Navigating Your Travels: A Guide to Using Python's sorted() Function
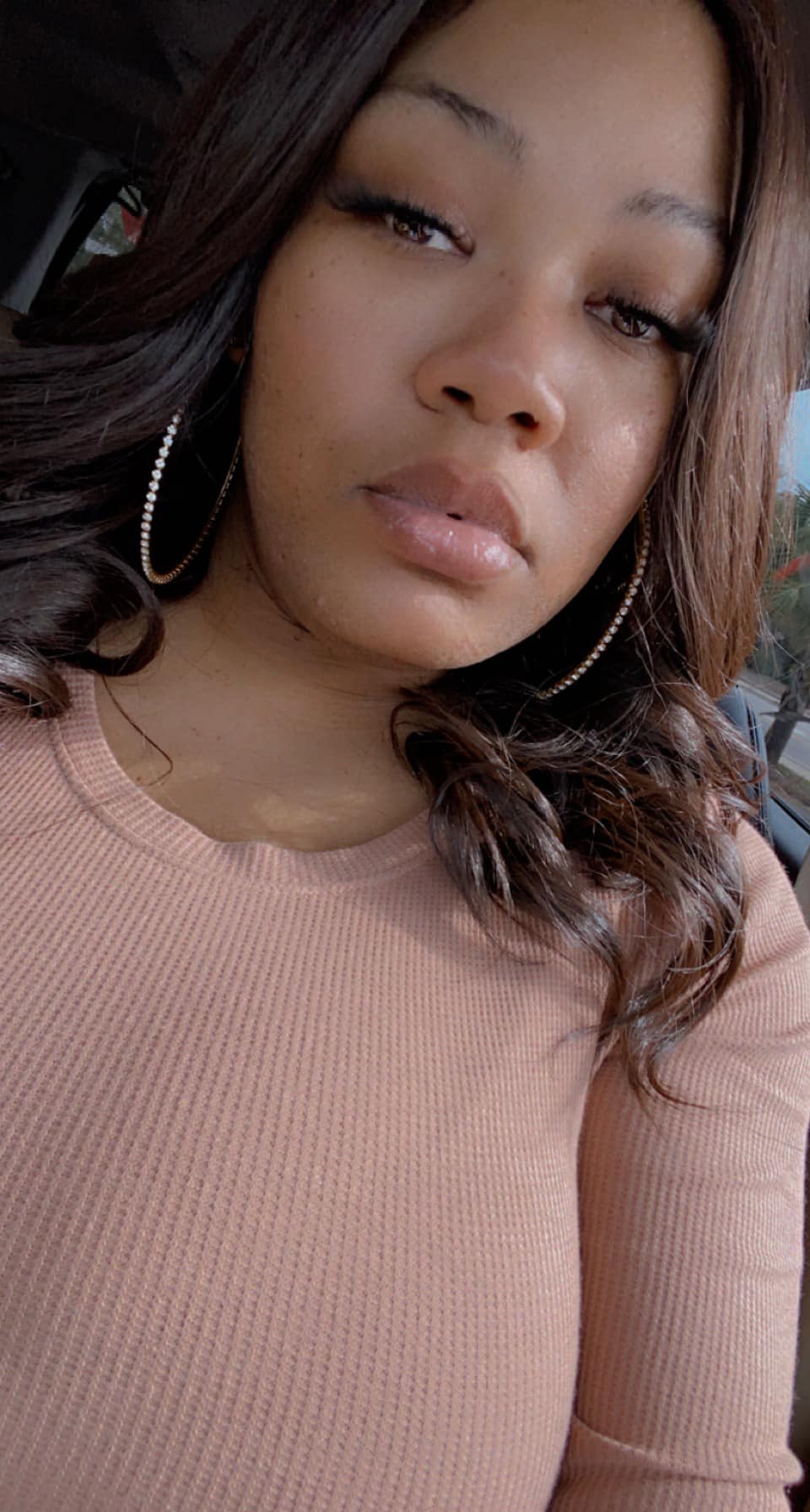
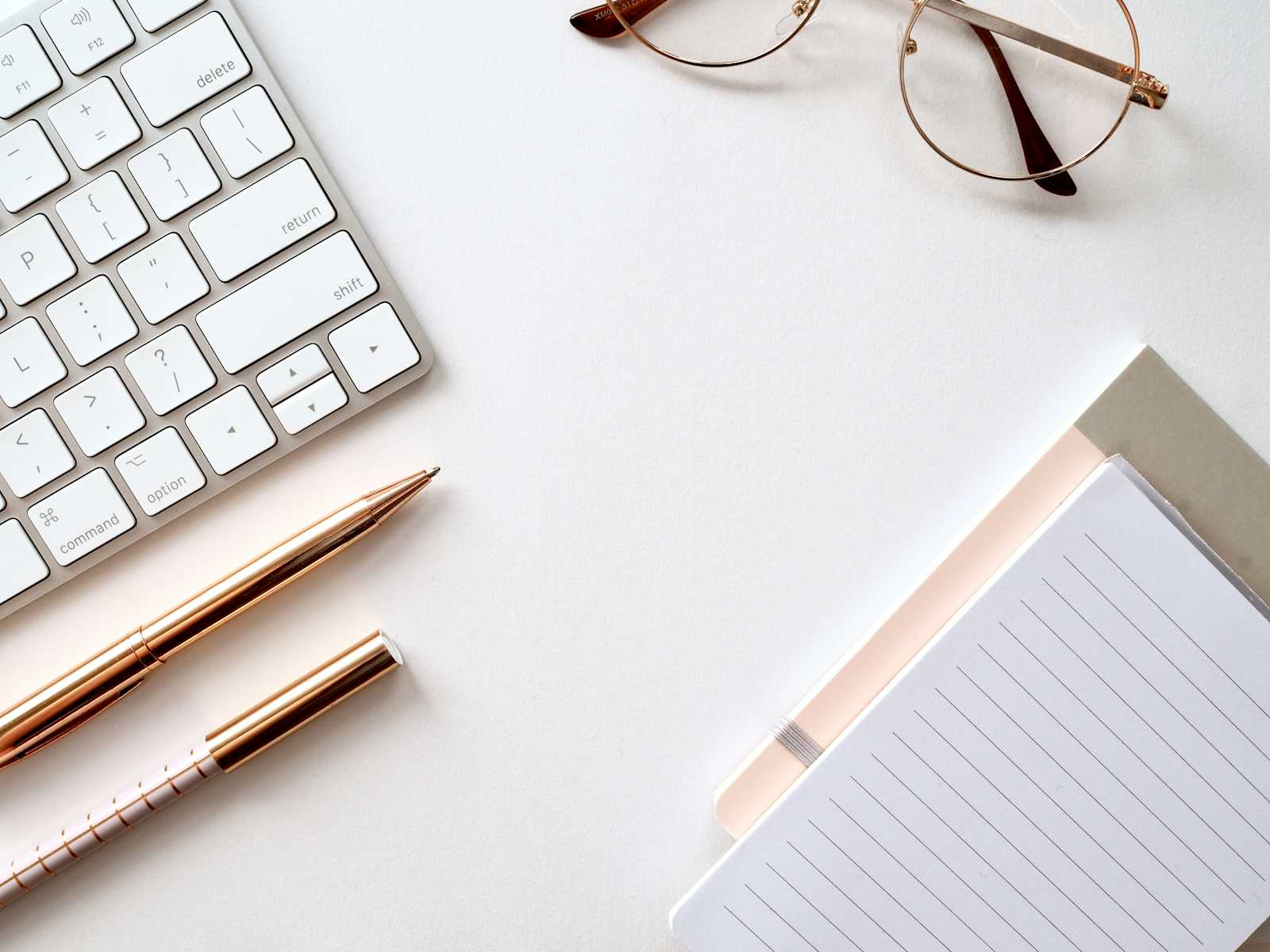
Hey, fellow coding enthusiasts! Today, I'm excited to delve into the intricacies of Python and share some insights on how to effectively use the sorted()
function. In this blog post, we'll explore a practical example – sorting travel data using the sorted()
function. So buckle up, and let's dive into the world of organized travel records!
First things first, let's take a look at the code snippet:
def sort_by_state():
trips = Trip.get_all_by_visit()
sorted_trips = sorted(trips, key=lambda x: x[2])
if sorted_trips:
results = [f'<name: {travels[0]}, city: {travels[1]},
state: {travels[2]}, country: {travels[3]},
month: {travels[4]}, year: {travels[5]},
stars_given: {travels[6]}>' for travels in sorted_trips]
print("No entries to display.")
This function, sort_by_state()
, is designed to organize travel records based on the state they are in. The magic happens in the line, where the sorted()
function is utilized. Let me walk you through the steps and enlighten you on the power of this function.
The sorted()
function takes an iterable (in this case, the trips
list) and returns a new sorted list. The key
parameter is crucial as it specifies a function of one argument that is used to extract a comparison key from each element in the iterable. In our scenario, the lambda function lambda x: x[2]
extracts the state information (at index 2) from each travel record for sorting purposes.
Now, let's break down the comprehension in the results
line:
results = [f'<name: {travels[0]}, city: {travels[1]}, state: {travels[2]},
country: {travels[3]}, month: {travels[4]}, year: {travels[5]},
stars_given: {travels[6]}>' for travels in sorted_trips]
This line creates a list of formatted strings, containing information about each trip. Notice that we are iterating over the sorted_trips
list, ensuring that our data is now organized based on the state.
To sum it up, using the sorted()
function with a custom key allows us to effortlessly organize our travel data by state. This is just one of the many ways you can leverage Python's built-in functions to make your code cleaner and more efficient.
Happy coding, and may your travels be as organized as your Python scripts! Feel free to share your thoughts or ask questions in the comments below. Until next time, happy coding!
Subscribe to my newsletter
Read articles from TaKeya McFadden directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
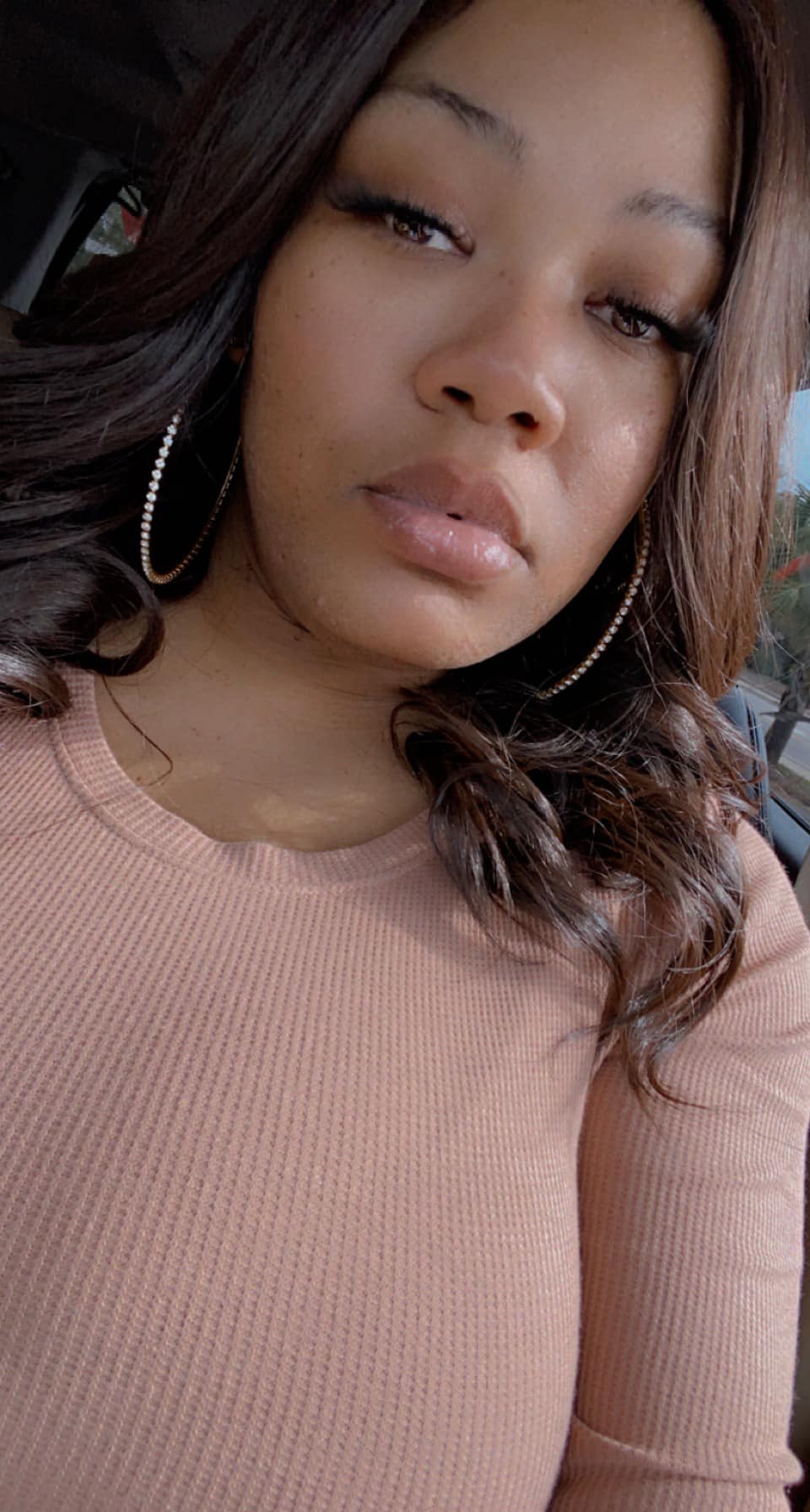