Basics of Python for DevOps Engineers
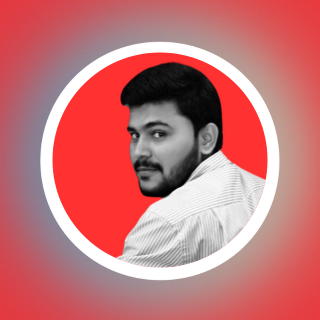
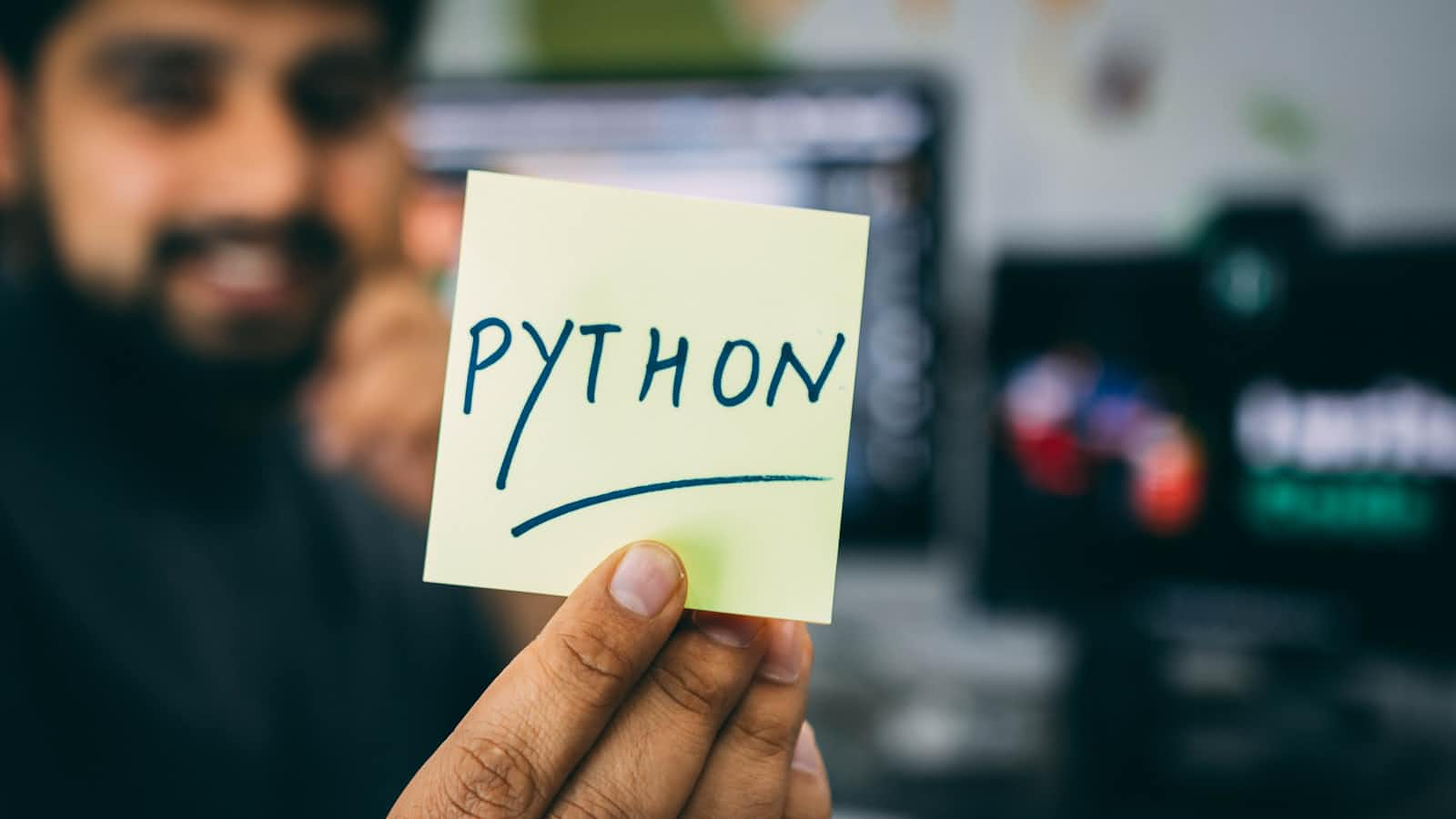
As a DevOps engineer, understanding programming languages is crucial for automating tasks, managing infrastructure, and ensuring smooth software delivery. Python, with its simplicity, versatility, and extensive libraries, is a preferred choice for DevOps professionals. In this article, we’ll explore the basics of Python and its relevance in the DevOps world.
Why Python for DevOps?
Automation: Python excels at automating repetitive tasks. Whether it’s provisioning cloud resources, configuring servers, or deploying applications, Python scripts can handle it efficiently.
Readability: Python’s clean syntax makes it easy to read and understand. This readability is essential when collaborating with other team members or maintaining code.
Rich Libraries: Python boasts a vast ecosystem of libraries (such as
requests
,boto3
, andparamiko
) that simplify tasks like working with APIs, managing databases, and interacting with cloud services.Cross-Platform Compatibility: Python runs on various platforms, including Windows, Linux, and macOS. This flexibility ensures consistency across different environments.
Python Basics
1. Variables and Data Types
Variables: Declare variables without specifying their data type. Python infers the type based on the assigned value.
Python
name = "DevOps Engineer" age = 30
AI-generated code. Review and use carefully. More info on FAQ.
Data Types: Common data types include strings, integers, floats, lists, dictionaries, and booleans.
2. Control Structures
Conditional Statements:
Python
if age >= 18: print("You are an adult.") else: print("You are a minor.")
AI-generated code. Review and use carefully. More info on FAQ.
Loops:
Python
for i in range(5): print(i)
AI-generated code. Review and use carefully. More info on FAQ.
3. Functions
Define functions using
def
:Pythondef greet(name): return f"Hello, {name}!"
AI-generated code. Review and use carefully. More info on FAQ.
4. File I/O
Read from a file:
Python
with open("myfile.txt", "r") as file: content = file.read()
AI-generated code. Review and use carefully. More info on FAQ.
Write to a file:
Python
with open("output.txt", "w") as file: file.write("Hello, world!")
AI-generated code. Review and use carefully. More info on FAQ.
Real-World Use Cases
CI/CD Pipelines: Python scripts automate build, test, and deployment stages in CI/CD pipelines.
Infrastructure as Code (IaC): Tools like Terraform and Ansible use Python for defining infrastructure.
Cloud Automation: Boto3 (Python SDK for AWS) simplifies cloud resource management.
Monitoring and Alerting: Custom scripts can enhance monitoring solutions.
Container Orchestration: Kubernetes operators and Helm charts often involve Python.
Learning Python
Online Tutorials: Websites like Real Python, GeeksforGeeks, and Codecademy offer Python tutorials.
Books: “Automate the Boring Stuff with Python” by Al Sweigart is an excellent resource.
Practice: Solve coding challenges on platforms like LeetCode and HackerRank.
Remember, Python is a tool in your DevOps toolbox. Mastering it will empower you to automate, optimize, and streamline your DevOps workflows.
Related Resources:
Python For DevOps: A Complete Guide For DevOps Engineers by Bibin Wilson
Practical Python for DevOps Engineers LiveLessons by Chris Jackson
Subscribe to my newsletter
Read articles from Sagar Bhosale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
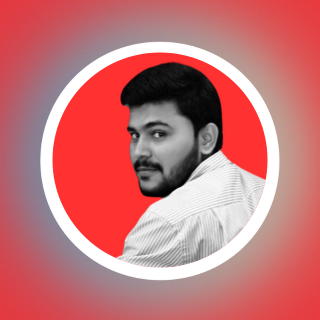