๐ญ IIFE (Immediately Invoked Function Expression): The Instant Performer
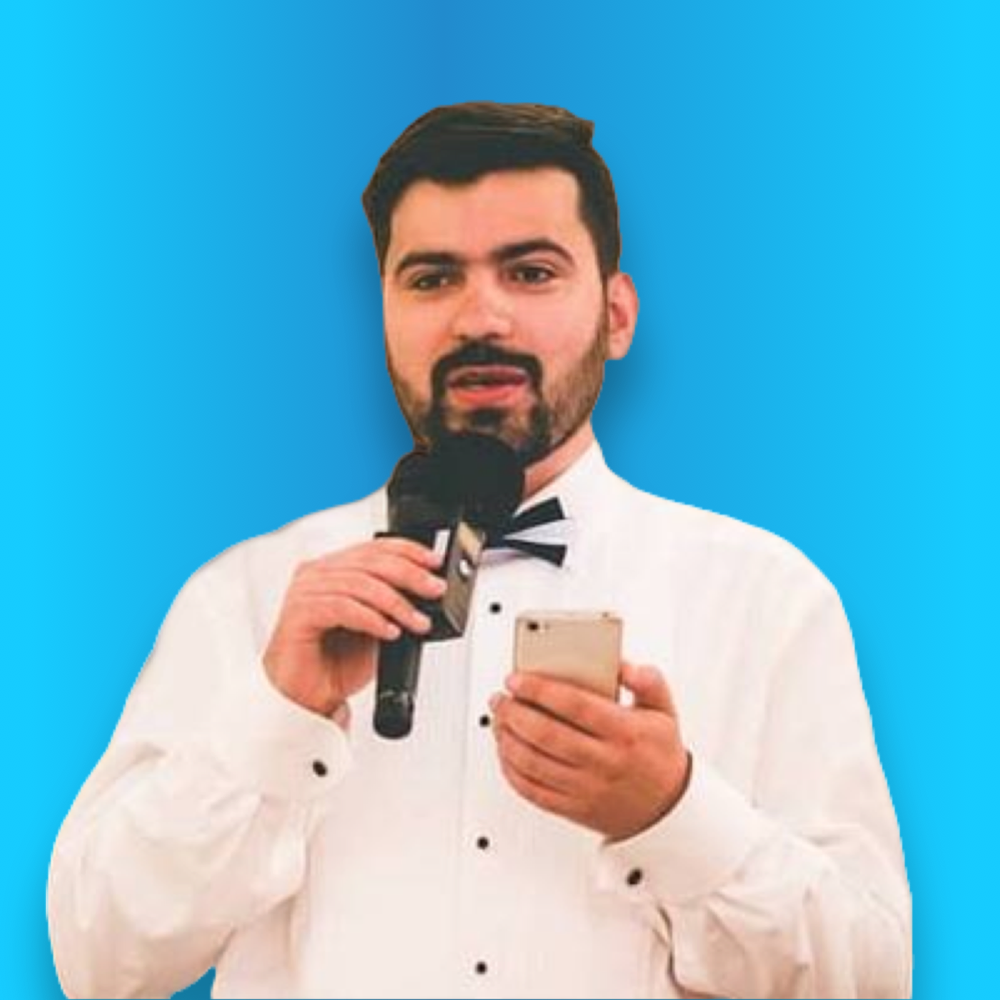
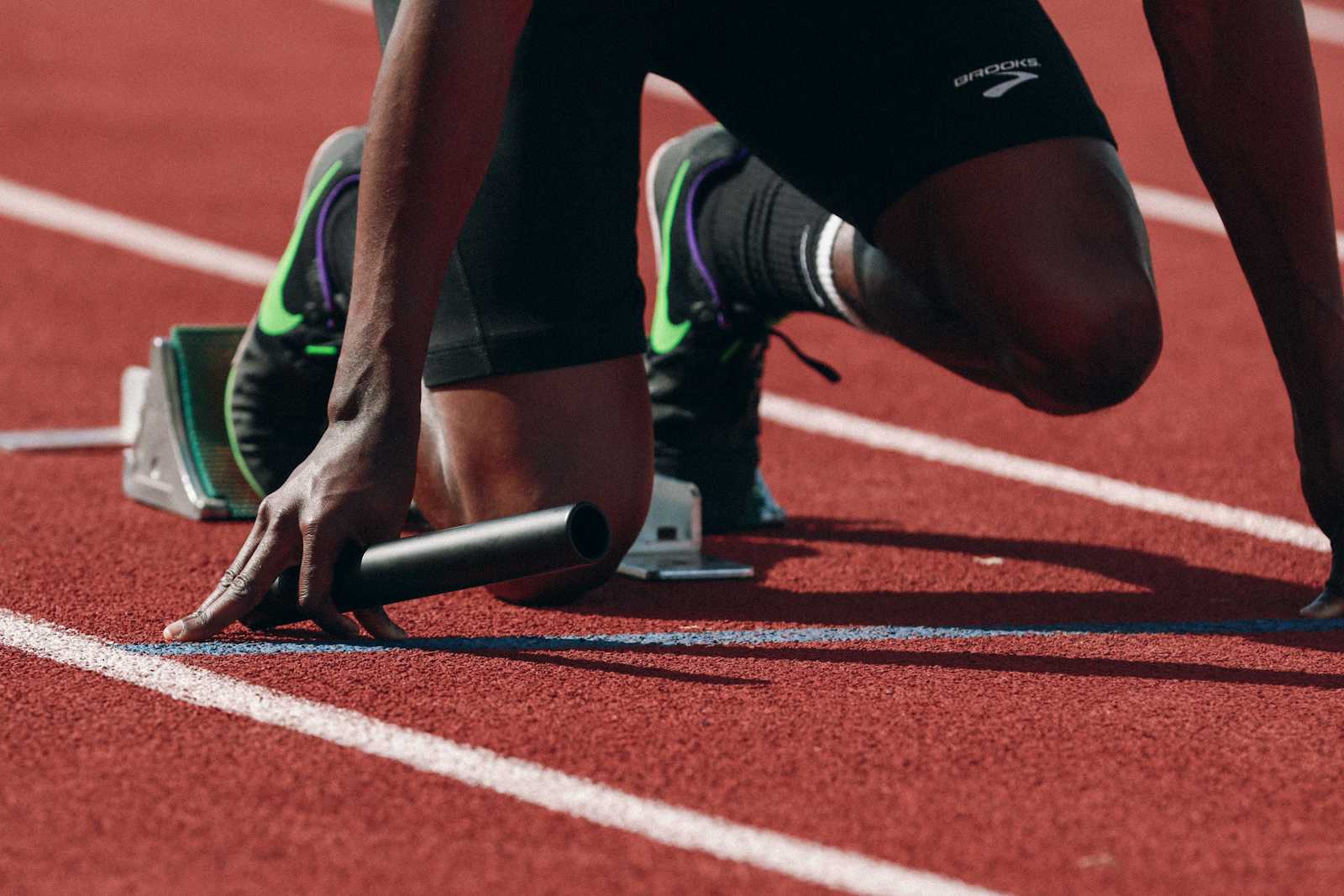
All right, fellow coders ๐ป!
Today, we're diving into the world of IIFE, those unsung heroes of JavaScript that swoop in, perform their magic and disappear without leaving a trace. Imagine a function that not only does its job but does it right away โ that's the essence of IIFE! ๐ซ
๐ What is IIFE?
IIFE stands for Immediately Invoked Function Expression. It's like the superhero of functions โ no cape, just instant action! In JavaScript, an IIFE is a function that's defined and executed right away, without being stored in a variable or waiting for a trigger. It's the ninja move of the coding world, silent and swift. ๐ฅท
(function() {
console.log("This code was executed ๐")
})();
The function is wrapped in parentheses, and the extra set at the end immediately invokes it. The result? A function that doesn't linger around like an awkward party guest but gets things done in a flash! โก๏ธ
โWhy IIFE, you ask
Immediately Invoked Function Expressions (IIFE) are the silent maestros of JavaScript, swooping in with unparalleled swiftness and disappearing without leaving a trace. ๐ญ The beauty lies in their ability to encapsulate code, preventing it from lingering in the global scope like an unwanted guest. ๐โจ IIFE excels in maintaining a tidy namespace, avoiding variable collisions, and performing quick, one-time operations. ๐ซ So, why IIFE? Because in the fast-paced world of coding, sometimes you need a function that acts like a ninja โ precise, immediate, and gone in a flash! โก
๐ Encapsulation Magic
IIFE is like a mini universe where variables play nicely without polluting the global scope. It's a neat trick to encapsulate your code and shield it from the chaos outside.
(function() {
var secret = "I am hidden!";
console.log(secret);
})();
// secret is undefined here
// console.log(secret)
๐ Preventing Variable Collisions
Since IIFE creates a private space for your code, it's fantastic for avoiding variable collisions. No more accidental clashes with other scripts or libraries โ it's your code's safe haven.
n this playful example, emojis are used to represent different aspects of the code. The rocket ๐ and globe ๐ represent the power of the IIFE to encapsulate variables, while the celebration ๐ emoji adds a cheerful touch to the function call. The caution ๐ซ emoji signals that attempting to access internalVariable
outside the IIFE will result in an error. Adding emojis can make your code not only functional but also visually engaging! ๐จ
(() => {
// ๐ This is an IIFE (Immediately Invoked Function Expression)
// ๐ Variables declared inside the IIFE have their own scope
let internalVariable = "I am inside the IIFE";
// ๐ Function that can access the internalVariable
function doSomething() {
console.log(internalVariable);
}
// ๐ Call the function
doSomething();
})();
// ๐ซ Attempting to access internalVariable outside the IIFE will result in an error
// console.log(internalVariable); // ๐จ This would throw an error: "Uncaught ReferenceError: internalVariable is not defined"
๐ Module Pattern Feels
IIFE is the unsung hero behind the module pattern in JavaScript. It enables you to create modular, reusable pieces of code without polluting the global namespace.
const myModule = (function() {
const privateVar = "I'm hidden!";
return {
getPrivateVar: function() {
return privateVar;
}
};
})()
console.log(myModule.getPrivateVar()) // Output: I'm hidden
console.log(myModule.privateVar) // Ouput: undefined
๐ซฃ Avoiding Hoisting Headaches
IIFE helps you sidestep the hoisting quagmire. Variables declared within an IIFE stay right where you put them, preventing unexpected behaviors.
(function() {
console.log(notHoisted); // undefined
var notHoisted = "I won't cause trouble";
})();
๐ช Going deep on IIFE
Now that we've uncovered the basics of Immediately Invoked Function Expressions (IIFE), let's embark on a journey to explore their versatility and unleash their full potential. Buckle up, fellow coders โ the thrill is just beginning! ๐
๐ซ Passing Arguments like a Pro
IIFE isn't just about executing code immediately; it's also about passing in arguments and creating dynamic, on-the-fly functionality. Think of it as a recipe where you toss in some ingredients, and voilร โ instant functionality costumize to your needs.
(function(name) {
console.log(`Hello, ${name}!`);
})("Coder"); // Outputs: Hello, Coder!
๐ Asynchronous Bliss with IIFE
IIFE's speed and agility make it an excellent candidate for handling asynchronous tasks. Wrap your asynchronous code within an IIFE, and you have a self-contained unit that won't interfere with the rest of your program. It's like having a race car for handling promises and callbacks!
// Imagine you have some asynchronous code using promises
function simulateAsyncOperation() {
return new Promise((resolve) => {
setTimeout(() => {
console.log("Async operation complete!");
resolve("Data from async operation");
}, 2000);
});
}
// Now, let's wrap it in an IIFE (Immediately Invoked Function Expression)
(() => {
console.log("Start of program");
// Inside the IIFE, you can handle asynchronous tasks with promises
(async () => {
try {
const result = await simulateAsyncOperation();
console.log("Async operation result:", result);
} catch (error) {
console.error("Error during async operation:", error);
}
})();
console.log("End of program");
})();
๐ค Embracing ES6 Arrow Functions
With the rise of ES6, arrow functions have become the cool kids on the block. IIFE plays exceptionally well with arrow functions, creating a concise and expressive syntax that's a joy to work with.
(() => {
// Arrow function IIFE goodness
})();
๐ฑ Event Handling Simplified
IIFE shines in scenarios where you want to attach event handlers without cluttering your code with unnecessary functions. This is particularly handy when you're working on small, self-contained tasks.
// Imagine you have a simple HTML button with an ID "myButton"
const myButton = document.getElementById("myButton");
// Without IIFE, you might define a separate function for the event handler
function handleClick() {
console.log("Button clicked!");
// Your code for handling the button click goes here
}
// Attach the event handler to the button
myButton.addEventListener("click", handleClick);
// With IIFE, you can achieve the same without cluttering your code
(() => {
// Your self-contained code here, maybe some setup
// Attach the event handler directly without a separate function
myButton.addEventListener("click", () => {
console.log("Button clicked!");
// Your code for handling the button click goes here
});
// Your self-contained code here, maybe some cleanup
})();
๐ป Debugging Friend or Foe?
While IIFE has its merits, debugging can be a tad tricky since you're working with anonymous functions. Fear not! Modern browser developer tools have evolved to assist in tracking down these elusive creatures, making debugging less of a headache.
๐ญ Final thoughts
In the grand symphony of JavaScript, IIFE plays a unique role โ the instant performer, the silent guardian of encapsulation, and the protector of variable harmony. So, the next time you want your code to shine without causing global chaos, call upon the magic of IIFE. ๐ฉ
As we wrap up our exploration of IIFE, remember that this instant performer is more than just a quick solution. It's a versatile tool that aids in encapsulation, prevents variable collisions, and enhances the modularity of your code. Embrace IIFE, experiment with its capabilities, and let it be the magic wand in your coding adventures! ๐๐ฎ Happy coding, and may your functions always be invoked swiftly and with purpose! ๐๐ป
โจ Happy coding, and may your functions be invoked swiftly! ๐๐ป
โ๏ธ References
Subscribe to my newsletter
Read articles from Ricardo Rocha // ๐จโ๐ป directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
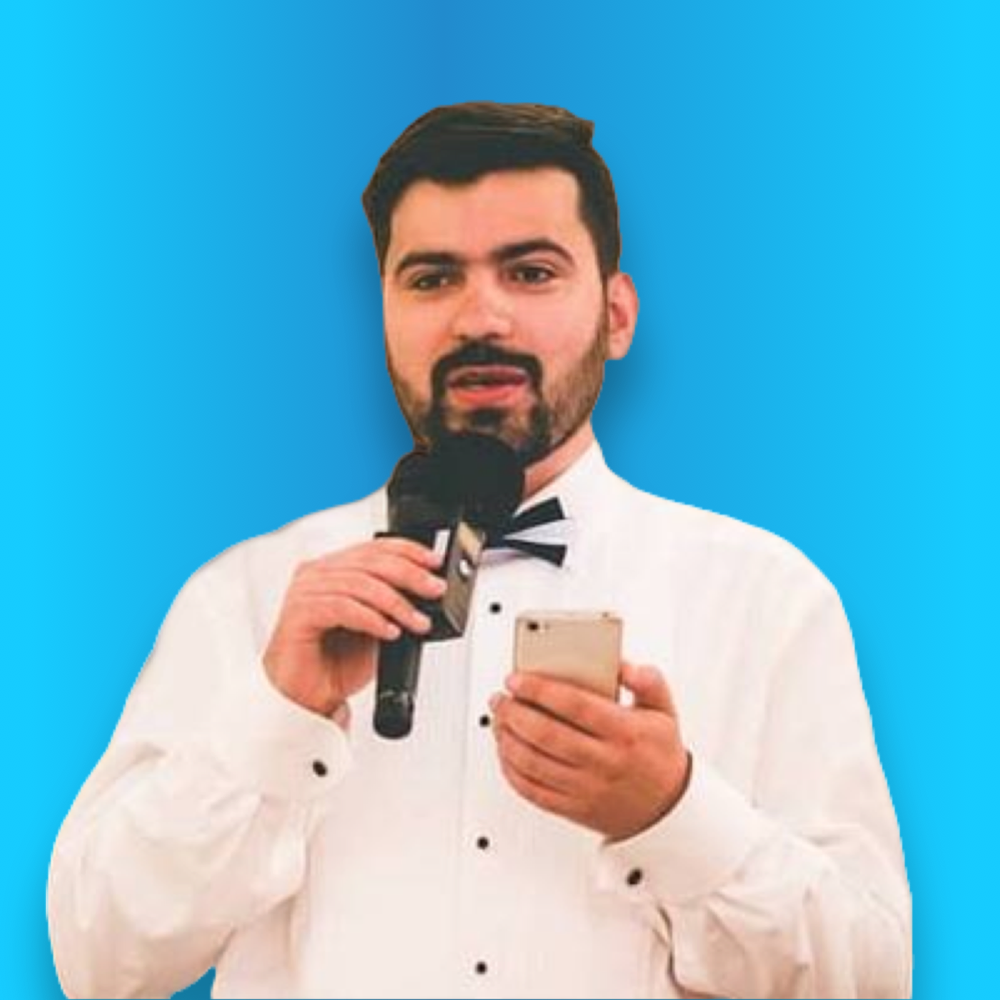
Ricardo Rocha // ๐จโ๐ป
Ricardo Rocha // ๐จโ๐ป
Hey there ๐! ๐ So, here's the lowdown โ I'm a full-stack web dev with a serious crush on front-end development. Armed with a master's in Software Engineering, I've been rocking the programming scene for a solid decade. I've got this knack for software architecture, team and project management, and even dabble in the magical realm of deep learning (yeah, AI, baby!). My coding toolbox ๐งฐ is stacked โ JavaScript, TypeScript, React, Angular, C#, SQL, NoSQL - you name it. Nevertheless, learning is my best tool ๐! But here's the thing โ I'm not just about the code. My soft skills game is strong โ think big-picture pondering, critical thinking, and communication skills sharper than a ninja's blade. Leading, mentoring, and rocking successful projects? Yeah, that's my jam as well. Now, outside the coding dojo, I'm a music lover. Saxophone and piano are my instruments of choice, teaching me the art of teamwork and staying cool under pressure. I've got a soft spot for giving back too ๐ฅฐ. I've lent a hand to the Jacksonville Human Society (dog shelter). And speaking of sharing wisdom, I also write blogs and buzz around on Twitter, LinkedIn, Stackoverflow and my own Blog. Go ahead and check me out: Linkedin (https://www.linkedin.com/in/ricardogomesrocha/) Stackoverflow (https://stackoverflow.com/users/5148197/ricardo-rocha) Twitter (https://twitter.com/RochaDaRicardo) Github (https://github.com/RicardoGomesRocha) Blog (https://ricardo-tech-lover.hashnode.dev/) Let's connect and dive into the exciting world of web development! Cheers ๐ฅ, Ricardo ๐