Common Data Types in Python
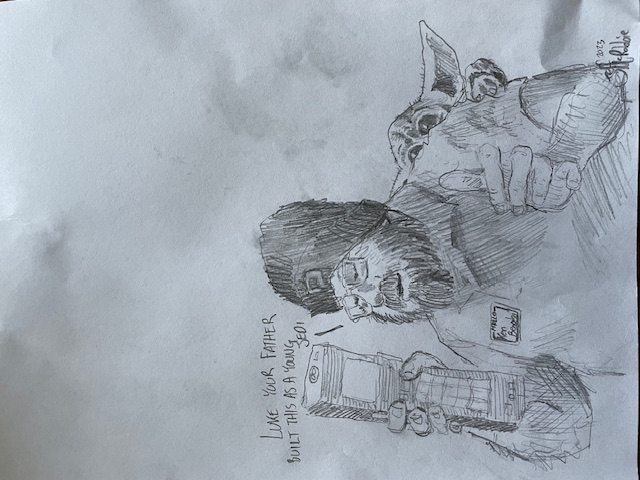
I want to talk about some of the common data types you will see as you code in Python.
First up is strings, if you have coded in JavaScript you already know what a string is, but if you are unfamiliar with this a string is anything that is wrapped in quotes. It can be "double-quotes", or 'single quotes', but in Python you cannot use `backticks ` (they are not valid in Python). You can use string interpolation in Python:
cat_name = "Brewster"
print (f"Say hello to my cat {cat_name}!")
The output will be: "Say hello to my cat Brewster!"
You can also use f strings for other things as well: lets say you want to display prices with two decimal places, here is an example of how we can do this with an f string:
price_1 = 9
price_2 = 4.5
print(f"Item 1 cost ${price_1 : .2f}")
print(f"Item 2 cost ${price_2 : .2f}")
Here the outputs will be:
"Item 1 cost $9.00"
"Item 2 cost $4.50"
Remember: in Python everything is an object!!!
Next up we have integers and floats (we just saw these in our pricing example above). The main difference between an integer and a float is that integers are whole numbers, while floats are numbers that have a decimal in them.
Here is something that is very important to remember in the sequence type of Python: there are [lists] and (tuples), and both can be used to store collections of items, but a list is mutable meaning that after creating a list you can add, remove or modify the elements. With a tuple however once it is created, you cannot change the elements meaning a tuple is immutable.
One thing about tuples in Python you need at least one comma ( 1, ) even if there is only one element.
There are Sets and Dictionaries that also store data, but the individual elements are unique.
Sets are unindexed, unordered and unchangeable . What does that mean? Well unindexed is that we cannot access elements of the set using square brackets as we do in lists and tuples. Unordered is that the contents of the set are in random order.😬 And finally unchangeable which is simply that the individual elements of a set cannot be changed.
Remember that immutable and unchangeable mean different things when talking about data types in Python.
Dictionaries are Python's equivalent of a vanilla JavaScript object. They are comprised of key:value pairs. You can create a dictionary by writing key:value pairs in {curly brackets}, make sure what is in the curly brackets is in a string format. To access data from the dictionary, you can use [square brackets] and pass the symbol for the key to access. Here is an example:
person = { "name": "John", "age": 30, "city": "New York" }
print(person["name"]) # Output: John
print(person["age"] # Output: 30
print(person["city"]) # Output: New York
You can also use the .get() method to retrieve the value for a key. If it returns None then there is no matching key.
Remember: Dot notation does not work in Python like it does in JavaScript, only bracket notation will work to access keys.
You can use the dict() function to create dictionaries in Python, here is an example: dict(x=1, y=2)
In Python when there is an absence of a value, it is represented by None. Unlike JavaScript, Python will not let you create a variable without assigning it a value. You must assign a of None if you want an "empty" variable. Here is an example: no_value = None
There is also boolean values (True and False) in Python just like in JavaScript. When you want to reverse the truth of a value, variable or statement you need to use the not operator. The exclamation point ! still plays a role in Python, but it is only used in the != (not equal to) operator that asserts when two values are not equal. Also just like in JavaScript, Python has many falsey values:
None
: The absence of a value or a null value.0
: The integer zero.0.0
: The floating-point zero.''
: An empty string.[]
: An empty list.()
: An empty tuple.{}
: An empty dictionary.set()
: An empty set.
Just be careful because they do not map perfectly to one another.
This was some of the more common data types and structures that you will see while you are coding in Python. I hope you found this useful, and as always,
Happy coding!!!
Subscribe to my newsletter
Read articles from Kenny McClelland directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
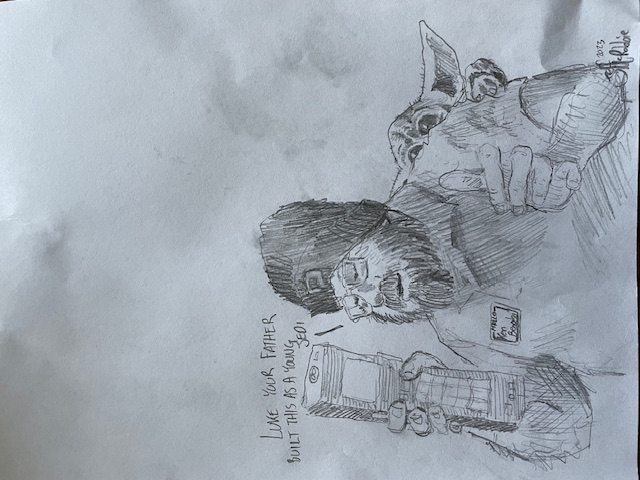
Kenny McClelland
Kenny McClelland
I am a full-time Software Engineering student with Flatiron school, and part-time CSA with Lowe's.