Day 6 . Shell Scripting Real-Time Scenarios
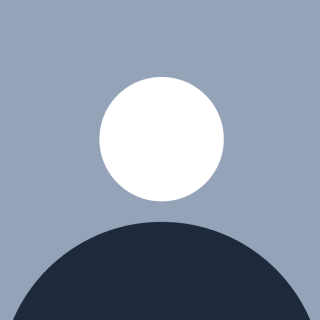
Table of contents
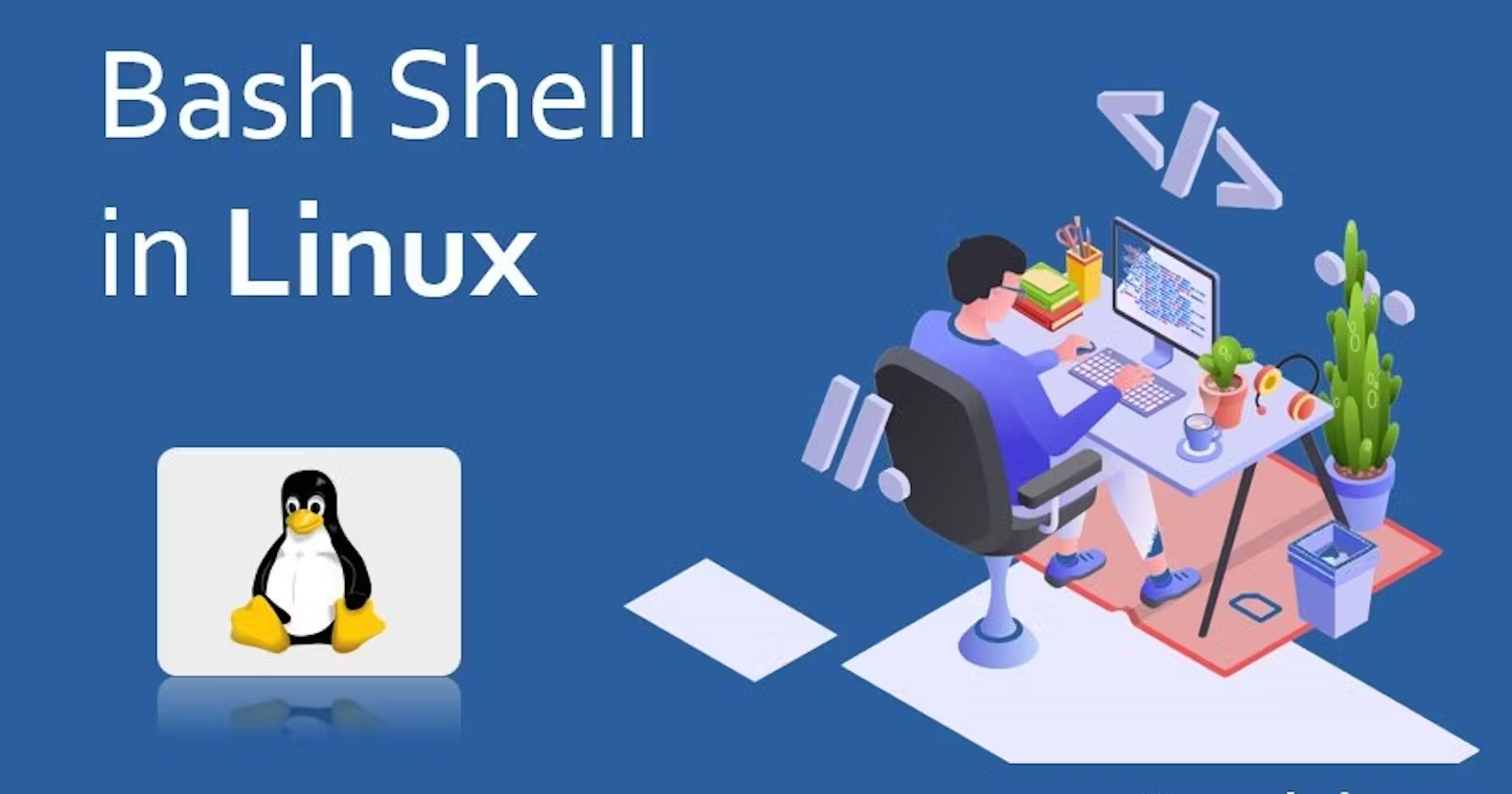
Write a shell script function to find and kill all the zombie processes. when a zombie is detected a log message is generated and sent to a log directory for when the kill is successful and not successful.
#You can create a sample zombie process using below command #(A=$BASHPID && ( kill -STOP $A )) killzombie() { #List PID of all zombie process for childid in $(ps aux | awk '$8 ~ /^[Zz]/' | awk '{print $2}') do #get parent procesid from child process parentid=$(ps -o ppid= -p $childid | awk '{print $1}') #Send Kill SIGCHLD signal to parent process echo "Sending signal to parent process to kill all zombie process" kill -s SIGCHLD $parentid #Identify process is still not killed if [[ $(ps -p $childid | awk 'NR > 1 {print $1}') -ge 0 ]] then #kill parent process echo "Kill parent process" kill -9 $parentid logger "Kill successfull $parentid" fi done } #call function to kill processes killzombie
Write a shell script to automate the process of creating new user accounts on a Linux server and setting up their permissions and SSH access.
#!/bin/bash #Create new user account createUser() { userName=$1 if id $userName then echo "User $userName already exists" exit 1 fi #Create user useradd -m $userName #Set password from user echo "Setting password for user $userName" passwd $userName #Add users to sudoers usermod -aG sudo $userName echo "User $userName is added to sudoers" #enable ssh access enableSSHAccess $userName } enableSSHAccess() { userName=$1 sshDir="/home/$userName/.ssh/" authkeyFile="$sshDir/authorized_keys" #Create ssh directory if not exist if [ ! -d $sshDir ]; then mkdir $sshDir #create ssh directory chmod 770 $sshDir #read permission for directory fi #read authorised keys from user echo "Enter public key for $userName:" read -r publicKey #write public key to authorised keys echo $publicKey >> $authkeyFile chmod 070 $authkeyFile #set ownership for ssh directory chown -R $userName $sshDir echo "SSH access enabled for $userName" } # Prompt for the username echo "Enter the username for the new user:" read -r userName # Call create user function createUser $userName
Shell script that displays the number of failed login attempts by IP address and location.
#!/bin/bash #log file logFile="/var/log/secure" #Get failed login ip addressess ipAddresses=$(cat $logFile | grep sshd | grep -v invalid | awk '{ print $11; }') #Get location of ip address getLocation() { ip=$1 location=$(geoiplookup $ip | awk -F ', ' '{ print $2 }') if [[ $location == *": "* ]] then location="${location#*: }" fi echo $location } #display all ip address for ip in $ipAddresses do noOfFailedAttempt=$(grep -c $ip $logFile) location=$(getLocation $ip) echo "Ip address=$ip, Location=$location, Failed attempt=$noOfFailedAttempt" done
Shell script to Copy files recursively to remote hosts.
#!/bin/bash hosts=("ec2-user@13.235.31.39" "ec2-user@13.235.31.39") # replace host with your hosts for host in "${hosts[@]}" do set timeout -1 #set timeout to copy all files scp -i linux.pem -r files $host:/home/ec2-user #copy all files recursively to remote host sleep 1 echo "Files copied to $host" done
Write a shell script to the list of users logged in by date and write it to an output file.
#!/bin/bash #output file outputFile="loggedinusers.txt" checkLoggedInUsers() { date=$1 who -v d="$date" | awk '{print $3" "$1}' | sort | uniq >> $outputFile } #Enter date echo "Enter date in format: YYYY-MM-DD" read date checkLoggedInUsers date
Shell script parses a log file and forwards a specific value with a timestamp to an output file.
#!/bin/bash logFile="path/logfile" #replace with your log file outputFile="/path/output.txt" #output file #pattern to be searched in file pattern="pattern" #parse file search pattern and save it to output file grep "$pattern" "$logFile" | awk '{ print $1, $2, $3 }' > "$outputFile" echo "Parsing complete, saved to #outputFile"
Shell script to monitor CPU, Memory, and Disk usage and send the output to a file in table format and send an alert if either of them exceeds a certain threshold.
#!/bin/bash ALERT=80 computestats() { printf "Memory\t\tDisk\t\tCPU\n" end=$((SECONDS+3600)) while [ $SECONDS -lt $end ]; do MEMORY=$(free -m | awk 'NR==2{printf "%.2f%%\t\t", $3*100/$2 }') if [[ $MEMORY -ge $ALERT ]] #ALert for out of memory then echo "Out of memory $MEMORY" fi DISK=$(df -h | awk '$NF=="/"{printf "%s\t\t", $5}') if [[ $DISK -ge $ALERT ]] #ALert for out of memory then echo "Disk out of space $DISK" fi CPU=$(top -bn1 | grep load | awk '{printf "%.2f%%\t\t\n", $(NF-2)}') if [[ $CPU -ge $ALERT ]] #ALert for out of memory then echo "More CPU utilization $CPU" fi echo "$MEMORY$DISK$CPU" sleep 5 done } #copy output to file computestats >> stats.txt
Write a shell script to check the status of a list of URLs and send an email notification if any of them are down.
#!/bin/bash sendEmail() { MSG_FROM=zac@server.it #Enter your mail id MSG_TO="zac@gmail.com" #Enter recepient email id MSG_SUBJ="Website down" #Enter email subject SMTP_HOST="relay.zac.it" #Enter your smtp host SMTP_PORT="487" #Enter your smtp port MSG_BODY=("Following websites are down:\n\n $1") #Enter email message body echo -e $MSG_BODY | mailx -s "$MSG_SUBJ" -r "$MSG_FROM" -S smtp="$SMTP_HOST:$SMTP_PORT" "$MSG_TO" } downUrls="" checkUrlStatus() { for url in $1 do response= $(curl -is $url | head -n 1) if [[ ! $response =~ "200 OK" ]]; then downUrls+="$url\n" fi done } #Read urls from user echo "Enter list of Urls:" readarray -t urls checkUrlStatus urls #send email if [[ -n $downUrls ]]; then sendEmail $downUrls echo "Email sent for down website" else echo "Websites are up" fi
Write a shell script to automate the process of updating a list of servers with the latest security patches.
#!/bin/bash updateServer() { server=$1 echo "Enter ssh user:" read sshuser echo "Enter path of sshkey for user:$sshuser" read sshkey #ssh into server ssh -i "$sshkey" "$sshuser" #Download the latest software packages from the repositories. sudo apt update && sudo apt upgrade -y #Will install only the security updates sudo apt-get -s dist-upgrade #Eliminates the need for unplanned maintenance sudo pro enable livepatch #Cleanup unused packages sudo apt autoremove -y } echo "Enter list of servers:" readarray -t servers for server in $servers do updateServer $server done
Shell script to find the files created and their sizes. It should accept the number of days as input. Or a from and to date format as inputs.
#!/bin/bash #Enter start date echo "Enter start date:" read startdate startdate=$(date -d $startdate +%Y-%m-%d) # format in YYYY-MM-DD #Enter end date echo "Enter end date:" read enddate enddate=$(date -d $enddate +%Y-%m-%d) echo "Size\ttDate" find /home/ec2-user -type f -newermt $startdate ! -newermt $enddate -ls | awk '{print $7" "$8" "$9}'Write a shell script to automate the process of updating a list of servers with the latest security patches.
Subscribe to my newsletter
Read articles from Ashvini Mahajan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Ashvini Mahajan
Ashvini Mahajan
I am DevOps enthusiast and looking for opportunities in DevOps.