Dart Null Safety
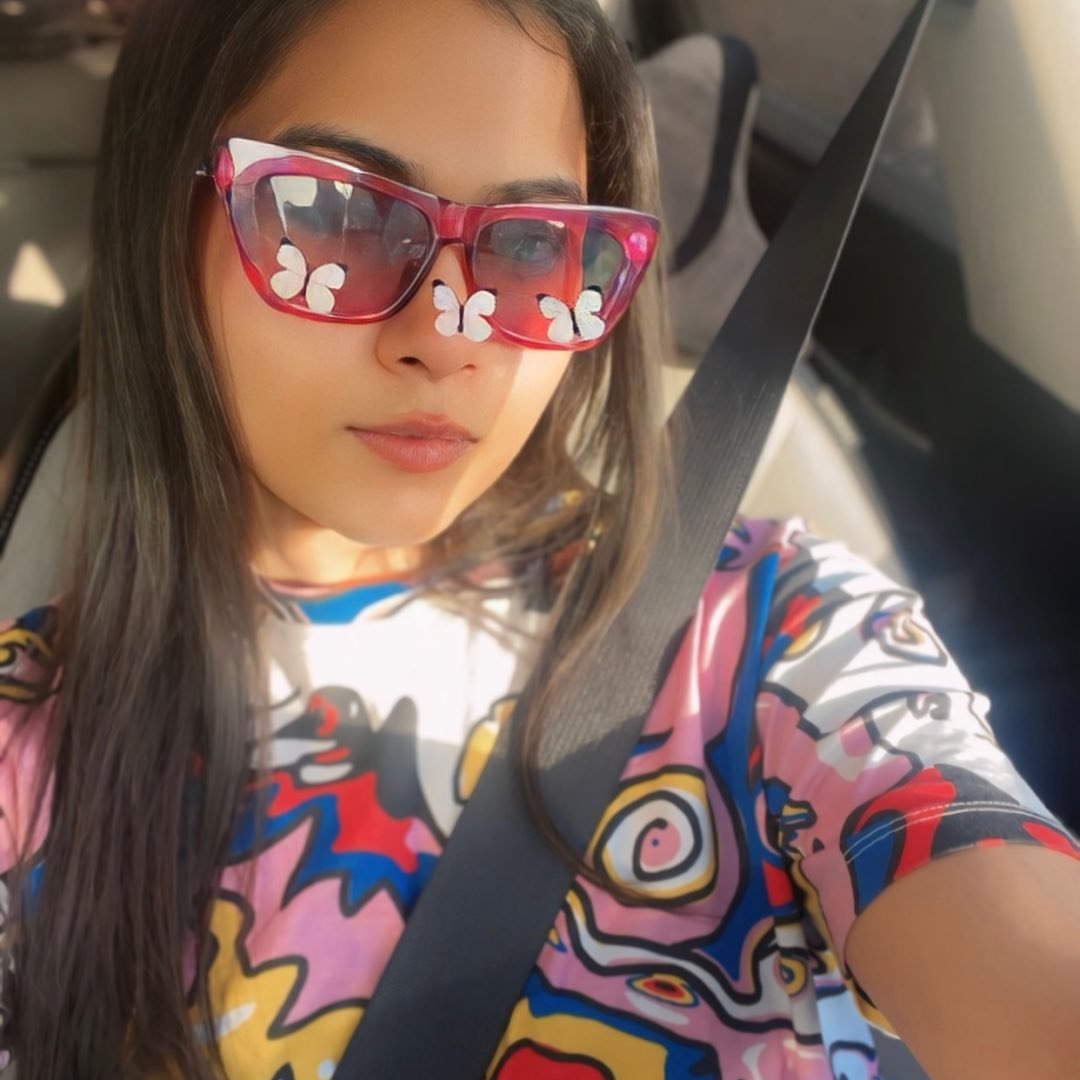
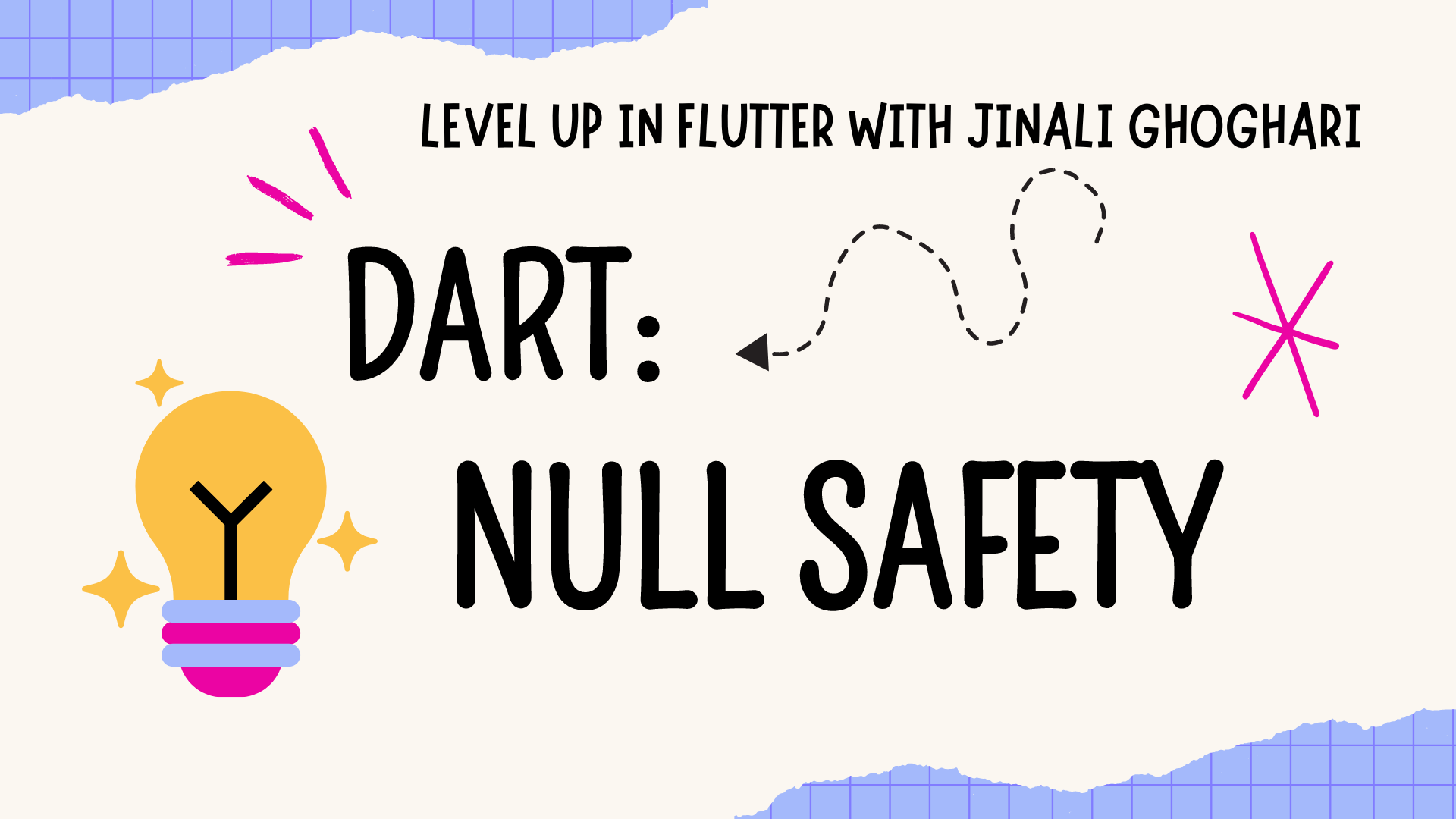
As we knew that all variables are non-null by default, we can use either the ? operator or the late keyword.
Null safety in simple words means a variable cannot contain a null value unless you initialized with null to that variable.
with null safety, all runtime null-dereference errors will now be shown in compile time.
String name = null ; // This means the variable name has a null valu
Null-Nullable Types
When we use null safety, all the types are non-nullable by default.
When we declare a variable of type int, the variable will contain some integer value.
void main() {
int marks;
// The value of type `null` cannot be
// assigned to a variable of type 'int'
marks = null;
}
Non-nullable variables must always be initialized with non-null values.
Nullable Types
To specify if the variable can be null, then you can use nullable type ? operator.
String? carName; // initialized to null by default
int? marks = 36; // initialized to non-null
marks = null; // can be re-assigned to null
The Assertion Operator (!)
Use the null assertion operator ( ! ) to make Dart treat a nullable expression as non-nullable if you’re certain it isn’t null.
int? someValue = 30;
int data = someValue!; // This is valid as value is non-nullable
In the above example, we are telling Dart that the variable someValue
is null, and it is safe to assign it to a non-nullable variable i.e. data
.
Type Promotion
Dart's analyzer, which tells you what compile-time errors and warnings, is intelligent enough to determine whether a nullable variable is guaranteed to have values that are not null.
Dart uses Flow Analysis at runtime for type promotion.
int checkValue(int? someValue) {
if (someValue == null) {
return 0;
}
// At this point the value is not null.
return someValue.abs();
}
void main(){
print(checkValue(5));
print(checkValue(null));
}
In the above code, if statement checks if the value is null or not.
After the if statement value cannot be null and is treated as non-nullable value.
This allows us to safely use someValue.abs().
Here, .abs()
function will return an absolute value.
Late Keyword
As we knew that all variables are non-null by default, we can use either the ? operator or the late keyword.
String? carName; // using ? operator
late String bikeName; // using "late" keyword
Subscribe to my newsletter
Read articles from Jinali Ghoghari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
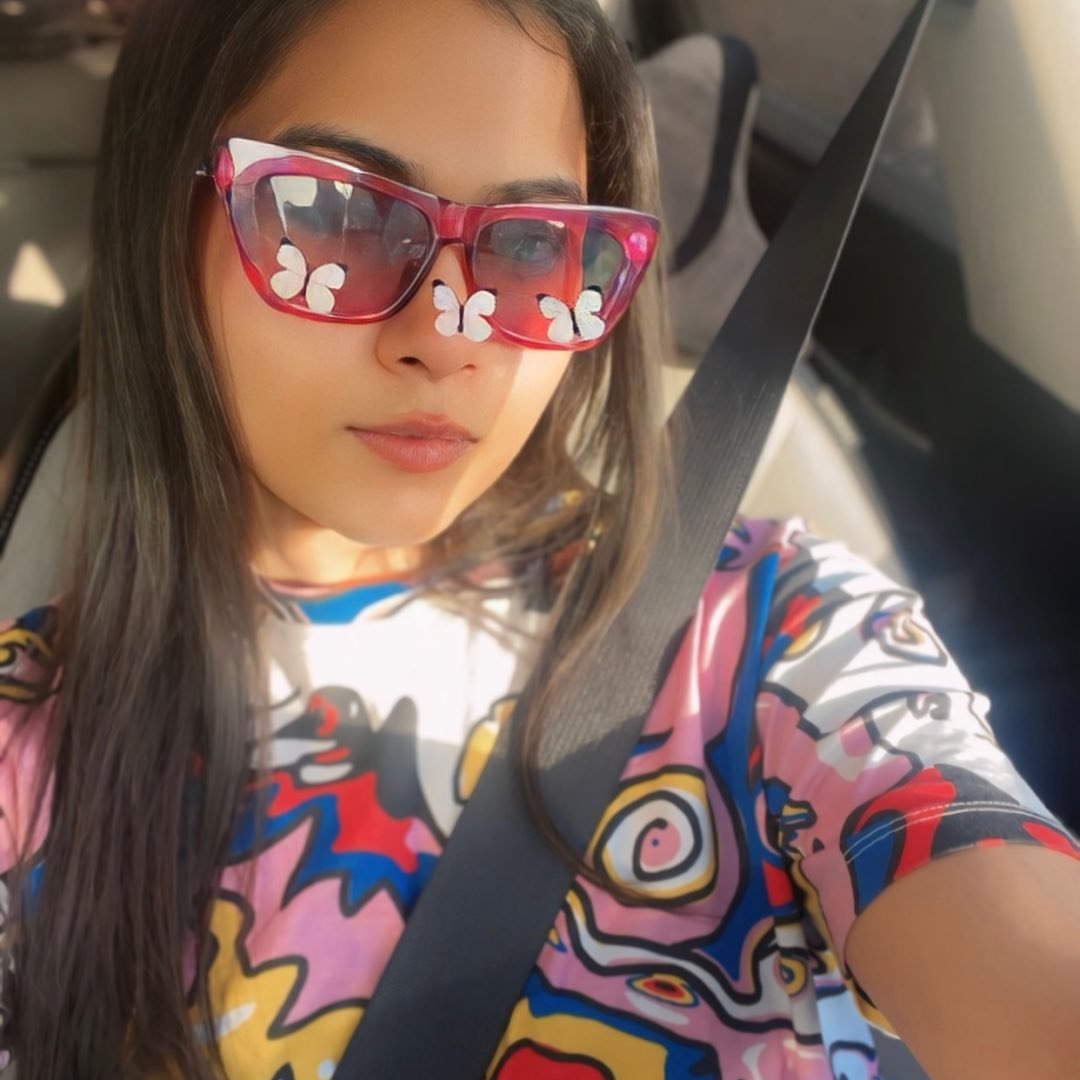