React Dynamic Routing

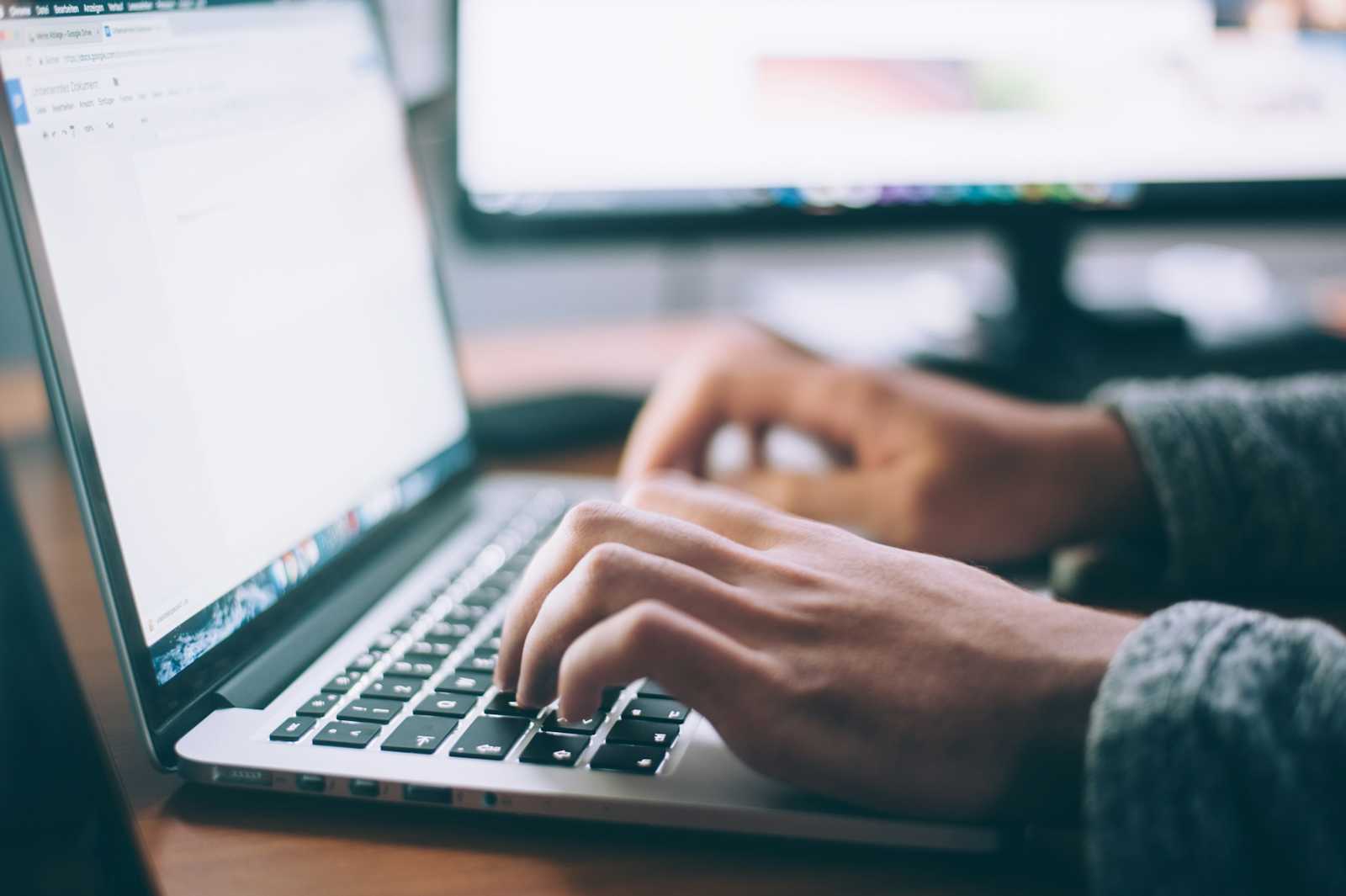
Hello,
So today we are going to handle routing in react. There are several ways that this can be achieved but today I will show you a clean way that I handle my routing. Having routes in you App file can get messy really fast especially if the project will later scale and be big. What you can do is modularization.
Process
We can define the form of routing that we want. Assume you want protected and public routes. Then you create a folder called types
inside create a file routes.ts
. Pass the Details of your routes here.
export type RoutesProps = {
path: string;
isProtected: boolean;
component: JSX.Element;
};
Now you can add the various paths that can be found in your project in the same file. Naming is very crucial in this format. I helps in a picking up the routes easy. The route is defined and assigned the path. The list can be as long as you wish.
export enum Routes {
Login = '/',
Register = '/register',
Home = '/dashboard',
Users = '/users'
UserProfile = '/users/:id'
Error = '*',
}
Now in the src
create a new folder called routes
. Create two files a provider file and the route definition file. We will start with the route definition, name this file routes.tsx
. Here you define whether the route and component will be protected or if it will be public.
const routes: RoutesProps[] = [
{
path: Routes.Login,
isProtected: false,
component: <Login />,
},
];
Now we create the provider for the routes. Here the routes will be mapped from the list of all the routes that have been defined. Create a file routeProvider.tsx
that defines the logic of your protected routes and those of your public routes then that can be used in the RoutesWrapper for authentication.
const RouterProvider = () => {
const renderRoutes = routes.map(
({
path,
component,
isProtected,
}: RoutesProps) => {
const RoutesWrapper = isProtected ? ProtectedRoute : PublicRoute;
return (
<Route
key={path}
path={path}
element={
<RoutesWrapper>
{component}
</RoutesWrapper>
}
/>
);
},
);
return (
<BrowserRouter>
<Routes>{renderRoutes}</Routes>
</BrowserRouter>
);
};
export default RouterProvider;
After that you are done with the routing in the application. Now all that is left is call the router in our App.jsx
const Demo = () => {
return (
<>
<RouterProvider />
</>
);
};
This method cleans up the app file as the only the global and necessary providers are called. Now you router is available in the whole application. Huraay!!!!!
To test you can create a component and call the router there. An example
<Link to={Routes.Login} />
And just like that the routing has been handled. Enjoy a clean and efficient way of handling routing in your react application.
Subscribe to my newsletter
Read articles from George Onyango directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
