Understanding JavaScript Behind the Scenes.
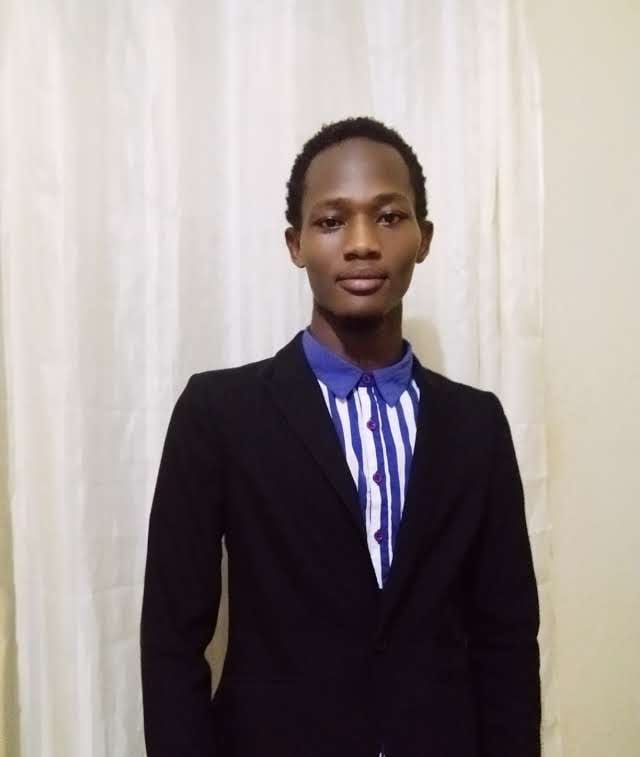
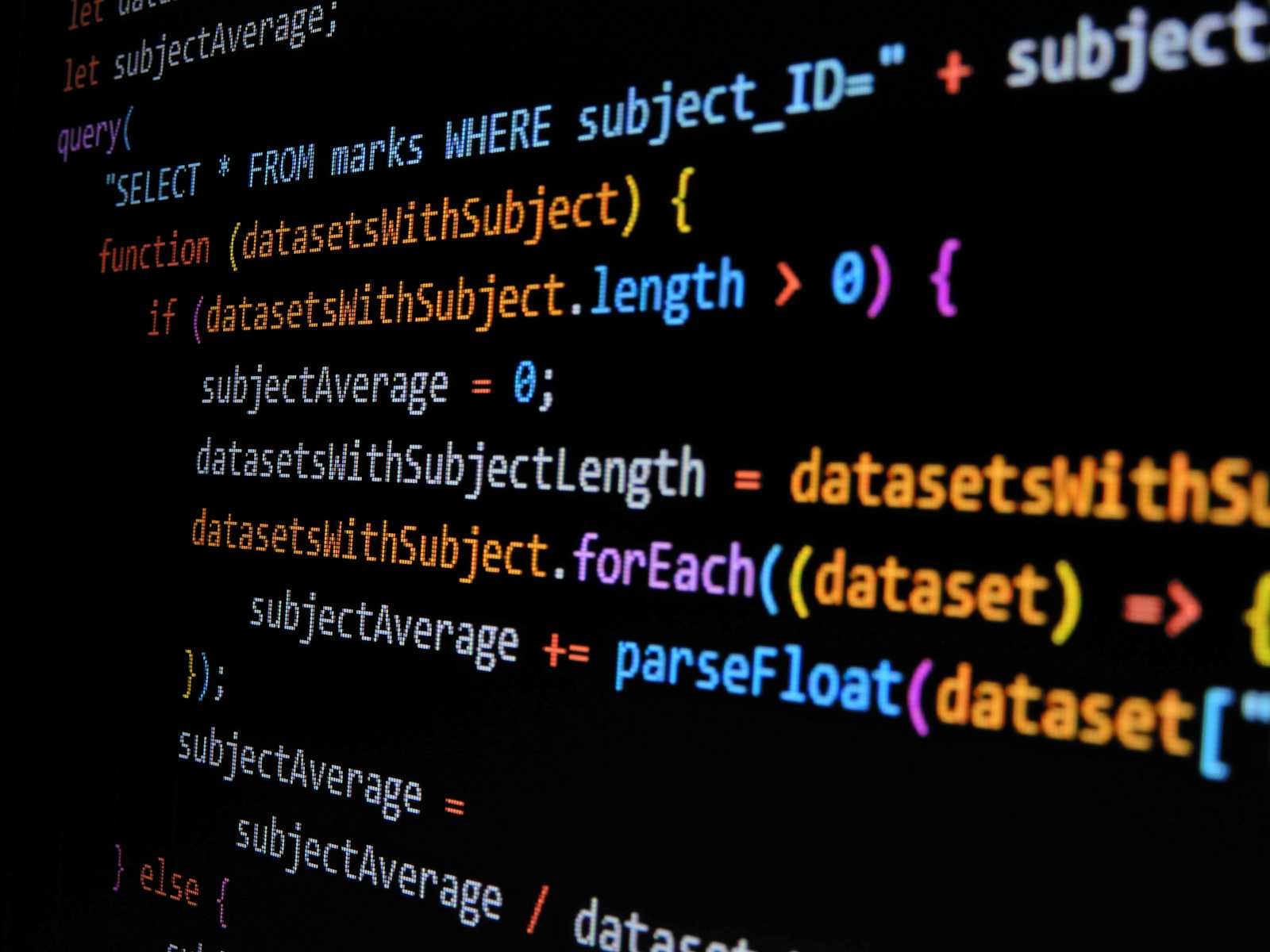
Introduction
Welcome to a behind-the-scenes glimpse of JavaScript.We will explore the inner workings that power this versatile language. In this exploration, we'll uncover the essentials like execution context, the call stack, and the crucial interplay between memory and the thread of execution.
Let's dive in!
Execution context
Created to run the code of a function.
Thread of execution
Memory
const name = “Emmett”
function sayGreetings(member){
const greet = `Hey there ${member}, it’s good having you here as our full-time learner!`
return greet;
}
const firstAttempt = sayGreetings(name)
const secondAttempt = sayGreetings("Elie");
console.log(firstAttempt)
console.log(secondAttempt)
Output
Hey there Emmett, it’s good having you here as our full time learner!
Hey there Elie, it's good having you here as our full time learner!
Parameters and arguments. All are placeholders.
Value: anything that is stored in the function. Not the labels.
And when you return, you’re going to ship what is evaluated from the function to the global scope.
Remember, JavaScript is single-threaded, meaning it can run one line of code at a time.
The thread of execution moves from the global scope to the mini functions (let’s say you’re running a block of code that receives params and then returns greetings), and after it has evaluated the value of the function, it goes out of the mini function to the global scope. This continues all the way through until the end of the file.
Const newMember = sayGreetings(“James”)
What we do here is declare a newMember
, we don't know what to assign to a newMember
: we don’t know what to store in newMember until we create another execution context (sayGreetings) and pass a new argument, which in our case is a string named “James”.
After running the context, we return a value, which is the greet
, and then this is accessible to the global and finally assigned to the newMember
as:
Hey there, James, it’s good having you here as our full-time learner!
Call stack
Thread of execution that moves in and out of mini-functions.
JavaScript keeps track of what function is currently running by using call stack.
Call stack is a traditional way of storing information on our computer, we have a number of ways, arrays, and stacks.
When we run a function, we add it to the call stack.
globalFns()
, this could be const secondAttempt = sayGreetings(“Elie”)
; or other external code
Now JavaScript has certainty about what function is currently running.
In the example above, in the case of a stack, we only engage with the thing at the top of it. Now we know where we are right now.
Return? It tells us we have finished a function—end it and move on.
Don't forget that global will always be at the bottom of the call stack, and when the function ends and the stack pops off, JavaScript jumps and runs global.
Core components of JavaScript
A memory is used to store data as we go through the code line by line and also to store code (functionality), and we can then run and trigger the code whenever we want (in the case of newMember or firstAttempt).
Thread of execution. Which goes through the code line by line, and a lot of time is storing data, but also taking some of the stored data, and functionality code and saying starts running it. We create a mini-program, execution context into which we go and categorize any data we’re storing while we’re making that function (let's take in a data, say “Elie” from the global, and use it in the function we're building, sayGreetings). And when we exit that function, all is deleted besides whatever we return out (in our case, “greet”) ... run another function, do the same, and so on.
Then you may wonder, what happens when we delete the function? How can we keep track of our data functions? Well, we keep track of our functions running and know where our thread of execution is using what we mentioned earlier as the “call stack.”
Conclusion
In conclusion, JavaScript operates within an execution context, utilizing a thread of execution and memory to execute functions and manage data. The call stack efficiently keeps track of the currently running functions, ensuring a sequential and organized execution of code in the single-threaded environment of JavaScript.
Let me know your thoughts in the comment section and what suggested topics you would like to learn more about that I may cover.
Subscribe to my newsletter
Read articles from Cornelius Lochipi Emase directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
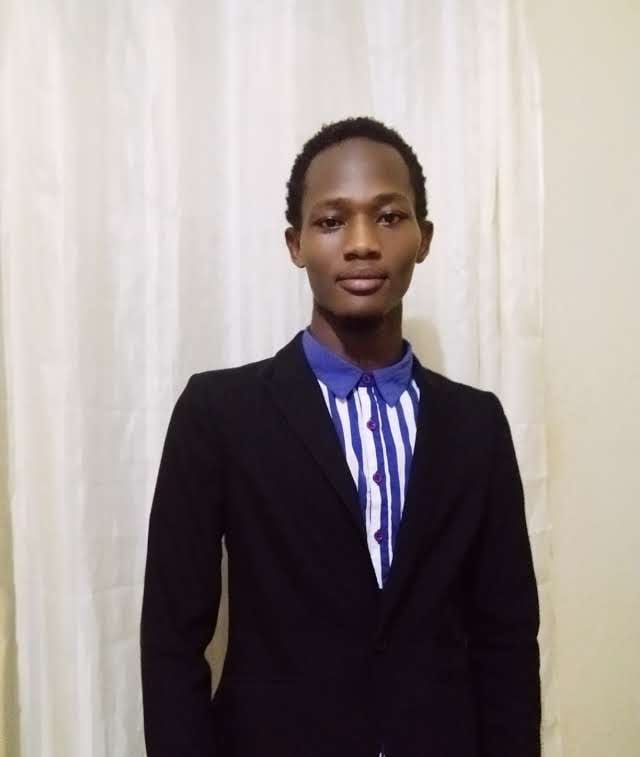
Cornelius Lochipi Emase
Cornelius Lochipi Emase
Software || Data Engineer || Writer.