Deploying a Two-Tier Application with Docker Compose
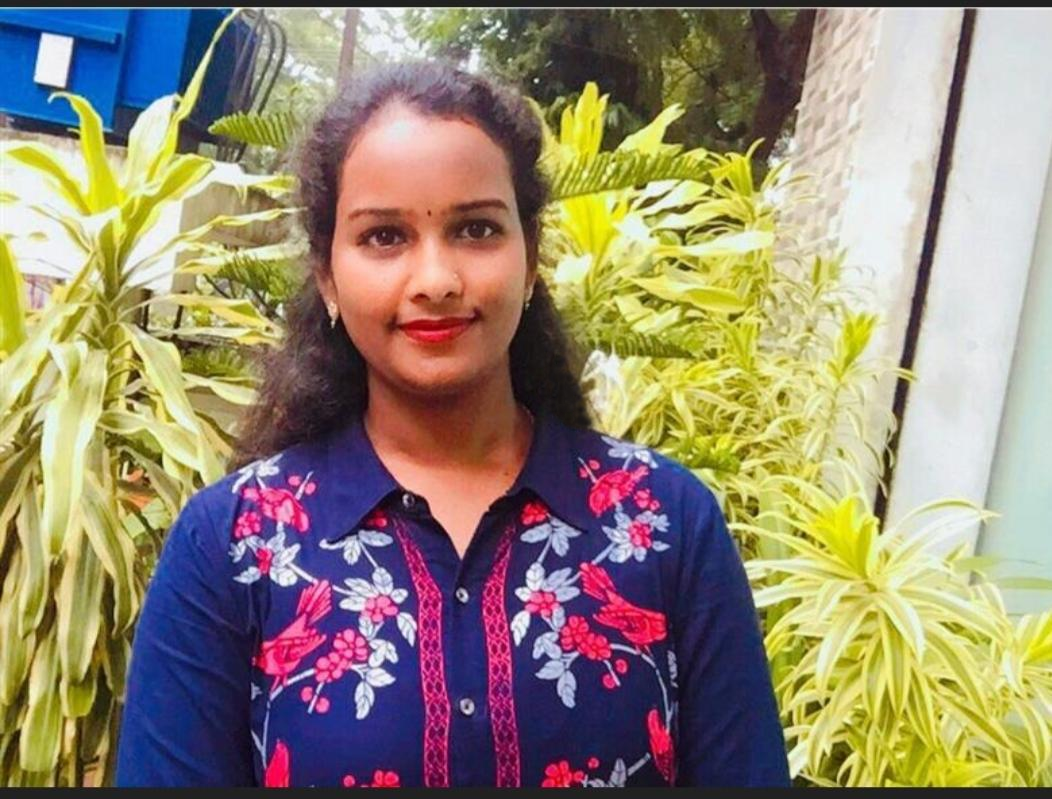
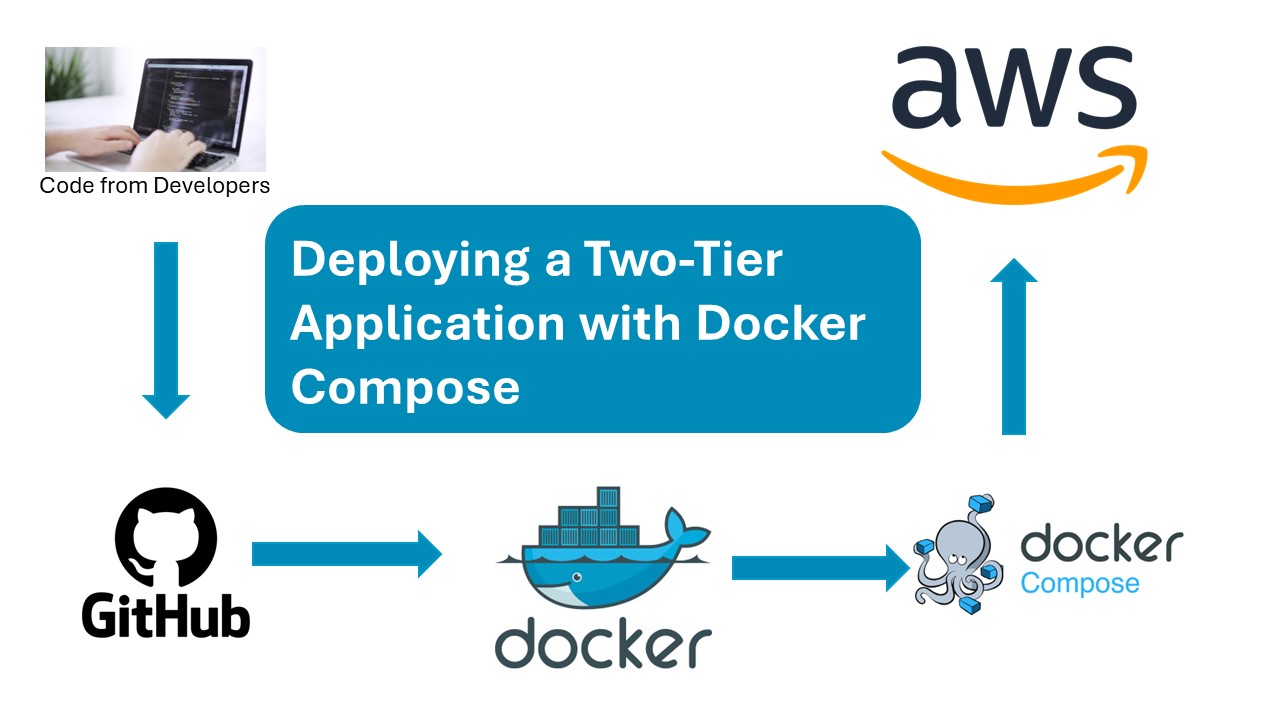
In the realm of modern software development and deployment, containerization has become an essential practice. Docker, a leading containerization platform, has revolutionized the way applications are packaged, shipped, and deployed. One common scenario in application deployment is the use of a two-tier architecture, which separates the presentation layer from the data layer. In this blog post, we'll explore how to deploy a two-tier application using Docker Compose, a tool that simplifies the management of multi-container Docker applications.
Understanding Two-Tier Architecture
Before diving into deployment strategies, let's first grasp the concept of a two-tier architecture. In this architectural pattern, an application is divided into two main layers:
Presentation Tier : Also known as the frontend layer, this tier is responsible for interacting with users and presenting data. It typically includes components such as web servers, APIs, or any interface that users interact with directly.
Data Tier : The backend layer, also known as the data tier, manages and stores data used by the presentation layer. This tier often consists of databases or other data storage systems.
By separating these layers, applications become more modular, scalable, and easier to maintain. Docker Compose allows us to define and manage both tiers as separate services within a single configuration file.
Docker Compose:
Simplifying Multi-Container Deployment
Docker Compose is a powerful tool for defining and running multi-container Docker applications. It uses a simple YAML file to specify the services, networks, and volumes required for an application. With Docker Compose, developers can define complex application stacks, manage dependencies, and streamline the deployment process.
Key Features of Docker Compose:
Service Definitions : Define individual components of your application as services, each running in its own container.
Dependency Management : Specify dependencies between services, ensuring they start in the correct order.
Environment Configuration : Set environment variables, volumes, and network settings for each service.
Development Workflow : Spin up the entire application stack with a single command, making it easy to test and iterate on changes.
In the context of a two-tier application, Docker Compose enables us to define and deploy both the frontend and backend components as separate services, each running in its own container. Let's walk through an example of deploying a two-tier application using Docker Compose.
Compose file
version: '3'
services:
backend:
build:
context: .
ports:
- "5000:5000"
environment:
MYSQL_HOST: mysql
MYSQL_USER: admin
MYSQL_PASSWORD: admin
MYSQL_DB: myDb
depends_on:
- mysql
mysql:
image: mysql:5.7
ports:
- "3306:3306"
environment:
MYSQL_ROOT_PASSWORD: root
MYSQL_DATABASE: myDb
MYSQL_USER: admin
MYSQL_PASSWORD: admin
volumes:
- ./message.sql:/docker-entrypoint-initdb.d/message.sql
- mysql-data:/var/lib/mysql
volumes:
mysql-data:
Now, let's break down each section of the Docker Compose file and explain its purpose:
Version
version: '3'
- Specifies the version of the Docker Compose file format being used. In this case, it's version 3.
Services
services:
- This section defines the services that make up your application. Each service represents a containerized component of your application.
Backend Service
backend:
build:
context: .
- Defines a service named
backend
and specifies that it should be built using the Dockerfile located in the current directory (.
).
ports:
- "5000:5000"
- Maps port 5000 on the host to port 5000 on the container, allowing external access to the backend service.
environment:
MYSQL_HOST: mysql
MYSQL_USER: admin
MYSQL_PASSWORD: admin
MYSQL_DB: myDb
- Sets environment variables required by the backend service to connect to the MySQL database.
depends_on:
- mysql
- Specifies that the backend service depends on the MySQL service, ensuring that MySQL is started before the backend service.
MySQL Service
mysql:
image: mysql:5.7
- Defines a service named
mysql
using themysql:5.7
Docker image from Docker Hub.
ports:
- "3306:3306"
- Maps port 3306 on the host to port 3306 on the container, allowing external access to the MySQL service.
environment:
MYSQL_ROOT_PASSWORD: root
MYSQL_DATABASE: myDb
MYSQL_USER: admin
MYSQL_PASSWORD: admin
- Sets environment variables required to configure the MySQL database.
volumes:
- ./message.sql:/docker-entrypoint-initdb.d/message.sql
- mysql-data:/var/lib/mysql
- Mounts a SQL script (
message.sql
) into the MySQL container's initialization directory to automatically create a table. Also, mounts a volume for persistent data storage.
Volumes
volumes:
mysql-data:
- Defines a named volume (
mysql-data
) that will be used to persist MySQL data.
Ports to Open on the Server for Deployment
This Docker Compose file defines a two-tier application consisting of a backend service (Flask application) and a MySQL database. The backend service communicates with the MySQL database using environment variables defined in the Docker Compose file. Additionally, it ensures that the MySQL service is started before the backend service to fulfill dependencies.
Feel free to customize this Docker Compose file according to your specific application requirements and deployment environment.
To deploy a two-tier application using Docker Compose, you typically need to consider the ports that need to be open on the server hosting the Docker containers. Here's a breakdown of the ports you might need to open:
Backend Service:
- The backend service in your Docker Compose file is configured to expose port 5000 (
- "5000:5000"
). This port is used by the backend service to listen for incoming requests. If your backend service communicates with external clients, you'll need to open this port to allow inbound traffic.
- The backend service in your Docker Compose file is configured to expose port 5000 (
MySQL Database:
- The MySQL service in your Docker Compose file is configured to expose port 3306 (
- "3306:3306"
). This port is used by MySQL for client connections. If your application needs to connect to the MySQL database from external clients or services, you'll need to open this port to allow inbound traffic.
- The MySQL service in your Docker Compose file is configured to expose port 3306 (
In summary, on the server hosting your Docker containers, you'll need to ensure that ports 5000 and 3306 are open to allow inbound traffic to the backend service and MySQL database, respectively. You can open these ports using your server's firewall configuration or by configuring network security groups if you're using a cloud provider like AWS or Azure.
Additionally, if your application communicates over other ports (for example, if the backend service communicates with other services or APIs), you'll need to open those ports as well. However, based on the Docker Compose file above, only ports 5000 and 3306 are explicitly exposed for communication.
Deployment Commands: Setting Up Your Two-Tier Application with Docker Compose
Here are the commands you would typically run to deploy the two-tier application using Docker Compose:
Clone the Repository: If you haven't already, clone the repository containing your application code and Docker Compose file.
git clone <repository_url> cd <repository_directory>
Build and Start Docker Containers: Use Docker Compose to build and start the Docker containers defined in the
docker-compose.yml
file.docker-compose up -d
Verify Containers: Check that the Docker containers are running.
docker ps
You should see containers for both the backend service and the MySQL database running.
Verify Application: Verify that your application is accessible. You can access the backend service via the specified port (e.g.,
http://<server_ip>:5000
).Optional: Access MySQL Database: If needed, you can access the MySQL database using a MySQL client. For example:
mysql -h <server_ip> -P 3306 -u admin -p
Replace
<server_ip>
with the IP address of the server where your Docker containers are running.Stop and Remove Containers: When you're done with your application, you can stop and remove the Docker containers.
docker-compose down
These commands will help you deploy and manage your two-tier application using Docker Compose. Adjust the parameters and options as needed based on your specific environment and requirements.
In summary, Docker Compose offers a streamlined approach to deploying two-tier applications, separating presentation and data layers. By defining services, networks, and volumes in a single YAML file, Docker Compose simplifies management. With clear service definitions, environment configurations, and volume mounts, Docker Compose facilitates efficient deployment. Using Docker Compose commands, starting, managing, and stopping containers becomes straightforward. Whether you're new to containerization or a seasoned developer, Docker Compose is a valuable tool for deploying multi-container applications with ease.
Subscribe to my newsletter
Read articles from SWATHI PUNREDDY directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
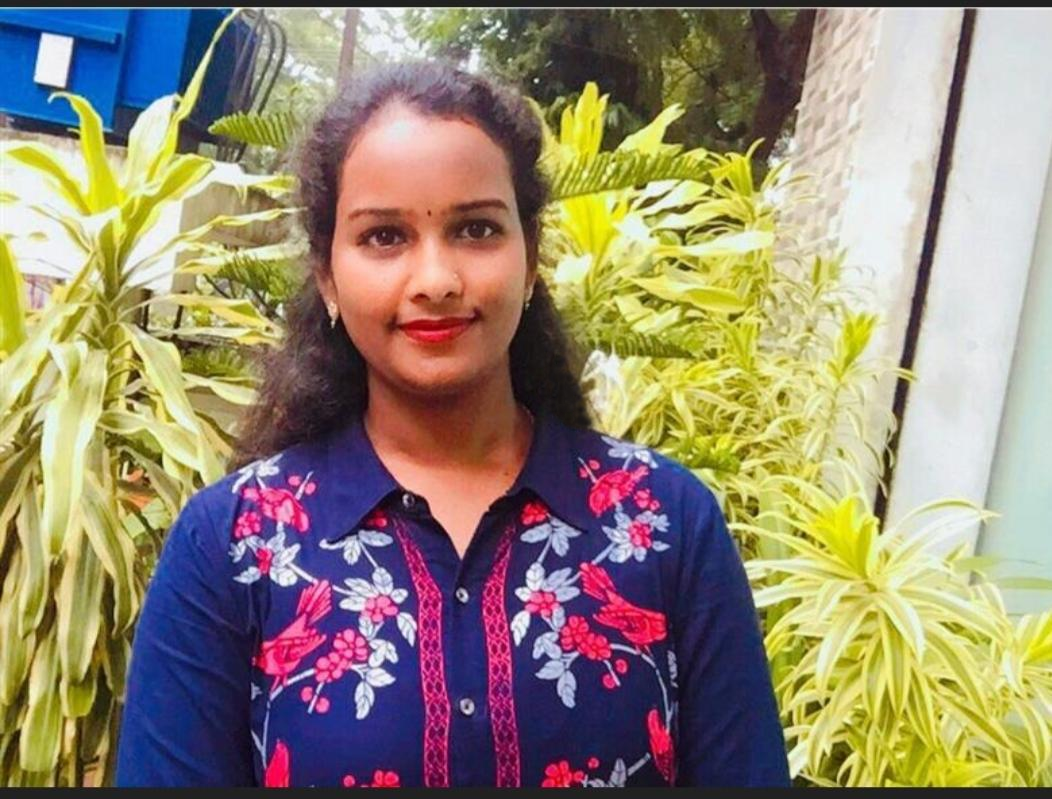