User Input in Go language
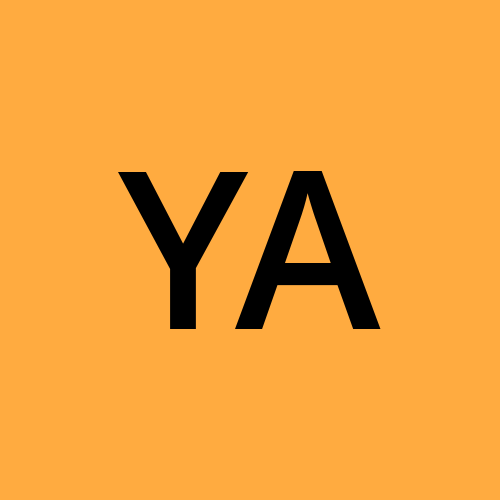
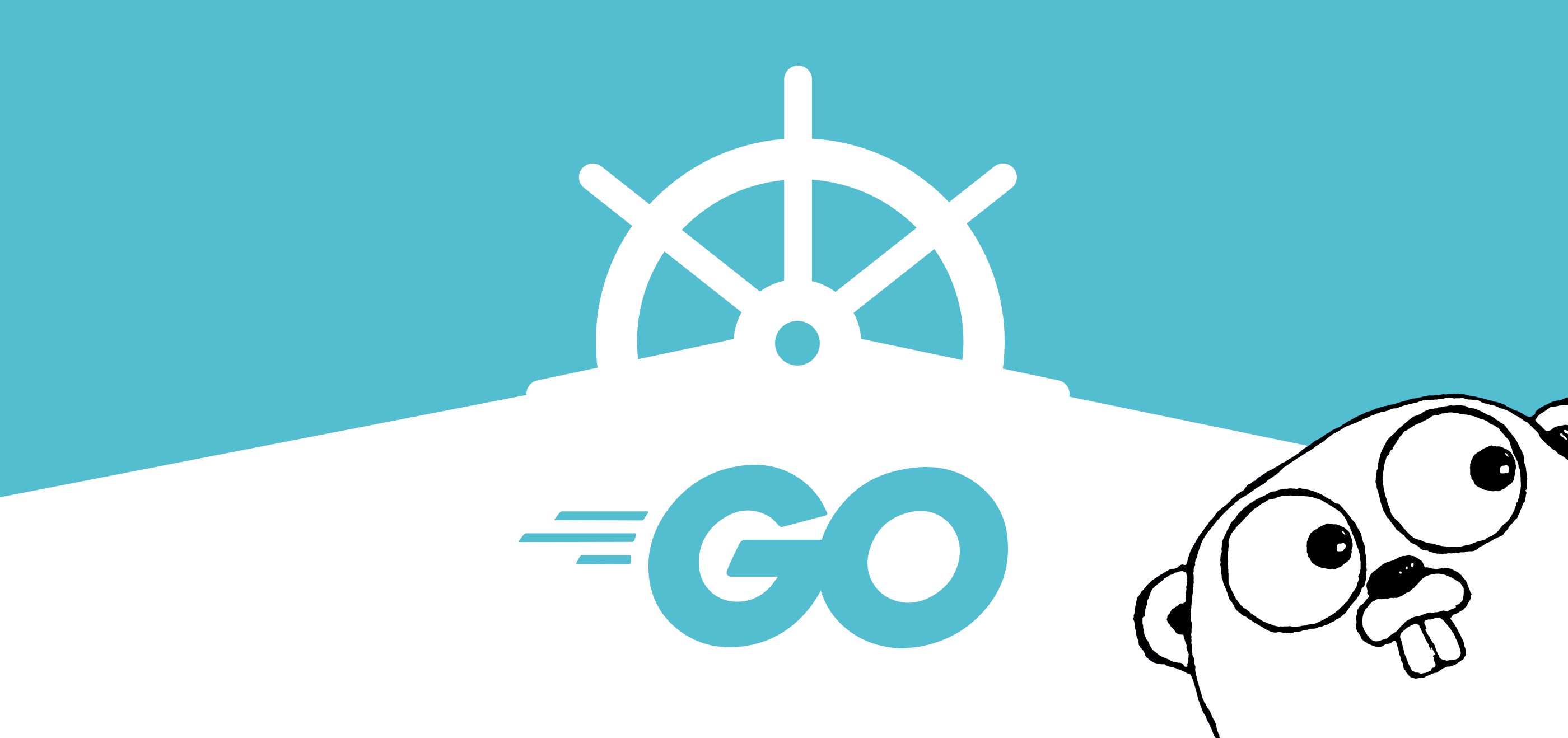
In order to take user input in Go language we need to create a reader variable using some inbuilt modules of Go
package main
import (
"bufio"
"fmt"
"os"
)
func main() {
fmt.Println("hi there")
reader := bufio.NewReader(os.Stdin)
fmt.Println("Enter your name: ")
name, _ := reader.ReadString('\n')
fmt.Println("you entered ", name)
}
bufio.Reader
The bufio.Reader
is a type in Go's bufio
package that provides buffered I/O operations for reading data from an underlying io.Reader
. It helps to efficiently read data from sources like files, network connections, or standard input.
Code Explanation
goCopy codereader := bufio.NewReader(os.Stdin)
In this line, we create a new bufio.Reader
instance by passing os.Stdin
as the underlying reader. os.Stdin
represents the standard input stream, which allows the program to read input from the user's keyboard.
goCopy codename, _ := reader.ReadString('\\n')
The reader.ReadString('\\n')
function reads input from the underlying reader until the delimiter '\\n'
(newline character) is encountered. It returns the data read as a string, including the delimiter.
The second value (_
in this case) is an error value. If there's no error during the read operation, it will be nil
. However, in this code, the error value is ignored using the blank identifier _
.
Delimiter
In the context of reader.ReadString('\\n')
, the delimiter '\\n'
represents the newline character. The function reads input until it encounters this character, which typically signifies the end of a line of user input.
Comma OK or Comma Error Syntax
The syntax name, _ := reader.ReadString('\\n')
is an example of the "comma ok" syntax in Go. It's a way to handle multiple return values from a function.
name
is the first return value, which is the string read from the input._
is the second return value, which represents the error value. By assigning it to the blank identifier_
, we're explicitly ignoring the error value.
This syntax is often used when you're interested in the first return value but don't want to handle the error value immediately. However, it's generally recommended to handle errors appropriately in production code.
Reader Functions
The bufio.Reader
provides several functions for reading data. Here are some common ones:
ReadString(delim byte)
: Reads data until the provided delimiter byte is encountered.ReadBytes(delim byte)
: Similar toReadString
, but returns a[]byte
instead of a string.ReadLine()
: Reads a single line of data, excluding the newline character.Read(p []byte)
: Reads data into the provided byte slicep
until the buffer is full or an error occurs.ReadByte()
: Reads and returns the next byte from the buffer.ReadSlice(delim byte)
: Reads data until the provided delimiter byte is encountered, returning a slice of bytes.
Subscribe to my newsletter
Read articles from Yasir Arafat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
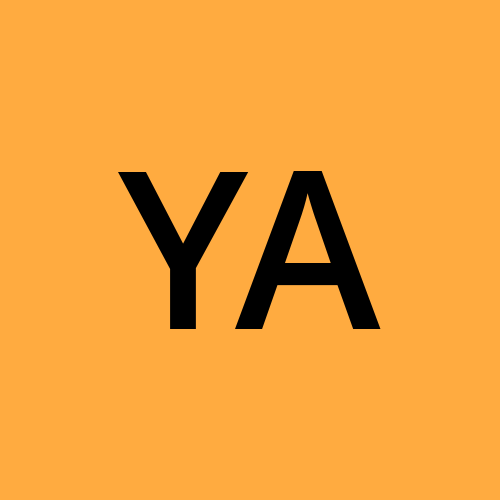
Yasir Arafat
Yasir Arafat
๐ Hello there! I'm Yasir Arafat, a motivated 3rd-year undergraduate at IIIT-Allahabad, driven by a passion for Data Structures and Algorithms (DSA) and Web Development. ๐ Achievements: Currently holding the esteemed title of a Knight at Leetcode and Specialist at Codeforces. Consistently achieving ranks near 1800 in various DSA/CC contests. ๐ป Technical Expertise: In-depth understanding of the MERN stack, with a focus on backend development. Noteworthy projects include the creation of a Virtual Trading Platform and a Student Portfolio Management system. Knowledgeable in Object-Oriented Programming (OOP), Database Management Systems (DBMS), and Operating Systems (OS). Proficient in Git version control. ๐ Outlook: Excited about the convergence of technology and creativity, I am open to new opportunities and collaborations.