Day 21 : Docker Important interview Questions.

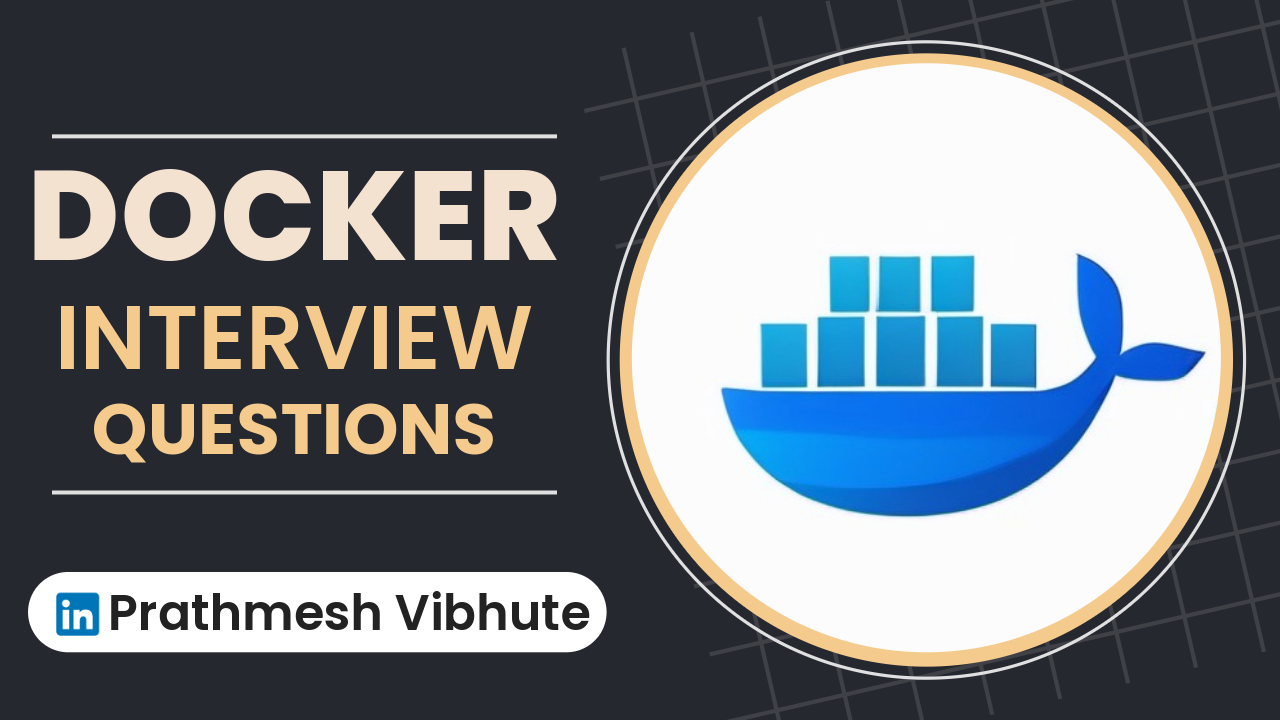
Difference between an Image, Container, and Engine:
Docker Image: A template that contains the necessary files and dependencies to run an application. It's essentially a snapshot of a container.
Docker Container: A running instance of a Docker image. It encapsulates the application along with its dependencies and runtime environment.
Docker Engine: The underlying software that runs and manages Docker containers. It provides an interface for interacting with Docker, managing containers, images, networks, and volumes.
Difference between Docker command COPY vs ADD:
COPY
: Copies files or directories from the host file system into the container file system. It is straightforward and is preferred when you only need to copy local files into the container.ADD
: Similar toCOPY
, but it also supports URLs and automatically extracts compressed files. It's more versatile but can be confusing, soCOPY
is preferred for clarity unless you specifically need the extra functionality.
Difference between Docker command CMD vs RUN:
CMD
: Specifies the default command to run when a container starts. It can be overridden at runtime. It's typically used to specify the main application or process to run within the container.RUN
: Executes commands during the build process to set up the environment for the image and install dependencies. These commands are run only once during image build time.
How to reduce the size of the Docker image:
Use smaller base images (e.g., Alpine Linux instead of Ubuntu).
Minimize the number of layers by combining multiple commands into one during image creation.
Remove unnecessary files and dependencies after installing packages.
Use multi-stage builds to discard build-time dependencies in the final image.
Compress files within the image to reduce its size.
Utilize Docker's build cache efficiently to avoid redundant operations.
Why and when to use Docker:
Docker is used for consistent development, testing, and deployment environments.
It enables isolation, making it easier to manage dependencies and conflicts between applications.
Docker facilitates scalability by allowing the deployment of multiple instances of an application across different environments.
It simplifies the deployment process, making it more efficient and reducing deployment-related issues.
Explanation of Docker components and how they interact:
Docker Engine: Responsible for managing containers, images, networks, and volumes.
Docker Image: A template for creating Docker containers.
Docker Container: A runnable instance of a Docker image.
Docker Compose: A tool for defining and running multi-container Docker applications.
Docker File: A text file that contains instructions for building a Docker image.
Docker Registry: A repository for Docker images where they can be stored and retrieved.
Real scenarios where Docker is used:
Containerizing microservices for easier deployment and scaling.
Creating development environments that closely mimic production.
Continuous integration and continuous deployment (CI/CD) pipelines.
Simplifying application deployment in cloud environments.
Running legacy applications in isolated environments.
Docker vs Hypervisor:
Docker uses containerization to run applications with less overhead compared to hypervisors, which create virtual machines (VMs) with separate operating systems.
Containers share the host OS kernel, making them more lightweight and efficient than VMs.
Docker containers typically start much faster than VMs, as they don't need to boot an entire OS.
Hypervisors provide stronger isolation between VMs since each VM has its own kernel, while containers share the host kernel.
Advantages and disadvantages of using Docker:
Advantages:
Simplifies application deployment and management.
Provides consistent environments across development, testing, and production.
Increases scalability and resource utilization.
Facilitates microservices architecture and container orchestration.
Disadvantages:
Requires learning curve for understanding containerization concepts.
May introduce security risks if containers are not properly configured.
Can lead to increased complexity in networking and storage management in large deployments.
Docker namespace:
Docker namespace provides isolation for various resources such as containers, images, volumes, and networks.
Namespaces ensure that each container operates in its own isolated environment, preventing conflicts with other containers and the host system.
Docker namespaces include PID (process IDs), net (network), ipc (inter-process communication), mnt (mount points), and user (user IDs).
Docker registry:
A Docker registry is a storage and distribution system for Docker images.
It allows users to push and pull images to and from repositories.
Docker Hub is a popular public Docker registry, while organizations can set up their private registries for security and compliance reasons.
Entry point:
The entry point is the command or script that is run when a Docker container starts.
It defines the default behavior of the container and is typically used to launch the main application process.
The entry point can be specified in the Dockerfile using the
ENTRYPOINT
instruction.
Implementing CI/CD in Docker:
CI/CD in Docker involves automating the build, test, and deployment processes using Docker containers.
Docker images can be built and tested within CI/CD pipelines using tools like Jenkins, GitLab CI, or Travis CI.
After successful testing, Docker images can be pushed to a Docker registry.
Docker Compose can be used to define multi-container applications and manage their deployment in various environments.
Data persistence in Docker containers:
Data stored within a Docker container's writable layer is typically lost when the container exits.
To persist data between container restarts, Docker provides mechanisms like volumes and bind mounts.
Volumes are preferred for persisting data because they are managed by Docker and can be shared among multiple containers.
Docker swarm:
Docker Swarm is a native clustering and orchestration tool for Docker containers.
It allows users to create and manage a cluster of Docker hosts, called nodes, as a single virtual system.
Docker Swarm provides features like service discovery, load balancing, and automatic scaling for deploying and managing containerized applications.
Common Docker commands:
View running containers:
docker ps
Run container under a specific name:
docker run --name <name> <image>
Export a Docker container:
docker export <container_id> > container.tar
Import an existing Docker image:
docker import <file> <repository:tag>
Delete a container:
docker rm <container_id>
Remove all stopped containers, unused networks, build caches, and dangling images:
docker system prune
Common Docker practices to reduce image size:
Use lightweight base images.
Minimize the number of layers.
Remove unnecessary files and dependencies.
Utilize multi-stage builds.
Optimize Dockerfile instructions.
Use .dockerignore file to exclude unnecessary files during image build.
I'm confident that this article will prove to be valuable, helping you discover new insights and learn something enriching .
thank you : )
Subscribe to my newsletter
Read articles from Prathmesh Vibhute directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
