Full stack with Vaadin as a Java developer
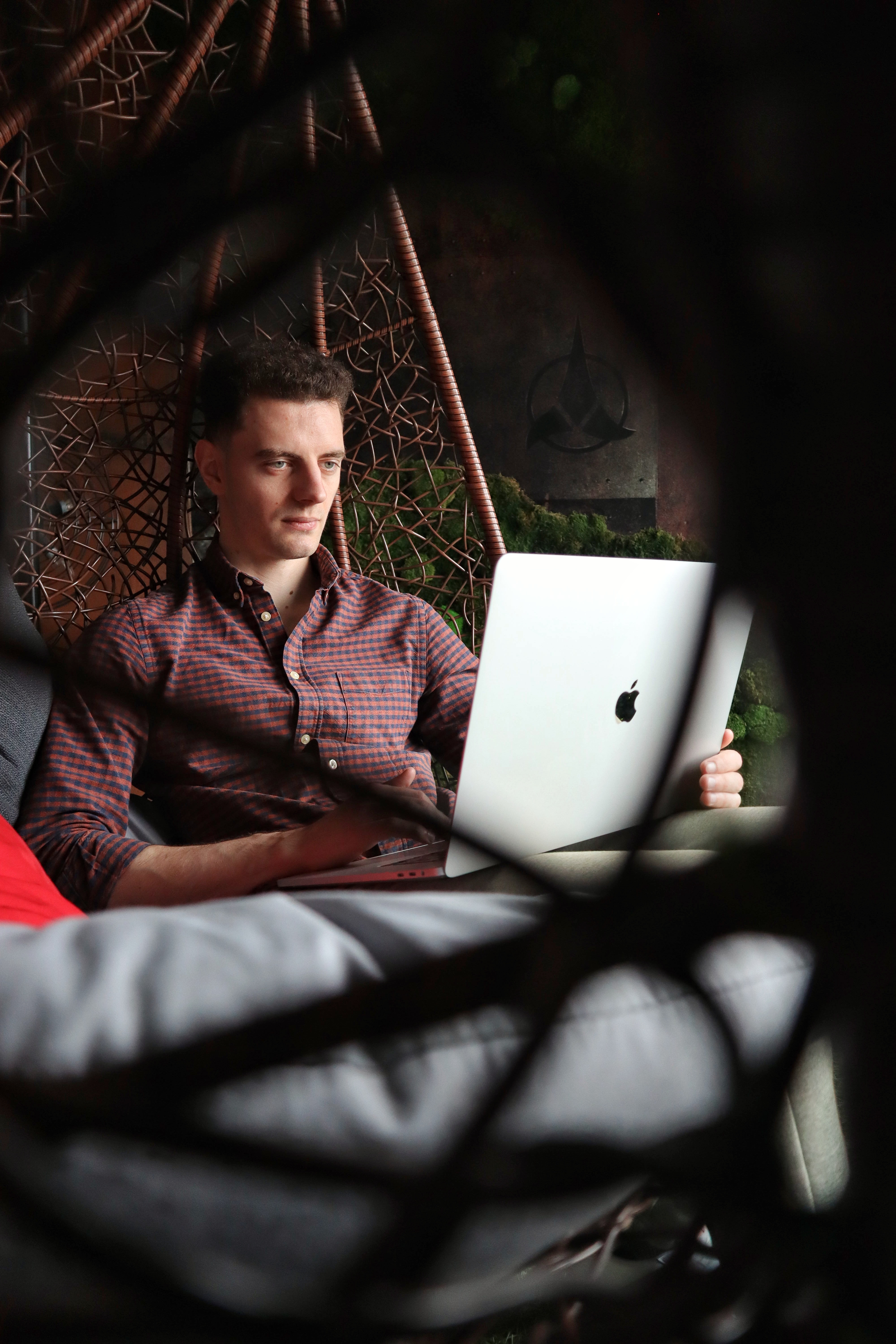
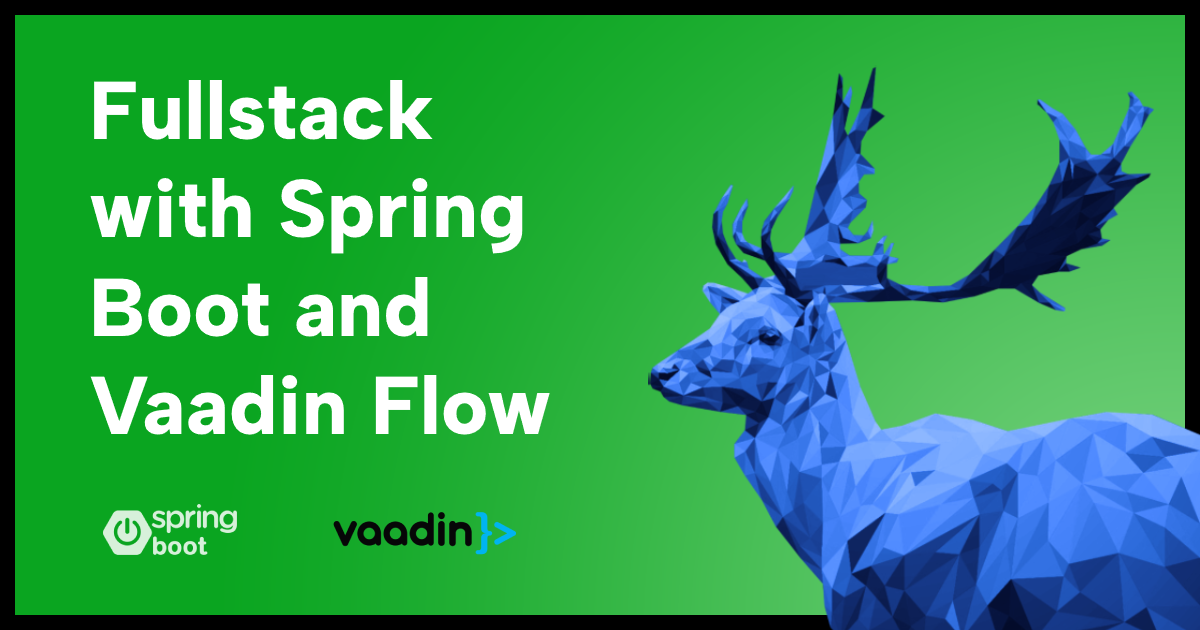
Vaadin Flow is a framework trying to offer a fast and easy solution for developers to create full stack applications using Spring Boot for the back end and, please sit down for this, their Java components for the front end. For some reason the idea of learning Javascript never seemed like something I would want to do, so the promise of being able to write an entire full stack application using only Java instantly got my attention. My instincts were telling me the Java code you would have to write to achieve that would be complicated and hideous and so too would be the resulting front end application. I can honestly say both my assumptions were proven wrong because you can indeed use Vaadin Flow to create a good looking application for your users with only Java, and writing that code feels surprisingly natural. Let’s go into details.
Testing methodology
In order to write this article I wanted to get a decent idea of what creating an entire application in Vaadin is like. Luckily for me, Vaadin offers many quality learning resources in all kinds of media formats. I decided to go with the official tutorial from their website which is also available as a two hour youtube video. These tutorials cover the creation of a fully fledged Web application, so completing them gives you a pretty good idea of what the framework is about.
Where can you learn Vaadin?
Before diving in the actual Vaadin Flow framework, I want to discuss the resources available for learning. I can honestly say I am impressed with how well structured the tutorials on the official Vaadin website are. The main tutorial goes through enough features that you feel confident to start experimenting yourself after completing it and even covers topics that are usually skipped, for example three types of testing(unit/integration/end-to-end testing) and deploying to most popular cloud providers. The tutorial is available in both video and text, which I appreciate a lot. I switched between both formats and these are some considerations before choosing which one to follow:
The video tutorial has many bits of information and explanations that have been distilled in the text version. Whenever I would go through a chapter I always felt like I understood it better from the video.
Both follow the same chapters structure which makes it really easy to alternate between, so don’t feel pressured to “choose the right one”. Just start with whichever you feel more comfortable with. Be aware of some very minor differences between code snippets. Small things like a variable called “closeButton” in the text version is named “cancelButton” in the video one, nothing to fear though.
The video was recorded in September 2021 which means there are small instances where the code no longer runs out of the box because it’s deprecated. The text version is up to date, which is to be expected since it’s easier to edit a text article than record an entire video again.
The video tutorial provides a link in the description for the project starter which is missing some important files(the entire data package and data.sql script). Make sure you download the project starter from the text version since that one has everything you need to follow along.
Personally I started with the video version and switched back and forth between the two options, depending on how I felt(I didn’t complete the tutorial in one sitting).
To get an idea of what the official tutorial teaches you to build, have a look at the screenshot below.
Working with Vaadin Flow
Ok, so what does working with Vaadin Flow actually look like? Vaadin is already available as a dependency for Spring Boot at https://start.spring.io/, which means adding it to your project is as simple as editing your Maven or Gradle dependencies list(detailed instructions). If that wasn’t enough, you can also use their own tool called Vaadin Start which offers amazing features like creating new views and entities at project creation.
Based on layouts
The way Vaadin Flow works is by using Java classes where we create the layout for each “view”. Depending on what we need, we can extend different kinds of layout classes(FormLayout, AppLayout, VerticalLayout etc.). Below you can see a simplified example of the ContactForm.java view from the tutorial.
public class ContactForm extends FormLayout {
// UI components declaration(TextFields, Buttons, etc)
public ContactForm(List<Company> companies, List<Status> statuses) {
addClassName("contact-form");
binder.bindInstanceFields(this);
company.setItems(companies);
company.setItemLabelGenerator(Company::getName);
status.setItems(statuses);
status.setItemLabelGenerator(Status::getName);
add(firstName,
lastName,
email,
company,
status,
createButtonsLayout());
}
// other methods declarations omitted for brevity
}
The essential part is the constructor where we give the component a CSS class name - “contact-form” so we can later style it, instantiate relevant items and ultimately add all the UI components to our layout, in the desired order, using the add() method. You can expect a similar structure for all your Vaadin views, so make sure you understand the detailed explanation you can find here.
Spring security
Spring Security works well with Vaadin and it makes securing and restricting access to your application based on login so much easier! The exact steps of how to do it are beyond the scope of this article, but take a look here if you want to see how you can achieve that.
PWA
Progressive web apps are very popular right now and Vaadin did not drop the ball with this either. All you have to do in order to turn you Vaadin application in a fully functional PWA is to add the intuitively named @PWA annotation to your Main java class(the class which also contains your main method). Once again the team behind Vaadin wanted to cover everything you would need in a realistic scenario so the tutorial even includes instructions on how to change your PWA icon and the offline page, check it out yourself.
Customization with CSS and events
The purpose of Vaadin is to offer a fast and reliable way to create a functional, good looking front end for your applications. We will not all build the next unicorn so for most of our needs I believe the default themes and styling are more than enough. This doesn’t mean the amazing team behind Vaadin doesn’t offer any customization support. First of all, the default theme is called Lumo and you can easily customise the aspect of it using this awesome editor www.demo.vaadin.com/lumo-editor . Yes, they even created an easy-to-use editor so you can edit the theme to fit your company look with a couple of clicks. This project is amazing! Second of all, custom CSS is also possible, if you really want to. Check this out if you are interested in doing that www.vaadin.com/docs/latest/styling .
Conclusions
Before I say anything about the actual framework, I would like to express my admiration for the team behind this project. The amount of documentation, tools and resources they made available for their product is amazing. I don’t remember the last time I saw a framework with such an amazing ecosystem made available directly by the creators. Now, that’s all nice and worthy of praise, but would I actually use the framework? Yes! A thousand times yes! I have a lot to learn and I am sure once I’ll use it for real world projects I’ll encounter shortcomings and pains(as it’s expected from anything) but currently I can say that Vaadin Flow will be my go to full stack solution when I’ll want to build an app fast and have a clean and functional front end for it. I hope this article sparked your curiosity too and if you do try Vaadin Flow for yourself I hope you’ll have the best experience while using it!
Subscribe to my newsletter
Read articles from Alexandru Tudor directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
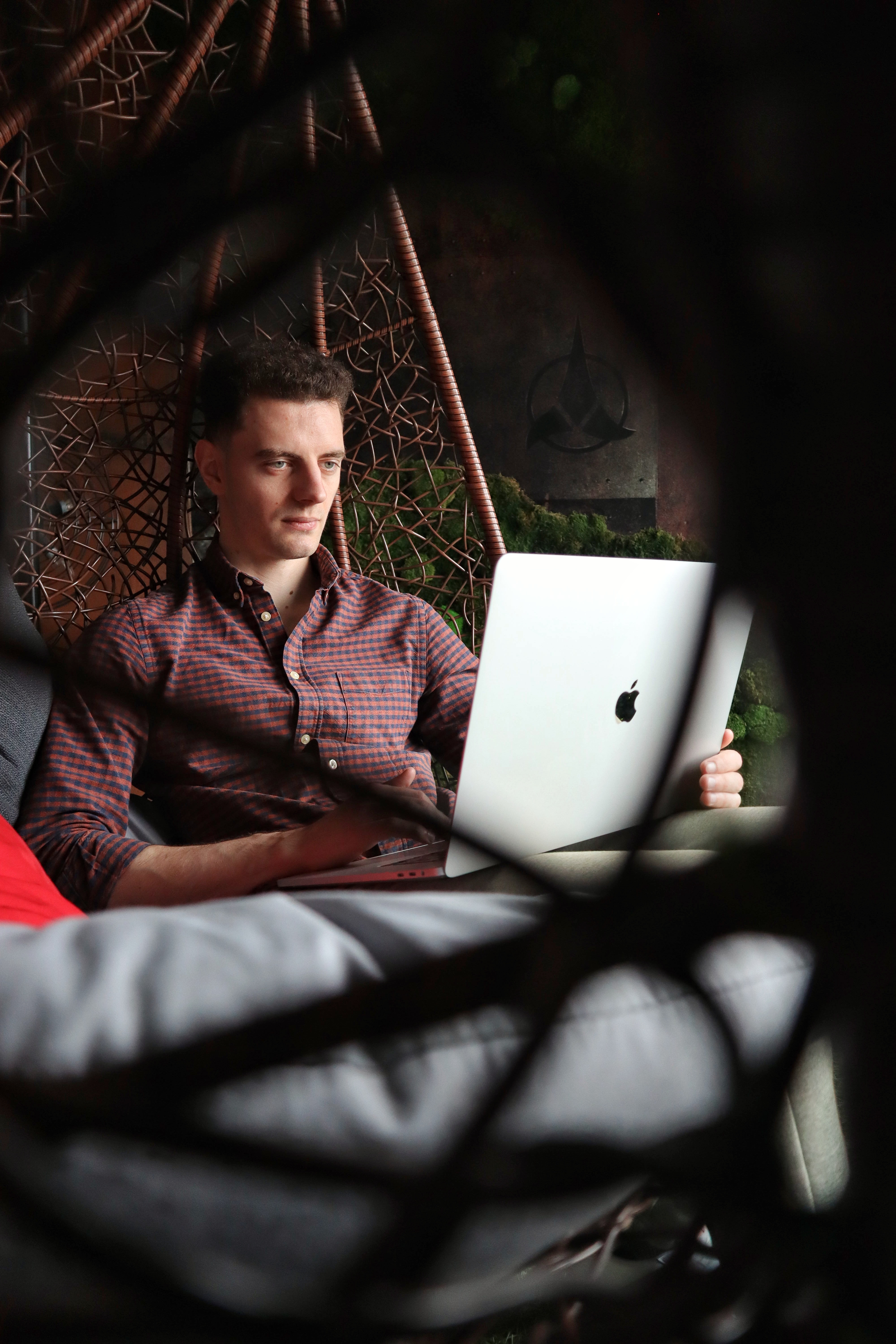
Alexandru Tudor
Alexandru Tudor
Curious Software Developer about all things Java and Python! Currently working as a Backend Software Engineer managing multiple microservices developed with Spring Boot 3 and Java 21.