Functional Components in React: Understanding the Simple Powerhouse
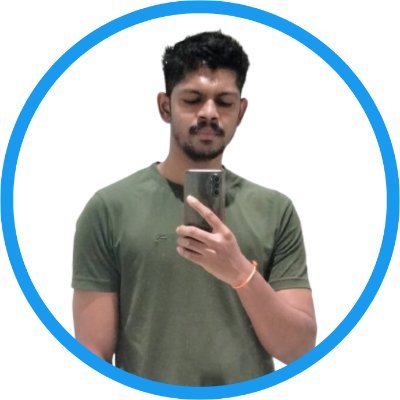
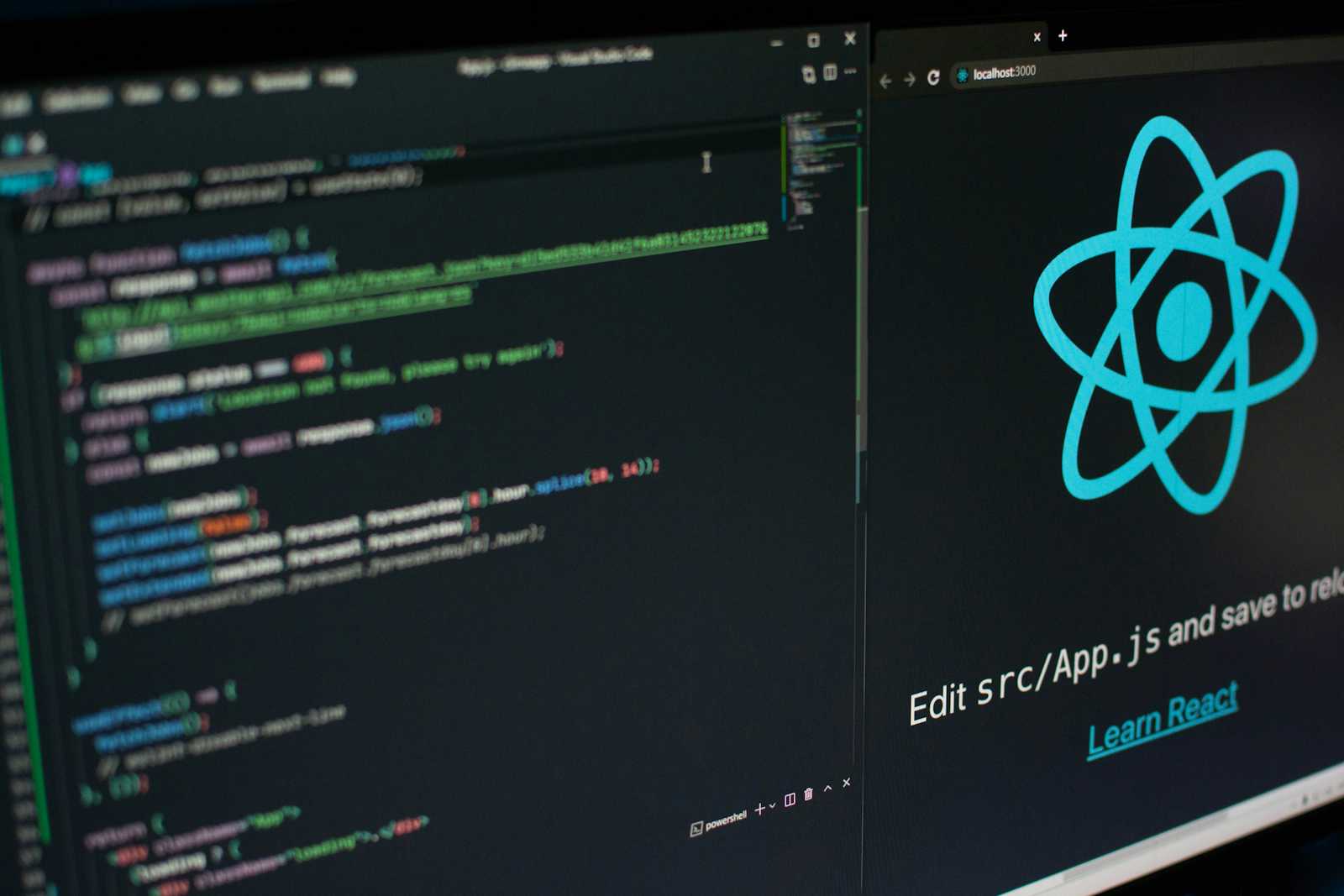
The world of React development might seem intimidating at first, with talk of components and frameworks. But fear not! At its core, building user interfaces in React is surprisingly approachable. This is especially true when it comes to functional components, the building blocks that form the foundation of reusable UI elements.
Functional Components: The Simplicity Revealed
Forget complex structures or esoteric concepts. Functional components in React are, well, just functions! They're plain JavaScript functions with a special superpower: they return JSX code, a syntax extension for describing what you want to see on the screen.
Here's a quick example to illustrate:
Key Points from the Code:
The code snippet defines two functional components:
Title
(regular function) andTitleOne
(arrow function).Both components return JSX code (
<h1></h1>
), but their core functionality is that of JavaScript functions.
Rendering Functional Components: Function Call vs. JSX Element
This section explores two ways to render functional components on your webpage: using a JSX instance and an inline function call. While both methods achieve the same result, we'll explore into the benefits of the preferred approach – JSX instances – for readability and maintainability.
JSX Element (Preferred Approach): You create an instance of the component directly in your JSX:
<Title /> <TitleOne />
Inline Function Calls (Less Common): While less common, you can also call a functional component directly as you would any other JavaScript function:
Title()
While you can directly call the component like any other function (Title()
), this approach is generally less favored for readability and separation of concerns. Here's a breakdown of the key differences:
Syntax: Function calls use parentheses
()
, while JSX elements use opening and closing tags< >
.Readability: JSX elements tend to be more readable as they explicitly state the component being rendered.
Separation of Concerns: JSX elements keep the component definition and rendering logic separate, promoting better organization.
Side Effects: Be cautious of side effects (like data fetching) within a component's render function when using inline calls. They might be executed too frequently, leading to performance issues.
Code output:
Understanding the Relationship Between Functional Components and JavaScript Functions
While rendering JSX to the UI is a crucial aspect of React, the true beauty of functional components lies in their versatility within the realm of JavaScript functions. By simply being JavaScript functions, functional components leverage the power and flexibility you're already familiar with:
Data as Arguments (Props): Just like any JavaScript function, you can pass data as arguments (props) to functional components, allowing them to adapt and display content dynamically.
Conditional Logic: You can use conditional logic within the function to control what JSX is returned. This allows components to behave differently based on various conditions.
Flexibility: Functional components inherit the power and flexibility of JavaScript functions. You can leverage familiar concepts like loops and expressions to create complex UI logic.
The Power of Simplicity
Remember, React's core philosophy is efficient UI development. Functional components, being simple JavaScript functions, perfectly embody this approach. They offer a straightforward way to create reusable UI pieces, keeping your code clean and manageable.
Ready to Build?
Functional components are a powerful tool in your React development arsenal. Start by creating small, reusable components that encapsulate specific UI logic. Gradually build more complex UIs from these building blocks. You'll be surprised at how much you can achieve with this simple yet effective concept!
Conclusion: This blog post has explored the concept of functional components in React. Functional components are the heart of React UIs. These JavaScript powerhouses, disguised as simple functions, return JSX to build reusable and maintainable UIs. As a newcomer to blogging, I welcome any feedback or suggestions you might have. Let me know if there's anything I can improve on or clarify further!
Want to Learn React from the best? (Not Paid Promotion)
Check out: https://namastedev.com/learn/namaste-react by Akshay Saini
References:
Official React Documentation: React Components: https://reactjs.org/docs/components-and-props.html by React Team
React Functional Components: In-Depth Guide:https://www.freecodecamp.org/learn/front-end-development-libraries/react/create-a-stateless-functional-component
ReactJS Functional Components:https://www.geeksforgeeks.org/reactjs-functional-components/
Subscribe to my newsletter
Read articles from Sanjit Gawade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
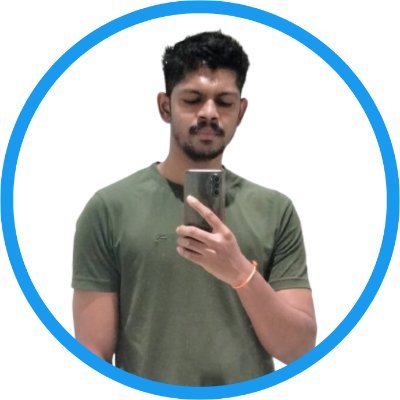
Sanjit Gawade
Sanjit Gawade
ME IT'22 GEC GOA