Boost Your Python Skills: 8 Sneaky List Comprehension Shortcuts Every Programmer Should Use

Table of contents
- 1. What is List Comprehension?
- 2. Why Should You Care About List Comprehension?
- 3. Basic Syntax of List Comprehension
- 4. Shortcut #1: Filtering Lists
- 5. Shortcut #2: Transforming Elements
- 6. Shortcut #3: Nested List Comprehension
- 7. Shortcut #4: Conditional List Comprehension
- 8. Shortcut #5: Using List Comprehension with Functions
- 9. Shortcut #6: Set and Dictionary Comprehension
- 10. Shortcut #7: Using List Comprehension for String Manipulation
- 11. Shortcut #8: List Comprehension vs. Traditional Loops
- 12. Conclusion
- 13. FAQs
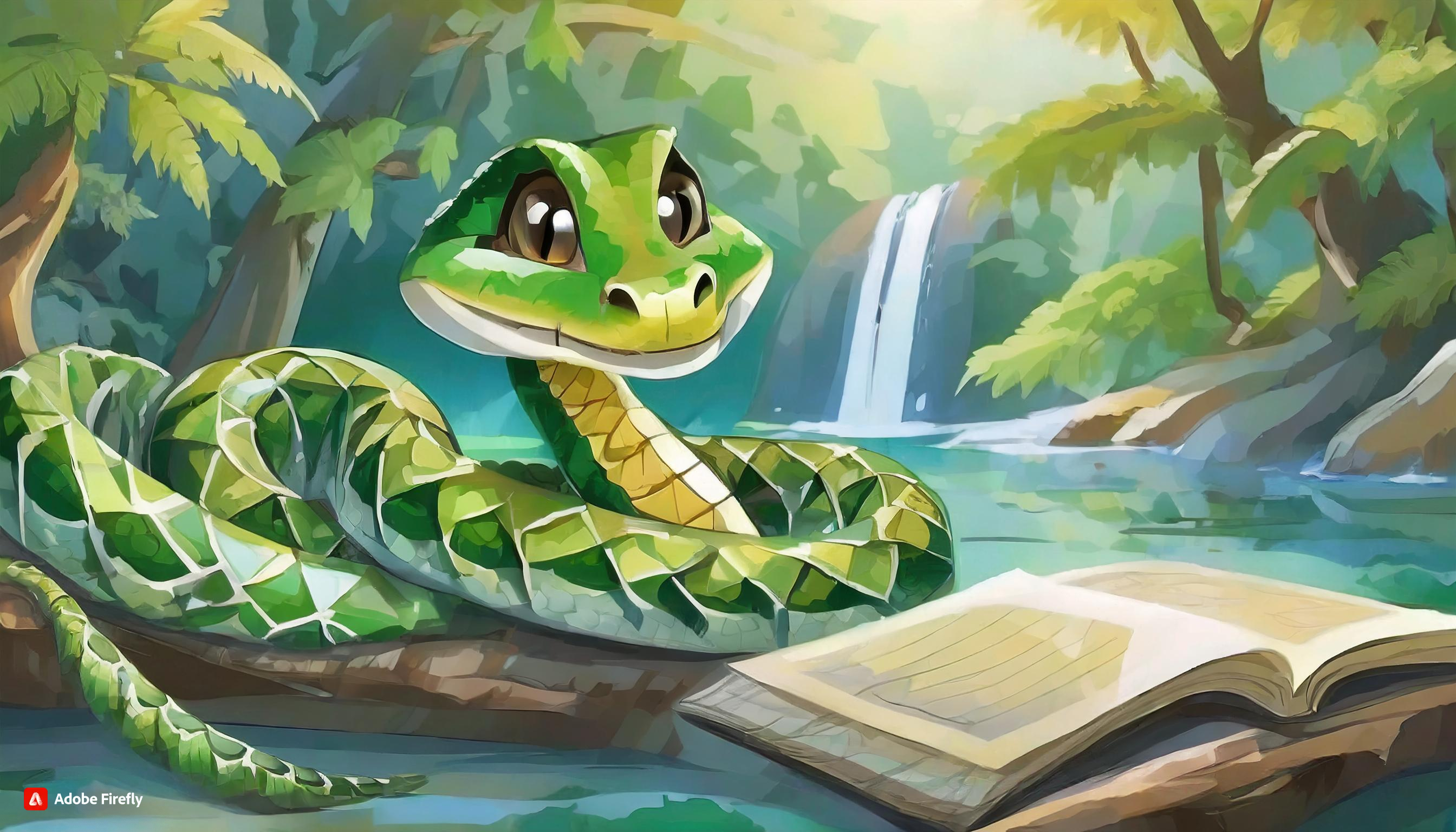
Hey there, fellow Python enthusiast! Are you ready to take your coding game to the next level? Buckle up because we're about to dive into the wonderful world of list comprehension in Python. If you've been writing Python code for a while, you've probably heard about list comprehension. But do you really understand its power? Stick around as we unravel some sneaky shortcuts that will turbocharge your Python skills!
1. What is List Comprehension?
List comprehension is like a magic wand for Python programmers. It's a concise and elegant way to create lists in Python by applying transformations to existing lists. Instead of writing multiple lines of code for simple tasks, you can accomplish them in just one line with list comprehension.
2. Why Should You Care About List Comprehension?
Imagine you're building a complex data processing pipeline. Efficiency matters, right? List comprehension is your secret weapon to write efficient, readable, and Pythonic code. It's not just about saving lines of code; it's about writing code that's easy to understand and maintain.
3. Basic Syntax of List Comprehension
To create a list comprehension, you typically follow this syntax:
new_list = [expression for item in iterable if condition]
4. Shortcut #1: Filtering Lists
Let's say you have a list of numbers, and you want to filter out the even ones. With list comprehension, you can do it in a snap:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [num for num in numbers if num % 2 == 0]
5. Shortcut #2: Transforming Elements
Want to square each number in a list? No problem! List comprehension makes it super easy:
numbers = [1, 2, 3, 4, 5]
squared_numbers = [num ** 2 for num in numbers]
6. Shortcut #3: Nested List Comprehension
Sometimes, you need to work with nested lists. List comprehension can handle that too:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened_matrix = [num for row in matrix for num in row]
7. Shortcut #4: Conditional List Comprehension
Need to handle different conditions? List comprehension lets you do that effortlessly:
numbers = [1, 2, 3, 4, 5, 6]
result = ["even" if num % 2 == 0 else "odd" for num in numbers]
8. Shortcut #5: Using List Comprehension with Functions
You can even use functions within list comprehension to make your code more modular:
def square(x):
return x ** 2
numbers = [1, 2, 3, 4, 5]
squared_numbers = [square(num) for num in numbers]
9. Shortcut #6: Set and Dictionary Comprehension
List comprehension isn't just for lists! You can also use it to create sets and dictionaries:
unique_numbers = {num for num in numbers}
number_to_square = {num: num ** 2 for num in numbers}
10. Shortcut #7: Using List Comprehension for String Manipulation
String manipulation becomes a breeze with list comprehension:
words = ["hello", "world", "python"]
capitalized_words = [word.upper() for word in words]
11. Shortcut #8: List Comprehension vs. Traditional Loops
List comprehension isn't always the best choice, especially for complex logic. Knowing when to use it and when to stick with traditional loops is key to writing clean and efficient code.
12. Conclusion
Congratulations! You've unlocked the power of list comprehension. By mastering these sneaky shortcuts, you're well on your way to becoming a Python ninja. So go ahead, experiment, and unleash the full potential of Python!
13. FAQs
Q1: What makes list comprehension so powerful?
A: List comprehension simplifies and streamlines the process of creating lists in Python, making code more efficient and readable.
Q2: Can list comprehension handle nested lists?
A: Absolutely! List comprehension can handle nested lists with ease, allowing you to flatten them or perform operations on inner lists.
Q3: Is list comprehension faster than traditional loops?
A: In most cases, yes. List comprehension often performs faster than traditional loops due to its optimized implementation in Python.
Q4: Are there any limitations to list comprehension?
A: While powerful, list comprehension may become less readable for complex logic. In such cases, it's better to use traditional loops for clarity.
Q5: Can I use list comprehension with functions?
A: Certainly! List comprehension can incorporate functions, adding modularity and flexibility to your code.
Ready to level up your Python skills? Dive into the world of list comprehension and watch your code soar to new heights! Happy coding! ๐
Subscribe to my newsletter
Read articles from Omkar Thorve directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Omkar Thorve
Omkar Thorve
I build intelligent systems that solve real-world problems. With expertise in deep learning, computer vision, and NLP, I'm passionate about pushing the boundaries of AI research and innovation.