Python Essentials for DevOps: A Beginner's Guide

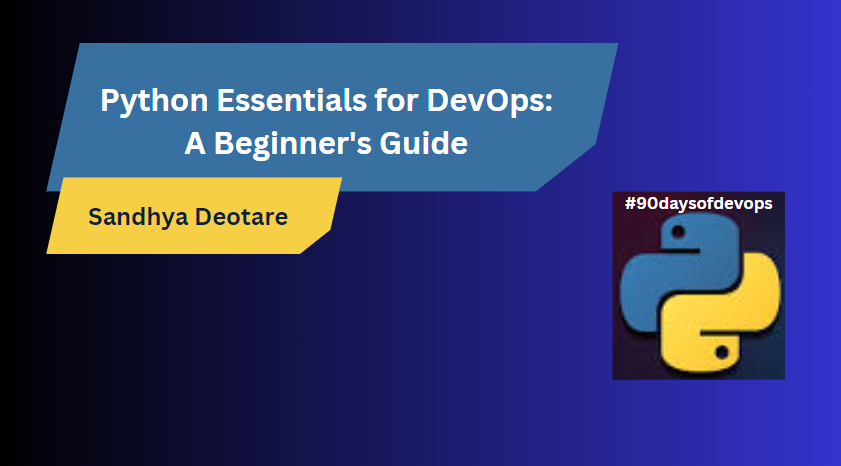
Introduction
Python is a programming language that is widely used and adaptable. DevOps engineers may construct programs and logic with Python to automate processes in their infrastructure. We'll go over the fundamentals of Python in this blog article, including its features, how to install it, and a starter task.
What is Python?
Python is a high-level, general-purpose, object-oriented, and open-source programming language. It was developed by Guido van Rossum and is very well-liked because of its ease of use, readability, and extensive ecosystem of libraries and frameworks. With popular frameworks like Django, TensorFlow, Flask, Pandas, and Keras, Python is a potent tool for a wide range of tasks, including automation, web development, data analysis, and artificial intelligence.
How to Install Python?
Python can be easily installed on various operating systems, including Windows, MacOS, Ubuntu, CentOS, and more. Below are the installation instructions for different platforms:
Windows Installation:
Visit the official Python website click here.
Navigate to the "Downloads" section.
Select the latest version compatible with your system (usually recommended for most users).
Run the installer and make sure to check the box that says "Add Python to PATH" during installation.
Ubuntu Installation:
sudo apt-get update
sudo apt-get install python3.6
This will install Python 3.6 on your Ubuntu system.
Task 1: Getting Started
Step 1: Install Python and Check the Version
Follow the installation instructions provided above for your respective operating system. Once installed, open a terminal or command prompt and type the following command to check the installed Python version:
python --version
Step 2: Understanding Data Types in Python
Python supports several data types, including integers, floats, strings, lists, dictionaries, and more. Familiarizing yourself with these types is crucial for writing effective Python programs.
Numeric Types:
int: Integer data type represents positive or negative whole numbers without any decimal point.
Syntax:
x = 5
Example:
x = 5
float: Floating-point numbers represent real numbers and are written with a decimal point dividing the integer and fractional parts.
Syntax:
y = 3.14
Example:
y = 3.14
String Type:
A string is a sequence of characters enclosed within single quotes, double quotes, or triple quotes.
Syntax:
str = 'Hello'
orstr = "Hello"
Example:
str = 'Hello'
Boolean Type:
Boolean represents a truth value which can be either True or False.
Syntax:
flag = True
orflag = False
Example:
flag = True
List Type:
A list is an ordered and mutable collection of items. Items can be of different data types.
Syntax:
list = [1, 2, 3, 'a', 'b']
Example:
list = [1, 2, 3, 'a', 'b']
Tuple Type:
A tuple is an ordered and immutable collection of items. Items can be of different data types.
Syntax:
tuple = (1, 2, 3, 'a', 'b')
Example:
tuple = (1, 2, 3, 'a', 'b')
Dictionary Type:
A dictionary is an unordered collection of key-value pairs.
Syntax:
dict = {'name': 'Riya', 'age': 30}
Example:
dict = {'name': 'Riya', 'age': 30}
Set Type:
A set is an unordered collection of unique items.
Syntax:
set = {1, 2, 3, 4}
Example:
set = {1, 2, 3, 4}
None Type:
The None type represents the absence of a value or a null value.
Syntax:
x = None
Example:
x = None
Take some time to read about these data types and understand how they are used in Python.
Conclusion
Congratulations! You've taken the first steps towards becoming proficient in Python. In this blog post, we covered the basics of Python, including its features, installation process, and an introductory task to check the Python version and explore different data types.
In the next installment, we'll delve deeper into Python programming concepts, including variables, control structures, and functions, to equip you with the foundational knowledge needed for DevOps automation.
Stay tuned for more Python adventures!
Happy Learning :)
Subscribe to my newsletter
Read articles from Sandhya Deotare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
