Building a simple price comparison server with nextjs

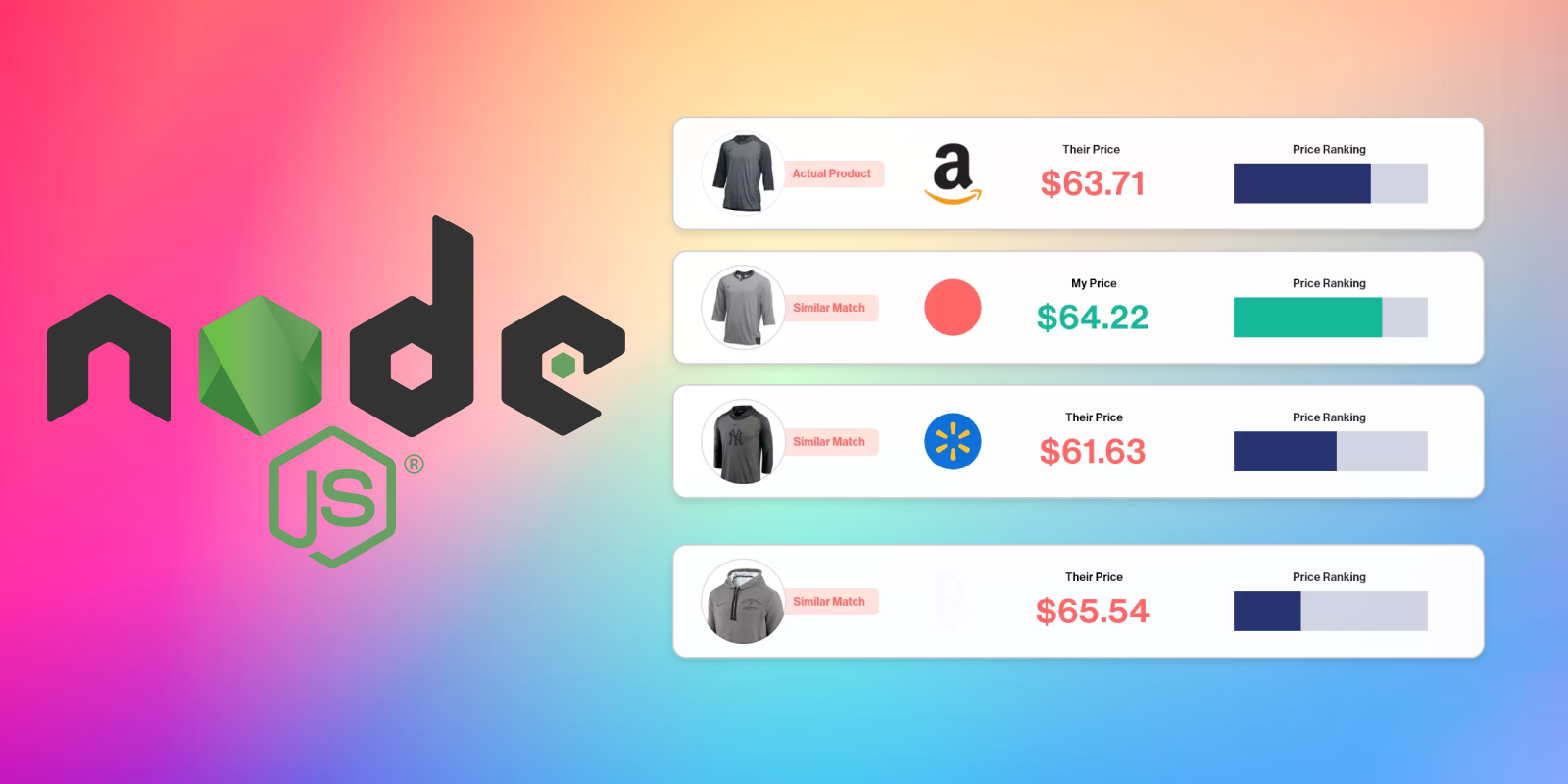
Hey there, tech enthusiasts! Get ready for a wild ride as we dive into the world of string matching and price comparison with a dash of Node.js magic! ๐
In this tutorial, we'll be building a simple Express server that can compare product names across multiple stores and return the matching products along with their prices. Buckle up, folks, because we're about to have some serious fun! ๐ค
First things first, let's set up our project. We'll need to install the necessary dependencies:
npm install express string-similarity
Now that we've got our dependencies sorted, it's time to start coding! Create a new file called app.js
and let's get cracking.
const express = require("express");
const app = express();
const stringSimilarity = require("string-similarity");
We're importing the express
module to create our server and the string-similarity
library to help us compare product names. Because, let's be real, people can be pretty creative when it comes to naming products!
Next, we'll define our sample product data from three different stores:
// Sample product data
const store1Products = [
{ name: "Whole Milk", price: 2.99 },
{ name: "Organic Apples", price: 3.49 },
{ name: "Cheddar Cheese", price: 4.99 },
];
const store2Products = [
{ name: "Dairy Pure Milk", price: 2.79 },
{ name: "Organic Apples", price: 3.29 },
{ name: "Sharp Cheddar", price: 5.49 },
];
const store3Products = [
{ name: "Milk Whole", price: 2.59 },
{ name: "Apples Organic", price: 3.99 },
{ name: "Cheddar Cheese Block", price: 4.79 },
];
Now, we need to define some helper functions to normalize and compare strings:
function normalizeString(str) {
return str.toLowerCase().replace(/\W/g, "");
}
function compareTwoStrings(str1, str2) {
const normalized1 = normalizeString(str1);
const normalized2 = normalizeString(str2);
return stringSimilarity.compareTwoStrings(normalized1, normalized2);
}
The normalizeString
function converts the input string to lowercase and removes all non-word characters (like punctuation and whitespace). The compareTwoStrings
function uses the normalizeString
function to preprocess the input strings and then compares them using the stringSimilarity.compareTwoStrings
method.
Now, for the main event! We'll set up an Express route to handle our price comparison requests:
app.get("/compare-prices", (req, res) => {
const query = req.query.query || "";
const threshold = 0.4; // Adjust this threshold as needed
const allProducts = [...store1Products, ...store2Products, ...store3Products];
console.log(allProducts.length);
const matchingProducts = allProducts.filter((product) =>
compareTwoStrings(product.name, query) > threshold
);
res.json(matchingProducts);
});
When a GET request is made to /compare-prices
, we first retrieve the search query from the query string (req.query.query
). We also set a similarity threshold (in this case, 0.4) to determine how similar the product names need to be to be considered a match.
Next, we combine the product data from all three stores into a single array (allProducts
). We then use the filter
method to find all products whose names are similar enough to the search query (based on the compareTwoStrings
function and the specified threshold).
Finally, we send the matching products as a JSON response (res.json(matchingProducts)
).
To start our server, we'll add the following lines:
const port = process.env.PORT || 3000;
app.listen(port, () => console.log(`Server running on port ${port}`));
And that's it, folks! Our price comparison server is up and running! ๐
To test it out, you can start the server with node app.js
and then make a GET request to http://localhost:3000/compare-prices?query=milk
. You should see an array of matching products from all three stores.
Of course, this is just a simple example, but you can expand on it and add more features like authentication, database integration, and even a fancy user interface. The possibilities are endless!
Happy coding, my fellow tech wizards! And remember, always have fun and don't be afraid to experiment. Who knows, you might just create the next big thing in price comparison technology! ๐ฉ
Clone the repo:
git clone https://github.com/your-username/price-comparison-server.git
Subscribe to my newsletter
Read articles from Siso Ngqolosi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
