Unlocking Local Storage in Flutter with Hive
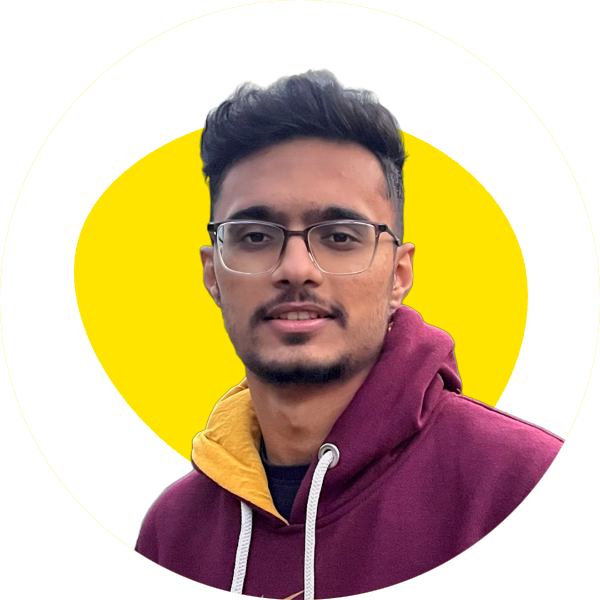
Table of contents
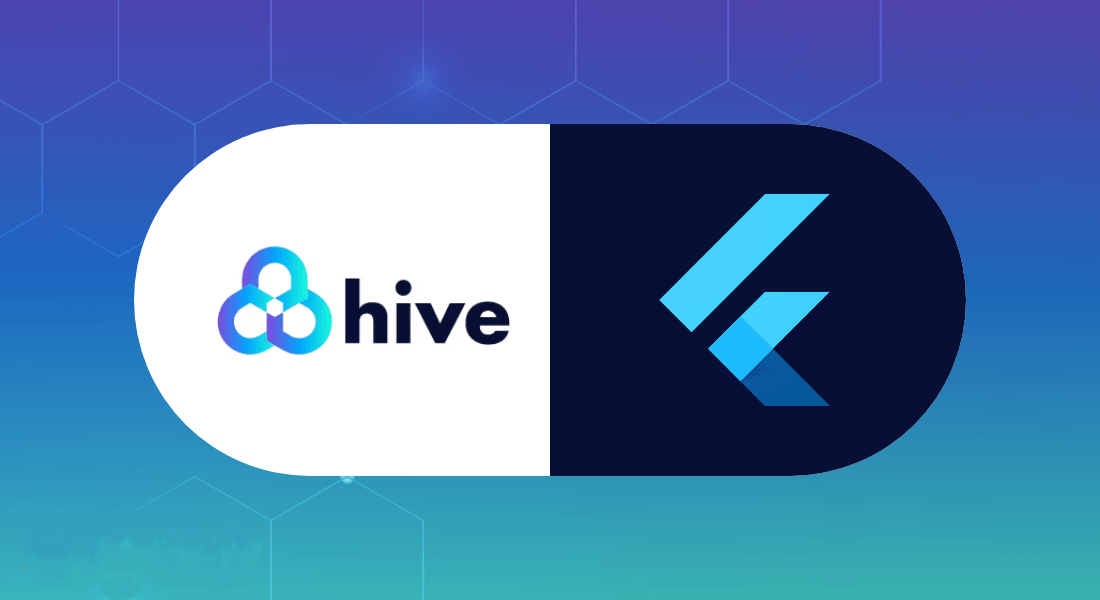
Hive is a lightweight and efficient key-value store for Flutter applications, providing a simple and flexible solution for local data storage. In this guide, we’ll dive into using Hive to store and retrieve data locally in Flutter apps, allowing you to persist user data across sessions seamlessly.
What is Hive?
Hive is a NoSQL database built specifically for Flutter and Dart. It’s designed to be fast, lightweight, and easy to use, making it an ideal choice for storing small to medium-sized data sets locally on a device. Hive stores data in boxes, where each box can hold multiple key-value pairs.
Step 0: Add the Dependencies
The first step in using Hive with Flutter is to add the necessary dependencies to your project. You can find the required packages on the Pub.dev website. For this tutorial, you'll need to add the following dependencies:
hive
: The main Hive packagehive_flutter
: The Flutter-specific package for Hivehive_generator
: A development dependency for generating Hive adaptersbuild_runner
: A development dependency used to generate the Hive adapter files
Once you've added these dependencies to your project's pubspec.yaml
file, run flutter pub get
to install them.
dependencies:
flutter:
sdk: flutter
hive: ^2.2.3
hive_flutter: ^1.1.0
hive_generator: ^2.0.1
build_runner: ^2.4.7
Step 1: Initializing Hive
Before you can use Hive, you need to initialize it in your Flutter app. This typically involves initializing Hive with the appropriate path for storing data on the device. Here’s how you can initialize Hive in your main.dart
file:
import 'package:flutter/material.dart';
import 'package:hive/hive.dart';
import 'package:path_provider/path_provider.dart' as path_provider;
void main() async {
WidgetsFlutterBinding.ensureInitialized();
final appDocumentDirectory =
await path_provider.getApplicationDocumentsDirectory();
Hive.init(appDocumentDirectory.path);
runApp(MyApp());
}
Step 2: Create a Class
Next, you'll need to create a class that you want to store in the Hive database. For this example, let's create a Person
class with two properties: name
and age
.
import 'package:hive/hive.dart';
part 'person.g.dart';
@HiveType(typeId: 0)
class Person {
@HiveField(0)
final String name;
@HiveField(1)
final int age;
Person({required this.name, required this.age});
}
In this code, we define the Person
class and annotate it with @HiveType(typeId: 0)
. This tells Hive that this class is a Hive type, and typeId
is used to uniquely identify it. We also annotate the class properties with @HiveField(0)
and @HiveField(1)
to specify the index of each field in the Hive database.
Step 3: Auto-generate the Adapter File
Hive requires an adapter for each class you want to store in the database. You can auto-generate this adapter file using the hive_generator
package and the build_runner
tool. In your terminal, run the following command:
flutter pub run build_runner build
This will generate a person.g.dart
file in the same directory as your Person
class. This file contains the necessary adapter code for Hive to work with your Person
class.
Step 4: Initialize Hive in Flutter
Before you can use Hive, you need to initialize it in your Flutter app. Add the following code to your main()
function, just before calling runApp()
:
Future main() async {
WidgetsFlutterBinding.ensureInitialized();
await Hive.initFlutter();
runApp(MyApp());
}
This ensures that Hive is properly initialized and ready to use in your Flutter app.
Step 5: Register the Adapter
After initializing Hive, you need to register the adapter for your Person
class. Add the following code after the Hive.initFlutter()
call:
Hive.registerAdapter(PersonAdapter());
This registers the adapter generated in the previous step, allowing Hive to work with your Person
class.
Step 6: Open a Box
Hive stores data in "boxes", which are similar to key-value stores. To open a box for your Person
class, use the following code:
final box = await Hive.openBox('personBox');
This opens a box named "personBox" that will store Person
objects. You can now use this box to interact with the data.
Step 7: Put Data
To add a new Person
object to the box, use the put()
method:
final person = Person(name: 'John Doe', age: 30);
await box.put('key1', person);
In this example, we create a new Person
object and store it in the box with the key "key1".
Step 8: Get Data
To retrieve a Person
object from the box, use the get()
method:
final retrievedPerson = box.get('key1');
print(retrievedPerson?.name); // Output: John Doe
print(retrievedPerson?.age); // Output: 30
Step 9: Delete Data
To delete a specific Person
object from the box, use the deleteAt()
method:
await box.deleteAt(0);
This will delete the person object at index 0 in the box.
Step 10: Clear the Box
To delete all the data in the box, use the clear()
method:
await box.clear();
This will remove all the Person
objects from the box.
Conclusion
By following these steps, you can now use Hive to store and retrieve data locally in your Flutter application. Hive provides a simple and efficient way to manage your app's data, making it a great choice for many Flutter projects.
Happy Coding!! 😁💗
Subscribe to my newsletter
Read articles from Nitin Chandra Sahu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
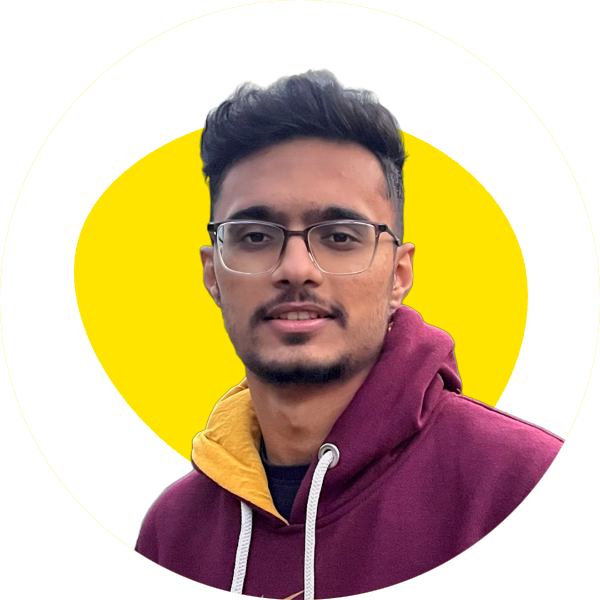