Exploring Essential Number Methods and Math object in JavaScript
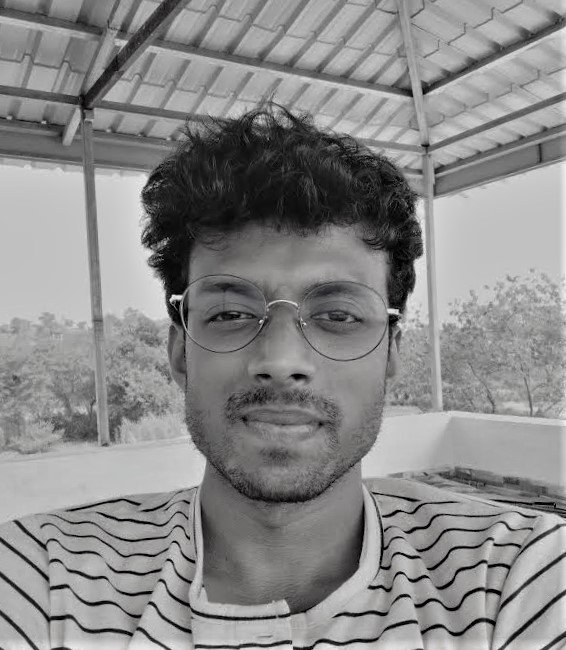
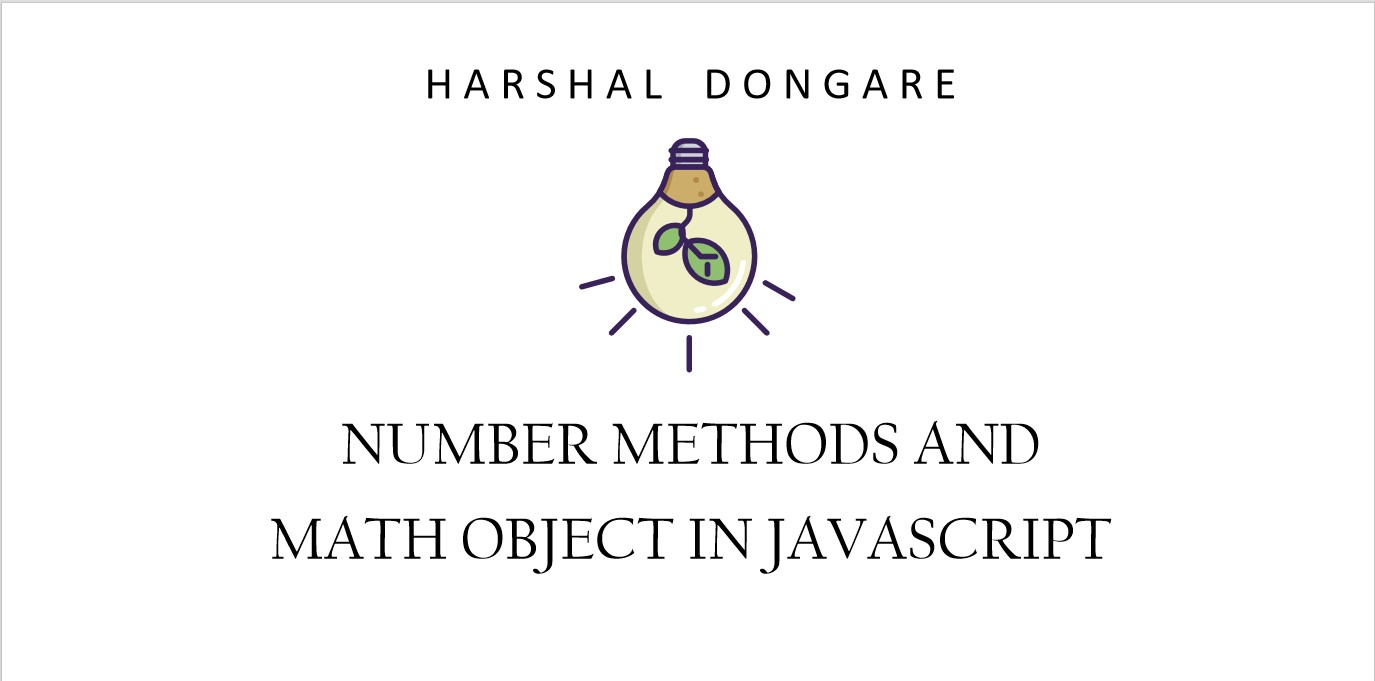
Most common methods on number data type
tostring()
: A method which converts a number into string format.const stuId = "456134"; const newStuId = stuId.tostring(); console.log(newStuId); // Output: 456134
toFixed(digits)
: A method which converts the number to string first and then rounds of the decimal part of the number upto specified digits, returning the result as a string.const totalBudget = 45264.36315; console.log(totalBudget.toFixed(2)); // Output: "45264.36"
toPrecision()
: A method which formats a number to a specified length. A decimal point and nulls are added (if needed), to create the specified length. The return result is in the string format.const stockPrice = 555.236; console.log(stockPrice.toPrecision(3)); // Output: 555 const stockPrice2 = 55.236; console.log(stockPrice.toPrecision(3)); // Output: 55.2
toLocaleString()
: A method is used to convert a number, date, or array into a string using the locale-specific formatting options. It returns a string representing the object in a format that is appropriate for the specified locale.const million = 1000000; const indianSystem = million.toLocaleString('en-IN'); console.log(indianSystem); // Output: 10,00,000
parseInt(string, base[optional])
: A function used to parse a string and convert it to an integer. It takes two parameters, the string to be parsed and and an optional parameter called the base of the numeral system. By default the base is 10.let str = "123"; let num = parseInt(str); console.log(num); // Output: 123
parseFloat(string)
: A method used to parse a string and convert it to a floating point number. It parses the input until it encounters a character that cannot be a part of floating point number and then it stops.let numStr = "3.14"; let circumference = "4.567abcdefgh"; let radius = "fdsasd"; console.log(parseFloat(numStr)); // Output: 3.14 console.log(parseFloat(circumference)); // Output: 4.567 console.log(parseFloat(radius)); // Output: NaN
toExponential(digit)
: A method used to convert a number into its exponential notationlet num = 12345.6789; let exponentialStr = num.toExponential(2); console.log(exponentialStr); // Output: "1.23e+4"
Some methods and properties using Number
Number.MAX_SAFE_INTEGER
: A property represents the maximum safe integer useful when working with integer values that need to stay within the range to ensure accurate representation and avoid loss of precision.console.log(Number.MAX_SAFE_INTEGER); // Output: 9007199254740991
Number.MIN_SAFE_INTEGER
: A property represents the minimum safe integer.console.log(Number.MIN_SAFE_INTEGER); // -9007199254740991
isInteger()
: A method determines whether the passed value is an integer or not. returns true if it is an integer.const amount = 123 console.log(Number.isInteger(amount)) // Output: true
Math Object and its properties
The Math
object in JavaScript is a built-in object that provides mathematical functions and constants. It allows you to perform mathematical tasks without creating an instance of the object.
Math.abs(x)
: Returns the absolute (positive) value of a numberx
.const negValue = -3; const absValue = Math.abs(negValue); console.log(absValue); // Output: 3
Math.round(x)
: Rounds a numberx
to the nearest integerconst value = 4.63; const roundOff = Math.round(value); console.log(roundOff); // Output: 5
Math.ceil(x)
: Returns the smallest integer greater than or equal to a numberx
const value = 4.6; const ceilValue = Math.ceil(value); console.log(ceilValue); // Output: 5
Math.floor(x)
: Returns the largest integer less than or equal to a numberx
const value = 4.6; const ceilValue = Math.floor(value); console.log(ceilValue); // Output: 4
Math.sqrt(x)
: A method is used to calculate the square root of a numberlet number = 16; let squareRoot = Math.sqrt(number); console.log(squareRoot); // Output: 4
Math.pow(base, exponent)
: A method is used to raise a base number to an exponent power. It takes two parameters: the base number and the exponent and return the result as a floating point number.let base = 2; let exponent = 3; let result = Math.pow(base, exponent); console.log(result); // Output: 8
Math.max(value1, value2....ValueN)
: A method used to find maximum value from a list of numberslet maxNumber = Math.max(10, 20, 5, 30); console.log(maxNumber); // Output: 30
Math.min(value1, value2....ValueN)
: A method used to find minimum value from a list of numberslet minNumber = Math.min(10, 20, 5, 30); console.log(minNumber); // Output: 5
Math.random()
: Returns a pseudo-random number between 0 (inclusive) and 1 (exclusive)let randomNumber = Math.random(); console.log(randomNumber); // Output: a random number between 0 and 1 (e.g., 0.8374928184235189)
To generate random numbers within a specific range, you can use
Math.random()
in combination with mathematical operations. For example, to generate a random integer betweenmin
(inclusive) andmax
(inclusive), you can use the formula:Math.floor(Math.random() * (max - min + 1)) + min;
Subscribe to my newsletter
Read articles from Harshal Dongare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
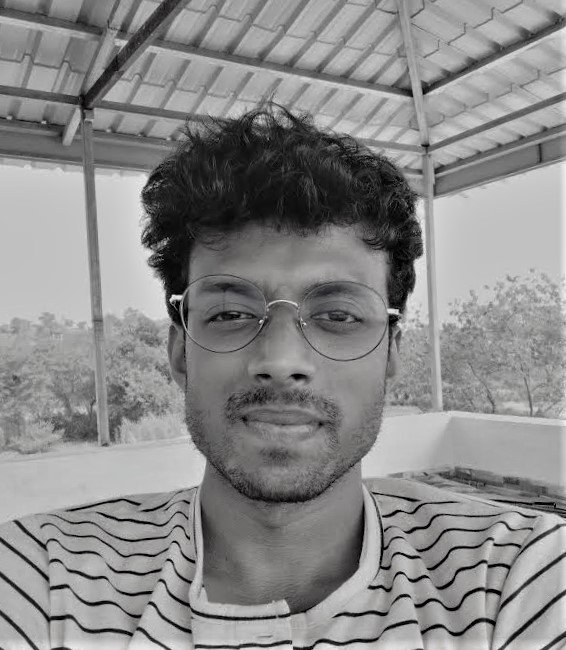
Harshal Dongare
Harshal Dongare
B. Tech IT '20