Building a Dynamic FAQ Section in ASP.NET Core MVC
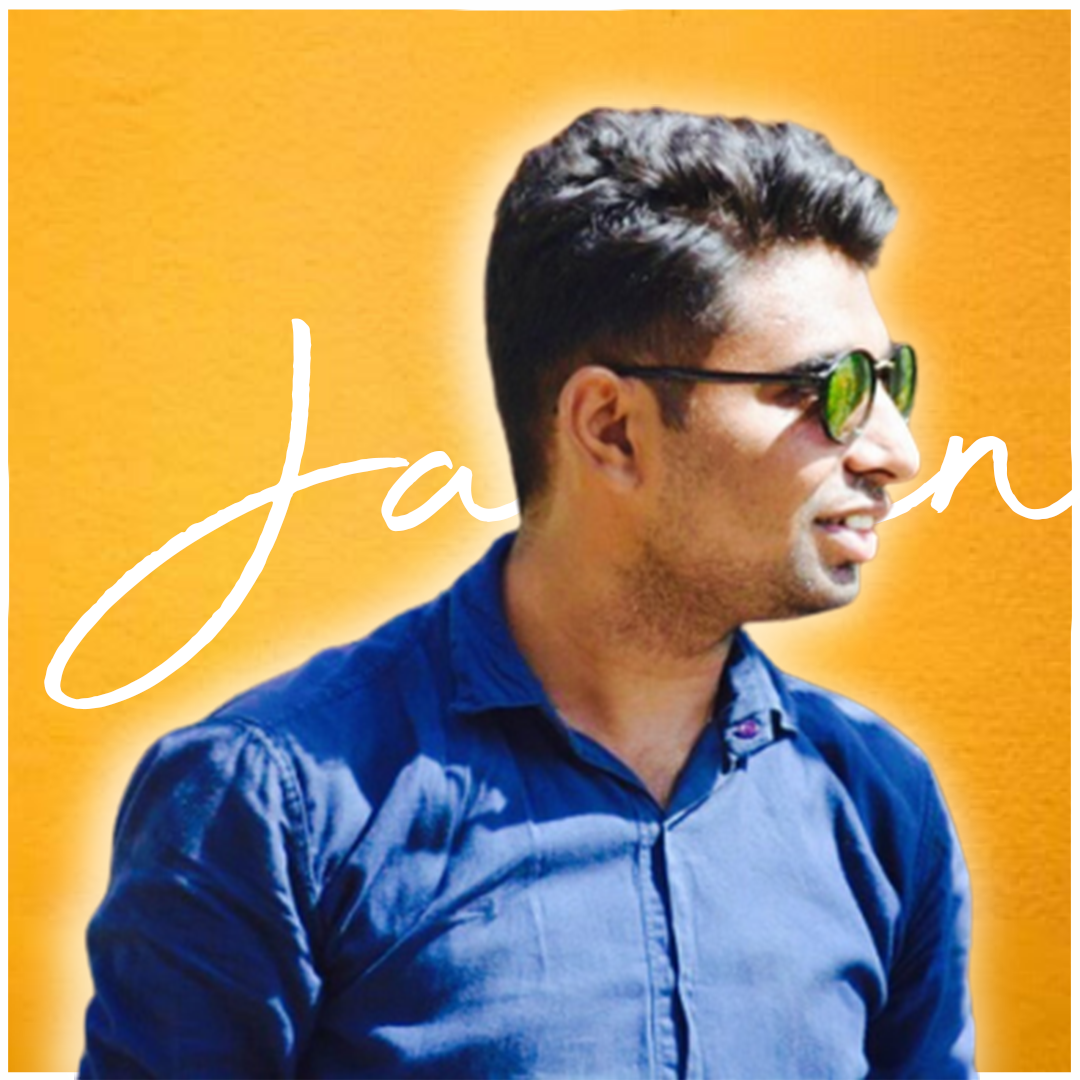

Introduction: Creating a Frequently Asked Questions (FAQ) section is a common requirement for many websites. In this article, we'll walk through the process of building a dynamic FAQ section in an ASP.NET Core MVC application. We'll utilize the Model-View-Controller (MVC) architecture pattern to separate concerns and improve maintainability.
Prerequisites: Before we begin, ensure you have the following prerequisites installed:
Step 1: Setting Up the Project: Start by creating a new ASP.NET Core MVC project in Visual Studio. Choose the appropriate project template and configure the project settings according to your requirements.
Step 2: Creating the Model: We'll begin by defining the Faq
model class. This class will represent each FAQ item and contain properties such as Number
, Question
, and Answer
.
public class Faq
{
public int Number { get; set; }
public string Question { get; set; }
public string Answer { get; set; }
}
Step 3: Implementing the Service: Next, we'll create a service class (FaqService
) responsible for providing the list of FAQ items. This separation of concerns enhances code organization and maintainability.
public static class FaqService
{
public static List<Faq> GetFaqList()
{
// Implement logic to fetch FAQ items from data source (e.g., database)
// For demonstration purposes, we'll hardcode the FAQ items
List<Faq> faqList = new List<Faq>();
// Add FAQ items to the list
// Example:
// faqList.Add(new Faq { Number = 1, Question = "Question 1", Answer = "Answer 1" });
// faqList.Add(new Faq { Number = 2, Question = "Question 2", Answer = "Answer 2" });
// ...
return faqList;
}
}
Step 4: Implementing the Controller: In the controller (HomeController
), retrieve the list of FAQ items from the service and pass them to the view.
public class HomeController : Controller
{
public IActionResult Index()
{
List<Faq> faqData = FaqService.GetFaqList();
return View(faqData);
}
}
Step 5: Creating the View: Finally, create the view (Index.cshtml
) to render the FAQ items dynamically using Razor syntax.
@model List<Faq>
<div class="accordion" id="accordionPanelsStayOpenExample">
@foreach (var faq in Model)
{
<div class="accordion-item">
<h2 class="accordion-header" id="panelsStayOpen-heading@(faq.Number)">
<button class="accordion-button" type="button" data-bs-toggle="collapse" data-bs-target="#panelsStayOpen-collapse@(faq.Number)" aria-expanded="true" aria-controls="panelsStayOpen-collapse@(faq.Number)">
@faq.Question
</button>
</h2>
<div id="panelsStayOpen-collapse@(faq.Number)" class="accordion-collapse collapse show" aria-labelledby="panelsStayOpen-heading@(faq.Number)">
<div class="accordion-body">
@Html.Raw(faq.Answer)
</div>
</div>
</div>
}
</div>
Conclusion: In this article, we've learned how to create a dynamic FAQ section in ASP.NET Core MVC. By following the Model-View-Controller architecture and separating concerns, we've built a scalable and maintainable solution. You can further enhance this implementation by integrating with a database or providing an administrative interface to manage FAQ items dynamically.
Subscribe to my newsletter
Read articles from Jaimin Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
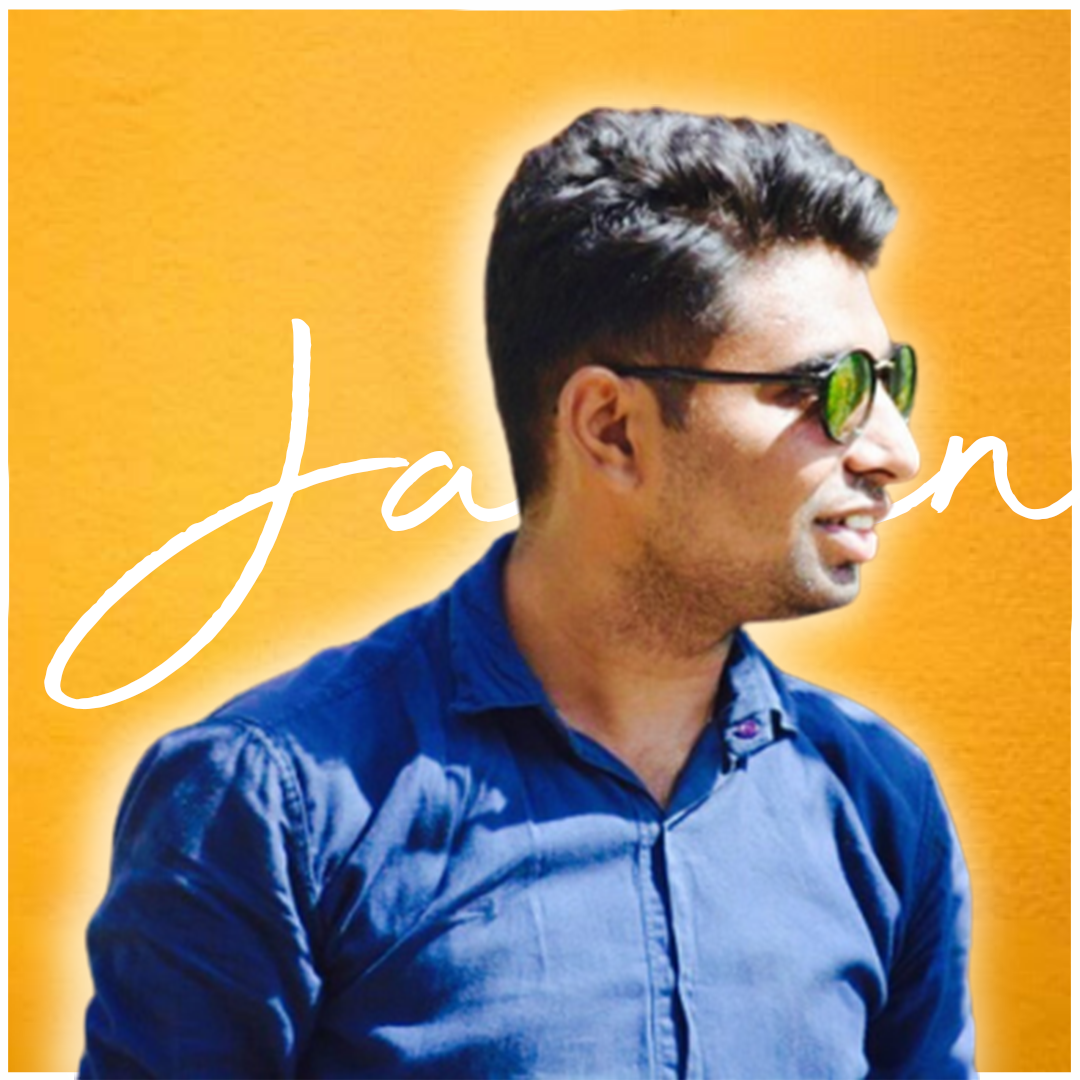
Jaimin Patel
Jaimin Patel
Hi, I'm Jaimin, a front-end developer with counting years of experience in HTML5, CSS3, JavaScript, and React. I am passionate about creating user-friendly web experiences that look great and drive engagement. In my free time, I enjoy exploring new hiking trails and checking out local coffee shops. Let's work together to bring your web project to life!