Dart Collections: Dart Map
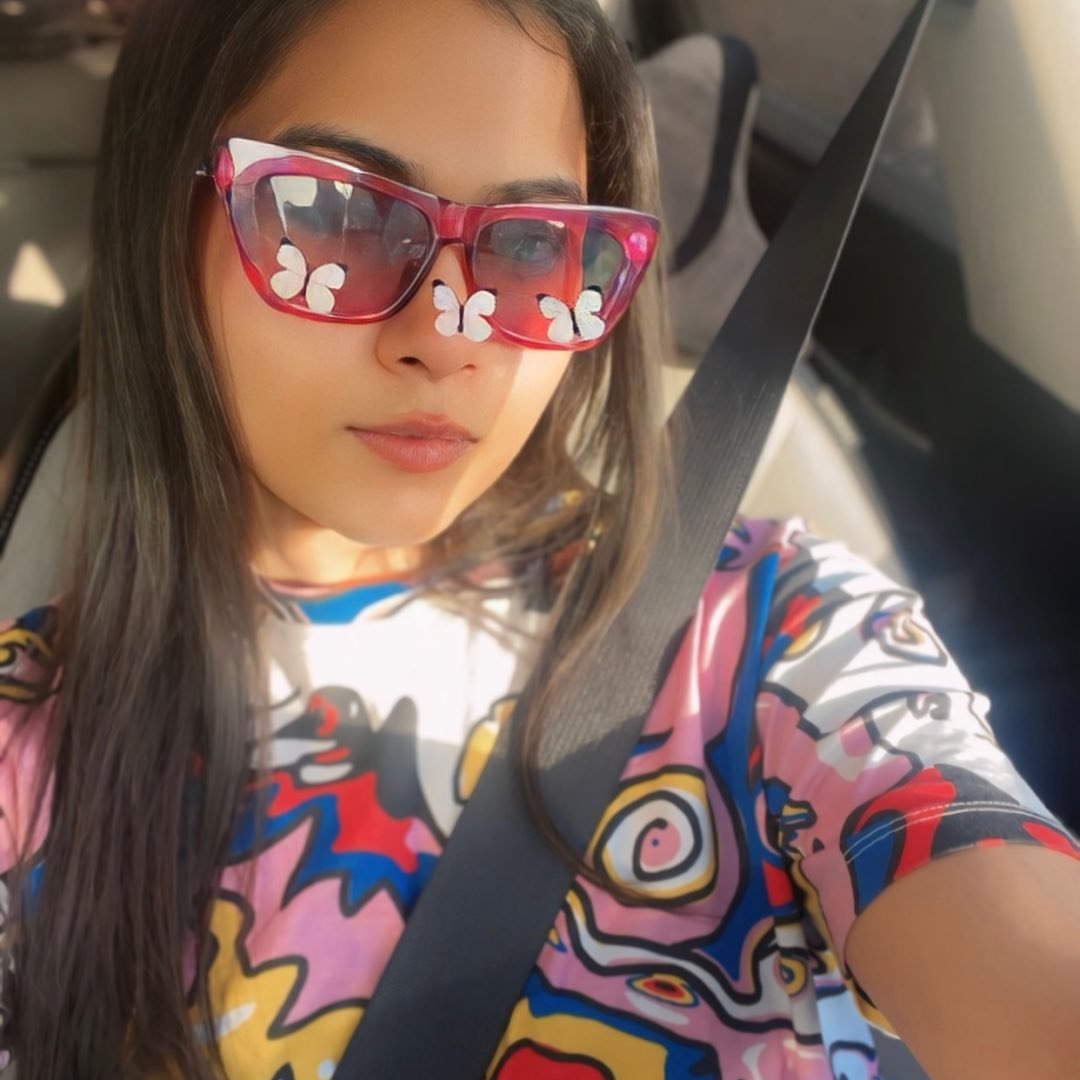
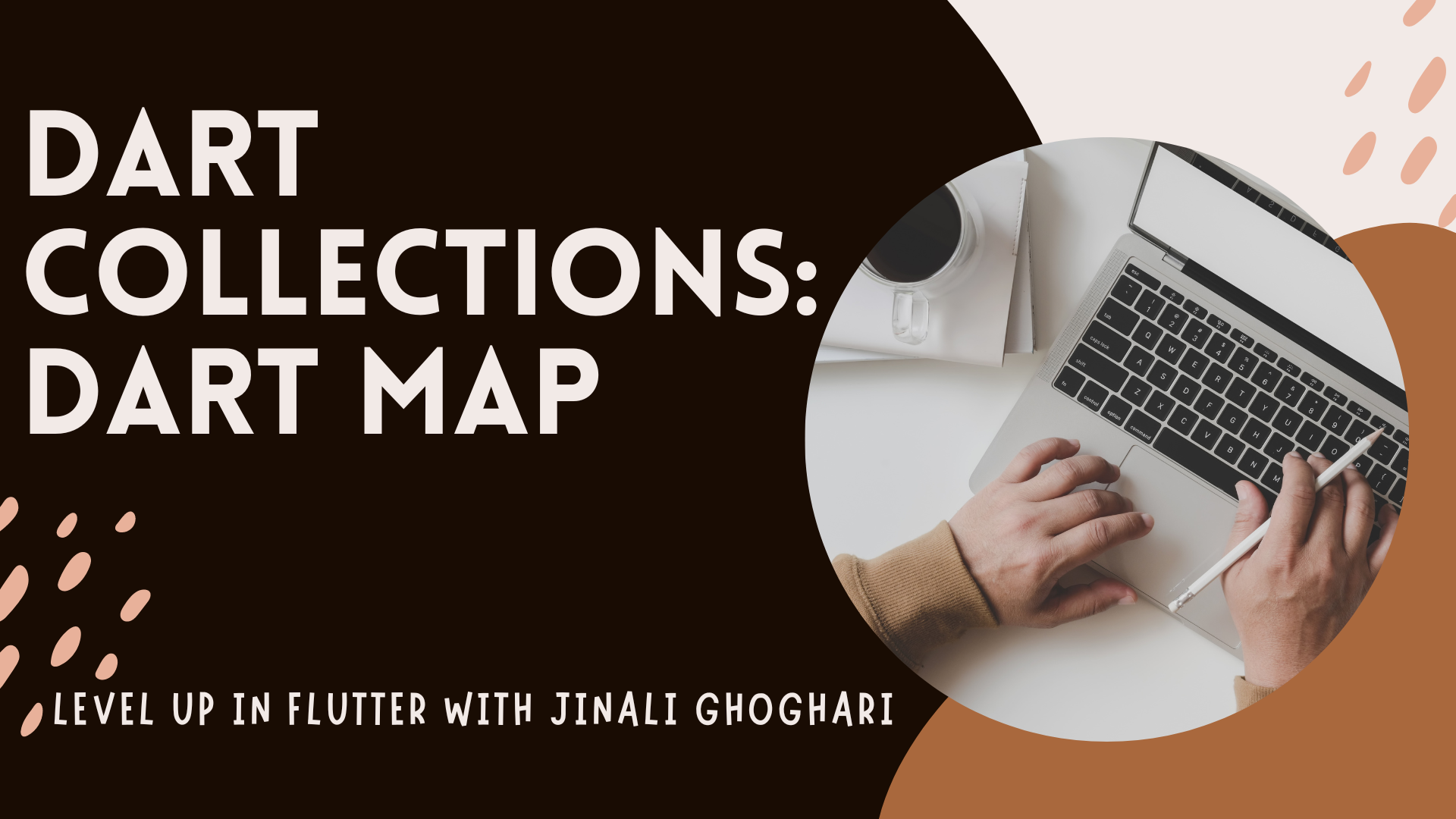
Imagine you have a box of labeled jars. Each jar has a unique label (key), and inside each jar, there's something different (value). For example:
Jar labeled "Apple" contains 5 apples.
Jar labeled "Banana" contains 3 bananas.
Jar labeled "Orange" contains 2 oranges.
Here, the labels ("Apple", "Banana", "Orange") are like keys in a Dart Map, and the contents of each jar (5 apples, 3 bananas, 2 oranges) are the corresponding values. Just like in Dart Maps, each label (key) is unique, and you can easily find out what's inside a jar (value) by reading its label (key).
So, in essence, a Dart Map is like your box of labeled jars, making it easy to organize and access different items quickly.
void main() {
// Using map literal
var fruits = {'Apple':5, 'Banana':3, 'Orange':2};
Creating Dart Maps:
// Using Map literal
var fruits = {'apple': 5, 'banana': 3, 'orange': 2};
// Using Map constructor
var vegetables = Map<String, int>();
vegetables['carrot'] = 30;
vegetables['broccoli'] = 25;
Accessing Values in Dart Maps
Accessing values in a Dart Map is simple. You can retrieve a value associated with a key using the square bracket notation []
or using the forEach
method to iterate over all key-value pairs.
print(fruits['apple']); // Output: 5
fruits.forEach((key, value) {
print('$key : $value');
});
Modifying Dart Maps
Dart Maps provide various methods to modify their contents. You can add new key-value pairs, remove existing ones, or update values associated with keys.
fruits['grapes'] = 12; // Adding a new key-value pair
fruits.remove('banana'); // Removing a key-value pair
fruits['apple'] = 15; // Updating the value associated with the 'apple' key
Dart Map Properties and Methods
Dart Maps come with several properties and methods to facilitate data manipulation. Some commonly used ones include:
length
: Returns the number of key-value pairs in the Map.keys
: Returns an iterable containing all keys in the Map.values
: Returns an iterable containing all values in the Map.containsKey(key)
: Returns true if the Map contains the specified key.
Subscribe to my newsletter
Read articles from Jinali Ghoghari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
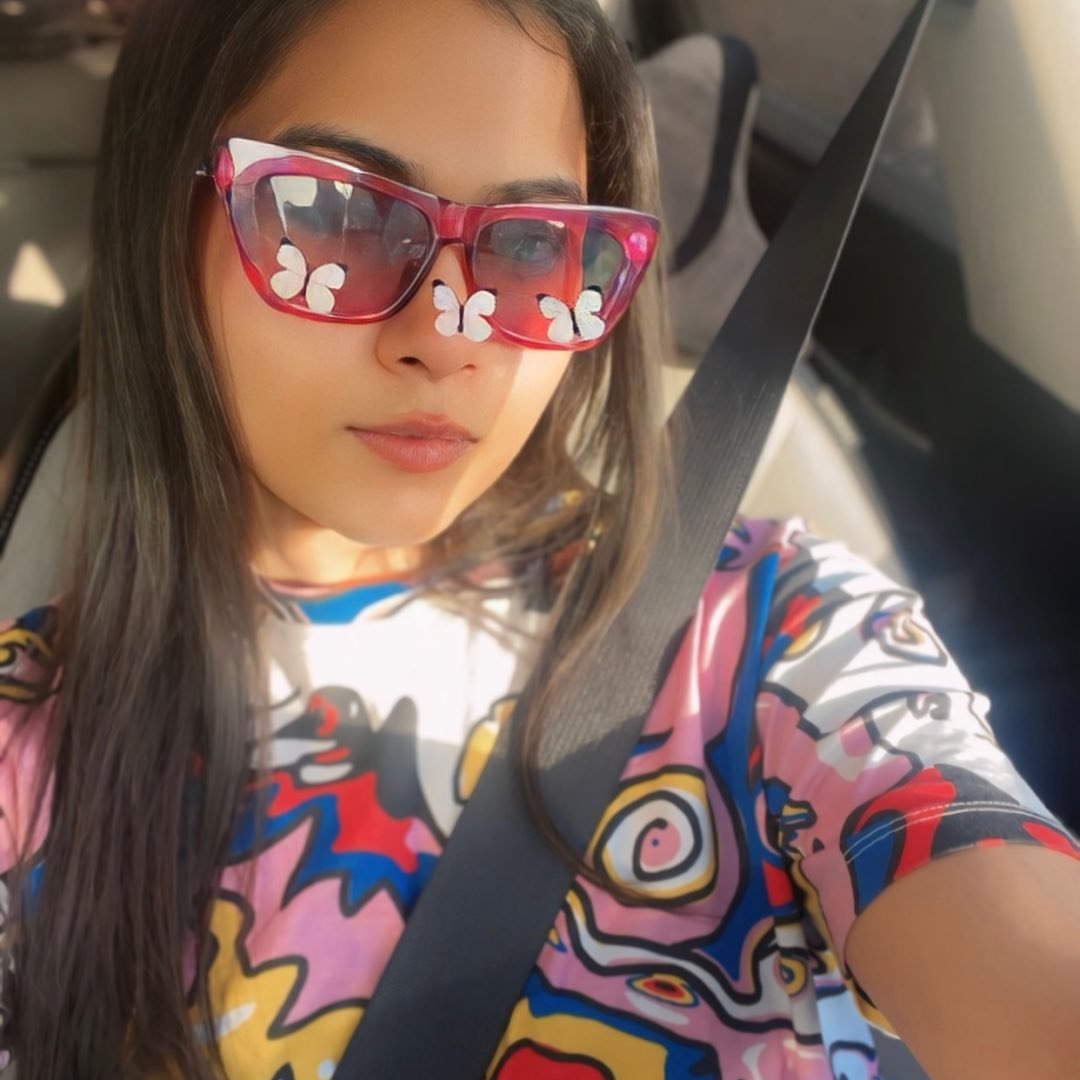