Python Revision Project - 1
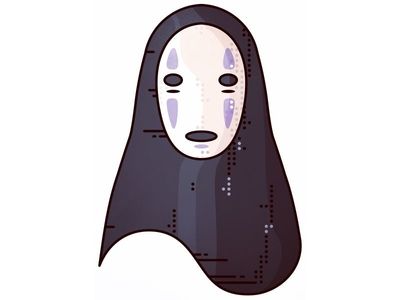
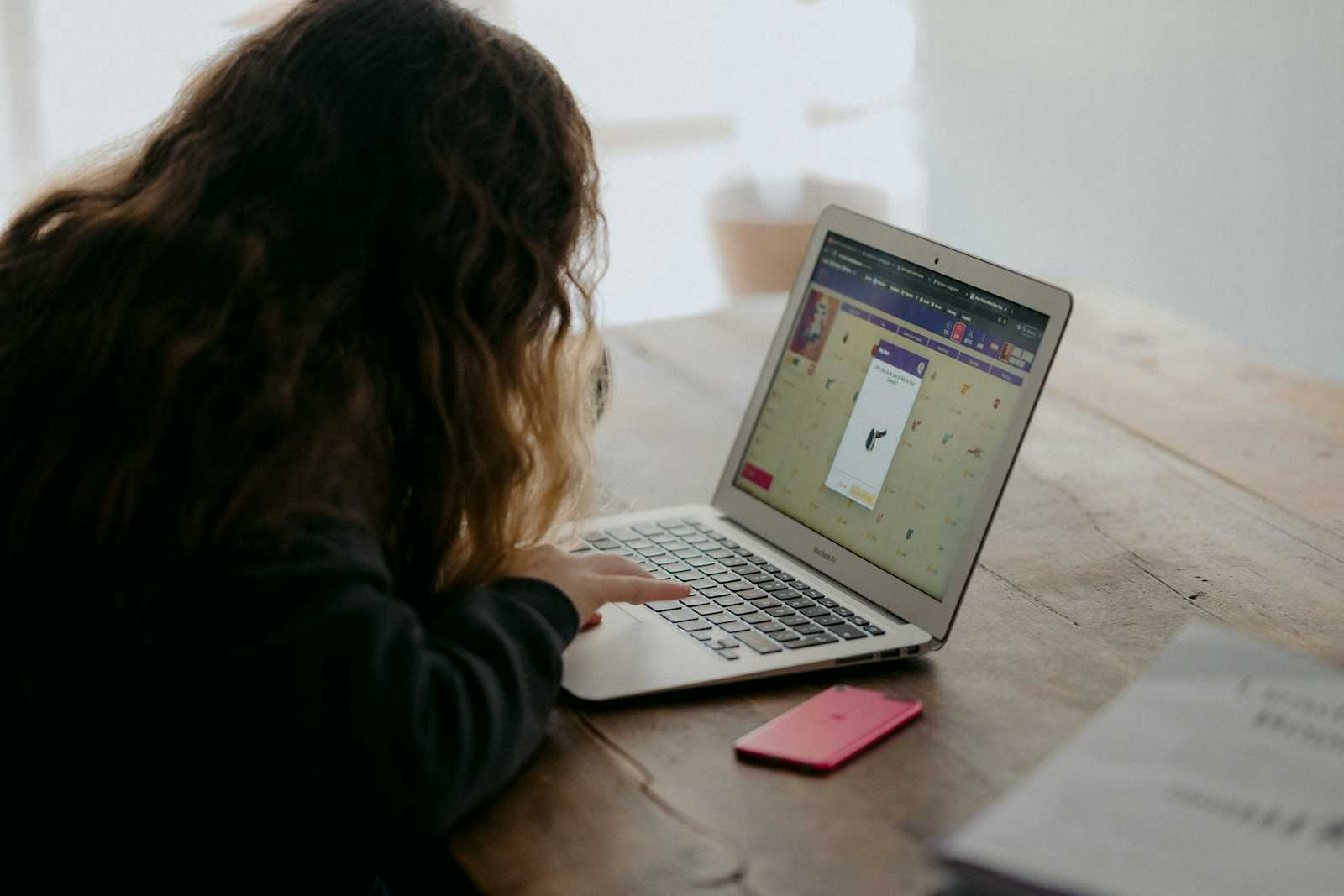
Project Description
This is a simple project where users can view, create, search, update and delete contacts through the CLI. The contact detail contains name, number and email, and the data is stored in a .txt
file. The entire code of the project can be found in this GitHub Repo.
Project Detail
The project structure is fairly simple and easy to follow.
ContactBookApplication/
│
├── main.py
├── contactManager.py
└── data/
└── contactData.txt
The main.py
is the file through which the project is initialized. It contains simple prompts that allow user to access various functionalities of the application. Similarly, contactManager.py
contains functions that allow the user to view, create, update, delete and find contacts. Finally, the contactData.txt
file contains all the contact data.
Here is the general description of each file:
# main.py
from contactManager import viewContacts, createContact, searchContact, updateContactDetail, deleteContact
print("Hello, Welcome to Contact Management System! \n")
userLoggedIn = True
while(userLoggedIn):
userChoice = input("What would you like to do?\n1. View contacts\n2. Create new contact\n3. Find a contact\n4. Update existing contact\n5. Delete a contact\n6. Exit the program\nEnter: ")
if userChoice == '1':
viewContacts()
elif userChoice == '2':
createContact()
elif userChoice == '3':
userNamePrompt = input('Enter contact owner name: ')
searchContact(userNamePrompt)
elif userChoice == '4':
userNumberPrompt = input('Enter the contact number: ')
contactName = input("Enter new contact name: ")
contactNumber = input("Enter new contact number: ")
contactEmail = input("Enter new contact email: ")
updateContactDetail(userNumberPrompt, contactName, contactNumber, contactEmail)
elif userChoice == '5':
userNumberPrompt = input('Enter contact number: ')
deleteContact(userNumberPrompt)
elif userChoice == '6':
userLoggedIn = False
print("Program ended successfully!\n")
The main.py
contains prompts that allows the user to manipulate contacts. It is fairly straight forward. Option 1 to 5
allows user to view, create, search, update and delete contacts respectively. And option 6
just terminates the program.
# contactManager.py
import os
filePath = 'data/contactData.txt'
# view all contacts
def viewContacts():
folderExist = os.path.isdir('data')
fileExist = os.path.exists(filePath)
if(folderExist and fileExist):
with open(filePath, "r") as fileContent:
print(fileContent.read())
else:
print('No contact details available!\n')
def userCreateContactPrompt():
contactName = input("Enter contact name: ")
contactNumber = input("Enter contact number: ")
contactEmail = input("Enter contact email: ")
contactData = f"{contactName}, {contactNumber}, {contactEmail}\n"
with open(filePath, "a") as file:
file.write(contactData)
print("Contact created successfully!\n")
# create a contact
def createContact():
# if folder doesnt exists
# create the folder
folderExist = os.path.isdir('data')
if not folderExist:
os.makedirs('data')
userCreateContactPrompt()
return
else:
# append the details provided by the user
# also creates the file, if does not exists
userCreateContactPrompt()
return
# find/search a contact
def searchContact(contactName):
with open(filePath, 'r') as file:
lines = file.readlines()
for line in lines:
if line.find(contactName) != -1:
print(line)
return
print('No such contact exists!\n')
# update existing contact
def updateContactDetail(contactNumber, updatedName, updatedNumber, updatedEmail):
updated_contact = f"{updatedName}, {updatedNumber}, {updatedEmail}\n"
contactFound = False
with open(filePath, "r+") as file:
lines = file.readlines()
file.seek(0) # file pointer at the beginning
for index, line in enumerate(lines):
if contactNumber in line:
lines[index] = updated_contact
contactFound = True
file.writelines(lines)
file.truncate()
if contactFound:
print('Contact detail updated successfully!\n')
else:
print('Contact detail not found!\n')
# delete existing contact
def deleteContact(contactNumber):
with open(filePath, "r") as file:
lines = file.readlines()
with open(filePath, "w") as file:
for line in lines:
if contactNumber not in line:
file.write(line)
print('Contact deleted successfully!\n')
ContactManager.py
contains the main logic of the application. I will briefly explain each method's functionality:
viewContacts()
: It displays the contact details stored in thecontactData.txt
file in the CLI. If no file or folder is found appropriate message is displayed.userCreateContactPrompt()
: It takes contact name, number and email from the user and writes in thecontactData.txt
file. If the file does not exist, the write method automatically creates the file. For more information on the method you can reference this blog by freeCodeCamp.createContact()
: It calls theuserCreateContactPrompt()
method and creates the contact.searchContact()
: It reads the file usingreadlines()
method, which returns an array of strings for each line. Then by using for loop, the name provided by the user as a prompt is searched in thecontactData.txt
file and the first occurrence is displayed. If no contact is found appropriate message is displayed.updateContactDetail()
: It accepts the contact number and new name, number and email from the user. After going through the array of lines, if the provided contact number matches, it is replaced by the new data provided by the user.deleteContact()
: It takes contact number as a parameter. Then after reading the file, it goes through the array of strings to write into the file except the line that contains the number provided by the user.
Insights
I decided to create this simple program to familiarize myself with the python syntax. The program is not perfect, not at all 😬. There are many ideal conditions that I have assumed, some of them being:
User will always input valid contact number and email
There will be no duplicate contact name, number or email
User will always input prompt between 1 and 6
So, the program can be definitely improved. Even viewing the data can be further improved. I've just printed the contents of the text file without even structuring them in a tabular format to make it more readable.
Conclusion
Concluding, feel free to update the code which can be found easily in my repository. This small and simple project was a great refresher to python. I will be doing a couple more refresher projects so see ya later 👋.
Subscribe to my newsletter
Read articles from Bidhan Bajracharya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
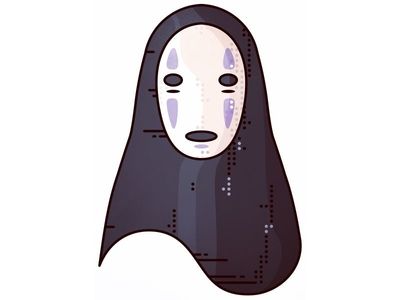
Bidhan Bajracharya
Bidhan Bajracharya
A passionate individual learning about data science and web development.