Building a BMI Calculator with Glassmorphism UI using HTML, CSS, and JavaScript.
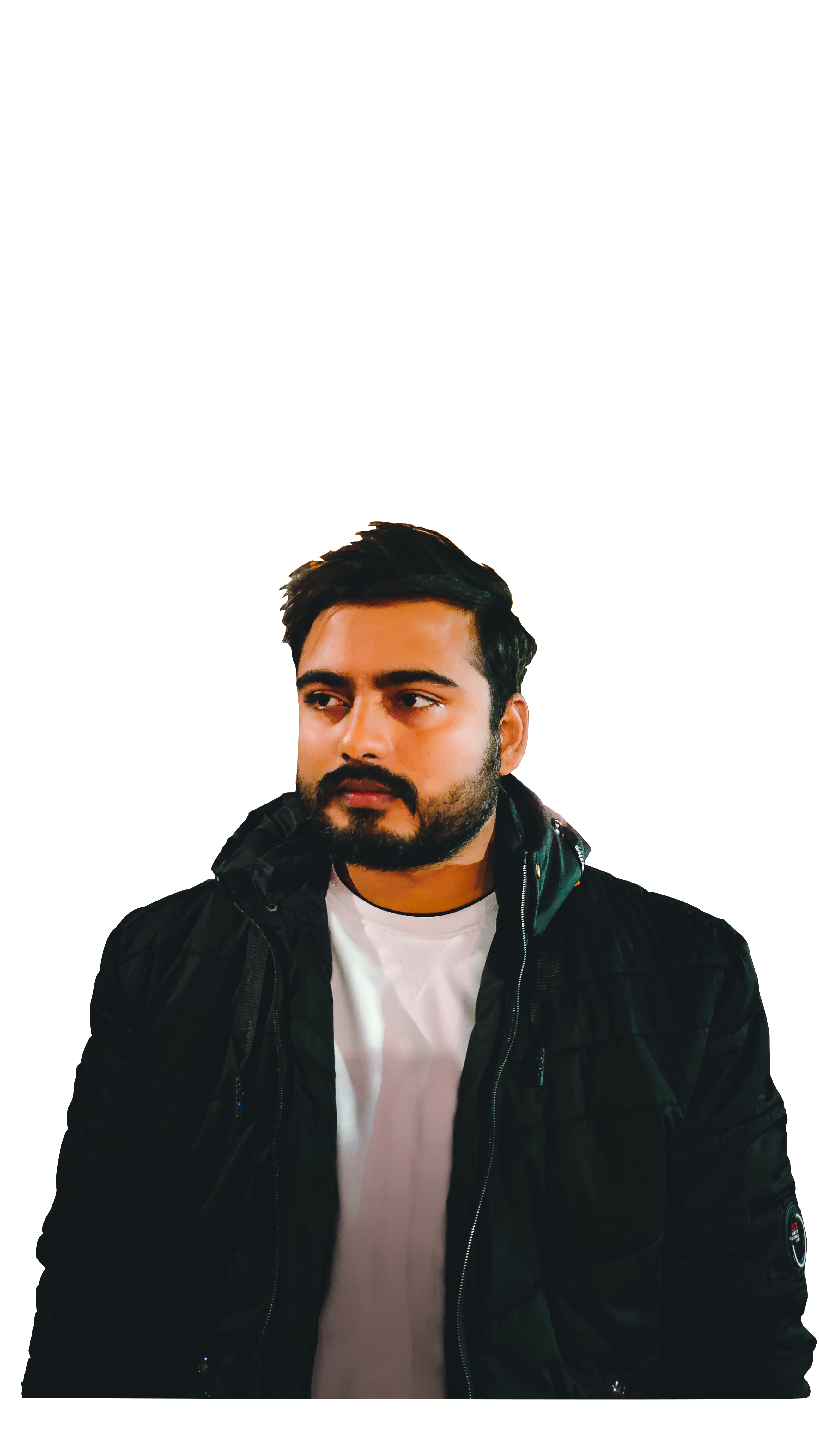
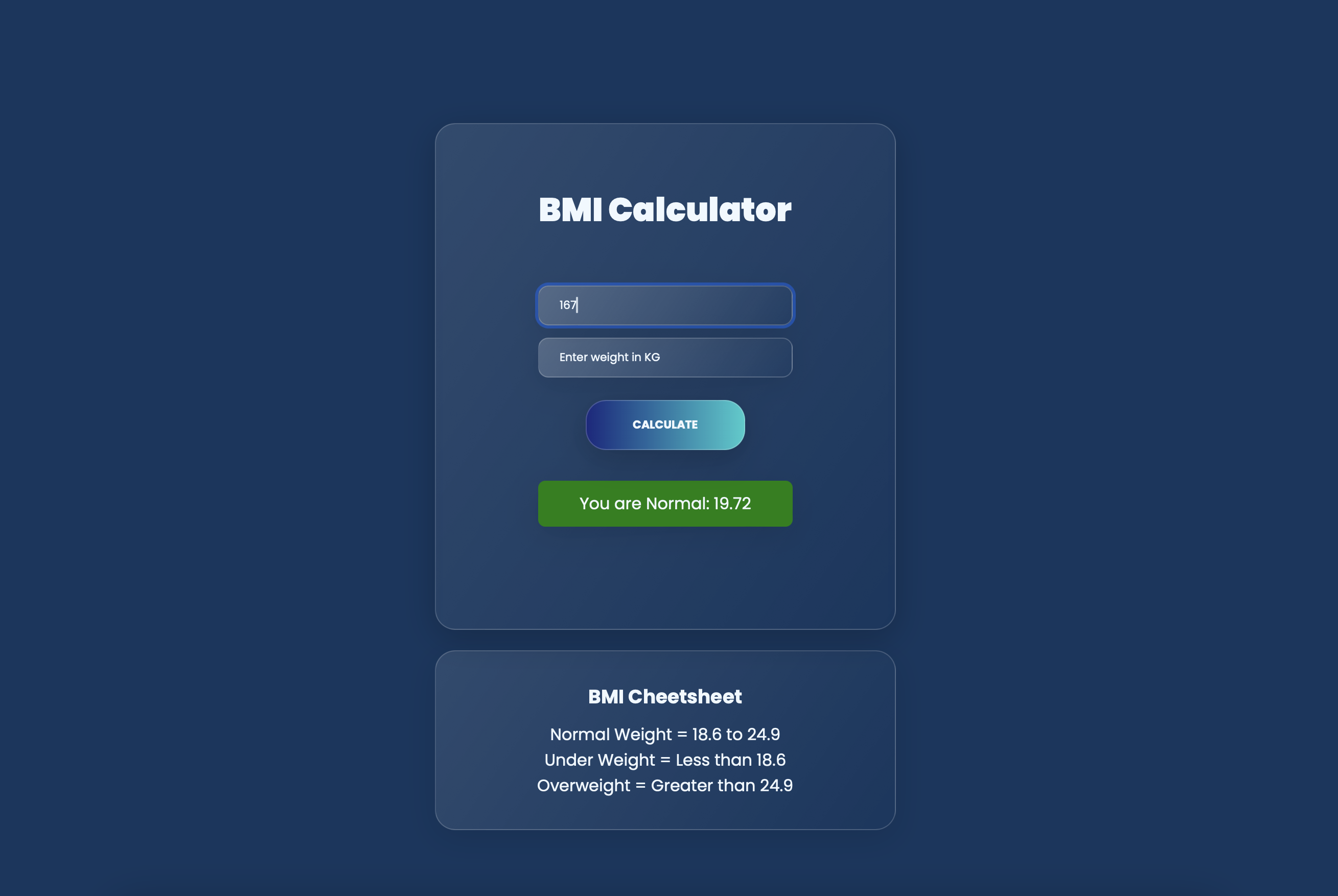
In today's world, where health and fitness have become paramount concerns for many individuals, having tools to monitor one's health is essential. Body Mass Index (BMI) is a widely used indicator to assess whether an individual has a healthy body weight relative to their height. With the advancement of technology, creating a BMI calculator has become relatively simple, especially with the combination of HTML, CSS, and JavaScript. In this article, we'll explore how to build a BMI calculator with a sleek Glassmorphism UI design.
Understanding BMI:
Before diving into the technical details, let's briefly review what BMI is and how it's calculated. BMI is a measure of body fat based on an individual's weight and height. It's calculated by dividing a person's weight in kilograms by the square of their height in meters. The formula is:
BMI=weight(kg)height(m)2BMI\=height(m)2weight(kg)
The resulting BMI value falls into one of the following categories:
Underweight: BMI less than 18.5
Normal weight: BMI ranging from 18.5 to 24.9
Overweight: BMI ranging from 25 to 29.9
Obesity: BMI of 30 or higher
Building the BMI Calculator:
Now, let's move on to the technical aspect of building the BMI calculator using HTML, CSS, and JavaScript. Additionally, we'll incorporate a Glassmorphism UI design to make the calculator visually appealing.
HTML Structure:
We start by creating the basic structure of our BMI calculator using HTML. This includes input fields for height and weight, a button to calculate BMI, and a section to display the result.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>BMI Generator project</title>
<link rel="stylesheet" href="style.css">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Poppins:ital,wght@0,100;0,200;0,300;0,400;0,500;0,600;0,700;0,800;0,900;1,100;1,200;1,300;1,400;1,500;1,600;1,700;1,800;1,900&display=swap" rel="stylesheet">
</head>
<body>
<div class="container">
<div class="main-area">
<h1 class = "guide">BMI Calculator</h1>
<form>
<p><label></label> <input type="text" placeholder="Enter height in CM"
id="height" /></p>
<p><label> </label><input type="text" id="weight" placeholder="Enter weight in KG"/></p>
<button>Calculate</button>
<div class="results" ><p></p></div>
</div>
<div id="weight-guide">
<h3>BMI Cheetsheet</h3>
<p>Normal Weight = 18.6 to 24.9</p>
<p>Under Weight = Less than 18.6</p>
<p>Overweight = Greater than 24.9</p>
</div>
</form>
</div>
</body>
<script src="main.js"></script>
</html>
CSS Styling:
Next, let's add CSS to style our calculator with a Glassmorphism effect.
*{
margin: 0;
padding: 0;
box-sizing: border-box;
/* font-family: openSans;
font-weight-normal */
font-family: Poppins, sans-serif ;
}
body{
/* background-image: linear-gradient(to right top, #051937, #11375f, #185a89, #187fb3, #08a6de); */
background-color: #11375f;
color: aliceblue;
text-align: center
}
.container{
display: inline-block;
justify-content: center;
align-items: center;
margin-top: 18vh;
}
.guide{
margin-bottom: 50px;
font-weight: 1000;
margin-top: -40px;
}
.main-area{
border: solid white 2px;
border-radius: 10px;
padding: 100px;
/* background: rgba(255, 255, 255, .5); */
background: linear-gradient(125deg, rgba(255,255,255, 0.1),rgba(255,255,255,0));
backdrop-filter: blur(10px);
-webkit-backdrop-filter: blur(10px);
border-radius: 20px;
border: 1px solid rgba(255,255,255,0.18);
box-shadow: 0 8px 32px 0 rgba(0, 0, 0, 0.2)
}
#weight-guide{
border: 1px solid rgba(255,255,255,0.18);
border-radius: 20px;
color: aliceblue;
background: linear-gradient(125deg, rgba(255,255,255, 0.1),rgba(255,255,255,0));
box-shadow: 0 8px 32px 0 rgba(0, 0, 0, 0.1);
padding: 30px;
margin-top: 20px;
}
#height{
padding: 10px;
border-radius: 10px;
padding-left: 20px;
padding-right: 20px;
background: linear-gradient(125deg, rgba(255,255,255, 0.2),rgba(255,255,255,0));
border: 1px solid rgba(255,255,255,0.20);
box-shadow: 0 8px 32px 0 rgba(0, 0, 0, 0.1);
margin-bottom: 12px;
transition: 0.5s;
color:aliceblue
}
#weight{
padding: 10px;
border-radius: 10px;
padding-left: 20px;
padding-right: 20px;
background: linear-gradient(125deg, rgba(255,255,255, 0.2),rgba(255,255,255,0));
border: 1px solid rgba(255,255,255,0.20);
box-shadow: 0 8px 32px 0 rgba(0, 0, 0, 0.1);
color:aliceblue;
transition: 0.5s;
}
::placeholder{
color:aliceblue
}
h3{
margin-bottom: 10px;
}
button{
padding: 10px;
margin-top: 22px;
border: 1px solid rgba(255,255,255,0.20);
box-shadow: 0 8px 32px 0 rgba(0, 0, 0, 0.2);
border-radius: 20px;
/* background-image: linear-gradient(to right, #314755 0%, #26a0da 51%, #314755 100%); */
background-image: linear-gradient(to right, #1A2980 0%, #26D0CE 51%, #1A2980 100%);
/* padding-left: 40px;
padding-right: 40px; */
padding: 15px 45px;
font-weight: 700;
font-size: 400;
color: aliceblue;
text-align: center;
text-transform: uppercase;
transition: 0.5s;
background-size: 200% auto;
}
button:hover {
background-position: right center; /* change the direction of the change here */
color: #fff;
text-decoration: none;
}
.results{
color: aliceblue;
margin-top: 30px;
font-size:300;
/* border: 1px solid rgba(255,255,255,0.18); */
border-radius: 7px;
/* background: linear-gradient(125deg, rgba(255,255,255, 0.2),rgba(255,255,255,0)); */
box-shadow: 0 8px 32px 0 rgba(0, 0, 0, 0.1);
padding: 10px;
transition : 0.5s
}
.btn-grad {
background-image: linear-gradient(to right, #1A2980 0%, #26D0CE 51%, #1A2980 100%);
margin: 10px;
padding: 15px 45px;
text-align: center;
text-transform: uppercase;
transition: 0.5s;
background-size: 200% auto;
color: white;
box-shadow: 0 0 20px #eee;
border-radius: 10px;
display: block;
}
.btn-grad:hover {
background-position: right center; /* change the direction of the change here */
color: #fff;
text-decoration: none;
}
JavaScript Functionality:
Finally, let's add JavaScript to calculate the BMI and display the result.
const form = document.querySelector('form')
form.addEventListener('submit', function(e){
e.preventDefault() //preventDefault is used to prevent form default actions.
const height = parseInt(document.querySelector('#height').value)
const weight = parseInt(document.querySelector('#weight').value)
// const weightGuide = document.querySelector('.guide')
const result = (document.querySelector('.results'))
if(height === '' || height < 0 || isNaN(height)){
result.innerHTML = `Please give a valid Height ${height}`;
}else if(weight === '' || weight < 0 || isNaN(weight)){
result.innerHTML = `Please give a valid Weight ${weight}`;
} else{
// bmi formula
const bmi = (weight / ((height*height)/10000)).toFixed(2);
// Result time.
if(bmi < 18.6){
result.innerHTML= `You are Under weight: ${bmi}`;
result.style.color = "black";
result.style.backgroundColor = "Yellow";
} else if(bmi > 18.6 && bmi < 24.9){
result.innerHTML = `You are Normal: ${bmi}`;
result.style.backgroundColor = "Green";
result.style.color = "aliceblue"
style.color = "Green"
} else if(bmi > 24.9){
result.innerHTML = `Do Exercise you are Overweight: ${bmi}`;
result.style.backgroundColor = "red";
console.log(bmi);
}
})
Now let me explain behind the scene of JS code.
Here you can see i selected form using query selector document.querySelector('form'
and i used an event listener with a submit js event form.addEventListener('submit', function(e){
and i selected height and width class from the HTML i created and i sued parseInt method to convert values into integer.
Then grab the result class from the HTML const result = (document.querySelector('.results'))
if(height === '' || height < 0 || isNaN(height)){
result.innerHTML = `Please give a valid Height ${height}`;
}else if(weight === '' || weight < 0 || isNaN(weight)){
result.innerHTML = `Please give a valid Weight ${weight}`;
} else{
I cropped some important code part of Javascript above that make ease to understand. So there you can see i used if loop to verify some conditions such as if class "height" equal to "empty" or "null" or height less then "0" or if entered value is not integer then it will print "Please give a valid Height" and same conditions goes with weight too.
Now If the both condition satisfy here then it will do the calculation using BMI formula (weight / ((height*height)/10000)).toFixed(2);
in the end of the line i used .toFixed javascript method to avoid extra values after decimal point. So basically it will grab only 2 values after the decimal point.
// Result time.
if(bmi < 18.6){
result.innerHTML= `You are Under weight: ${bmi}`;
result.style.color = "black";
result.style.backgroundColor = "Yellow";
You can see above code part there is also have some condition using if loop.
So the "bmi" is the identifier name of BMI formula where i stored calculated result to return the result into the HTML result section. So if the result of bmi after calculated is less then 18.6 then it will print You are Under weight with bmi result under the screen.
result.style.color = "black";
this code convert the result text into black to make perfect visibilty to in yellow background to user for under weight persons.
else if(bmi > 18.6 && bmi < 24.9)
result.innerHTML = `You are Normal: ${bmi}`;
result.style.backgroundColor = "Green";
result.style.color = "aliceblue"
}
Now a little bitt different condition goes with here. Here i defined two conditions using if loop. The condtion is if bmi(result) value greater than the 18.6 either less than 24.9 then it print You are normal : with bmi result value.
and result.style.backgroundColor = "Green"
is used to change the background color into green for the normal patient.
and result.style.color = "Aliceblue"
is used to change the text color into aliceblue.
and same thing goes with thired condition that is overweight.
CLick here to see Live BMI calculator : BMI Calculator
We appreciate you stopping by to explore this content. Thank you!
Subscribe to my newsletter
Read articles from Abdul Shamad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
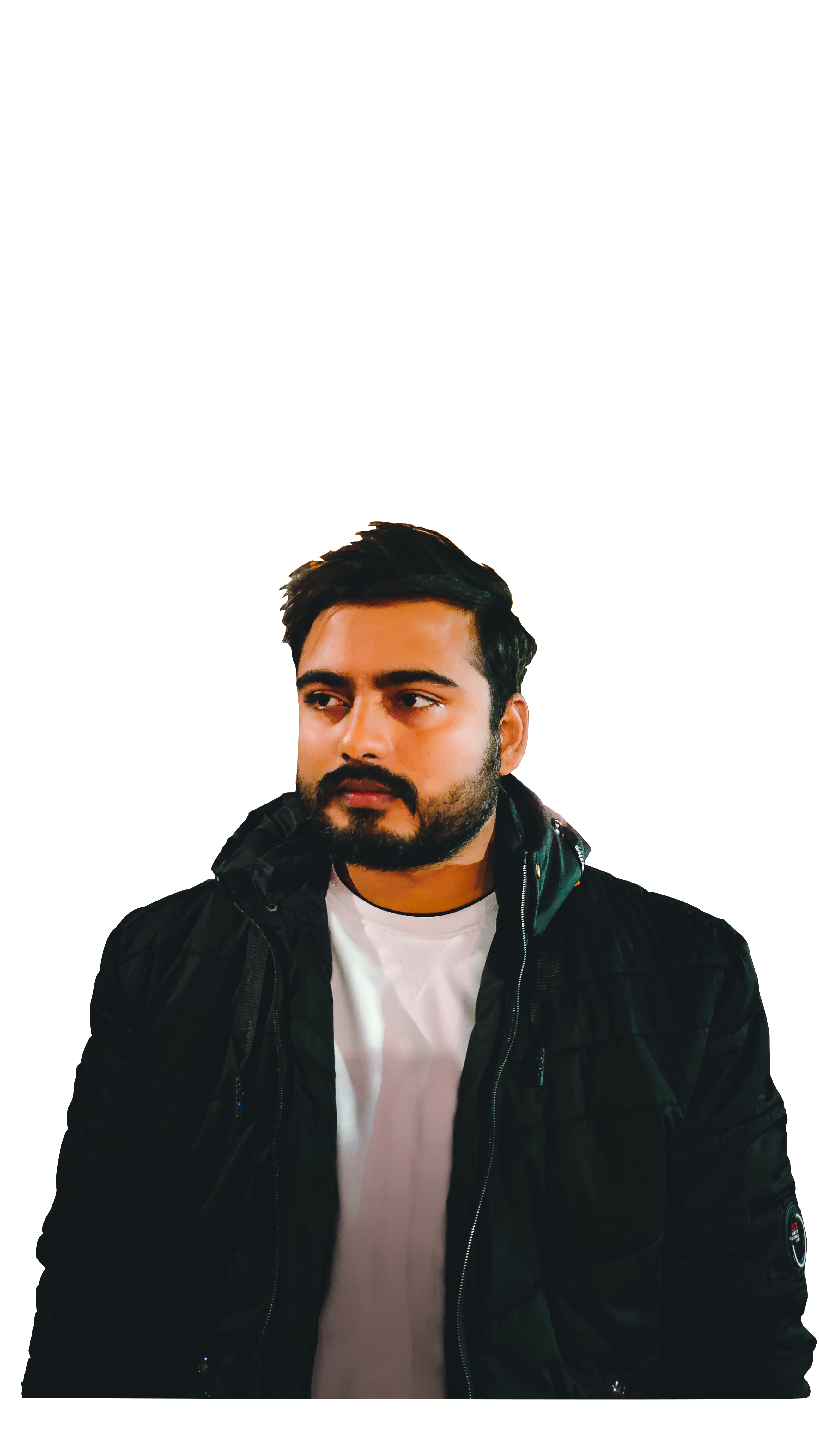
Abdul Shamad
Abdul Shamad
Hello! I'm Abdul Shamad, a passionate and innovative web developer with a mission to bring digital ideas to life.💻 🚀 As a lifelong learner in the ever-evolving world of web development, I thrive on staying at the forefront of emerging technologies and trends. My journey began with a fascination for coding and has evolved into a full fledged career dedicated to crafting exceptional online experiences. 🌐 My expertise spans across a wide spectrum of web technologies, including: • Front-end development: Transforming designs into responsive, user-friendly interfaces using HTML5, CSS3, Javascript, and modern frameworks like React. • Back-end development: Building robust, scalable server-side applications with languages like Node.js • Database management: Crafting efficient data solutions with database like MongoDB.