Dart String Manipulations: Strings RegExp
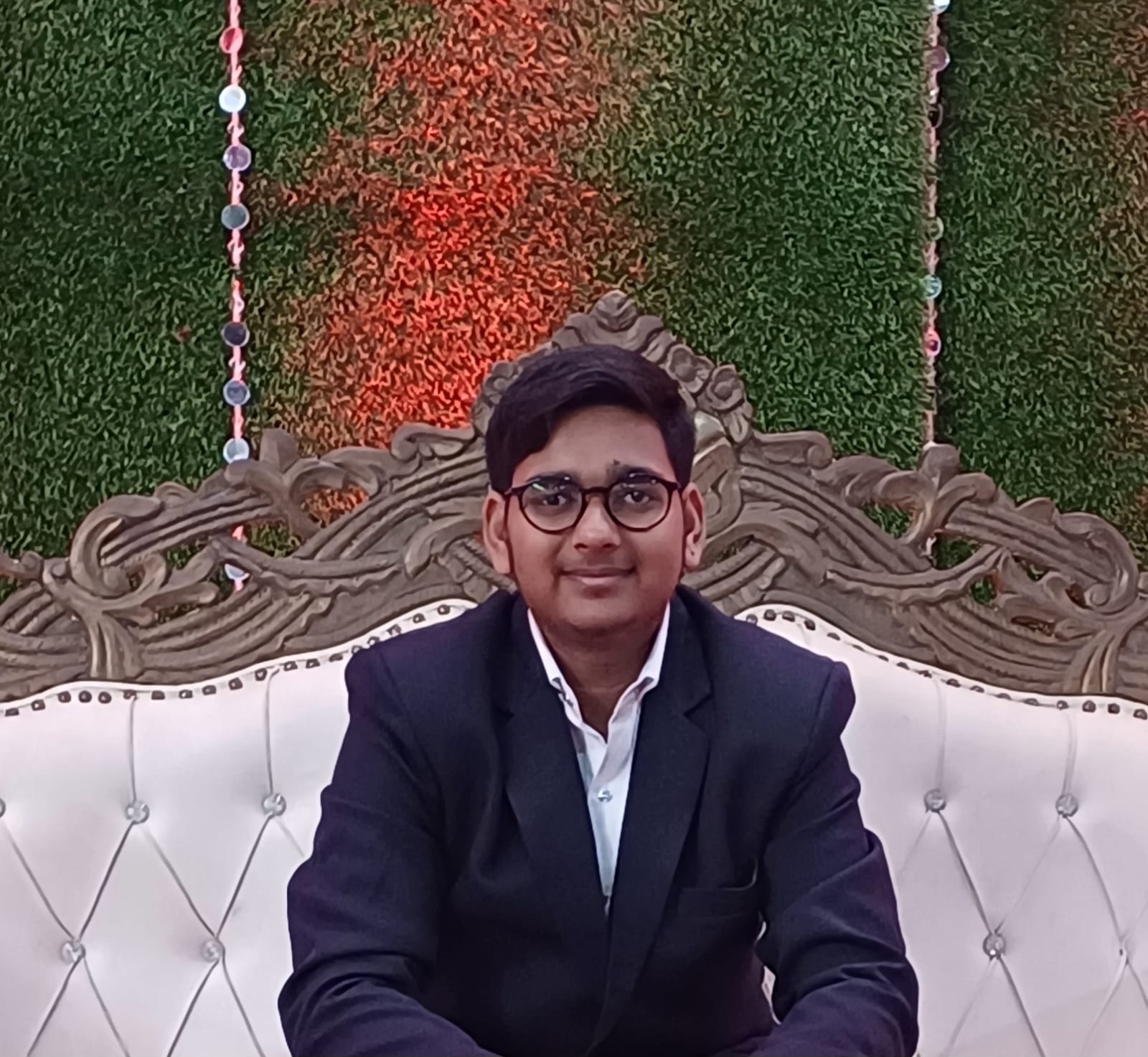
1 min read
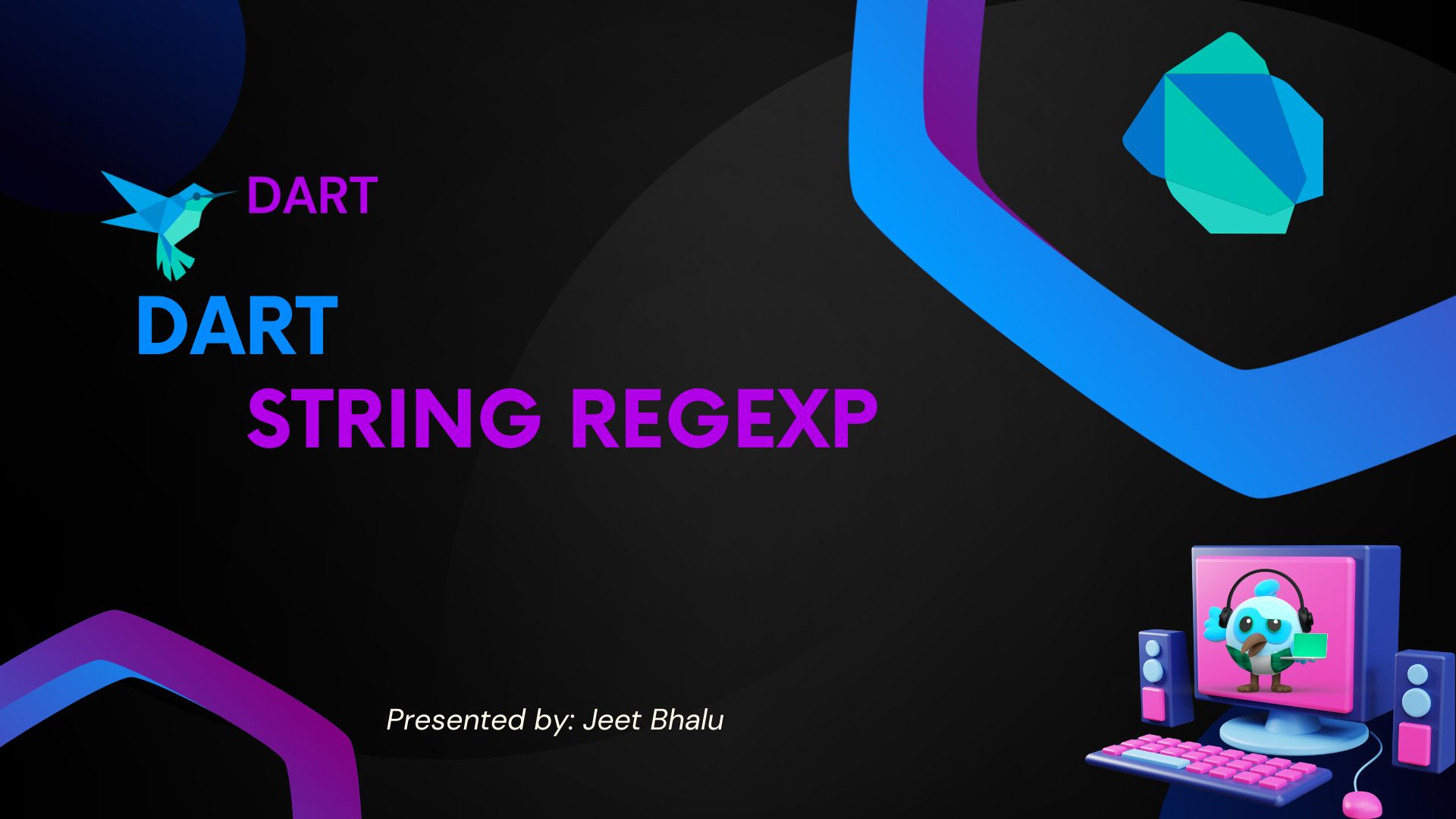
What is RegExp?
Regular expressions are Patterns, and can as such be used to match strings or parts of strings.
Regex in Dart works much like other languages. You use the RegExp to define a matching pattern. Then use hasMatch() to test the pattern on a string.
Example:
void main() {
final alphanumeric = RegExp(r'^[a-zA-Z0-9]+$');
print(alphanumeric.hasMatch('abc123')); // true
print(alphanumeric.hasMatch('abc123%'));
RegExp hexColor = RegExp(r'^#?([0-9a-fA-F]{3}|[0-9a-fA-F]{6})$');
print(hexColor.hasMatch('#3b5')); // true
print(hexColor.hasMatch('#FF7723')); // true
print(hexColor.hasMatch('#000000z')); // false
final myString = '25F8..25FF ; Common # Sm [8] UPPER LEFT TRIANGLE';
// find a variable length hex value at the beginning of the line
final regexp = RegExp(r'^[0-9a-fA-F]+');
// find the first match though you could also do `allMatches`
final match = regexp.firstMatch(myString);
//print(match);
// group(0) is the full matched text
// if your regex had groups (using parentheses) then you could get the
// text from them by using group(1), group(2), etc.
final matchedText = match?.group(0); // 25F8
//print(matchedText);
}
Output:
true
false
true
true
false
0
Subscribe to my newsletter
Read articles from Jeet Bhalu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Dart String Manipulations: Strings RegExpStrings RegExpDart#dart language#dart-for-beginnersdart programming tutorial#dart-keywords#JeetbhaluJeet BhalujeetDart String ManipulationsRegexp
Written by
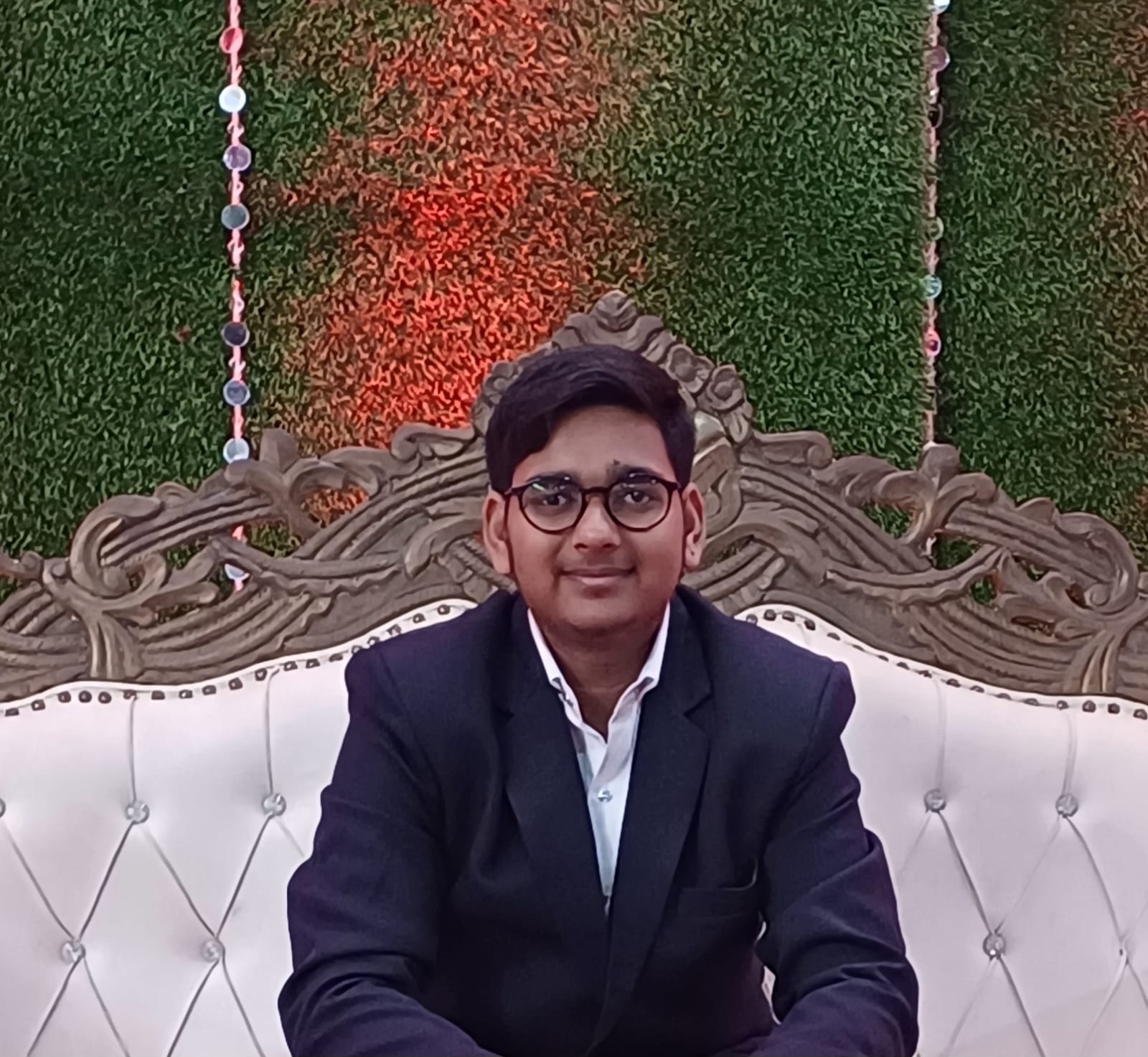
Jeet Bhalu
Jeet Bhalu
i am Jeet Bhalu i am flutter App developer