Mastering Algorithm Efficiency: Time and Space Complexity in Python

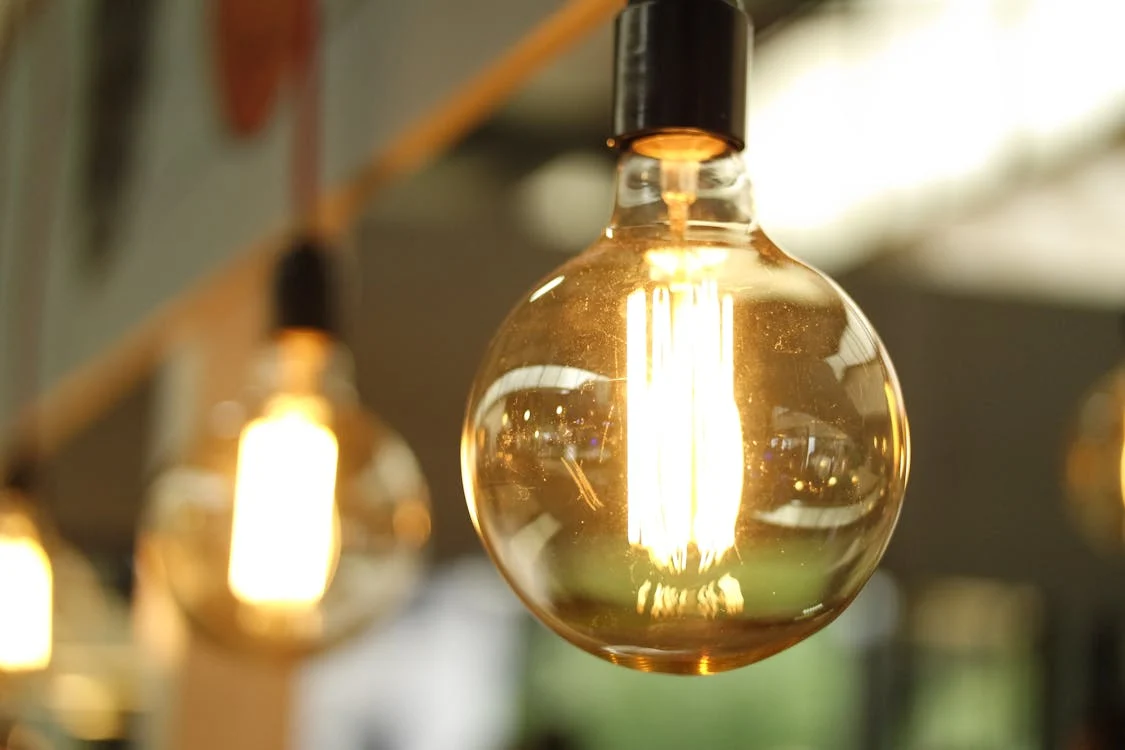
As programmers, we often face challenges that require writing efficient code to solve complex problems. When dealing with large amounts of data or computationally intensive tasks, the efficiency of our algorithms can significantly impact the performance and scalability of our applications. In this article, we'll explore the concepts of time and space complexity, which are fundamental metrics for analyzing and comparing the efficiency of algorithms in Python.
Understanding Time Complexity: Time complexity is a measure of how the execution time of an algorithm scales with the size of its input. It quantifies the amount of time an algorithm takes to complete its execution as the input size grows. By analyzing the time complexity of an algorithm, we can predict its performance for different input sizes and identify potential bottlenecks.
Common Time Complexity Classes
Constant Time (O(1)): Algorithms with constant time complexity have an execution time that remains the same regardless of the input size. Examples include accessing an element in an array or a constant-time operation in a hash table.
Logarithmic Time (O(log n)): Algorithms with logarithmic time complexity have an execution time that grows logarithmically with the input size. Examples include binary search on a sorted array or certain operations on balanced binary search trees.
Linear Time (O(n)): Algorithms with linear time complexity have an execution time that grows linearly with the input size. Examples include iterating over an array or a linked list.
Quadratic Time (O(n^2)): Algorithms with quadratic time complexity have an execution time that grows quadratically with the input size. Examples include the naïve implementation of sorting algorithms like bubble sort or insertion sort.
Exponential Time (O(2^n)): Algorithms with exponential time complexity have an execution time that grows exponentially with the input size. These algorithms are generally avoided due to their inefficiency, except for specific cases with small input sizes.
Understanding Space Complexity: Space complexity is a measure of how much additional memory an algorithm requires to execute, beyond the space needed to store the input data. It quantifies the amount of memory or auxiliary space an algorithm uses as the input size grows.
Common Space Complexity Classes
Constant Space (O(1)): Algorithms with constant space complexity use a fixed amount of additional memory, regardless of the input size. Examples include simple arithmetic operations or swapping variables.
Linear Space (O(n)): Algorithms with linear space complexity require additional memory that scales linearly with the input size. Examples include creating a new array or list to store intermediate results.
Quadratic Space (O(n^2)): Algorithms with quadratic space complexity require additional memory that grows quadratically with the input size. These algorithms are generally avoided due to their inefficiency, except for specific cases with small input sizes.
Analyzing Time and Space Complexity in Python: To analyze the time and space complexity of an algorithm in Python, we need to understand the time and space requirements of the individual operations and data structures used in the implementation. Python's built-in data structures and operations have well-defined time and space complexities, which can be used to analyze the overall complexity of an algorithm.
Example: Analyzing the Time Complexity of a Search Algorithm Let's consider a simple linear search algorithm that finds the index of a target element in an unsorted list:
pythonCopy codedef linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i
return -1
In the worst case, where the target element is not present or is the last element in the list, the algorithm needs to iterate over all elements. Therefore, the time complexity of this linear search algorithm is O(n), where n is the size of the input list.
Example: Analyzing the Space Complexity of a Recursive Algorithm Let's consider a recursive implementation of the factorial function:
pythonCopy codedef factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
In this implementation, each recursive call adds a new stack frame to the call stack, consuming additional memory proportional to the input size n. Therefore, the space complexity of this recursive factorial implementation is O(n).
Optimizing Algorithms: Understanding the time and space complexity of algorithms is crucial for optimizing their performance and memory usage. By identifying bottlenecks and inefficient operations, we can explore alternative algorithms or implement optimization techniques like caching, memoization, or data structure modifications to improve efficiency.
In conclusion ,Mastering the concepts of time and space complexity is essential for writing efficient algorithms in Python. By analyzing the scalability and resource requirements of our algorithms, we can make informed decisions about their suitability for different problem sizes and computational constraints. Consistently evaluating and optimizing the time and space complexity of our code can lead to significant performance improvements, enabling us to tackle more complex problems effectively. Whether you're working on data-intensive applications, machine learning models, or any computationally intensive task, understanding time and space complexity will be a valuable skill in your Python programming journey.
Subscribe to my newsletter
Read articles from David Oshare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

David Oshare
David Oshare
I am a Python developer with 2 years of experience. I love building things with code and sharing that knowledge with others. Looking to collaborate and keep learning!