Exploring Python's Concise Syntax
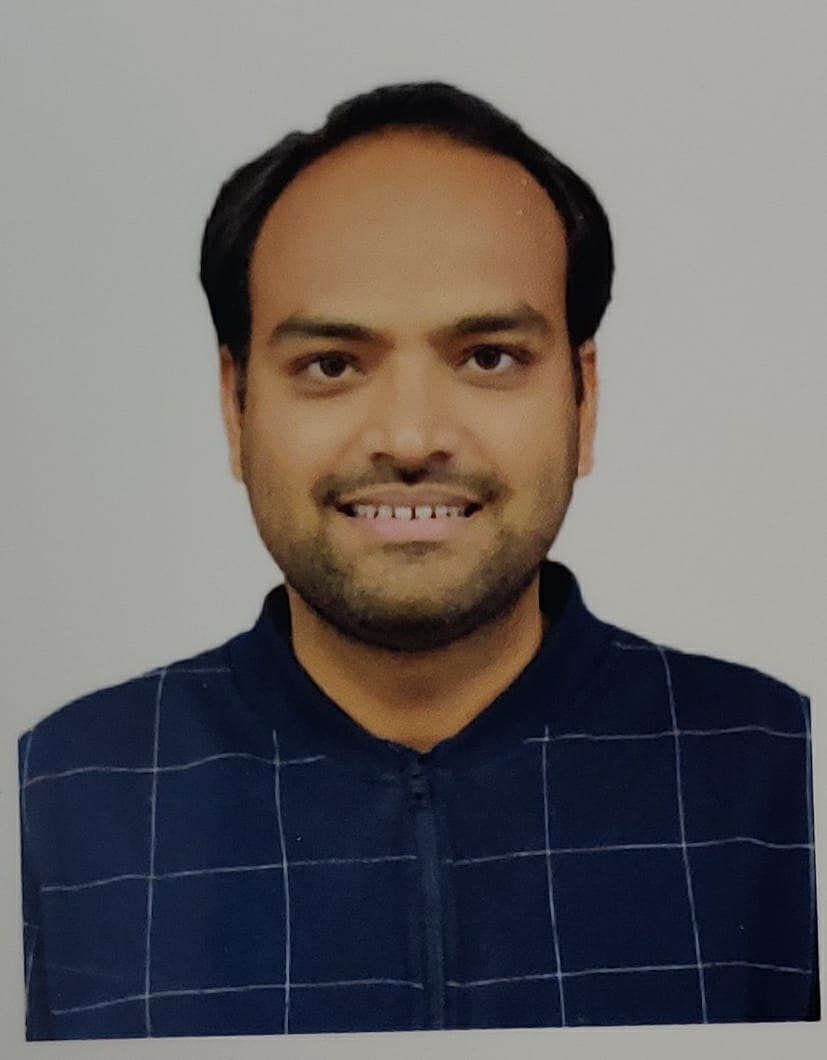
1 min read
Let's see some examples of Python's concise syntax.
List Comprehension
List comprehension provides a compact way to create lists based on existing lists. Let's say we want to generate a list containing even numbers till 10.
With For Loop:
even_numbers = []
for num in range(0, 12, 2):
even_numbers.append(num)
print(even_numbers)
With List Comprehension:
even_numbers = [num for num in range(0, 12, 2)]
print(even_numbers)
Dictionary Comprehension
Similar to list comprehension, Python provides a concise way for creating dictionaries. Suppose we want to create a dictionary containing squares of numbers up to 5.
With For Loop:
squares = {}
for num in range(1, 6):
squares[num] = num ** 2
With Dictionary Comprehension:
squares = {num: num ** 2 for num in range(1, 6)}
Now, Let's say now you want to print even numbers up to 10, it can be done in following ways.
Print using a for
loop with range
:
for num in range(0, 12, 2):
print(num, end=' ')
'Unpack' the range with the *
operator:
print(*range(0, 12, 2), end=' ')
Conclusion
While various syntax options are available to achieve the same task in Python, in the end, writing clear and maintainable code should be the goal.
0
Subscribe to my newsletter
Read articles from Alok Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
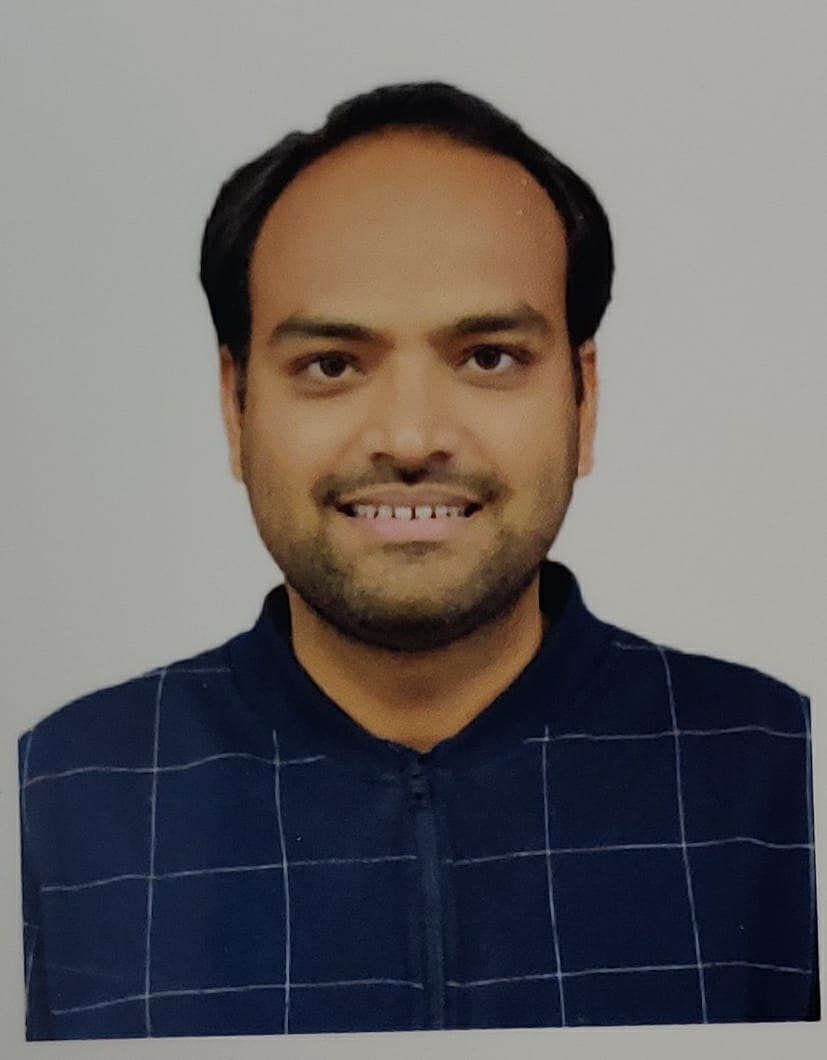