Dart Collections: Map Operations | Map Extensions | Map Methods
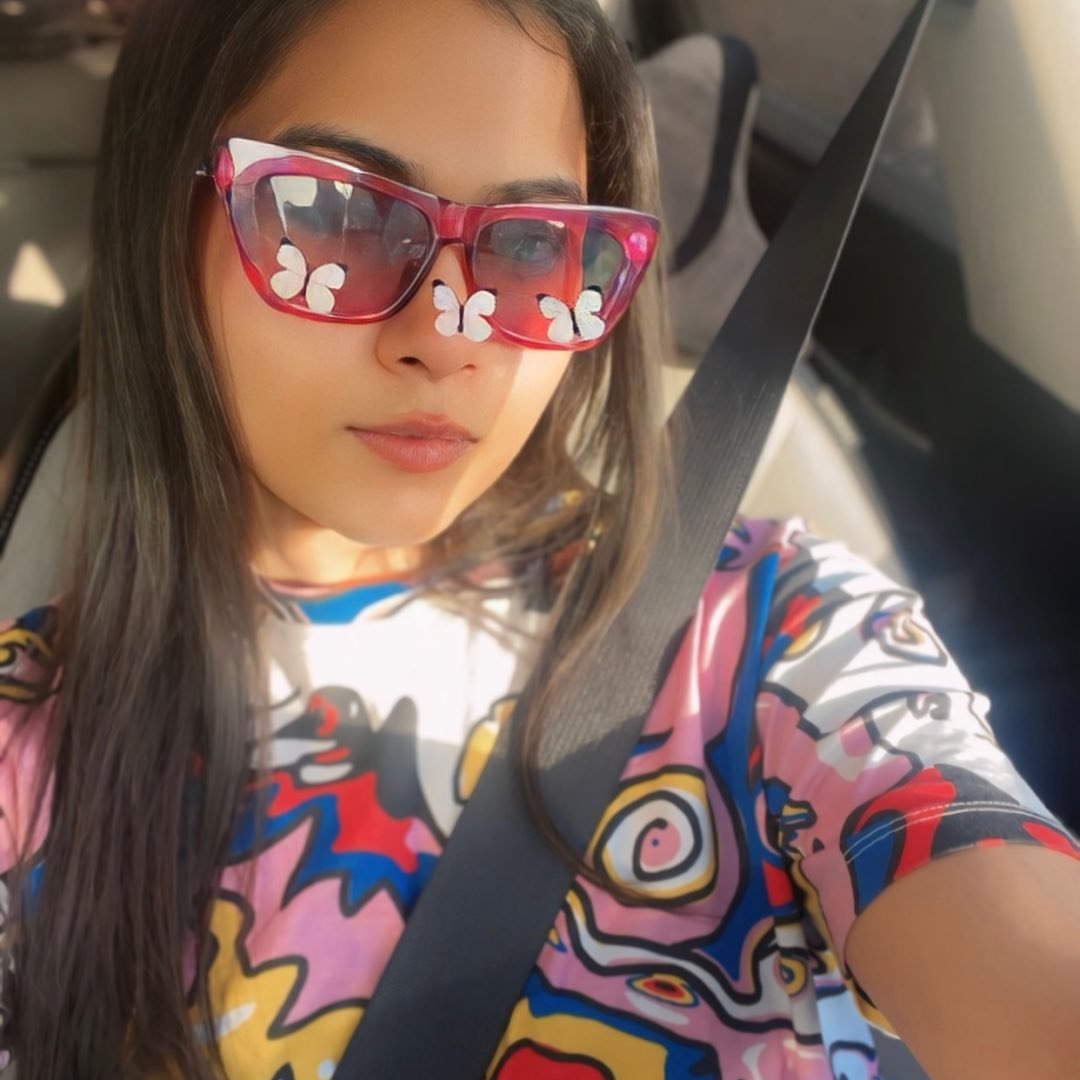
2 min read
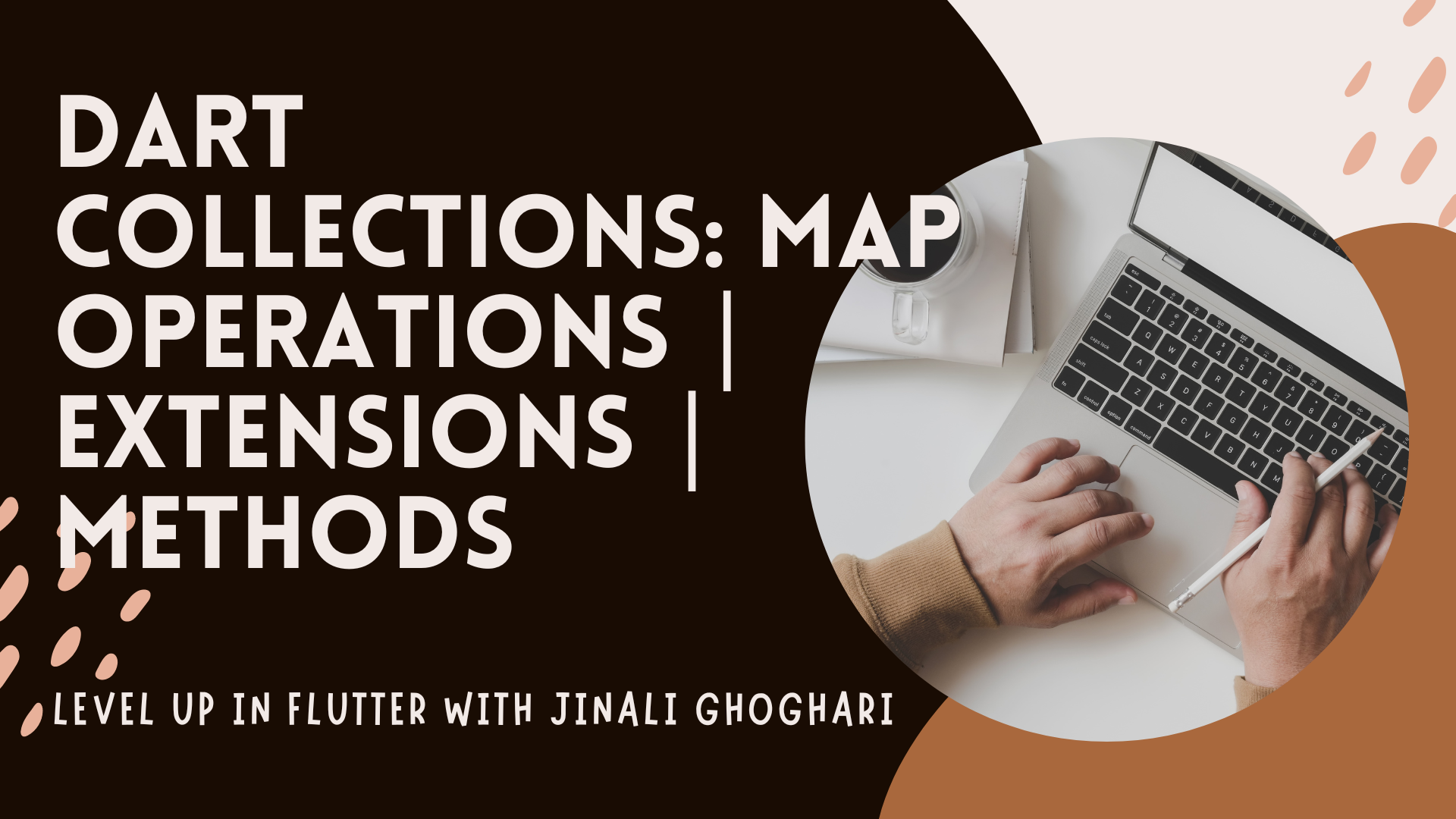
Map Operations:
1. Adding a Key-Value Pair:
- You can add a new key-value pair to a map using the square bracket notation.
Map<String, int> ages = {'Alice': 25, 'Bob': 30};
ages['Charlie'] = 22;
2. Updating a Value for a Key:
- You can update the value associated with a key.
Map<String, int> ages = {'Alice': 25, 'Bob': 30};
ages['Alice'] = 26;
3. Removing a Key-Value Pair:
- You can remove a key-value pair from the map using the
remove
method.
Map<String, int> ages = {'Alice': 25, 'Bob': 30};
ages.remove('Bob');
Map Extensions:
1. forEach:
- Iterates through the map and applies a function to each key-value pair.
Map<String, int> ages = {'Alice': 25, 'Bob': 30};
ages.forEach((key, value) {
print('$key\'s age is $value');
});
Map Methods:
1. containsKey:
- Checks if the map contains a specific key.
Map<String, int> ages = {'Alice': 25, 'Bob': 30};
print(ages.containsKey('Alice')); // Output: true
2. containsValue:
- Checks if the map contains a specific value.
Map<String, int> ages = {'Alice': 25, 'Bob': 30};
print(ages.containsValue(25)); // Output: true
3. keys:
- Returns an iterable of all keys in the map.
Map<String, int> ages = {'Alice': 25, 'Bob': 30};
print(ages.keys); // Output: (Alice, Bob)
4. values:
- Returns an iterable of all values in the map.
Map<String, int> ages = {'Alice': 25, 'Bob': 30};
print(ages.values); // Output: (25, 30)
5. isEmpty:
- Checks if the map is empty.
Map<String, int> ages = {};
print(ages.isEmpty); // Output: true
6. isNotEmpty:
- Checks if the map is not empty.
Map<String, int> ages = {'Alice': 25};
print(ages.isNotEmpty); // Output: true
These are some common operations, extensions, and methods you can use with Dart Map
collections. Depending on your specific needs, you can choose the appropriate operation or method for your map manipulation.
0
Subscribe to my newsletter
Read articles from Jinali Ghoghari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
dart map operationsdart map methodsdart map extensionsDart#dart languagedart programming tutorialdart-map
Written by
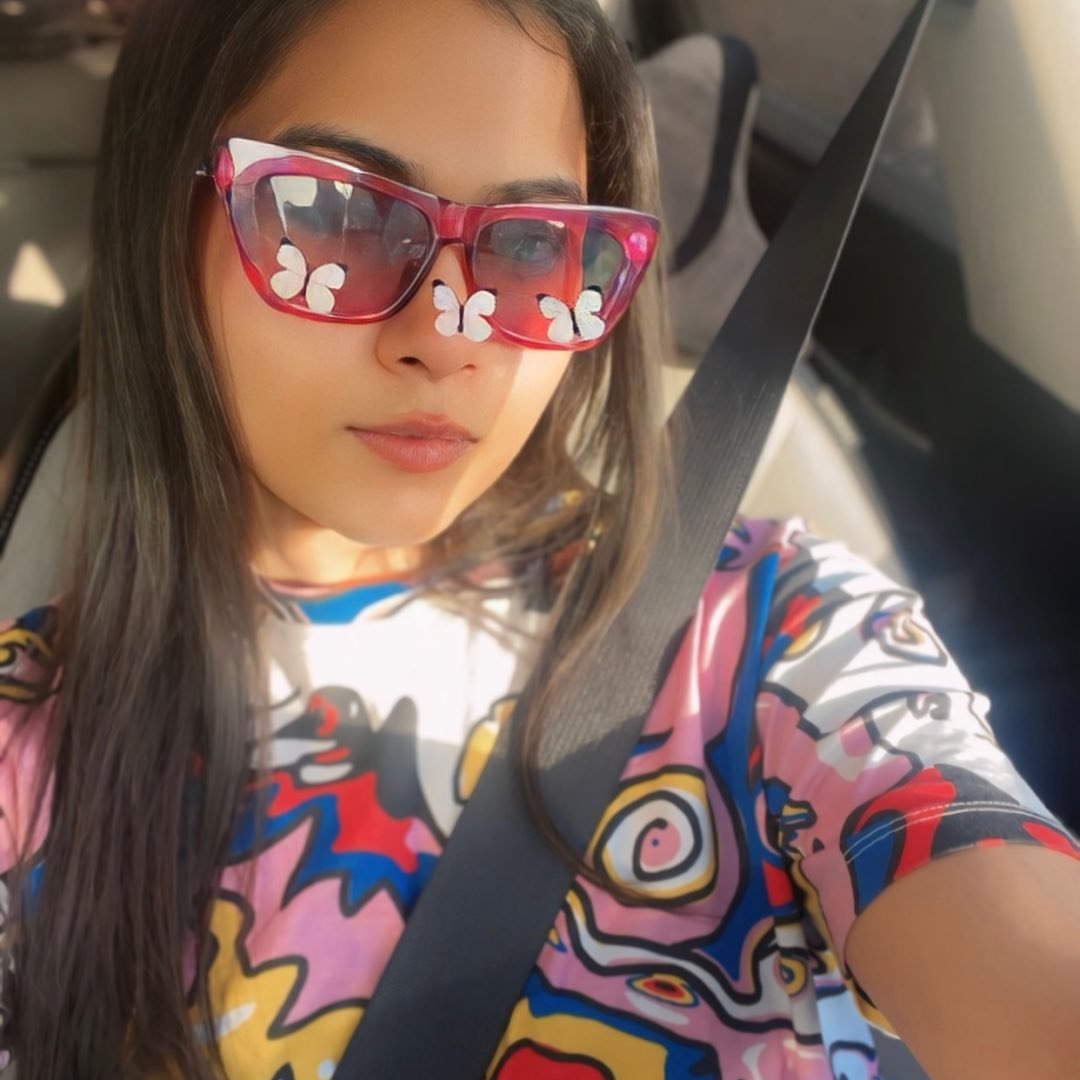