Unlocking Frontend Development Creativity with OpenAI

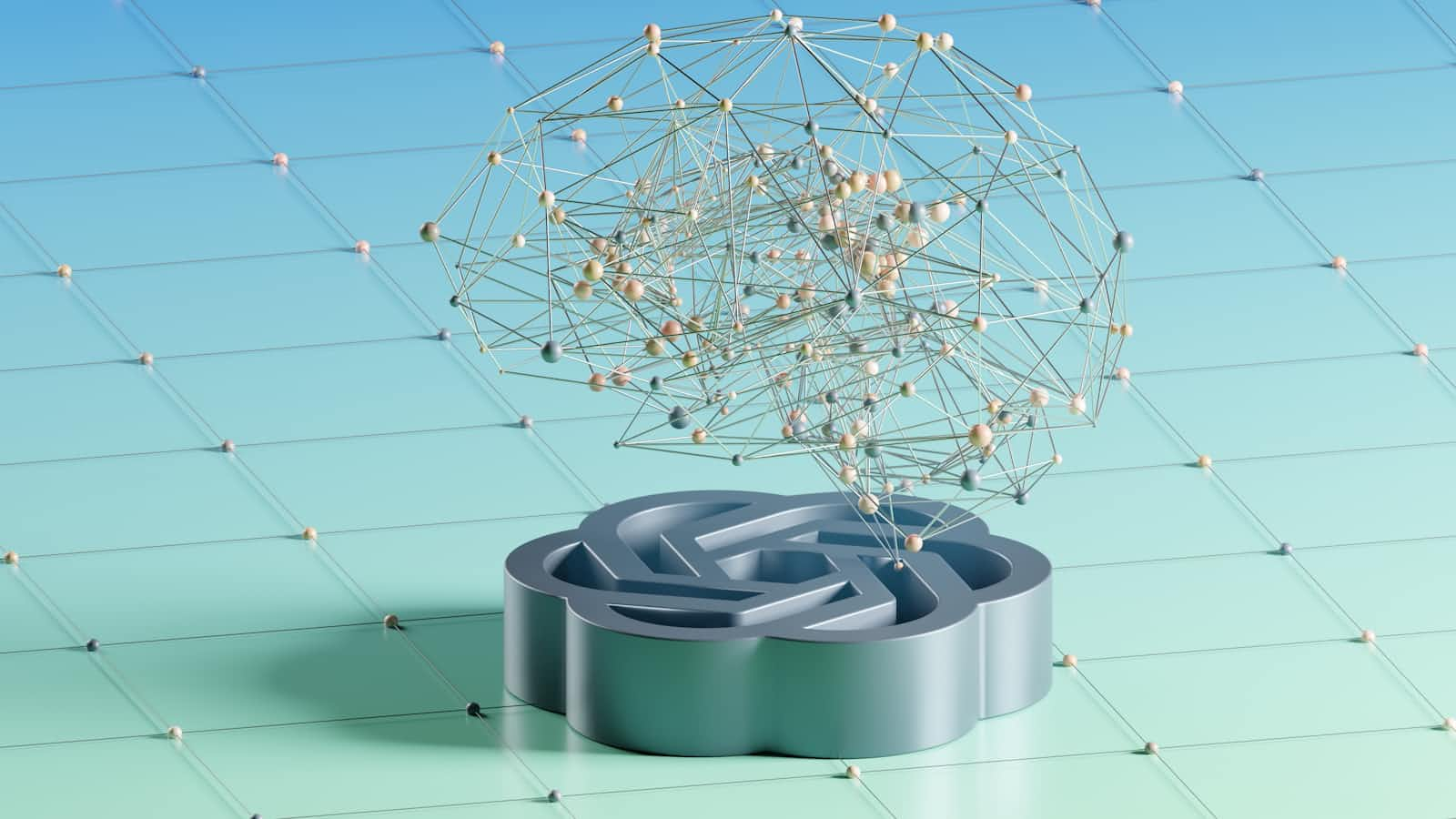
Picture the capability to effortlessly generate product descriptions, blog posts, or even chatbot replies using only a handful lines of code. Thanks to the OpenAI API, this vision transforms into reality. Harnessing it's text generation ability enables us to automate everyday tasks, liberating time for more imaginative pursuits.
Harnessing the Potential of OpenAI:
The OpenAI API offers developers access to advanced artificial intelligence models trained on extensive datasets. These models excel in tasks ranging from generating human-like text to producing realistic images, unlocking a multitude of possibilities for developers.
Enhancing User Experience:
As frontend developers, our goal is to create engaging and interactive experiences for users. With the OpenAI API, we can take user interactions to the next level by incorporating natural language processing (NLP) capabilities into our applications. For example, we can use the API to generate dynamic and personalized content based on user input, leading to a more immersive user experience.
Adding a Touch of Magic:
Imagine having the ability to automatically generate product descriptions, blog posts, or even chatbot responses with just a few lines of code. By leveraging it's text generation capabilities, we can automate tedious tasks and free up time for more creative endeavors.
Empowering Creativity:
One of the most exciting aspects of the OpenAI API is it's ability to inspire creativity. Whether we're brainstorming ideas for a new project or seeking inspiration for a design, the API can generate a wealth of content to spark our imagination. From generating poetry to creating artwork, the possibilities are endless.
Integrating with React:
For frontend developers working with React, integrating the OpenAI API is straightforward. We can use libraries like Axios
import React, { useState } from 'react';
import axios from 'axios';
const OpenAIComponent = () => {
const [response, setResponse] = useState(null);
const [error, setError] = useState(null);
const [input, setInput] = useState("");
const fetchData = async () => {
try {
// Replace this with your OpenAI API key
const apiKey = 'YOUR_OPENAI_API_KEY';
const response = await axios.post(
// Choose the api endpoint you want to use
'https://api.openai.com/v1/completions',
{
// Choose the model you want to use
model: 'gpt-3.5-turbo',
messages: [
{
role: 'user',
content: 'input'
},
],
},
{
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
}
}
);
const botResponse = response.data.choices[0].response.content;
setResponse([...messages, { text: botResponse, user: "bot" }]);
setInput("");
} catch (error) {
setError(error.message);
}
};
};
or the built-in fetch API to make HTTP requests to the API endpoints.
import React, { useState } from 'react';
const OpenAIComponent = () => {
const [response, setResponse] = useState(null);
const [error, setError] = useState(null);
const [input, setInput] = useState("");
const fetchData = async () => {
try {
// Replace this with your OpenAI API key
const apiKey = 'YOUR_OPENAI_API_KEY';
const response = await fetch(
// Choose the api endpoint you want to use
'https://api.openai.com/v1/completions', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
},
body: JSON.stringify({
// Choose the model you want to use
model: 'gpt-3.5-turbo',
messages: [
{
role: 'user',
content: 'input'
},
],
})
});
const responseData = await response.json();
const botResponse = responseData.choices[0].message.content;
setResponse([...messages, { text: botResponse, user: "bot" }]);
setInput("");
} catch (error) {
setError(error.message);
}
};
};
Once we receive the response, we can easily incorporate the generated content into our React components, seamlessly.
Conclusion
In conclusion: The integration of OpenAI into frontend development revolutionizes user experiences by automating tasks and fostering creativity. Through natural language processing, we dynamically generate content, personalize interactions, and infuse applications with innovation. With seamless integration into React, OpenAI empowers developers to push boundaries, shaping richer digital experiences for users.
Subscribe to my newsletter
Read articles from Yahya Dahir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
