Deep Copy vs Shallow Copy in Javascript
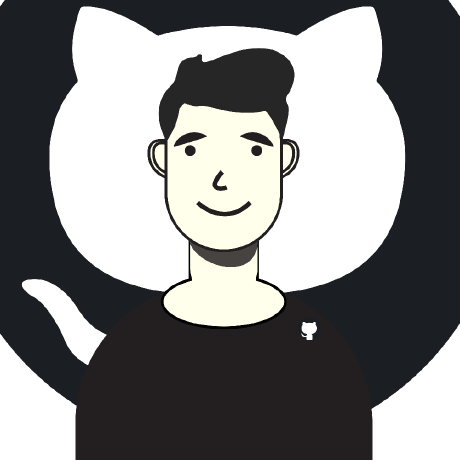
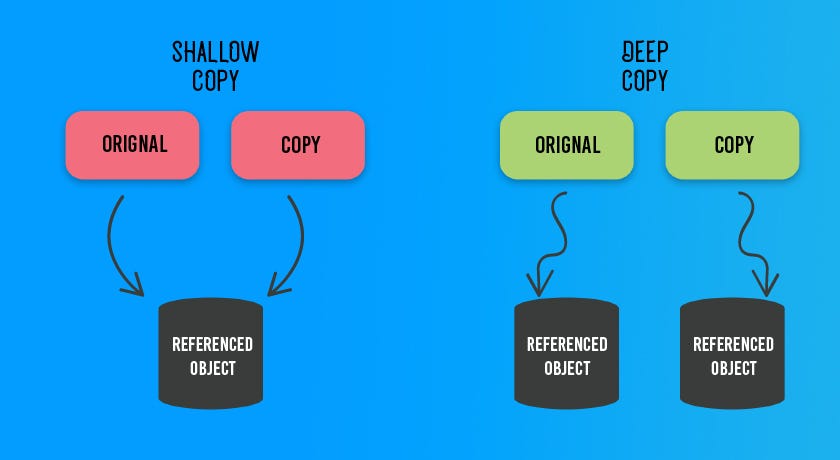
In JavaScript, copying objects or arrays might seem straightforward at first glance, but there are important distinctions between deep copy and shallow copy that developers need to understand. Let's delve into what each term means and when to use them.
Shallow Copy
A shallow copy creates a new object or array and copies the top-level structure of the original object or array. In Shallow copies instead of creating a new object copy it references it to the memory location of the original object. This means that changes made to nested objects in the copied structure will affect the original object, and vice versa.
Generally, this is faster and more efficient to save space as compared to deep copy.
Example of Shallow Copy:
const original = { a: 1, b: { c: 2 } };
const shallowCopy = { ...original };
shallowCopy.b.c = 3;
console.log(original); // { a: 1, b: { c: 3 } }
console.log(shallowCopy); // { a: 1, b: { c: 3 } }
Deep Copy
On the other hand, a deep copy creates a completely new object or array and recursively copies all nested objects and arrays. This ensures that changes made to the copied structure do not affect the original object, and vice versa.
Example of Deep Copy:
const original = { a: 1, b: { c: 2 } };
const deepCopy = JSON.parse(JSON.stringify(original));
deepCopy.b.c = 3;
console.log(original); // { a: 1, b: { c: 2 } }
console.log(deepCopy); // { a: 1, b: { c: 3 } }
When to Use Each
Shallow Copy: Use shallow copy when you only need to copy the top-level structure of an object or array, and you're certain that the nested objects or arrays won't be modified independently.
Deep Copy: Use deep copy when you need to create a fully independent copy of an object or array, including all nested objects and arrays, to avoid unintended side effects.
I hope you've grasped the difference between deep copy ๐ป and shallow copy ๐. Share your thoughts on this topic in the comments! ๐ฌ Keep coding, keep learning. ๐ Don't forget to share this post on X. ๐คณ Until next time, happy coding! ๐จโ๐ป๐ฉโ๐ป
Subscribe to my newsletter
Read articles from Vishal Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
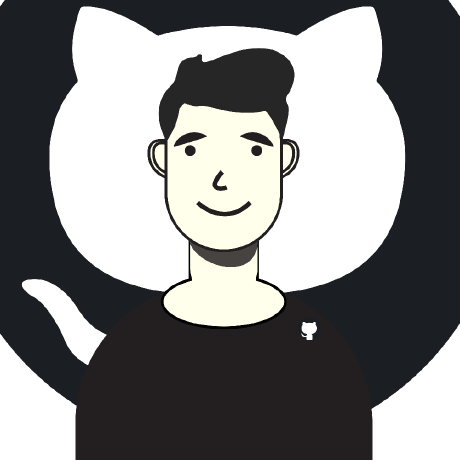
Vishal Sharma
Vishal Sharma
I am a developer from Surat who loves to write about DSA,Web Development, and Computer Science stuff.