Demystifying Python: A Peek into How Your Code Comes to Life
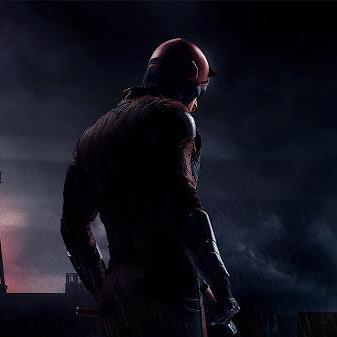
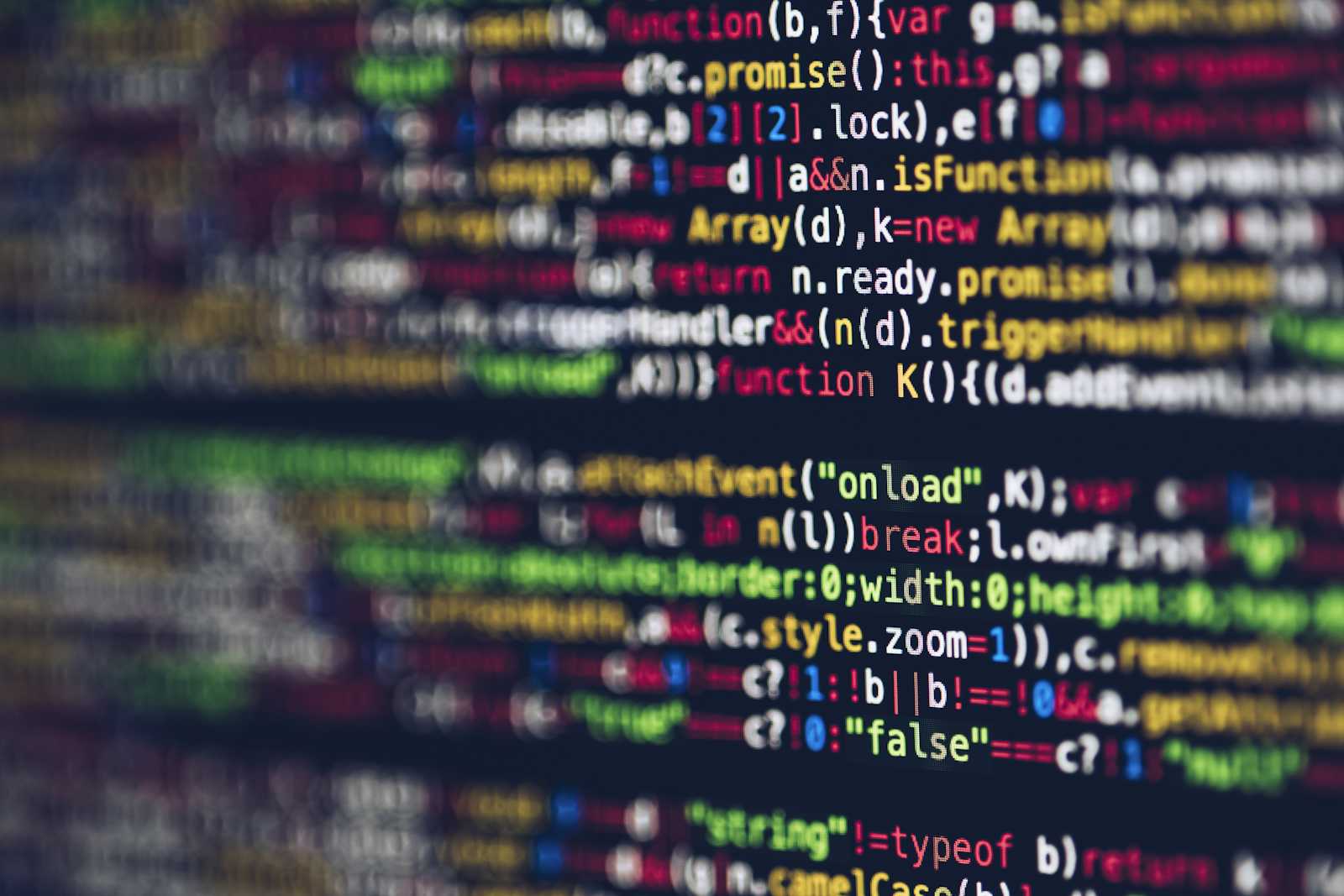
Welcome to the backstage tour of Python! Ever wondered what happens behind the scenes when you run your Python code? Let's take a journey through the inner workings of this beloved language, breaking down complex concepts into bite-sized pieces.
Installation:
Windows:
Visit Python's official website.
Download the latest version for Windows.
Run the installer and check the box that says, "Add Python to PATH" during installation.
Mac:
MacOS usually comes with Python pre-installed. Open a terminal and type
python3
to check the version.If not installed, or you want the latest version, consider using Homebrew:
brew install python
.
Linux:
- Most Linux distributions come with Python pre-installed. To install or upgrade, use your package manager. For example, on Ubuntu:
sudo apt-get update && sudo apt-get install python3
.
- Most Linux distributions come with Python pre-installed. To install or upgrade, use your package manager. For example, on Ubuntu:
After installation, you can verify by running python --version
or python3 --version
in your terminal.
1. Interpreter and Compilation:
Picture the Python interpreter as a wizard who translates your code into a magical language called byte code. It's like turning your spell book (code) into a potion (byte code) that the Python Virtual Machine (PVM) can understand and execute.
# Your code
print("Hello, Python!")
# Inside working (bytecode)
3 0 LOAD_NAME 0 (print)
2 LOAD_CONST 0 ('Hello, Python!')
4 CALL_FUNCTION 1
6 POP_TOP
8 LOAD_CONST 1 (None)
10 RETURN_VALUE
2. Memory Management:
Python is like a friendly roommate who helps you manage memory. Every object (like a list or a number) has a friend count. When no one is using an object, Python's roommate swoops in and cleans up the room (de-allocates memory).
# Memory management
a = [1, 2, 3]
b = a # Now a has a new friend, b
del a # Oops, a's friend count decreases
3. Object Model:
In Python, everything is like a mini superhero – an object with special powers. Understanding objects, classes, and instances is like getting to know the superheroes in your code universe.
# class
class Car:
def __init__(self, brand):
self.brand = brand
my_car = Car("Tesla")
print(my_car.brand) # Accessing car attributes
4. Global Interpreter Lock (GIL):
Imagine the GIL as a traffic cop making sure only one car (thread) moves on the road (executes byte code) at a time. It keeps things safe, but might slow down the traffic.
# GIL traffic example
from threading import Thread
counter = 0
def increase_counter():
global counter
for _ in range(1000000):
counter += 1
# Traffic jam alert!
5. Modules and Packages:
Think of modules and packages as neatly organized drawers in your coding toolbox. They help you keep things tidy and find what you need easily.
# Organizing with modules and packages
# Drawer 1: my_module.py
def greet(name):
print(f"Hello, {name}!")
# Drawer 2: main_script.py
from my_module import greet
greet("Pythonista")
6. Built-in Data Types and Functions:
Python has special toys (data types and functions) that make coding fun and easy. They're like LEGO bricks – simple, but you can build wonderful things with them.
# Playing with LEGO bricks
numbers = [1, 2, 3, 4, 5]
sum_of_numbers = sum(numbers)
average = sum_of_numbers / len(numbers)
print("Average:", average)
7. C-Extensions and Performance Optimization:
When you need a superhero to move faster, you call in a C-Extension – a superpower boost for your code.
# Superhero with a C-Extension boost
# File: my_extension.c
#include <Python.h>
static PyObject* my_function(PyObject* self, PyObject* args) {
// Super fast C implementation
// ...
return Py_BuildValue("i", result);
}
// ...
There you have it – a backstage pass to Python's magical world! As you explore more, you'll discover that Python's simplicity is like a treasure chest hiding powerful tools. Keep coding and let the magic unfold! 🚀✨
To get started in more easy mode, you can follow the below resource, which in itself helped me get going in the python world.
Internal working of python by Hitesh Choudhary (Also shoutout to him for explaining in such a simple but informative manner Hitesh Choudhary)
Let's explore the tech world together. Connect with me on LinkedIn ash1ni
Subscribe to my newsletter
Read articles from Ashwini Kumar Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
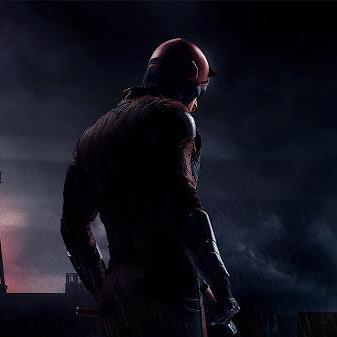
Ashwini Kumar Pandey
Ashwini Kumar Pandey
Hi!, I am Ashwini, and I am a developer who is regularly exploring the majestic world of programming and development.