such as comparison operators, logical operators, type checks, and function calls. Guards provide a way to add additional conditions to function clauses, making the function definitions more robust and flexible.
Subscribe to my newsletter
Read articles from Stephan Yu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
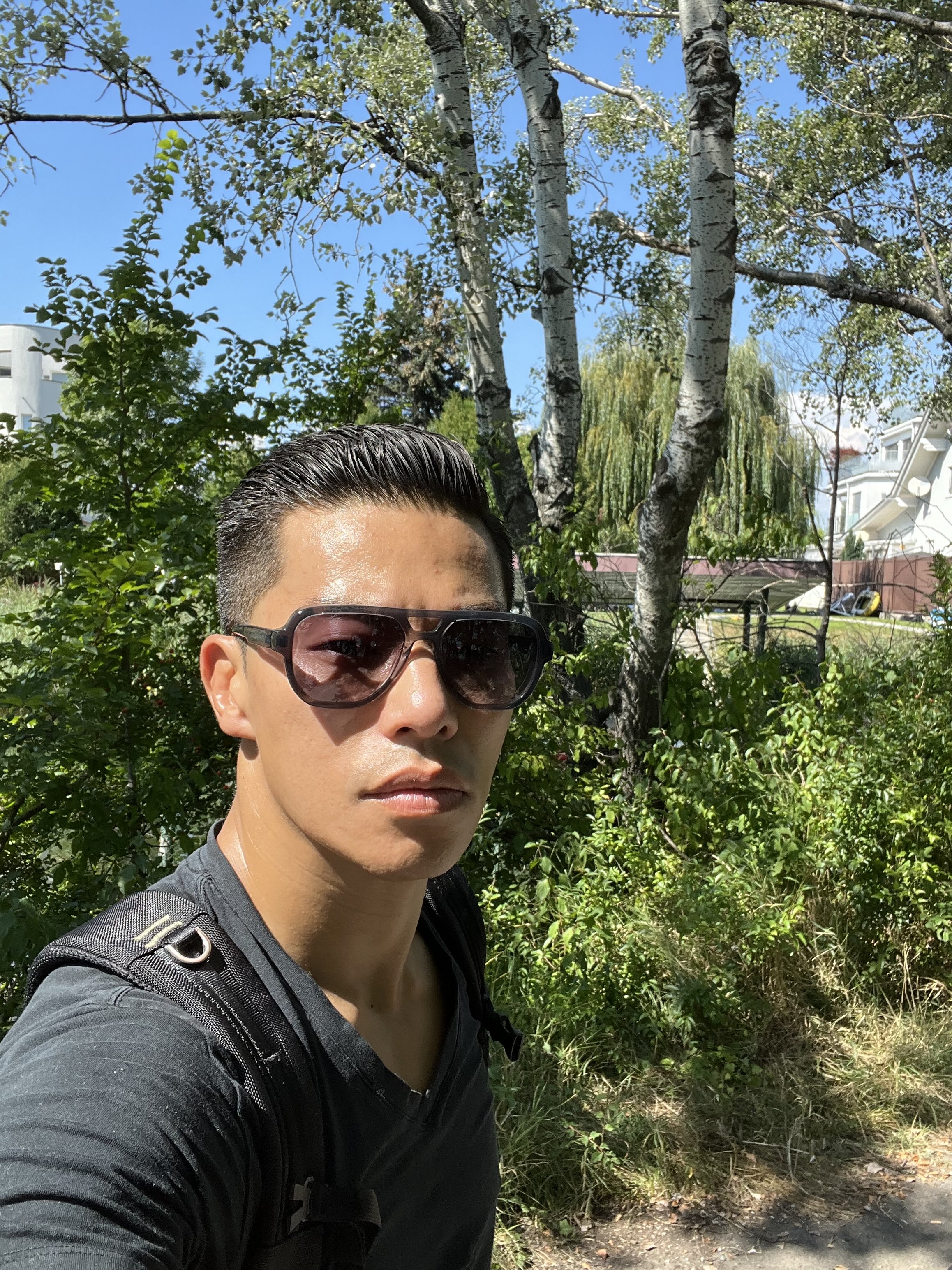
Stephan Yu
Stephan Yu
I am a full-stack developer from Austria who has studied and worked in many countries including the UK, Australia, and the US. I have worked extensively with Ruby on Rails, React, and Typescript, and I am also building projects using Elixir and Phoenix.