Dart Functions
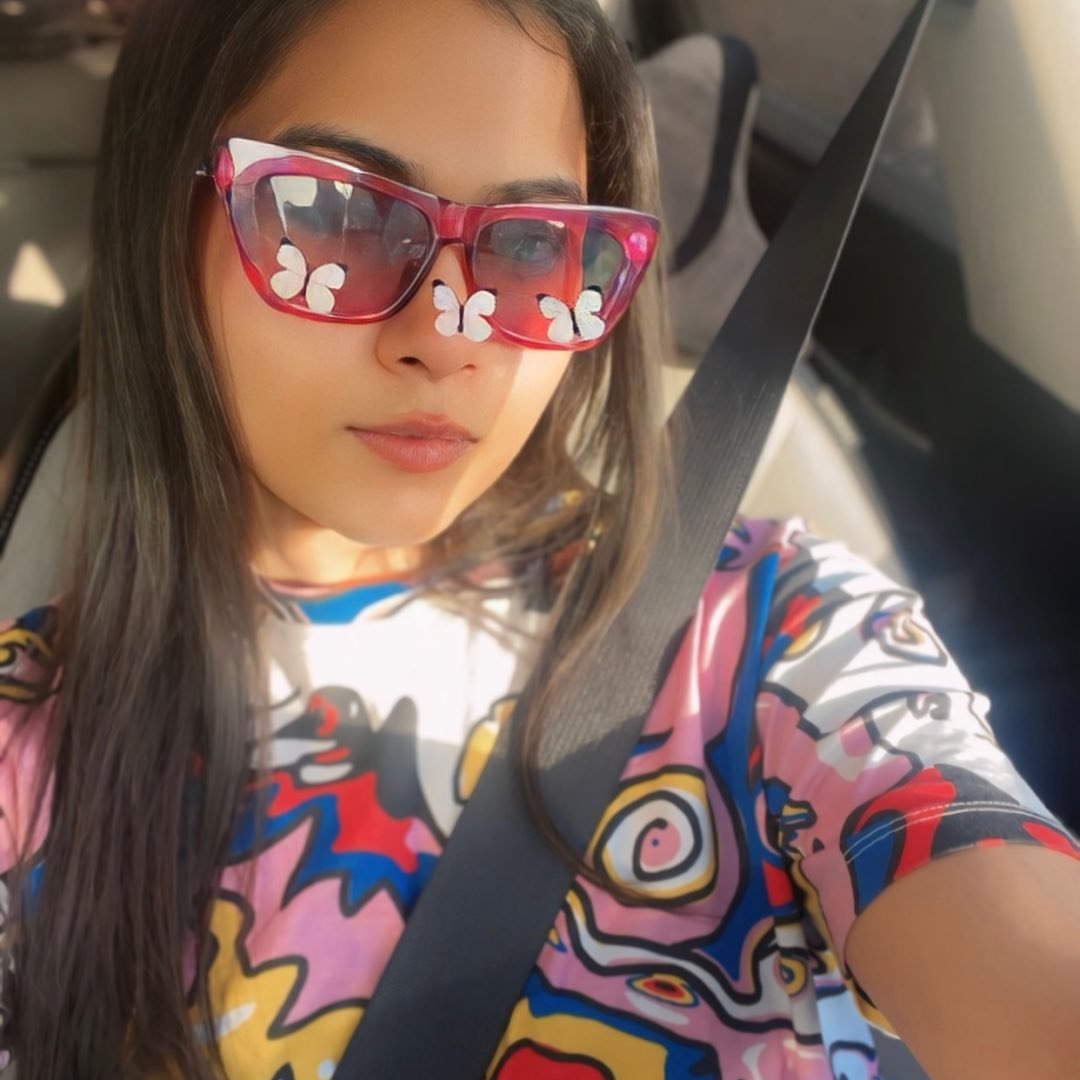
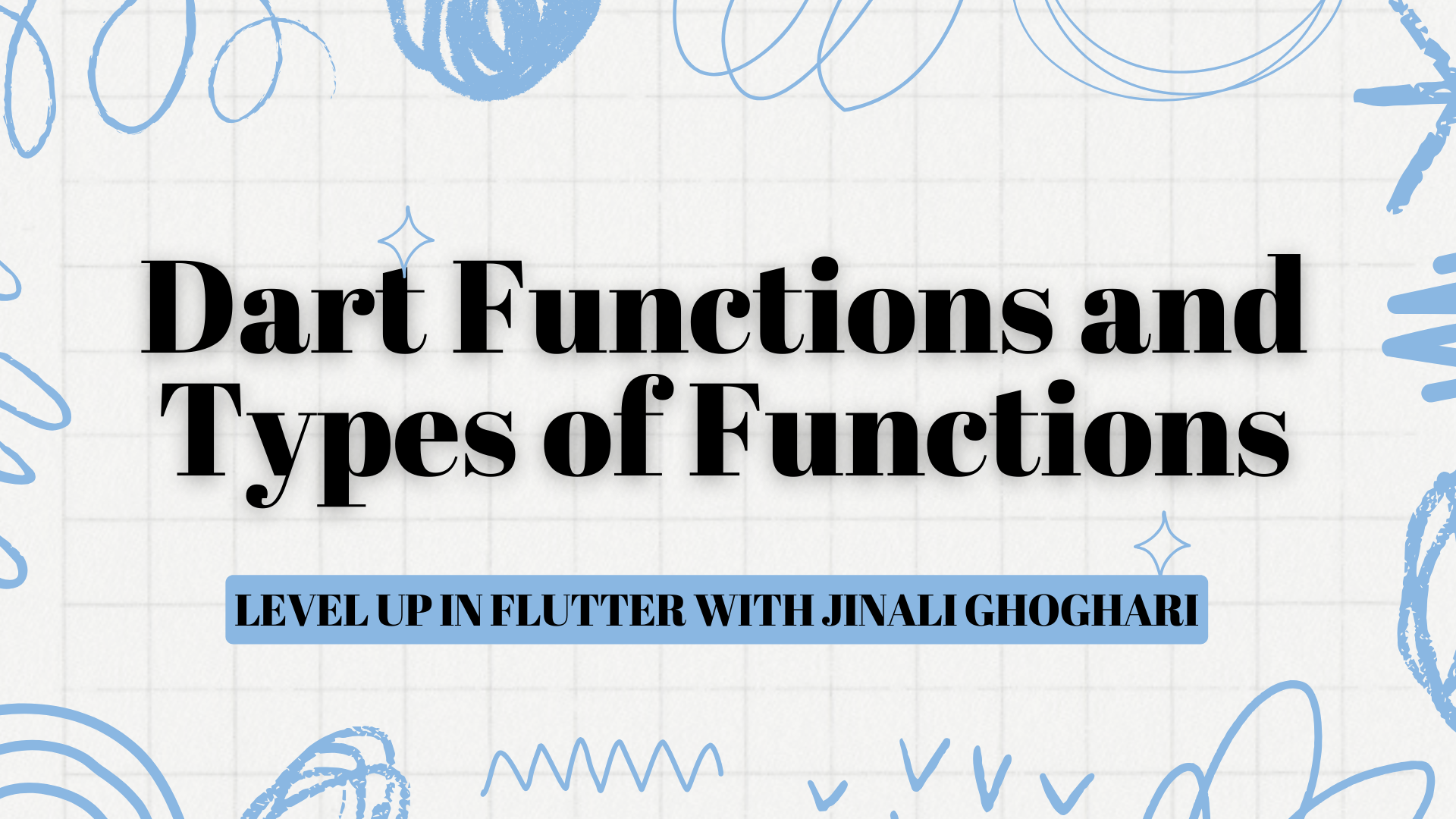
The function is a set of statements that take inputs, do some specific computation, and produce output.
Functions are created when certain statements are repeatedly occurring in the program and a function is created to replace them.
Functions make it easy to divide the complex program into smaller sub-groups and increase the code reusability of the program.
Different Types of Functions in Dart
Function with no arguments and no return type
Function with no arguments but return type
Function with arguments but no return type
Function With arguments and with return type
No arguments and no return type
Generally in this function, we do not give any arguments and expect no return type.
void myName() {
print("Jinali Ghoghari");
}
void main() {
print(" I am learning dart language -");
myName();
}
So myName is the function that is void means it is not returning anything and the empty pair of parentheses suggest that there is no argument that is passes to the function.
With no arguments but return type
Generally in this function, we are giving an argument and expect no return type.
int myPrice() {
int price = 0;
return price;
}
void main() {
int Price = myPrice();
print(" DART is the best plateform for developers which costs: ${Price}/-"};
}
With Arguments but no return type
Generally in this function, we do not give any argument but expect a return type.
myPrice(int price) {
print(price);
}
void main() {
print("DART is the best plateform for developers which costs: ");
print(0);
}
So myPrice is the function that is void means it is not returning anything and the pair of parentheses is not empty this time which suggests that it accept an argument.
With arguments and with return type
Generally in this function, we are giving an argument and except return type.
int mySum(int firstNumber, int secondNumber) {
return (firstNumber + secondNumber);
}
void main() {
int additionoftwoNumbers = mySum(400, 500);
print(additionoftwoNumbers);
}
So mySum is the function that is int means it is returning int type and the pair of parentheses is having two arguments that are used further in this function and then in the main function we are printing the addition of two numbers.
Subscribe to my newsletter
Read articles from Jinali Ghoghari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
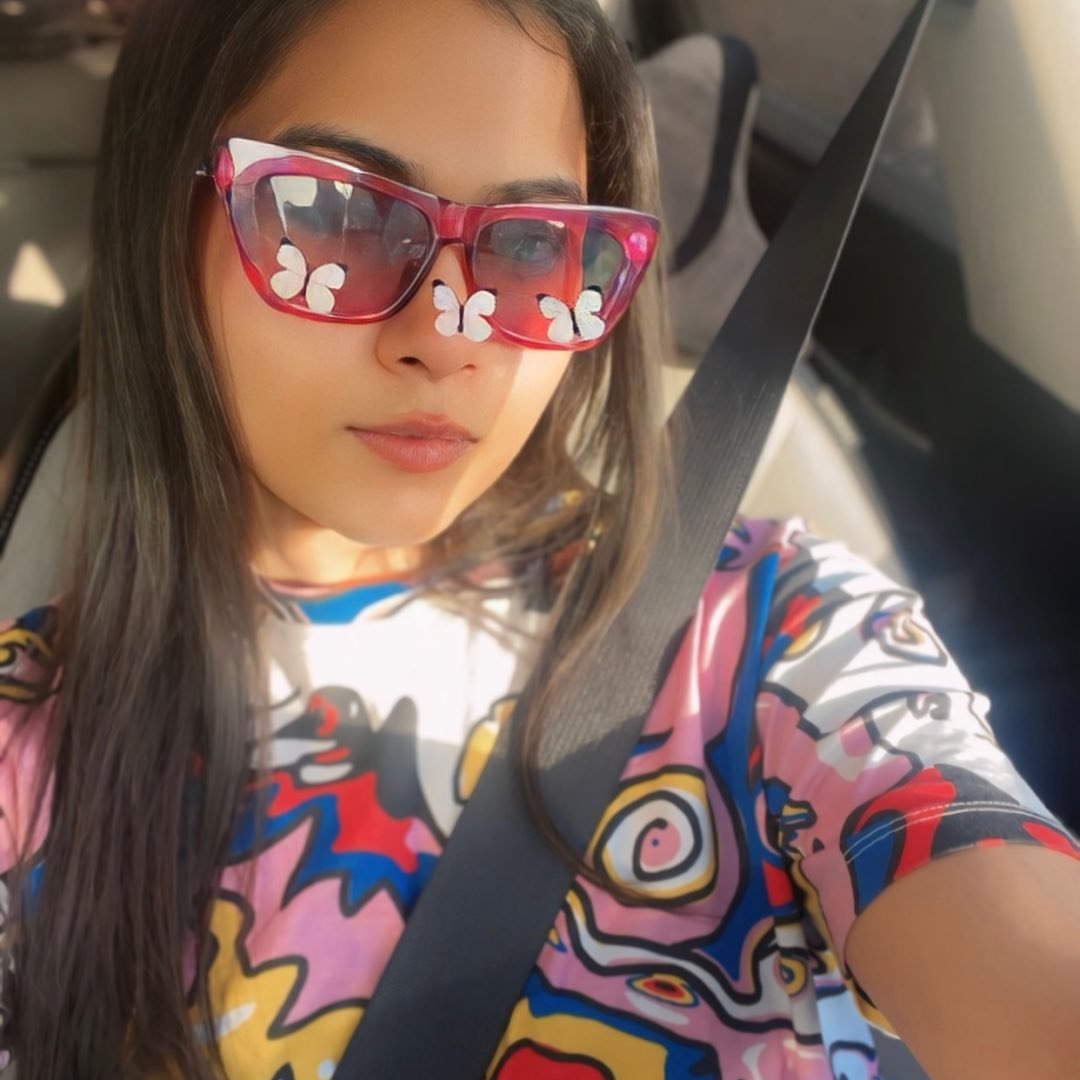