Dart Sets: Set Relationships
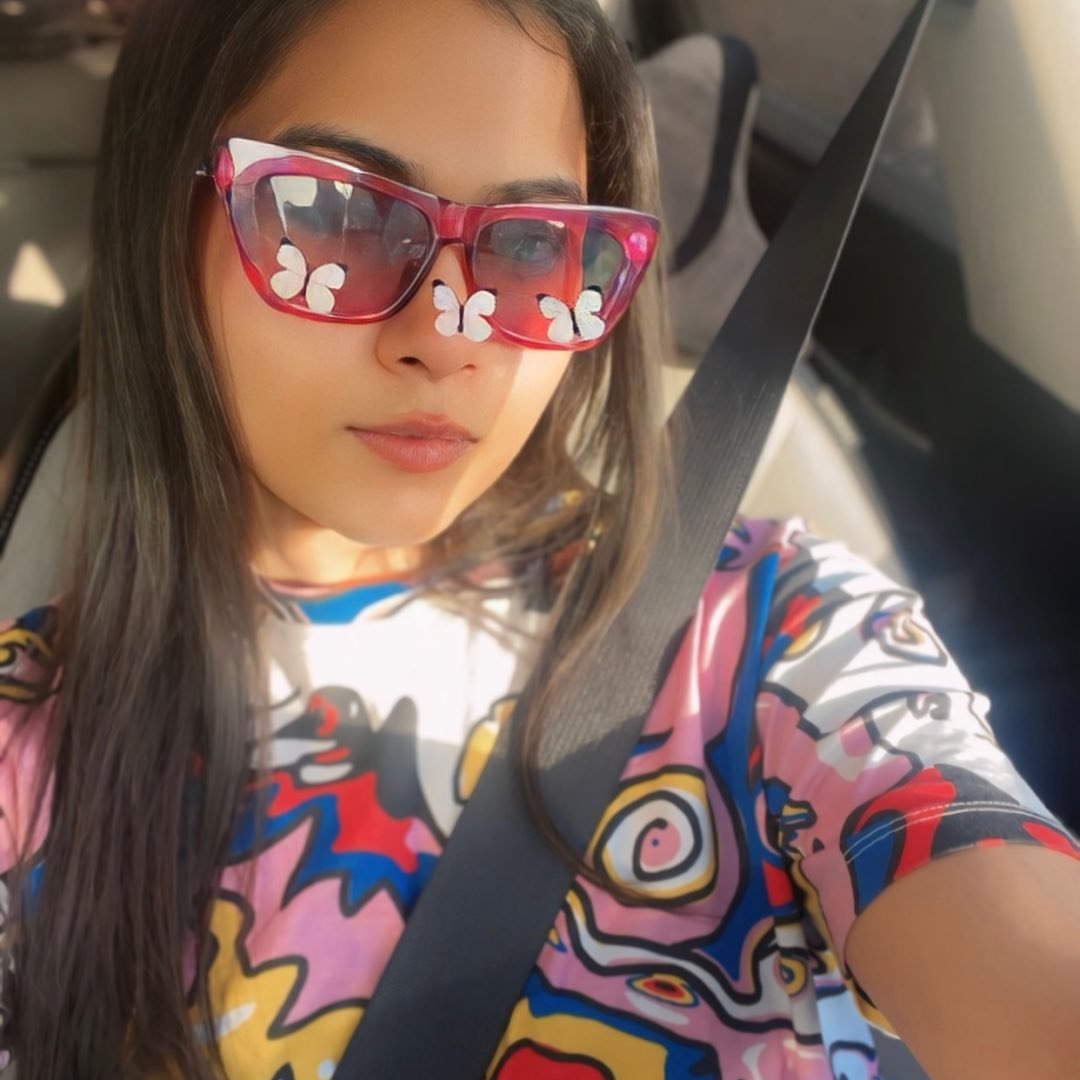
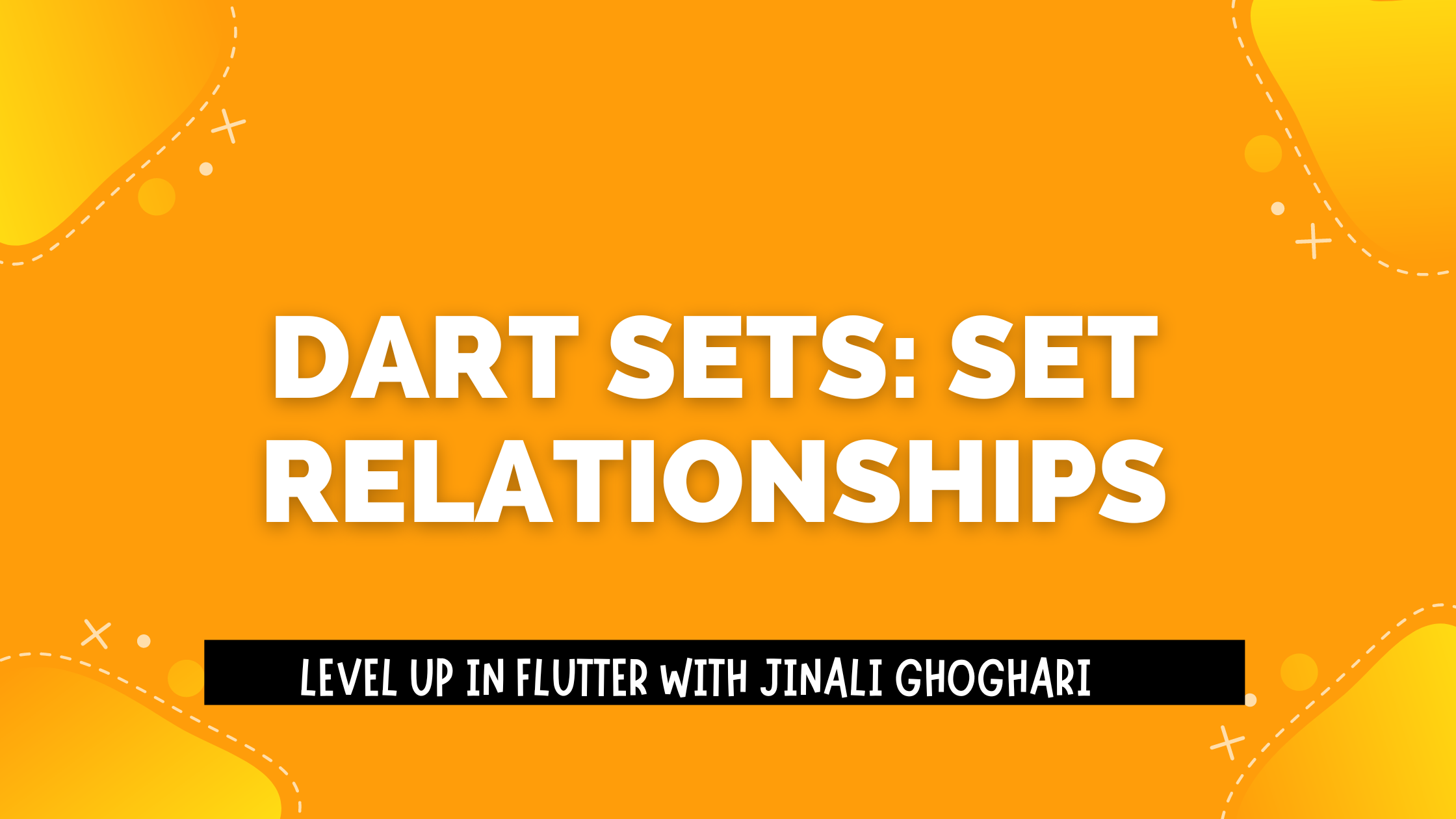
In Dart programming, sets are invaluable data structures for managing collections of unique elements.
While sets provide efficient methods for adding, removing, and checking elements, they also offer functionalities to explore relationships between sets.
Subset and Superset Relationships:
In Dart, you can check if one set is a subset or superset of another using the containsAll
method.
Subset: A set A is considered a subset of another set B if every element of A is also in B.
Superset: A set A is considered a superset of another set B if A contains all elements of B.
void main() {
var set1 = {1, 2, 3, 4};
var set2 = {2, 4};
print('Is set2 a subset of set1? ${set1.containsAll(set2)}'); // true
print('Is set1 a superset of set2? ${set2.containsAll(set1)}'); // false
}
Disjoint Sets:
Two sets are considered disjoint if they have no common elements.
Dart provides a concise way to check if sets are disjoint using the intersection
method.
void main() {
var set1 = {1, 2, 3};
var set2 = {4, 5, 6};
print('Are set1 and set2 disjoint? ${set1.intersection(set2).isEmpty}'); // true
}
Set Equality:
Set equality refers to two sets having exactly the same elements, regardless of their order.
Dart offers the equals
method to compare sets for equality.
void main() {
var set1 = {1, 2, 3};
var set2 = {3, 2, 1};
print('Are set1 and set2 equal? ${set1.equals(set2)}'); // true
}
Conclusion:
By leveraging methods like containsAll
, intersection
, and equals
, you can explore and manipulate sets to suit your application's needs effectively.
Subscribe to my newsletter
Read articles from Jinali Ghoghari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
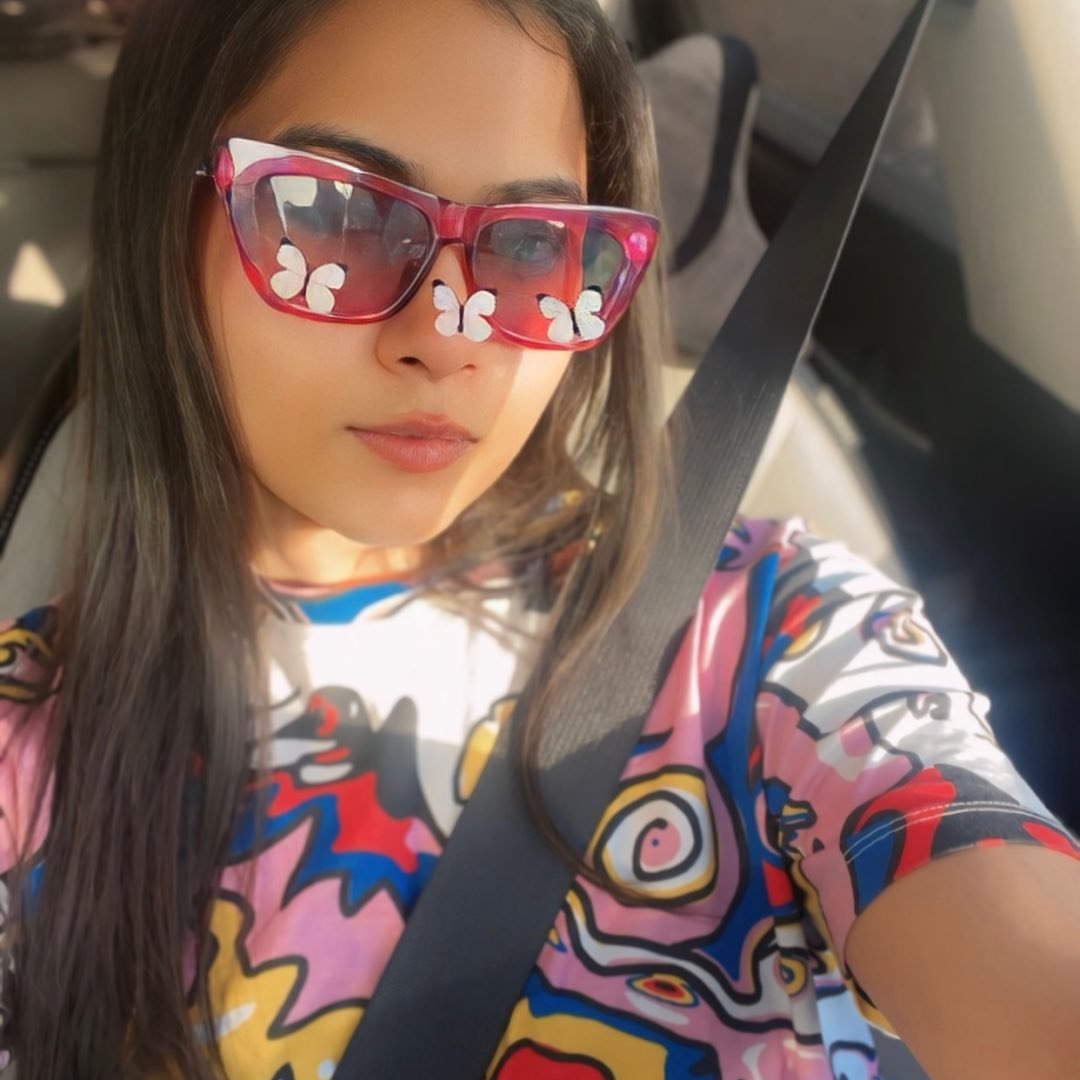