Dart List: List Extensions | List Methods | List Properties
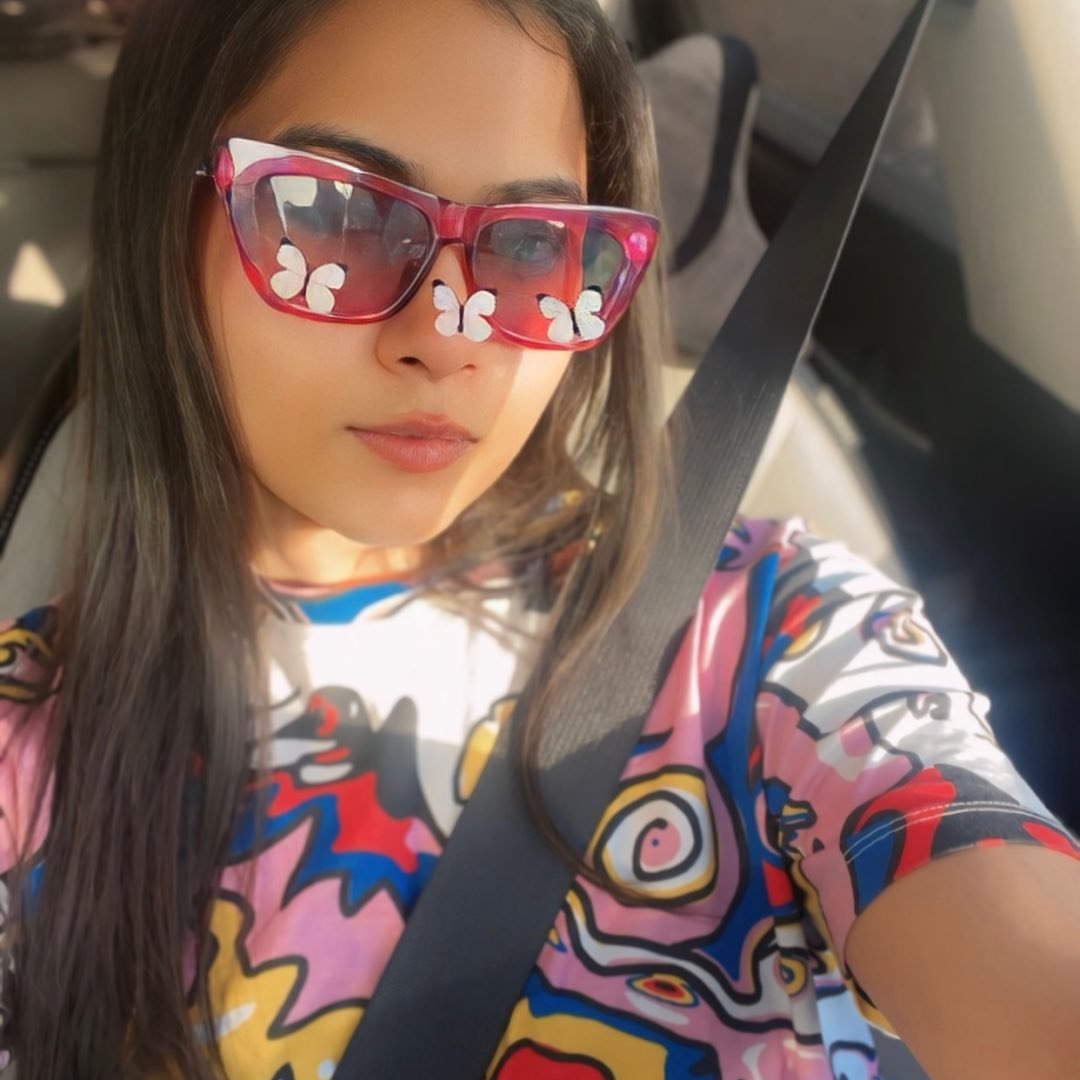
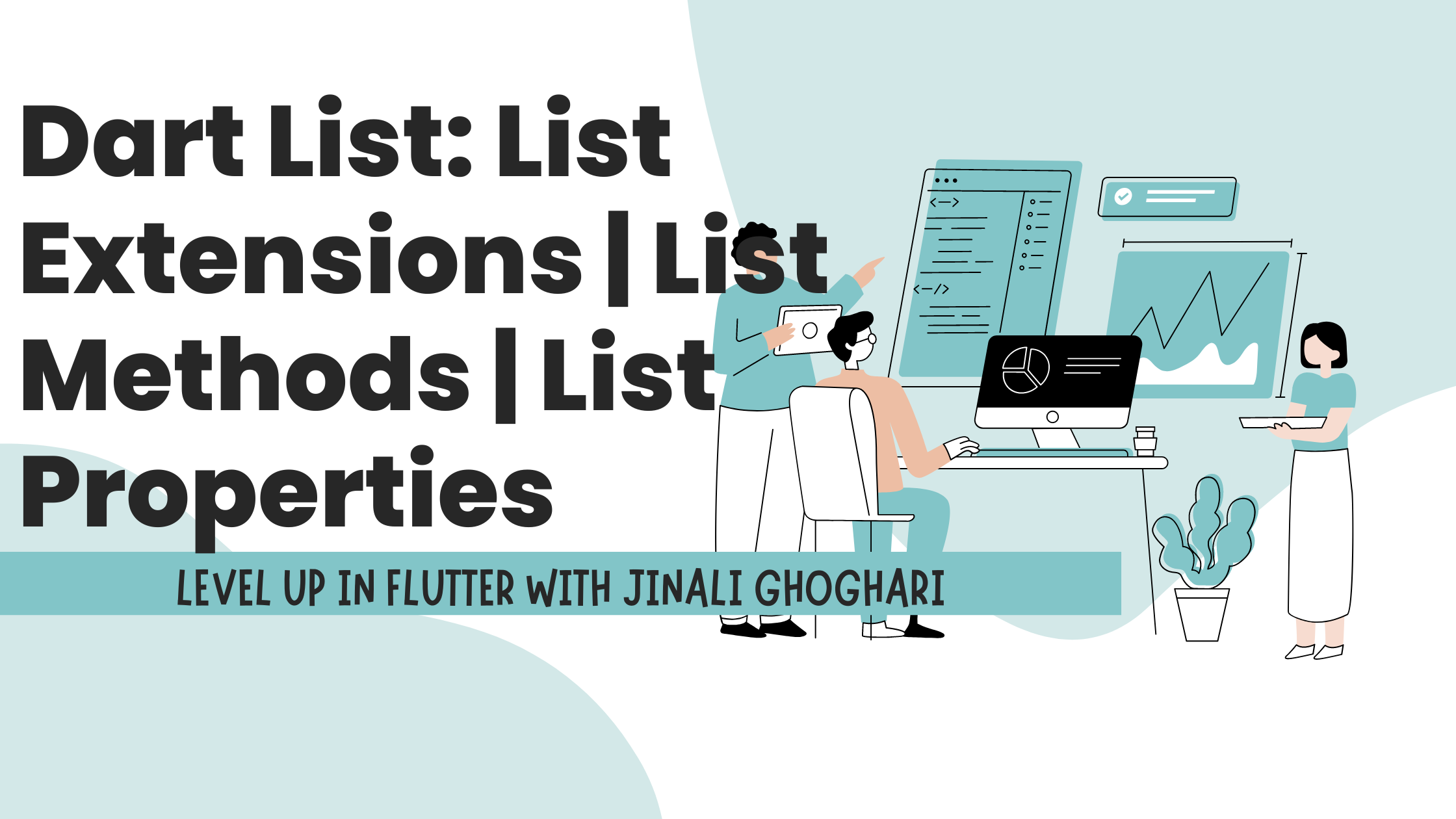
Dart's List class is a fundamental data structure used for storing collections of objects. Understanding how to work with lists efficiently is crucial for any Dart developer. In this blog post, we'll delve into Dart Lists, exploring extensions, methods, and properties that make manipulating lists easier and more powerful.
List Extensions:
Dart allows you to extend the functionality of the List class using extensions.
Extensions provide a way to add new methods to existing classes without modifying their source code.
Here's how you can create extensions for Lists:
extension ListExtensions<T> on List<T> { // Custom extension methods can be defined here }
Common List Methods:
Dart provides a rich set of methods for working with lists.
Let's explore some of the most commonly used ones:
add(element)
: Adds the specified element to the end of the list.remove(element)
: Removes the first occurrence of the specified element from the list.addAll(elements)
: Adds all elements of the specified iterable to the end of the list.removeAt(index)
: Removes the element at the specified index from the list.contains(element)
: Returns true if the list contains the specified element, otherwise returns false.
Essential List Properties:
Understanding the properties of lists can be invaluable for efficient programming.
Here are some key properties of Dart lists:
length
: Returns the number of elements in the list.isEmpty
: Returns true if the list is empty, otherwise returns false.isNotEmpty
: Returns true if the list is not empty, otherwise returns false.first
: Returns the first element of the list.last
: Returns the last element of the list.
Example Usage:
Let's put some of these methods and properties into action with a simple example:
void main() { List<int> numbers = [1, 2, 3, 4, 5]; // Using list methods numbers.add(6); numbers.remove(3); // Using list properties print("Length of the list: ${numbers.length}"); print("Is the list empty? ${numbers.isEmpty}"); print("First element of the list: ${numbers.first}"); }
Subscribe to my newsletter
Read articles from Jinali Ghoghari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
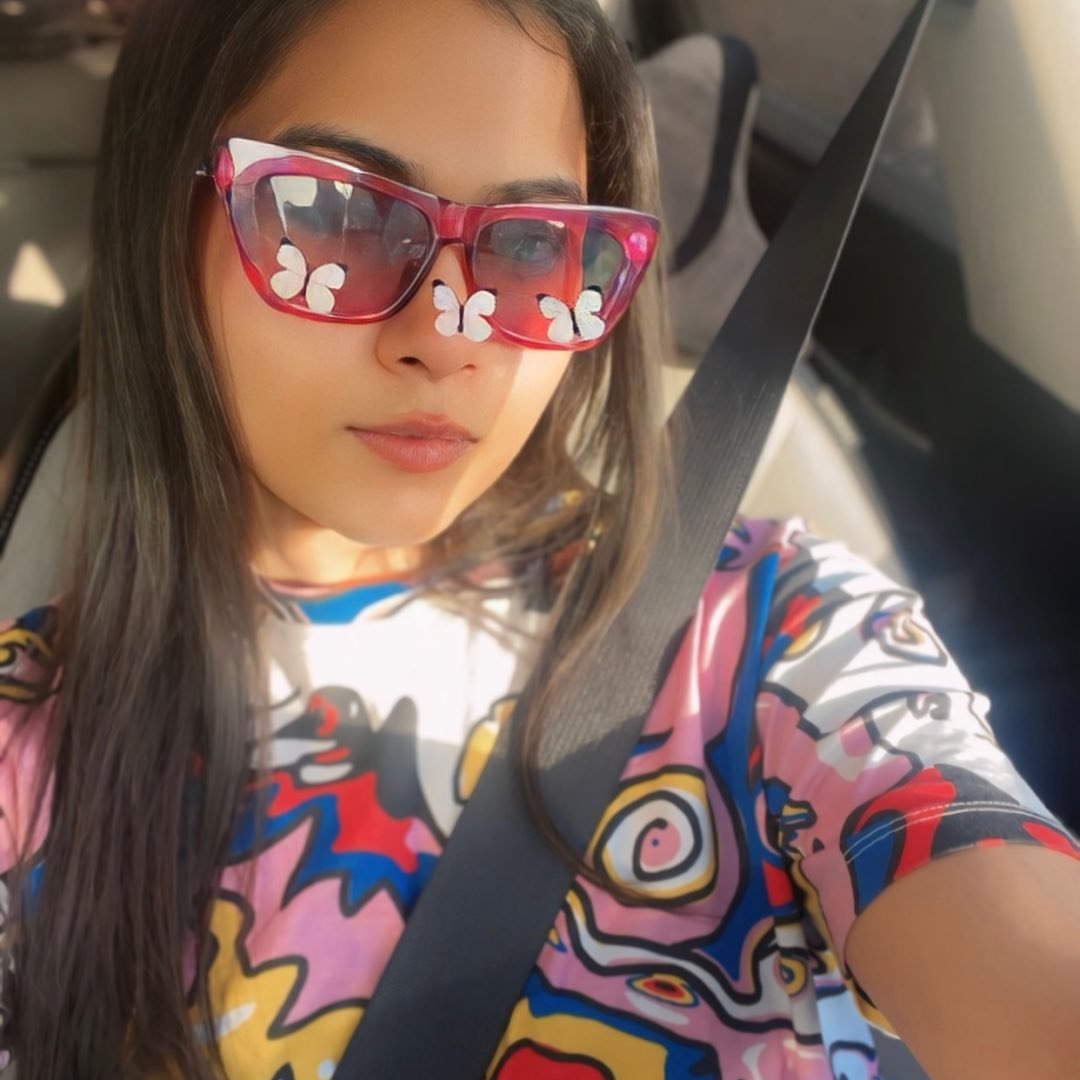