Day 34: Python and Redis combination

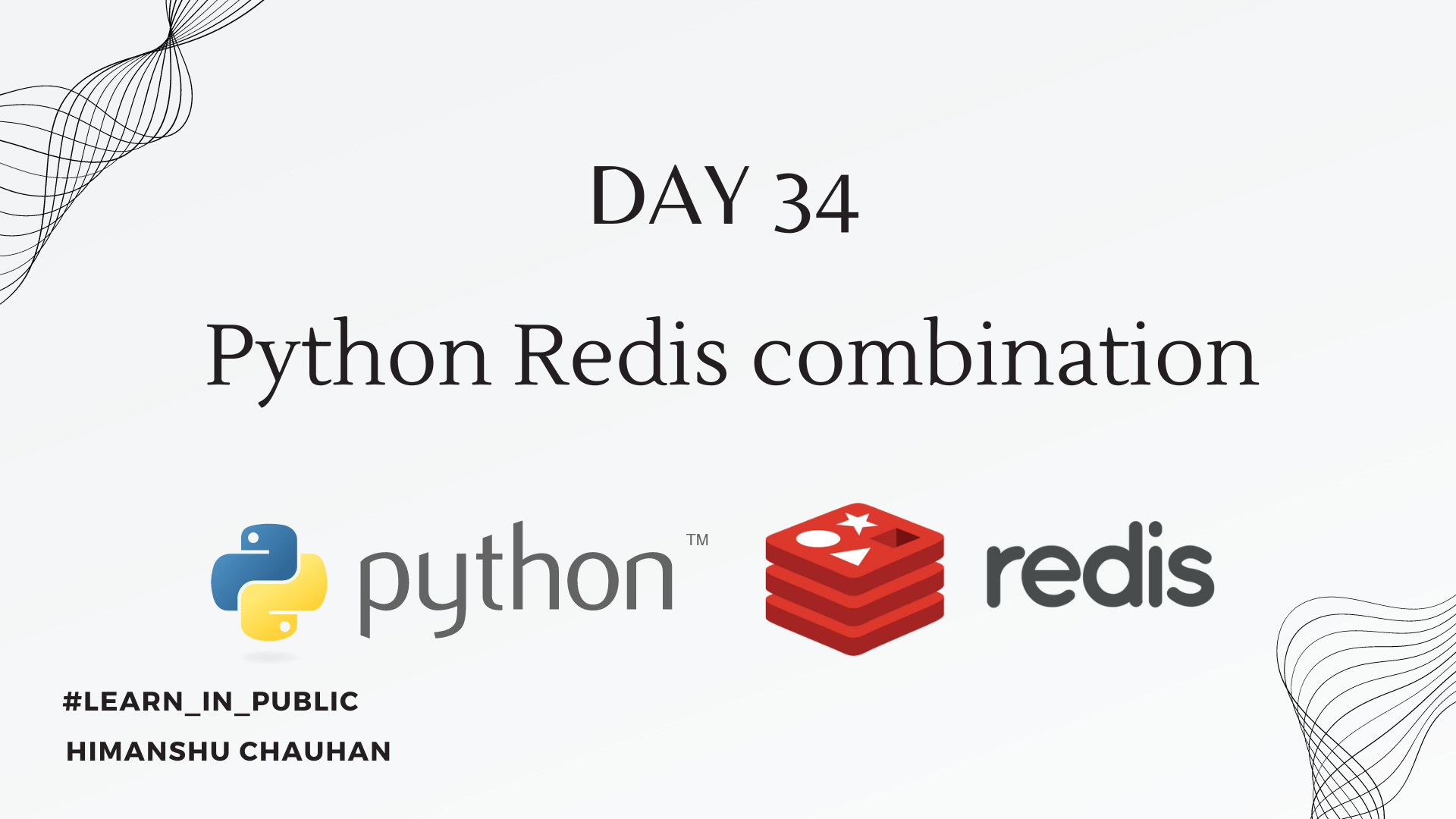
In this blog post, we will explore the synergies between Python and Redis, a combination that leverages Python's robustness in application development with Redis's efficiency in data storage and caching.
By combining Python and Redis, developers can optimize application performance and scalability. Python's versatility and extensive libraries make it ideal for building complex applications, while Redis's in-memory data store and caching capabilities ensure fast data retrieval and processing.
Redis
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It is known for its high performance, flexibility, and versatility in handling various data types and use cases.
In-Memory Database: Redis primarily stores data in RAM, which allows for extremely fast read and write operations. This makes it well-suited for applications that require low-latency data access.
Data Structures: Redis supports a variety of data structures, including strings, lists, sets, sorted sets, hashes, and more. Each data structure has associated commands for performing operations such as insertion, retrieval, modification, and deletion.
Persistence: While Redis is an in-memory database, it provides options for data persistence to disk. It offers different persistence mechanisms such as RDB (snapshotting) and AOF (append-only file) to ensure data durability and recovery in case of failures.
Caching: One of the common use cases for Redis is caching. It can store frequently accessed data in memory, reducing the need to fetch data from a slower backend database or external APIs. This improves application performance and responsiveness.
Code Setup for Redis
Installing Redis:
pip install redis
Create a connection with Redis:
import redis # Create a Redis connection redis_host = 'localhost' redis_port = 6379 redis_db = 0 # Default database redis_password = None # No password by default redis_client = redis.StrictRedis(host=redis_host, port=redis_port, db=redis_db, password=redis_password)
Read/Write Data with Redis:
# Set a key-value pair redis_client.set('my_key', 'Hello, Redis!') # Get the value for a key value = redis_client.get('my_key') print(value.decode('utf-8')) # Decode the bytes to string # Increment a key's value redis_client.incr('counter') # If the key doesn't exist, it will be initialized to 0 and then incremented counter_value = redis_client.get('counter') print(counter_value.decode('utf-8')) # Decode and print the incremented value # Delete a key redis_client.delete('my_key') # Check if a key exists key_exists = redis_client.exists('my_key') print(f'Key "my_key" exists: {key_exists}')
Conclusion
In conclusion, the Python-Redis combination streamlines application development by leveraging Redis's in-memory data storage and caching capabilities alongside Python's extensive libraries. This synergy enables developers to optimize data access, implement caching strategies, and enhance application responsiveness efficiently. This powerful duo simplifies data handling, improves performance, and provides a robust solution for building scalable and responsive applications across various domains.
Thank you๐๐
Subscribe to my newsletter
Read articles from Himanshu Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Himanshu Chauhan
Himanshu Chauhan
A coder how works for excellence...