How to create a multi-page website in React ?
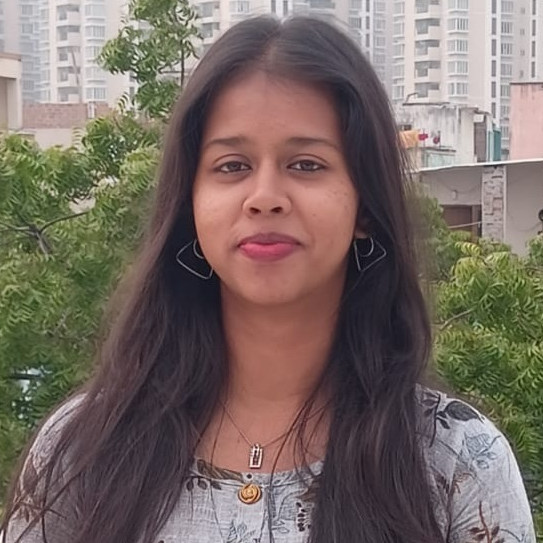
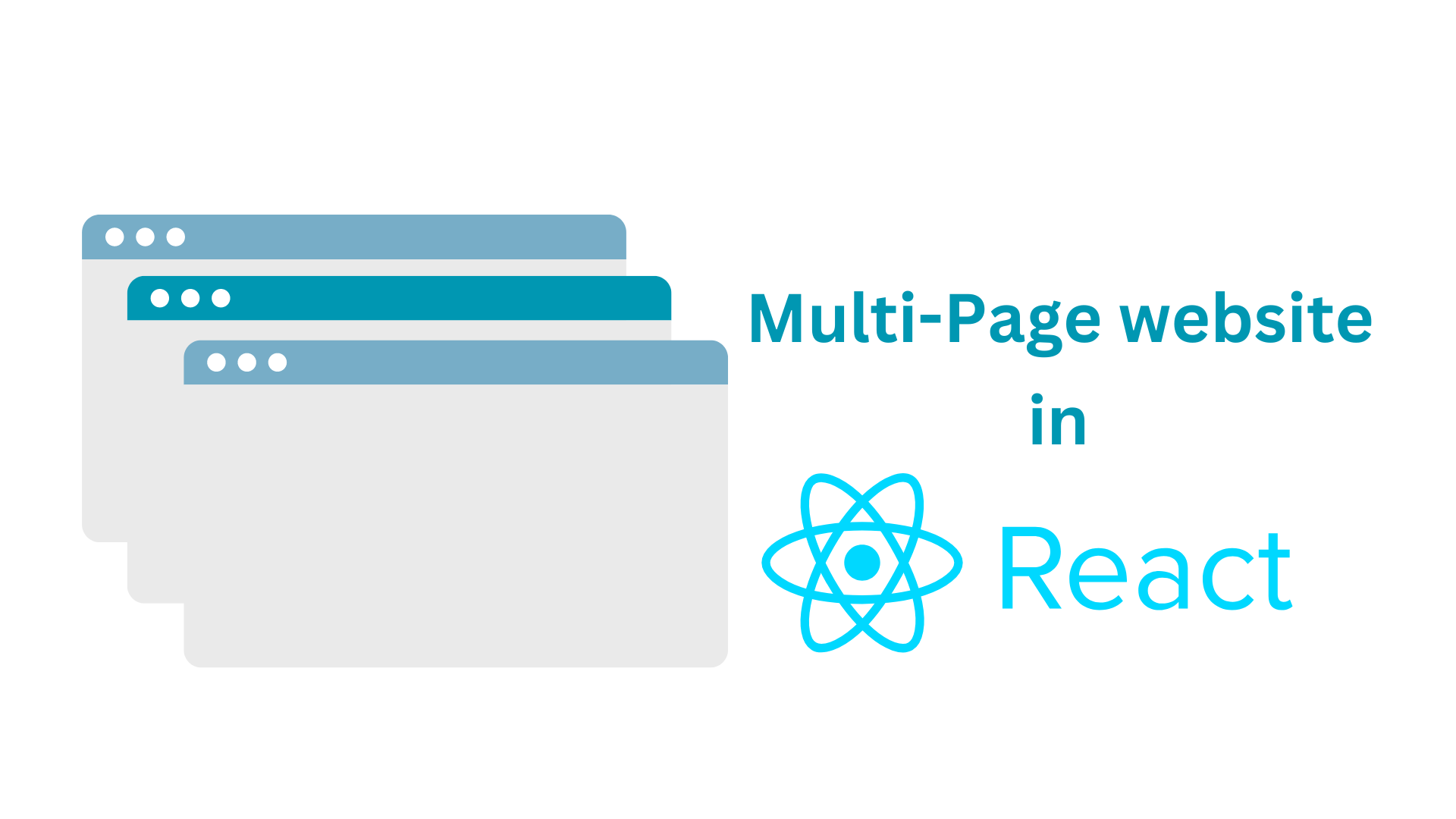
Introduction
Creating a multi-page website in React JS includes creating pages by reusing the components and rendering them on defined paths using routing
Multi-page website in React JS can be achieved by using a React dependency called react-router-dom
. It helps developers create dynamic routing for React applications. It also allows them to define different paths for their applications.
In this blog, we will see how to create a simple multi-page website using React JS
Create React App
The initial step for creating a multi-page website is to create a React app. Open the VS-code
editor and paste the command below in the terminal
.
npx create-react-app multi-page-react-app
Here, multi-page-react-app
is the name of the app.
Create a Navbar component
Create a
component
folder insidesrc
Create a
Navbar
component inside component folderPaste the below code to create a navigation bar for home, about and blog pages
import React from 'react';
function Navbar() {
return (
<div className='navigation-menu'>
<ol>
<li>Blogs</li>
<li>About</li>
<li>Home</li>
</ol>
</div>
)
}
export default Navbar
Add Styling to Navbar
/* navbar.css */
ol{
list-style-type: none;
background-color: #282828;
color: #fff;
overflow: hidden;
margin: 0;
border: 0;
}
li{
float: right;
display: inline-block;
padding: 25px;
}
.navigation-menu{
width: 100%;
}
Create Pages
We are going to create 3 pages, such as home, about and blog. Follow the steps below:
Create
pages
folder insrc
folderCreate 3 JavaScript files for 3 pages
Write the below code for the respective pages
// Home.js import React from 'react' import Navbar from '../components/Navbar'; function Home() { return ( <div> <Navbar /> <h1> This is Home page </h1> </div> ) } export default Home
//About.js import React from 'react' import Navbar from '../components/Navbar' function About() { return ( <div> <Navbar /> <h1> This is a multi-page React App </h1> </div> ) } export default About
// Blog.js iimport React from 'react' import Navbar from '../components/Navbar' function Blog() { return ( <div> <Navbar /> <h1> Write your blogs here! </h1> </div> ) } export default Blog
Install react-router-dom dependency
Copy and paste the command in the terminal to install react-router-dom dependency
cd multi-page-react-app
npm install react-router-dom
Configure index.js
Import createBrowserRouter
and RouterProvider
from react-router-dom
to create a router logic and history stack
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import {
createBrowserRouter,
RouterProvider,
} from "react-router-dom";
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
Create router
object for paths such as /
, /home
, /about
and /blog
where element
defines JavaScript file for respective pages
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import {
createBrowserRouter,
RouterProvider,
} from "react-router-dom";
import Home from './pages/Home';
import About from './pages/About';
import Blog from './pages/Blog';
const router = createBrowserRouter([
{
path: "",
element: <App />,
},
{
path: "/Home",
element: <Home />,
},
{
path: "/About",
element: <About />,
},
{
path: "/Blog",
element: <Blog />,
},
]);
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
Add RouterProvider
inside the render function
// Final code for index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import {
createBrowserRouter,
RouterProvider,
} from "react-router-dom";
import Home from './pages/Home';
import About from './pages/About';
import Blog from './pages/Blog';
const router = createBrowserRouter([
{
path: "",
element: <App />,
},
{
path: "/Home",
element: <Home />,
},
{
path: "/About",
element: <About />,
},
{
path: "/Blog",
element: <Blog />,
},
]);
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<RouterProvider router={router} />
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
Update the Navbar
Update the links for the paths in Navbar
component using the Link
tag from react-router-dom
// Updated code for Navbar.js
import React from 'react';
import './Navbar.css';
import {Link} from "react-router-dom";
function Navbar() {
return (
<div className='navigation-menu'>
<ol>
<li><Link to={"/blog"}>Blogs</Link></li>
<li><Link to={"/about"}>About</Link></li>
<li><Link to={"/home"}>Home</Link></li>
</ol>
</div>
)
}
export default Navbar
Run the React App
To start running the react app, paste the below command in the terminal
npm start
Important Links
Subscribe to my newsletter
Read articles from Roopa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
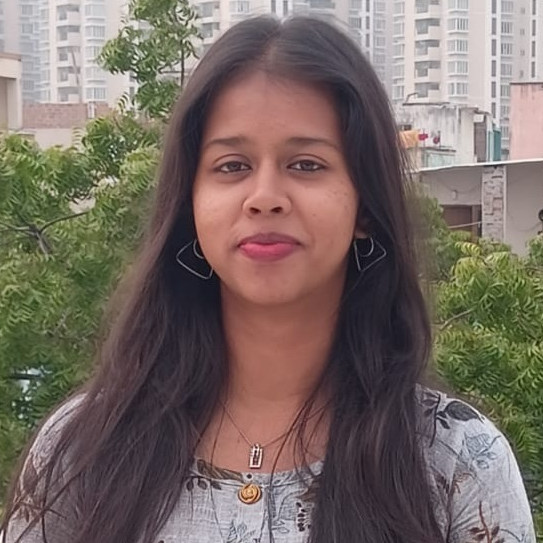
Roopa
Roopa
I'm a pre-final year computer science student at SRM with a strong academic background and technical skills. I am actively contributing to the beginner-friendly open-source repositories to showcase my skills in web development. I love to share my knowledge of technical skills and am a pro at debugging errors.