Formatting Strings in Python: The Easy Way

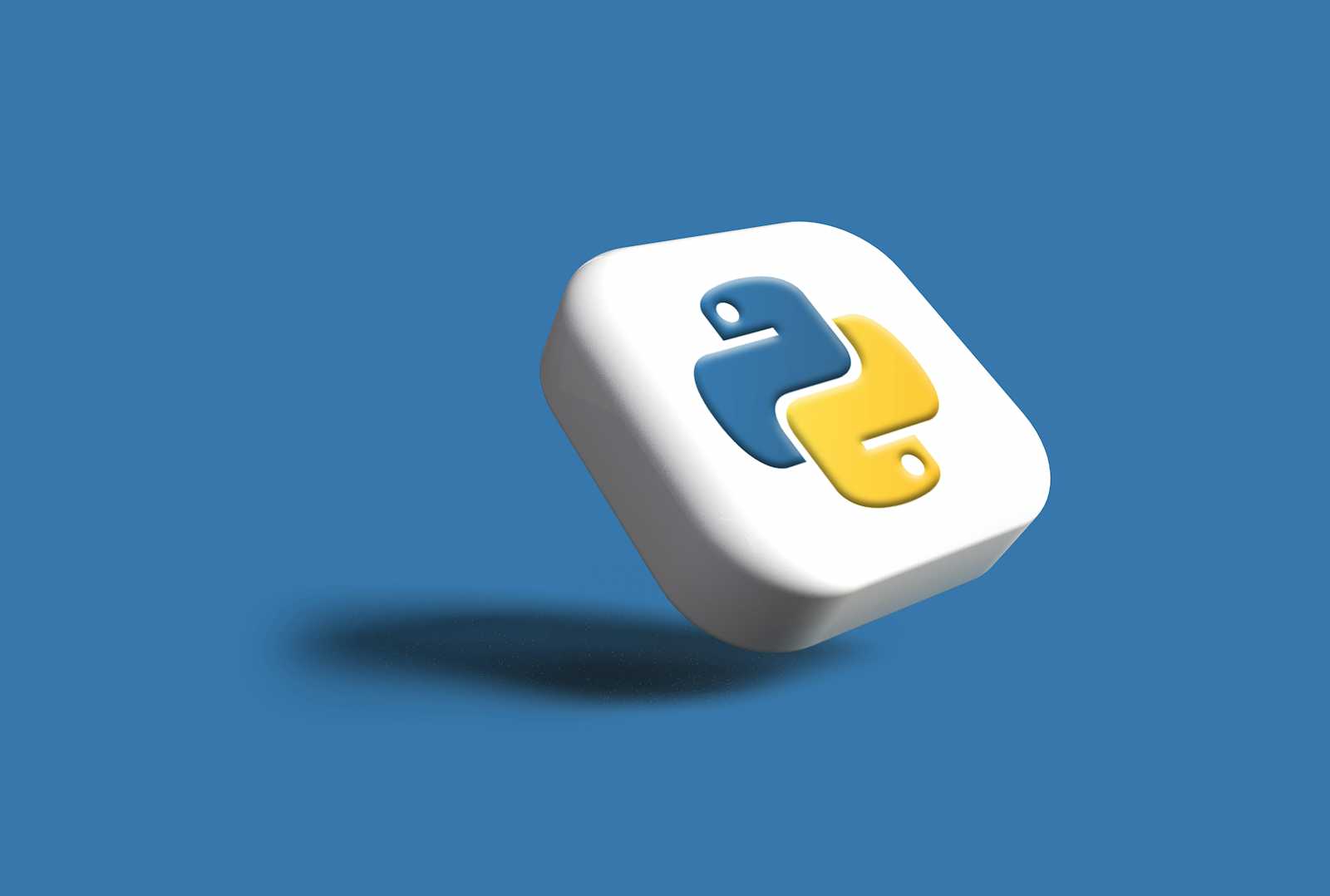
Python allows you to insert text and variables into strings to create informative messages. There are two main ways to do this:
Old Style Formatting (Less Flexible)
Uses a percent sign
%
and numbers to specify placeholders for your data.Not easy to read or understand for somplex formatting.
New Style Formatting (More Flexible and Recommended)
Uses curly braces
{}
and names to define placeholders.Offers more control over how your data is displayed (alignment, padding etc.).
Let's see some examples!
Basic Formatting:
name = "John"
# Old style (confusing)
greeting = "Hello, %s" % name # %s is the placeholder
# New style (better)
greeting = "Hello, {}".format(name) # {} is the plaaceholder
print(greeting) # Output: Hello, Alice
Making Things Clearer:
New style formatting allows you to give cleare names to your placeholders, making your code easier to understand, especially when dealing with multiple pieces of data.
person = {"name": "John", "age": 21}
# Old style (confusing)
message = "My name is %s and I am %d years old." % (person["name"], person["age"])
# New style (better)
message = "My name is {name} and I am {age} years old.".format(**person) # **person unpacks the dictionary
print(message) # Output: My name is John and I am 21 years old.
Advanced Formatting Options
Padding with zeros:
money_formatted = "{:>10.2f}".format(123.4567) # Right align, 10 spaces, 2 decimal places
print(money_formatted) # Output: 123.46
Shortening long text:
long_text = "This is an extremely long sentence that goes on and on..."
shortened_text = "{:.10}".format(long_text) # Shorten to 10 characters
print(shortened_text) # Output: This is an...
Formatting Dates:
from datetime import datetime
now = datetime.now()
# Date only (YYYY-MM-DD)
formatted_date = "{:%Y-%m-%d}".format(now)
print(formatted_date) # Example output: 2024-03-11
# Date and time (YYYY-MM-DD HH:MM:SS)
formatted_datetime = "{:%Y-%m-%d %H:%M:%S}".format(now)
print(formatted_datetime) # Example output: 2024-03-11 19:23:11
Key tips:
Use new style formatting
.format()
for better readability and flexibility.Give clear names to your placeholders to make your code easier to understand.
Play around with formatting options to achieve the results you want.
Thank you for reading ๐
Subscribe to my newsletter
Read articles from Thabani Takwena directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Thabani Takwena
Thabani Takwena
I am a full-stack Developer based in South Africa.