First-Class Objects in Python
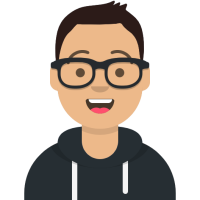
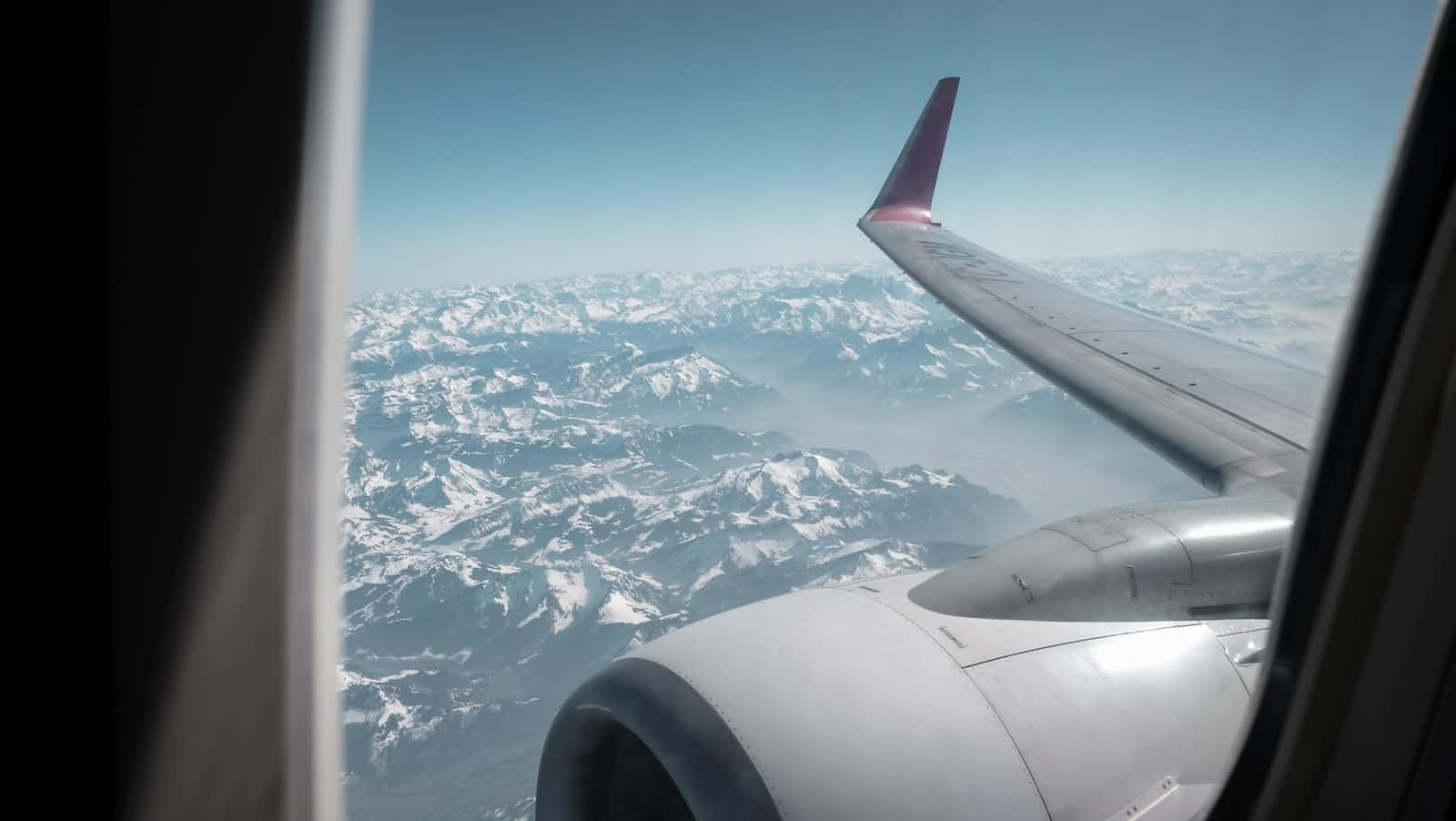
In Python, first-class objects (also known as first-class citizens) refer to entities that can be dynamically created, destroyed, passed to a function, returned as a value from a function, and assigned to a variable. This concept is crucial in understanding Python's flexible programming model, especially its function-handling capabilities. Functions, integers, strings, classes, dictionaries and virtually all other types of Python objects are treated as first-class objects.
Here are some key properties and examples to illustrate how first-class objects operate in Python:
1. Assigning to Variables
Any Python object can be assigned to a variable. This is true for functions, integers, strings, etc.
def my_function():
print("Hello, World!")
# Assigning function to a variable
var = my_function
var() # This will print "Hello, World!"
2. Passing as Arguments to Functions
You can pass objects as arguments to functions. This is particularly useful for higher-order functions that take other functions as parameters.
def greet(name):
return f"Hello, {name}!"
def process_function(func, arg):
return func(arg)
# Passing the 'greet' function as an argument to 'process_function'
result = process_function(greet, "Alice")
print(result) # This will print "Hello, Alice!"
3. Returning from Functions
Functions can return other objects, including functions.
def parent_function():
def child_function():
print("I'm the child!")
return child_function
# Getting a function returned from another function
child = parent_function()
child() # This will print "I'm the child!"
4. Storing in Data Structures
Objects can be stored in data structures, such as lists, dictionaries, sets, etc.
def add(x, y):
return x + y
def subtract(x, y):
return x - y
# Storing functions in a list
operations = [add, subtract]
for operation in operations:
print(operation(10, 5)) # This will print the results of add(10, 5) and subtract(10, 5)
These examples demonstrate the flexibility and power of first-class objects in Python, enabling dynamic and expressive programming patterns.
References:
Subscribe to my newsletter
Read articles from Maxat Akbanov directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
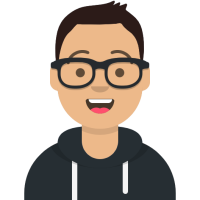
Maxat Akbanov
Maxat Akbanov
Hey, I'm a postgraduate in Cyber Security with practical experience in Software Engineering and DevOps Operations. The top player on TryHackMe platform, multilingual speaker (Kazakh, Russian, English, Spanish, and Turkish), curios person, bookworm, geek, sports lover, and just a good guy to speak with!