Mastering Concurrency in Python: Threads, Processes, and the Global Interpreter Lock (GIL)

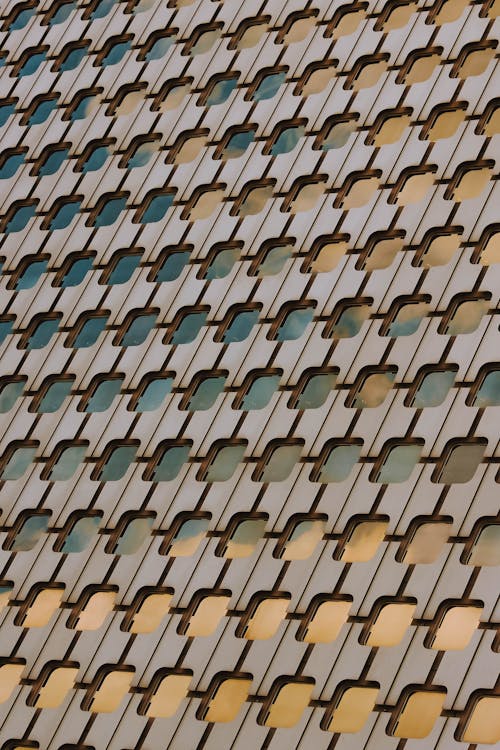
Concurrency is a crucial concept in modern programming, allowing applications to perform multiple tasks simultaneously and utilize system resources efficiently. Python provides several mechanisms for achieving concurrency, including threads and processes. However, Python's concurrency model is unique due to the presence of the Global Interpreter Lock (GIL), which introduces some limitations and considerations. In this article, we'll explore threads, processes, and the GIL in Python, and how they impact concurrent programming.
Understanding Threads in Python: Threads are lightweight units of execution within a process, allowing for concurrent execution of multiple tasks. In Python, the threading
module provides a simple and intuitive way to create and manage threads. While threads can improve application responsiveness and efficiency, they are not a silver bullet for CPU-bound tasks due to the GIL.
The Global Interpreter Lock (GIL): The GIL is a mechanism in Python's interpreter that ensures thread safety by allowing only one thread to execute Python bytecode at a time. This means that even though Python supports threads, they are not truly parallel because only one thread can run Python code simultaneously. The GIL is particularly relevant for CPU-bound tasks, as it limits the benefits of multithreading for such workloads.
When to Use Threads in Python: Despite the limitations imposed by the GIL, threads can still be useful in Python for certain types of tasks, such as:
I/O-bound operations: When performing I/O operations (e.g., network requests, file I/O), threads can improve performance by allowing other threads to run while one is blocked waiting for I/O.
GUI applications: Threads are commonly used in GUI applications to prevent the main thread from becoming unresponsive during long-running operations.
Multi-threaded libraries: Some Python libraries, such as NumPy and Pandas, can leverage multiple threads for certain operations, effectively bypassing the GIL.
Understanding Processes in Python: While threads are limited by the GIL for CPU-bound tasks, processes offer true parallelism in Python. A process is an instance of a computer program being executed, with its own memory space and system resources. Python's multiprocessing
module allows you to create and manage processes, enabling parallel execution of CPU-intensive tasks.
Processes vs. Threads: Processes are more heavyweight than threads, as they require separate memory spaces and system resources. However, processes can take full advantage of multiple CPU cores and are not limited by the GIL. Communication and data sharing between processes are more complex, often relying on techniques like pipes, queues, or shared memory.
When to Use Processes in Python: Processes are particularly useful for CPU-bound tasks that can benefit from parallel execution, such as:
Computationally intensive operations: Tasks like data processing, scientific calculations, and machine learning can significantly benefit from parallelization using processes.
Long-running tasks: If you have a long-running task that can be divided into independent subtasks, using processes can speed up the overall execution time.
Isolation and fault tolerance: Processes provide isolation, ensuring that a failure in one process does not affect the others, improving fault tolerance.
Combining Threads and Processes: In some cases, a combination of threads and processes can be an effective approach for maximizing concurrency in Python applications. Threads can be used for I/O-bound tasks, while CPU-bound tasks can be parallelized using processes. This hybrid approach can leverage the strengths of both concurrency models while mitigating their respective limitations.
Example:
pythonCopy codeimport multiprocessing
import threading
import time
def cpu_bound_task(result):
# Simulate a CPU-intensive task
count = 0
for i in range(100000000):
count += i
result.put(count)
def main():
# Create a pool of processes
pool = multiprocessing.Pool(processes=4)
results = []
# Submit CPU-bound tasks to the process pool
for _ in range(4):
result = multiprocessing.Manager().Queue()
results.append(result)
pool.apply_async(cpu_bound_task, args=(result,))
# Wait for all processes to complete
pool.close()
pool.join()
# Process the results
for result in results:
print(result.get())
if __name__ == "__main__":
main()
In this example, we use the multiprocessing
module to create a pool of four processes. Each process executes a CPU-bound task, and the results are collected in queues. The apply_async
method allows us to submit tasks to the process pool asynchronously, enabling parallel execution. By combining processes and appropriate synchronization mechanisms (like queues), we can effectively leverage multiple CPU cores for computationally intensive tasks.
In Conclusion, Concurrency is a powerful concept in Python programming, enabling efficient utilization of system resources and improved application performance. While threads are limited by the GIL for CPU-bound tasks, they can be valuable for I/O-bound operations and GUI applications. Processes, on the other hand, offer true parallelism and are well-suited for CPU-intensive workloads. By understanding the strengths and limitations of threads, processes, and the GIL, Python developers can make informed decisions about which concurrency model to use based on their specific requirements. Additionally, combining threads and processes can lead to even more effective concurrent solutions in Python.
Subscribe to my newsletter
Read articles from David Oshare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

David Oshare
David Oshare
I am a Python developer with 2 years of experience. I love building things with code and sharing that knowledge with others. Looking to collaborate and keep learning!