Stop Using .lowercased() to Compare Strings in Swift
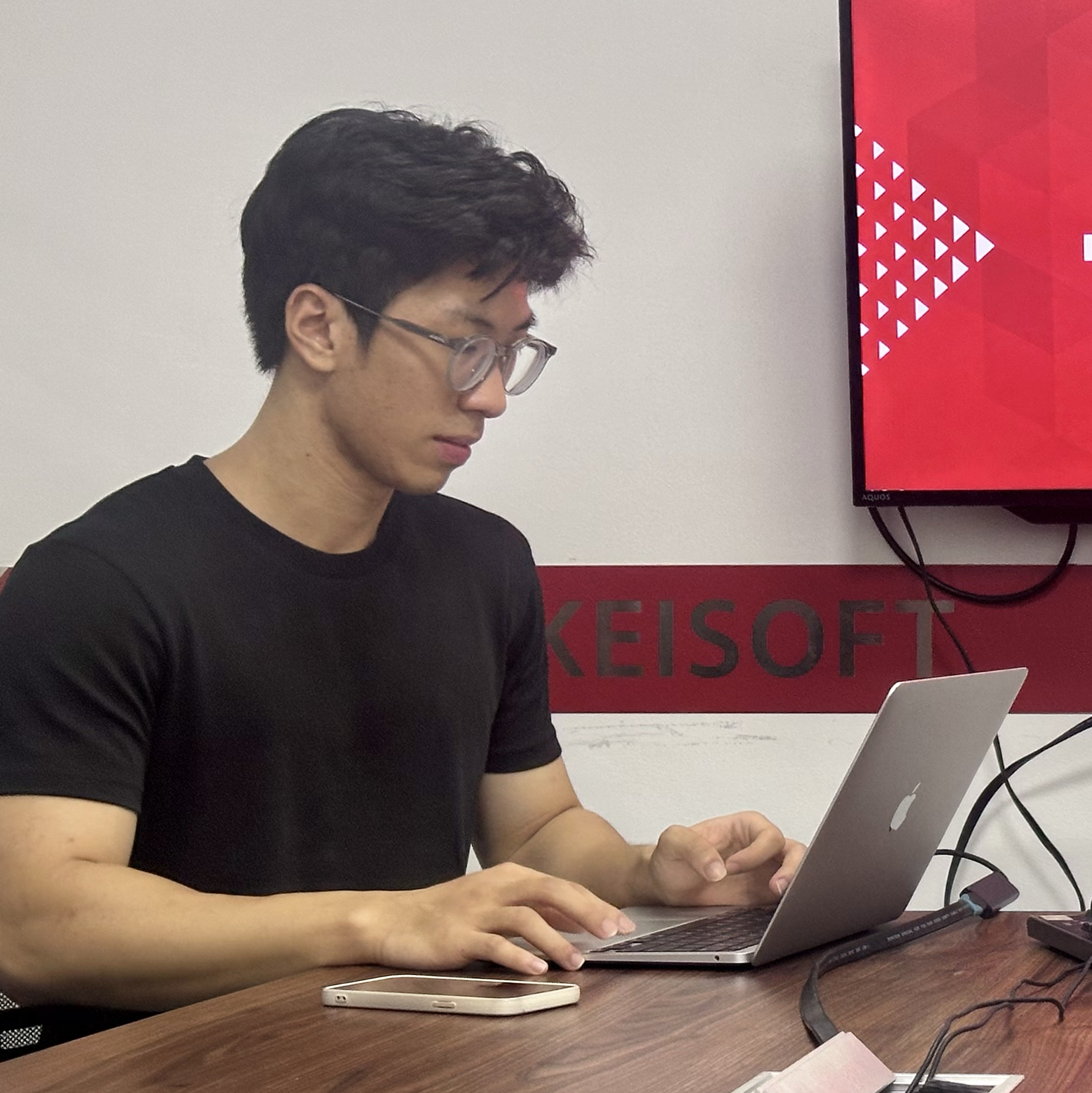
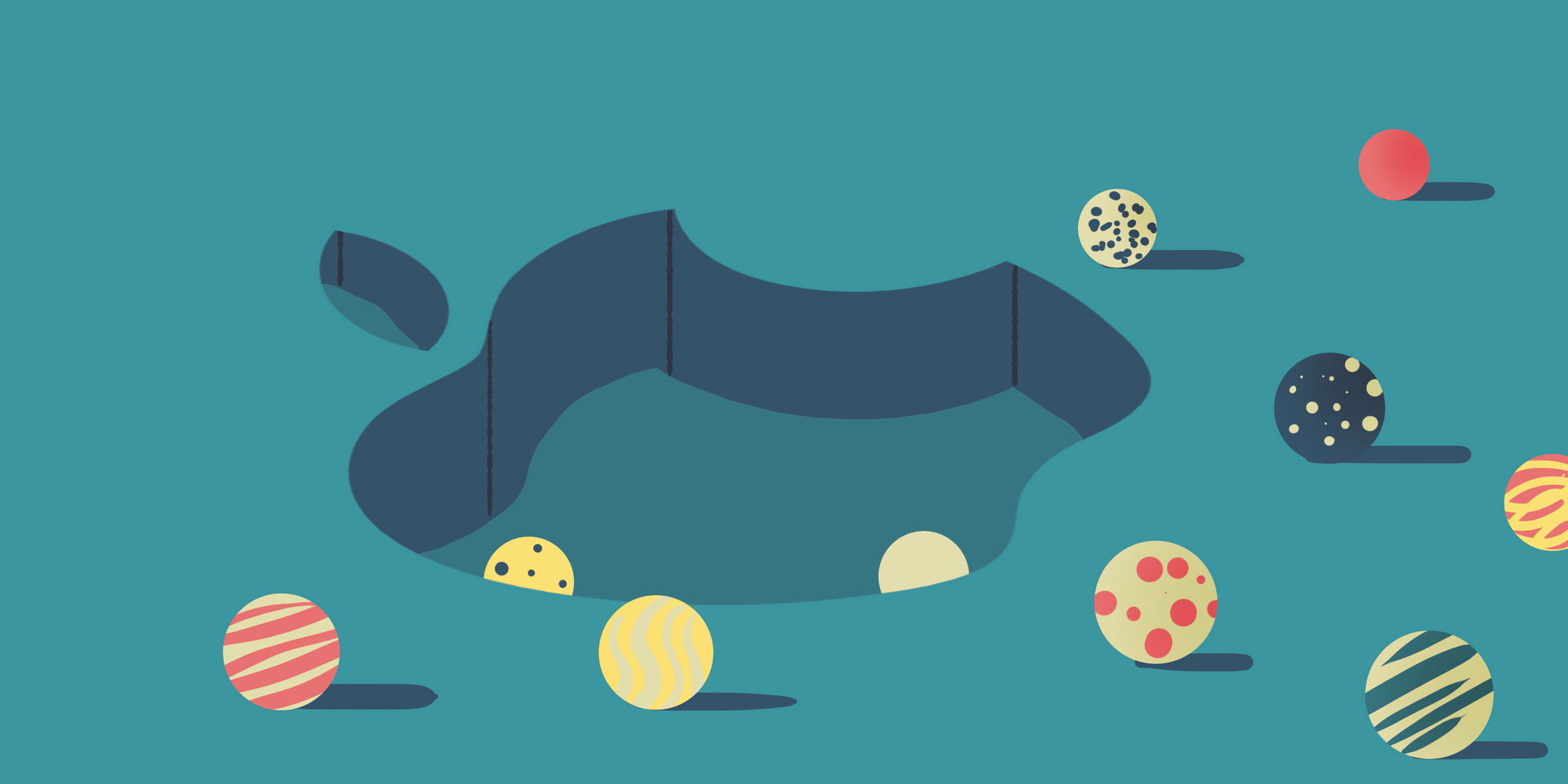
When developing in Swift, a common task you may come across is comparing strings. However, did you know that using the .lowercased()
method might not be the best way to do it?
Let's take a look at an example where we compare two strings using .lowercased()
:
import Foundation
let searchQuery = "cafe"
let databaseValue = "Café"
if searchQuery.lowercased() == databaseValue.lowercased() {
//...
}
At first glance, this seems like a reasonable approach. After all, the .lowercased()
method is simple and easy to use. However, it has a couple of significant drawbacks.
Firstly, the .lowercased()
method creates a new copy of each String every time it's called. This could potentially harm performance, especially if you're dealing with large strings or performing the operation numerous times.
Secondly, using .lowercased()
only provides a case-insensitive search. It doesn't account for diacritics, such as accents. For instance, it wouldn't match "cafe" with "Café".
So, what's the solution? Swift provides the compare()
method, allowing us to specify comparison options. This method is more flexible and can handle diacritics and case sensitivity.
Here's how you can use it:
import Foundation
let searchQuery = "cafe"
let databaseValue = "Café"
let comparisonResult = searchQuery.compare(
databaseValue,
options: [.caseInsensitive, .diacriticInsensitive]
)
if comparisonResult == .orderedSame {
//...
}
In this example, we're using the .caseInsensitive
and .diacriticInsensitive
options to compare the strings. This means the comparison will ignore both case and diacritics, making it a more robust solution for string comparison in Swift.
In conclusion, while .lowercased()
may seem like an easy solution for string comparison, it's worth considering the compare()
method for a more versatile and efficient approach.
Thanks for Reading! ✌️
If you have any questions or corrections, please leave a comment below or contact me via my LinkedIn account Pham Trung Huy.
Happy coding 🍻
Subscribe to my newsletter
Read articles from Phạm Trung Huy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
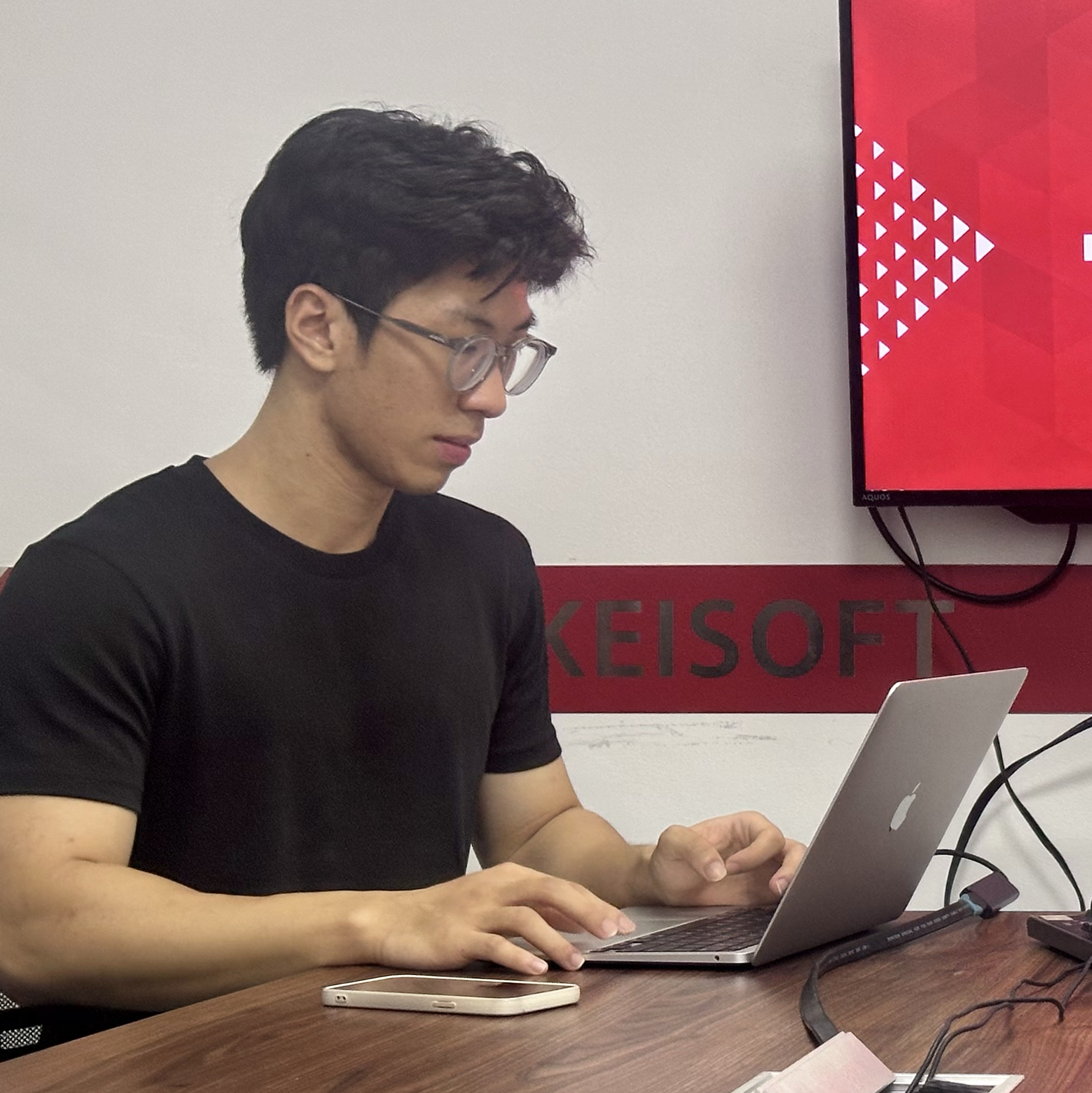
Phạm Trung Huy
Phạm Trung Huy
👋 I am a Mobile Developer based in Vietnam. My passion lies in continuously pushing the boundaries of my skills in this dynamic field.