Generics in Java

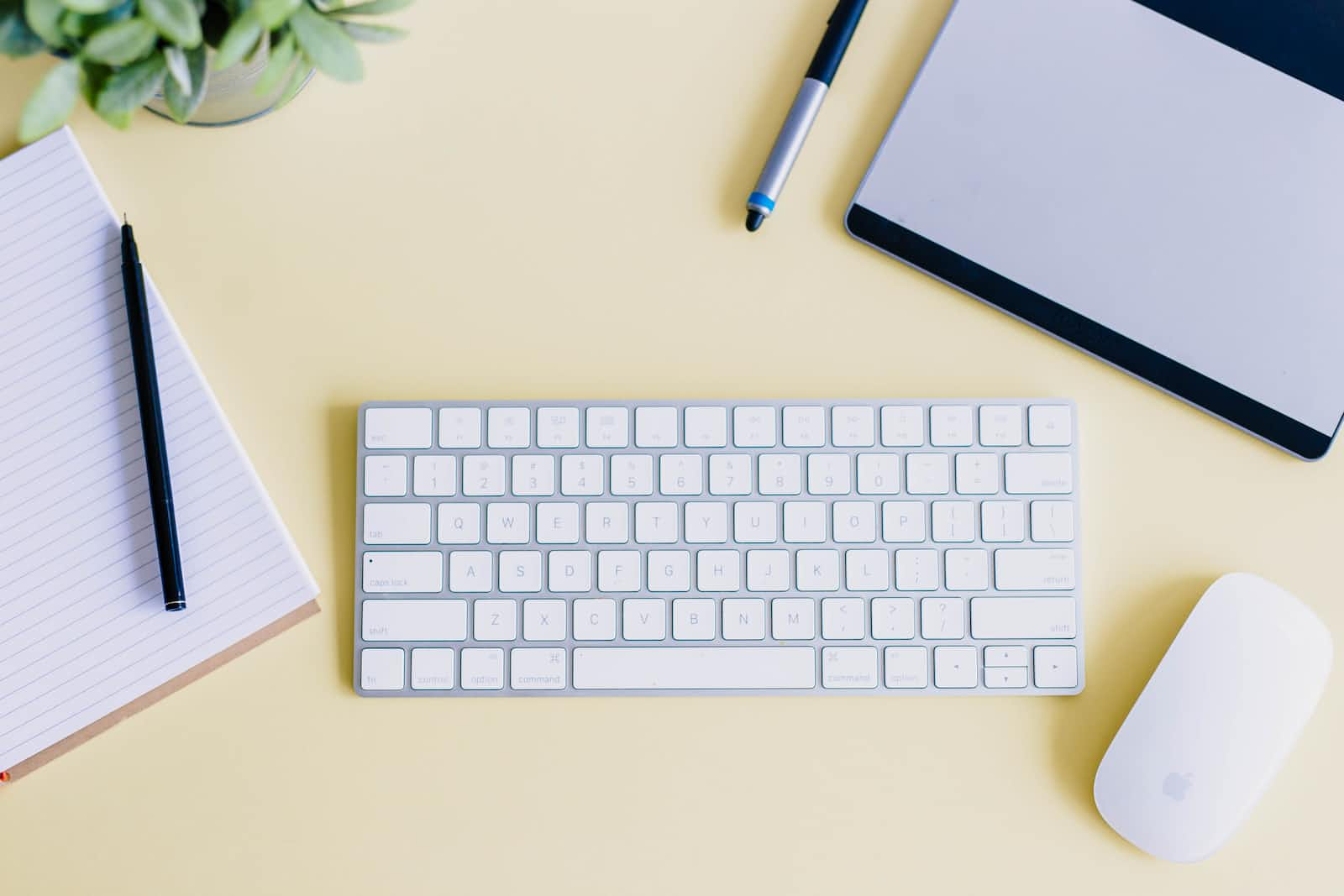
Look at code example below
class Pair {
double x;
double y;
public void setx(double x){
this.x = x;
}
public void sety(double y){
this.y = y;
}
}
public class Main {
public static void main(String[] args){
Pair p = new Pair();
p.setx(3.15);
p.sety(6.12);
}
}
We are creating a pair class which can store a coordinate with attributes x and y coordinates on 2D Plane.
what if there is an another use case in the application where we want to store a firstname and lastname in some class. you would have thought to create the similar class like Pair, which will have two string attributes.
Imagine the applications grows more and you get many usecases to create more data types like Pair. Look at below code now
class Pair {
Object x;
Object y;
public void setx(Object x){
this.x = x;
}
public void sety(Object y){
this.y = y;
}
}
public class Main {
public static void main(String[] args){
// storing a coordinate
Pair p = new Pair();
p.setx(3.15);
p.sety(6.12);
// storing firstname and lastname
Pair p2 = new Pair();
p2.setx("Java");
p2.sety("Language");
}
}
The above code works fine, because Object data type is super class of all data types in Java.So, it can store any time of data in it. But it can create some problems in the code.
class Pair {
public Object x;
public Object y;
public void setx(Object x){
this.x = x;
}
public void sety(Object y){
this.y = y;
}
}
public class Main {
public static void main(String[] args){
// storing a coordinate
Pair p = new Pair();
p.setx(3.15);
p.sety(6.12);
// storing firstname and lastname
Pair p2 = new Pair();
p2.setx("Java");
p2.sety("Language");
String firstName = (String)p.x;
}
}
The last line of code can cause run time error because we are trying to convert type double into type string.
To Solve this problem Generics was introduced in Java
Generics is a concept that allows you to define a class with parameterized data types of their attributes.
class Pair<V, S> {
public V x;
public S y;
public void setx(V x){
this.x = x;
}
public void sety(S y){
this.y = y;
}
}
public class Main {
public static void main(String[] args){
Pair<double, double> coordinate = new Pair<>();
coordinate.setx(3.15);
coordinate.sety(6.12);
Pair<String, String> p2 = new Pair();
p2.setx("Java");
p2.sety("Language");
}
}
If you see the when we declared the Pair class in the above code example, we have used some new syntax <V, S>. V and S are Placeholders for data type which means you can pass any data type while creating an Object.
This will solve the previous run time error problem becuase all the data types are known at compile time only. so if we making the same mistake like explicit conversion of double to string data type, it will cause compile time error rather than run time error.
That is where Generics are helpful.
Subscribe to my newsletter
Read articles from chaitanya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
