Exploring sessionStorage: A Guide to Temporary Data Storage

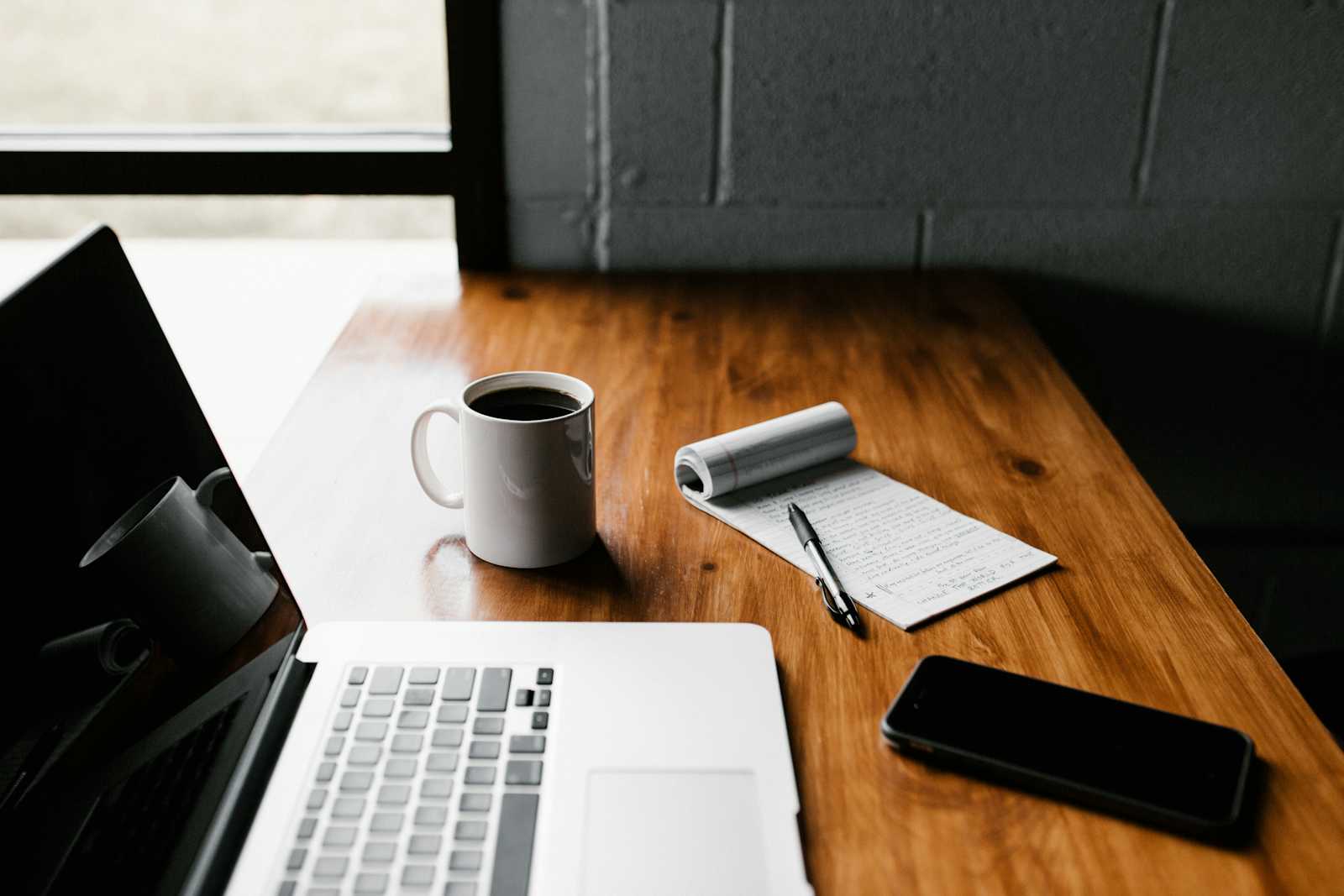
In the realm of web development, managing data efficiently is paramount to providing users with seamless experiences. One key player in this domain is sessionStorage, a client-side storage mechanism that offers temporary data storage within the user's browser session. Unlike localStorage, which persists data even after the browser is closed, sessionStorage holds onto data only for the duration of the page session. Let's delve into the intricacies of sessionStorage, its implementation, practical applications, and how to integrate it into popular frameworks like React.js and Next.js.
Understanding sessionStorage:
Temporary Data Storage: sessionStorage provides a temporary storage solution for web applications. It maintains data for the duration of the page session, allowing developers to store and access information as long as the user navigates within the same browsing session.
Isolated to the Page Session: Unlike localStorage, which retains data across multiple browser sessions, sessionStorage is isolated to the current browsing session. Once the session ends—either by closing the browser tab or navigating away from the page—the stored data is cleared automatically.
Implementing sessionStorage:
Setting and Retrieving Data: Utilizing sessionStorage in JavaScript is straightforward. The sessionStorage object offers methods to set and retrieve data, similar to localStorage.
// Set data
sessionStorage.setItem('key', 'value');
// Retrieve data
const data = sessionStorage.getItem('key');
Clearing and Removing Items: sessionStorage allows developers to clear all stored data or remove specific items as needed, using methods like clear() and removeItem().
// Clear all items
sessionStorage.clear();
// Remove specific item
sessionStorage.removeItem('key');
Practical Use Cases:
Form Data Management: sessionStorage proves handy in managing form data within a single session. It can store user input temporarily, allowing users to navigate between pages without losing their form entries.
User Authentication: Web applications often use sessionStorage to store authentication tokens or user credentials temporarily. This ensures that users remain authenticated within the same browsing session.
Page State Preservation: sessionStorage can preserve the state of a page across multiple interactions. This is useful in scenarios where users navigate back and forth between pages within the same session.
Temporary Preferences: Developers can utilize sessionStorage to store temporary preferences or settings selected by the user during their browsing session, providing a personalized experience without persisting the data beyond the current session.
Integrating sessionStorage into React.js and Next.js:
In React.js and Next.js, sessionStorage can be utilized similarly to vanilla JavaScript. You can access sessionStorage methods directly within your components to manage temporary data storage. Here's a basic example of how you can use sessionStorage in a React component:
import React, { useEffect } from 'react';
const MyComponent = () => {
useEffect(() => {
// Set data
sessionStorage.setItem('key', 'value');
// Retrieve data
const data = sessionStorage.getItem('key');
// Clear all items
sessionStorage.clear();
}, []);
return <div>My Component</div>;
};
export default MyComponent;
Conclusion:
sessionStorage serves as a valuable tool in web development, offering a means to store temporary data within the user's browser session. Its simplicity and temporary nature make it ideal for managing data that is only relevant for the duration of a single browsing session. By understanding how to implement sessionStorage and leveraging its capabilities, developers can enhance user experiences and streamline data management in their web applications, whether using vanilla JavaScript or integrating it into popular frameworks like React.js and Next.js.
Subscribe to my newsletter
Read articles from SANKALP HARITASH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

SANKALP HARITASH
SANKALP HARITASH
Hey 👋🏻, I am , a Software Engineer from India. I am interested in, write about, and develop (open source) software solutions for and with JavaScript, ReactJs. 📬 Get in touch Twitter: https://x.com/SankalpHaritash Blog: https://sankalp-haritash.hashnode.dev/ LinkedIn: https://www.linkedin.com/in/sankalp-haritash/ GitHub: https://github.com/SankalpHaritash21 📧 Sign up for my newsletter: https://sankalp-haritash.hashnode.dev/newsletter