Method overriding and super keywords
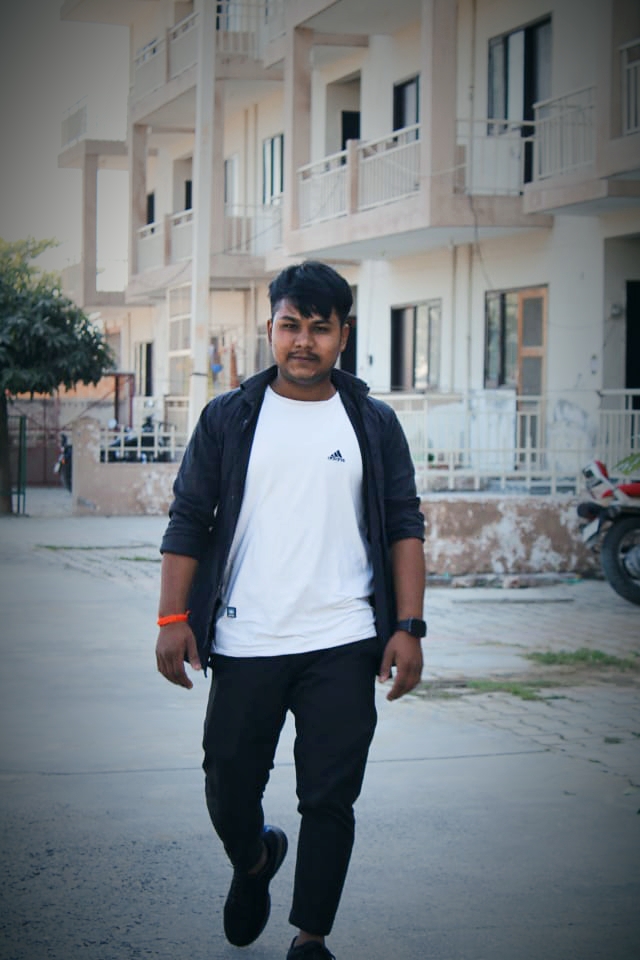
In JavaScript, method overriding can be achieved by redefining a method in a subclass that was originally defined in the superclass. Unlike languages like Java, JavaScript does not have a built-in mechanism for method overriding, but the concept can still be implemented using prototypal inheritance. Here's how you can achieve method overriding in JavaScript:
// Define a superclass
function Animal(name) {
this.name = name;
}
// Define a method in the superclass
Animal.prototype.speak = function() {
return "Animal speaks";
};
// Define a subclass
function Dog(name) {
// Call the superclass constructor
Animal.call(this, name);
}
// Set up prototypal inheritance
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
// Override the speak method in the subclass
Dog.prototype.speak = function() {
return "Woof!";
};
// Create instances of the classes
var animal = new Animal("Generic Animal");
var dog = new Dog("Buddy");
// Call the speak method on instances
console.log(animal.speak()); // Output: "Animal speaks"
console.log(dog.speak()); // Output: "Woof!"
In the above example,
We define a superclass
Animal
with a methodspeak
.We define a subclass
Dog
that inherits fromAnimal
.We override the
speak
method in theDog
subclass to make it specific to dogs.When we create instances of
Animal
andDog
and call thespeak
method on them, you'll see that the overridden method is called for instances ofDog
.
This is a basic example of achieving method overriding in JavaScript using prototypal inheritance. Alternatively, you can use ES6 classes to achieve similar behavior with a more class-oriented syntax.
supper keyword
Thesuper
keyword is used within derived classes (subclasses) to call methods or access properties from the parent class (superclass). It provides a way to access and call functions defined on the superclass within the subclass. Thesuper
keyword can be used in two ways:
Call Parent Constructor: Inside the constructor of a subclass,
super()
can be used to call the constructor of the superclass. This is necessary to initialize properties defined in the superclass.Call Parent Method: Within methods of a subclass, it
super.methodName()
can be used to call a method of the same name defined in the superclass.
Here's an example demonstrating the use of thesuper
keyword:
// Define a superclass
class Animal {
constructor(name) {
this.name = name;
}
speak() {
return "Animal speaks";
}
}
// Define a subclass
class Dog extends Animal {
constructor(name, breed) {
super(name); // Call the constructor of the superclass
this.breed = breed;
}
speak() {
return super.speak() + " Woof!"; // Call the speak method of the superclass
}
}
// Create instances of the classes
let animal = new Animal("Generic Animal");
let dog = new Dog("Buddy", "Golden Retriever");
// Call the speak method on instances
console.log(animal.speak()); // Output: "Animal speaks"
console.log(dog.speak()); // Output: "Animal speaks Woof!"
In theDog
class:
The constructor
super(name)
calls the constructor of theAnimal
superclass, initializing thename
property.The
speak
methodsuper.speak()
calls thespeak
method of theAnimal
superclass, and then the subclass appends "Woof!" to it.
This way, thesuper
keyword facilitates accessing superclass functionality from within a subclass.
Subscribe to my newsletter
Read articles from Arun kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
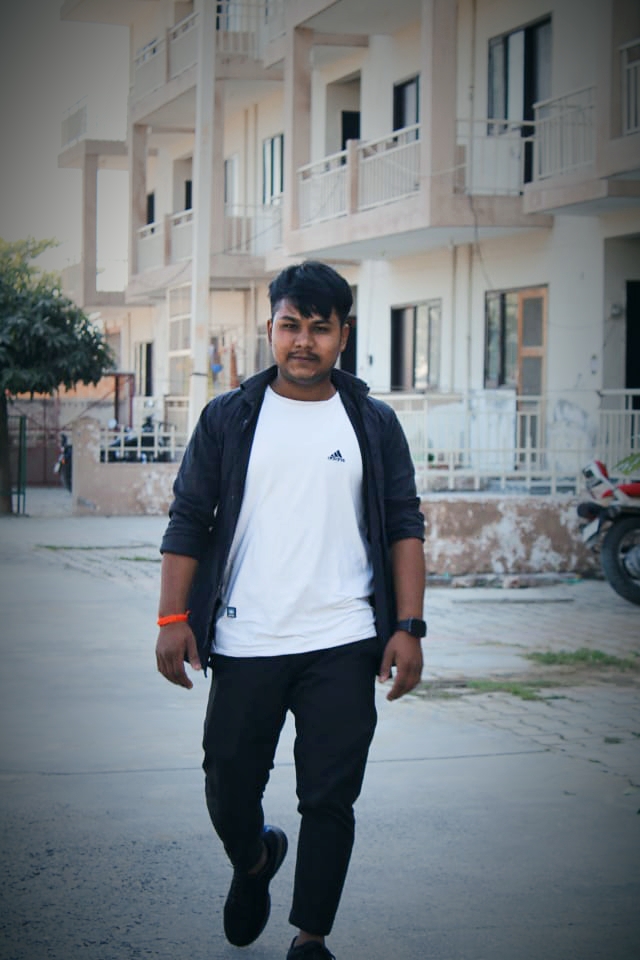