Demystifying Django Channels: Unleashing Real-Time Power in Your Web Apps

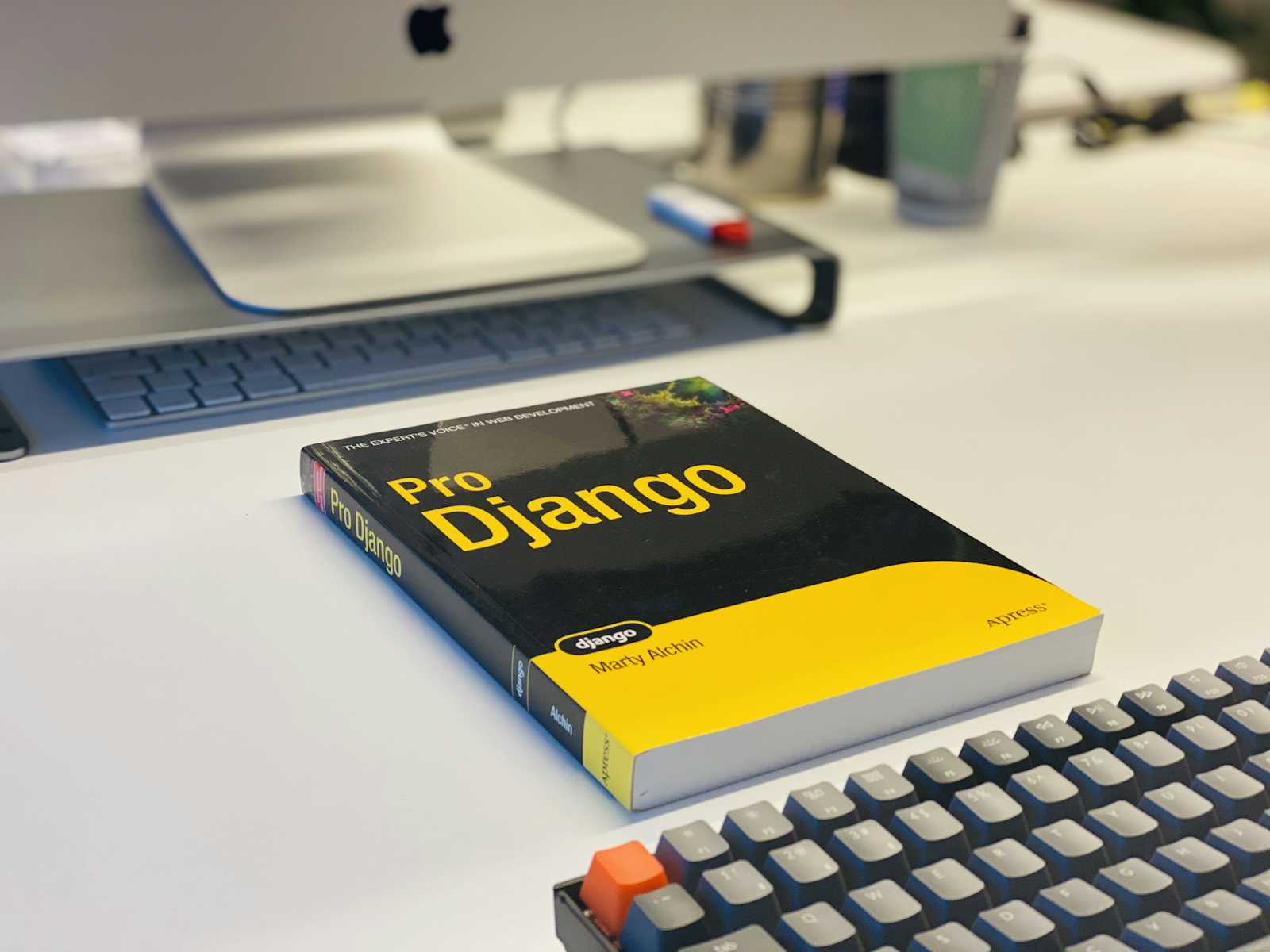
What is Django?
Before we delve into the exciting world of Django Channels, let's establish a foundation. Django is a free and open-source web framework built with Python. Imagine it as a powerful toolkit that streamlines the development process for web applications. Django takes care of many tedious back-end tasks, allowing you to focus on crafting the unique features and functionality of your application.
Enter Django Channels: The Real-Time Revolution
Now, let's meet Django Channels – an extension that supercharges your Django projects with real-time capabilities. Web development is constantly evolving, and users crave dynamic, interactive experiences. Django Channels empowers you to meet this demand by enabling real-time communication and persistent connections.
How Does it Work? Unveiling the Key Features
Asynchronous Magic: Traditionally, web applications handle requests one at a time. Django Channels breaks this mold with asynchronous support. Your application can now juggle multiple connections simultaneously, ensuring smooth and responsive performance even under heavy traffic.
WebSockets: The Two-Way Street: WebSockets are the game-changer for real-time interaction. Unlike standard HTTP requests, WebSockets establish a two-way conversation between the browser and the server. This allows for seamless data exchange in real-time, fostering a more engaging user experience. Django Channels integrates WebSockets seamlessly, enabling messages to flow back and forth instantaneously.
Channels Layers: The Internal Messaging Hub: Imagine a complex application where different parts need to communicate in real-time. Channels layers provide the solution. They act as a messaging system within your application, ensuring messages reach the intended recipients efficiently. In a chat application built with Django Channels, the Channels layer guarantees that messages are delivered to the right users the moment they are sent.
Consumers: The Workhorses of Real-Time Communication: Consumers are Python classes designed to handle the nitty-gritty of message processing. They act as the bridge between your application logic and the Channels layer. Consumers listen for incoming messages, process them according to your application's requirements, and generate outgoing responses.
Routing: Delivering Messages to the Right Destination: Just like roads direct traffic, routing in Django Channels ensures messages are delivered to the appropriate consumers. The routing mechanism analyzes incoming messages and directs them based on predefined rules. This ensures efficient message handling and avoids confusion within your application.
Backend Flexibility: Django Channels offers flexibility when it comes to backends. You can choose from options like Redis, in-memory channels, and ASGI (Asynchronous Server Gateway Interface) based on your project's specific needs and infrastructure.
Getting Started with Django Channels: A Quick Guide
Ready to harness the power of real-time? Integrating Django Channels is a breeze:
Install the
channels
package usingpip install channels
.Add
channels
to yourINSTALLED_APPS
setting in your Django project'ssettings.py
file.Configure your routing mechanism and define your consumers.
Explore the official Django Channels documentation for detailed instructions: Django channels documentation
Structure of Django channels files:
This is the consumers file and it defines the consumers that handle real-time communication within your application.
from channels.generic.websocket import WebsocketConsumer
# Import other dependencies as needed (e.g., JSON)
class YourConsumerName(WebsocketConsumer):
def connect(self):
# Handle connection establishment logic (e.g., accept, join groups)
def disconnect(self, close_code):
# Handle connection closing logic (e.g., leave groups)
def receive(self, text_data):
# Process incoming messages
# Send responses or broadcast messages
Key Methods:
● connect(): This method is called when a client establishes a WebSocket connection with the server. Here, you typically perform tasks like accepting the connection, joining channel groups (for broadcasting messages), or potentially authenticating the user.
● disconnect(): This method is invoked when the connection is closed (either by the client or server). You can use this to clean up resources, remove the user from channel groups, or perform any necessary actions upon disconnection.
● receive(): This is the heart of the consumer. It's called whenever the server receives a message from the client. Here, you process the message data (which is usually in text format), perform appropriate actions (e.g., database updates, broadcast messages), and potentially send responses back to the client using self.send()
The next file is the routing.py and plays a vital role in Django Channels applications by defining the routing mechanism for messages. It acts as the traffic controller, directing incoming messages to the appropriate consumers for handling.
from channels.auth import AuthMiddlewareStack
from channels.routing import ProtocolTypeRouter, URLRouter
application = ProtocolTypeRouter({
'websocket': AuthMiddlewareStack(
URLRouter([
r'^chat/connect/$', ChatConsumer.as_asgi(),
])
),
})
Explanation:
This example defines a
ProtocolTypeRouter
that differentiates between WebSocket connections and potentially other protocols handled by the application.The
websocket
key maps to anAuthMiddlewareStack
(optional for authentication) followed by aURLRouter
.The
URLRouter
defines the message pattern-consumer mapping. Here, the pattern^chat/connect/$
matches messages sent to the/chat/connect/
endpoint and routes them to theChatConsumer
class.
Bringing it to Life: Captivating Use Cases
Django Channels opens a treasure trove of possibilities for building modern web applications. Here are some exciting use cases:
Chat Applications: Create dynamic chat experiences where messages appear instantly, fostering a sense of real-time conversation.
Real-Time Notifications: Keep users informed with real-time updates without requiring constant page refreshes. Imagine stock tickers or social media notifications that come alive with Django Channels.
Live Updates: Take collaborative applications or dashboards to the next level with live updates. Django Channels ensures that data visualizations and content reflect changes instantaneously, providing an unparalleled user experience.
Want to see more Django magic?
For tips, tricks, and the latest in web dev! Follow me on:
Twitter @boyambassador21
Instagram @boyambassador
Subscribe to my newsletter
Read articles from Leroy Mapunzwana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
