Reference equality in JavaScript
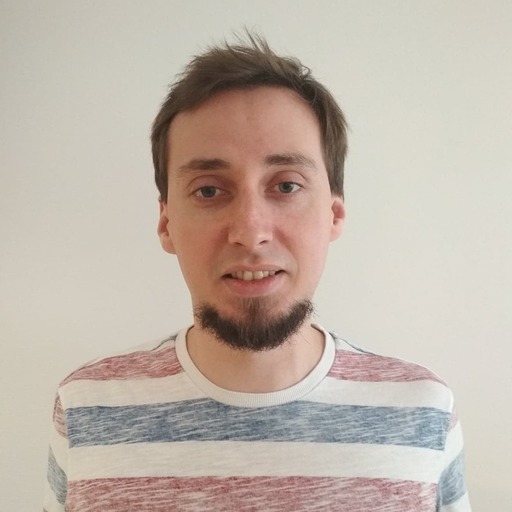
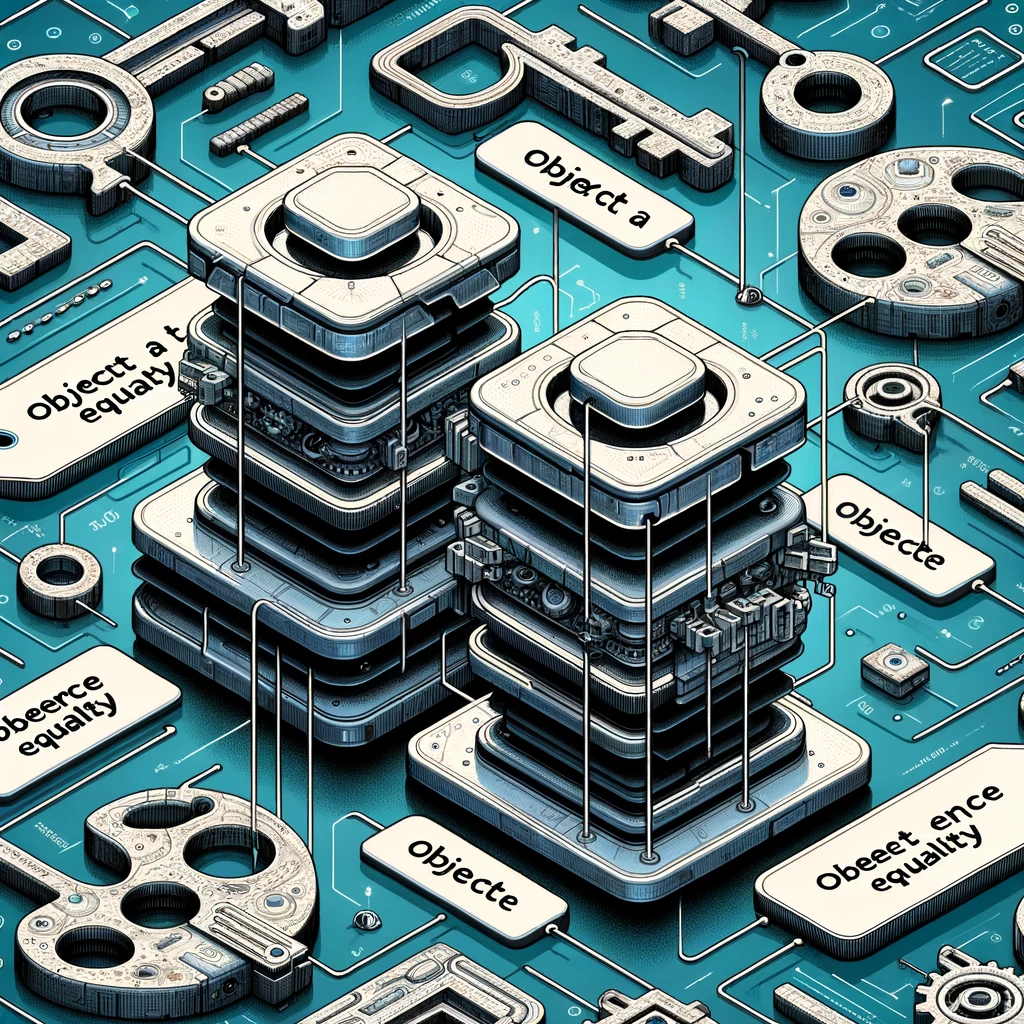
Introduction: JavaScript, being a versatile and dynamic language, offers various ways to compare values. While primitive types like numbers and strings are compared based on their content, objects and arrays are compared based on their reference in memory. This concept, known as reference equality, is fundamental to understanding how JavaScript handles complex data structures. In this article, we'll delve deeper into reference equality, its implications, and how it affects your JavaScript code.
What is Reference Equality?: Reference equality in JavaScript refers to comparing two objects or variables to determine if they refer to the same object in memory. Unlike primitive types where values are compared directly, objects and arrays are compared based on their memory addresses. If two variables reference the exact same object, they are considered equal; otherwise, they are not.
Understanding Object Comparisons: Consider the following example:
let obj1 = { name: 'John' };
let obj2 = { name: 'John' };
console.log(obj1 === obj2); // Output: false
In this case, even though both objects have the same properties and values, they are stored in different memory locations. Hence, the comparison obj1 === obj2
evaluates to false
.
Reference vs. Value: Understanding reference equality involves grasping the distinction between comparing by reference and comparing by value. Primitive types, like strings and numbers, are compared by value, meaning their content is directly compared. However, objects and arrays are compared by reference, where their memory addresses determine equality.
Implications in Function Arguments: Understanding reference equality is crucial when passing objects or arrays as function arguments. Consider a scenario where a function modifies an object passed to it as an argument:
function modifyObject(obj) {
obj.name = 'Alice';
}
let originalObj = { name: 'Bob' };
modifyObject(originalObj);
console.log(originalObj); // Output: { name: 'Alice' }
Here, originalObj
is modified within the function modifyObject()
because the function operates directly on the reference to the object, not a copy of it.
Optimizing Performance with Reference Equality: Reference equality can also be leveraged to optimize performance in JavaScript. By comparing object references instead of their contents, you can quickly determine if two objects are the same, which can be useful in scenarios like caching or memoization.
Conclusion: Reference equality is a fundamental concept in JavaScript that dictates how objects and arrays are compared. By understanding reference equality, you gain insights into how JavaScript handles complex data structures and how to effectively manage them in your code. Remember, when dealing with objects and arrays, always consider their references in memory to ensure your comparisons yield the expected results.
Understanding reference equality empowers you to write more robust and efficient JavaScript code, ultimately enhancing the performance and maintainability of your applications. So, embrace reference equality in your JavaScript journey, and unlock its potential to write cleaner and more effective code.
Subscribe to my newsletter
Read articles from Dawid Paszek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
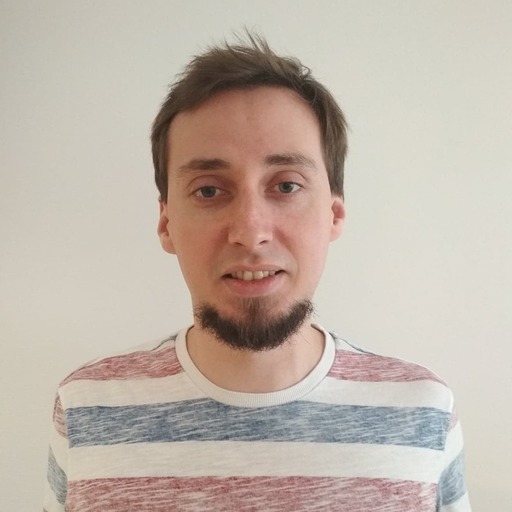