Valid Anagram
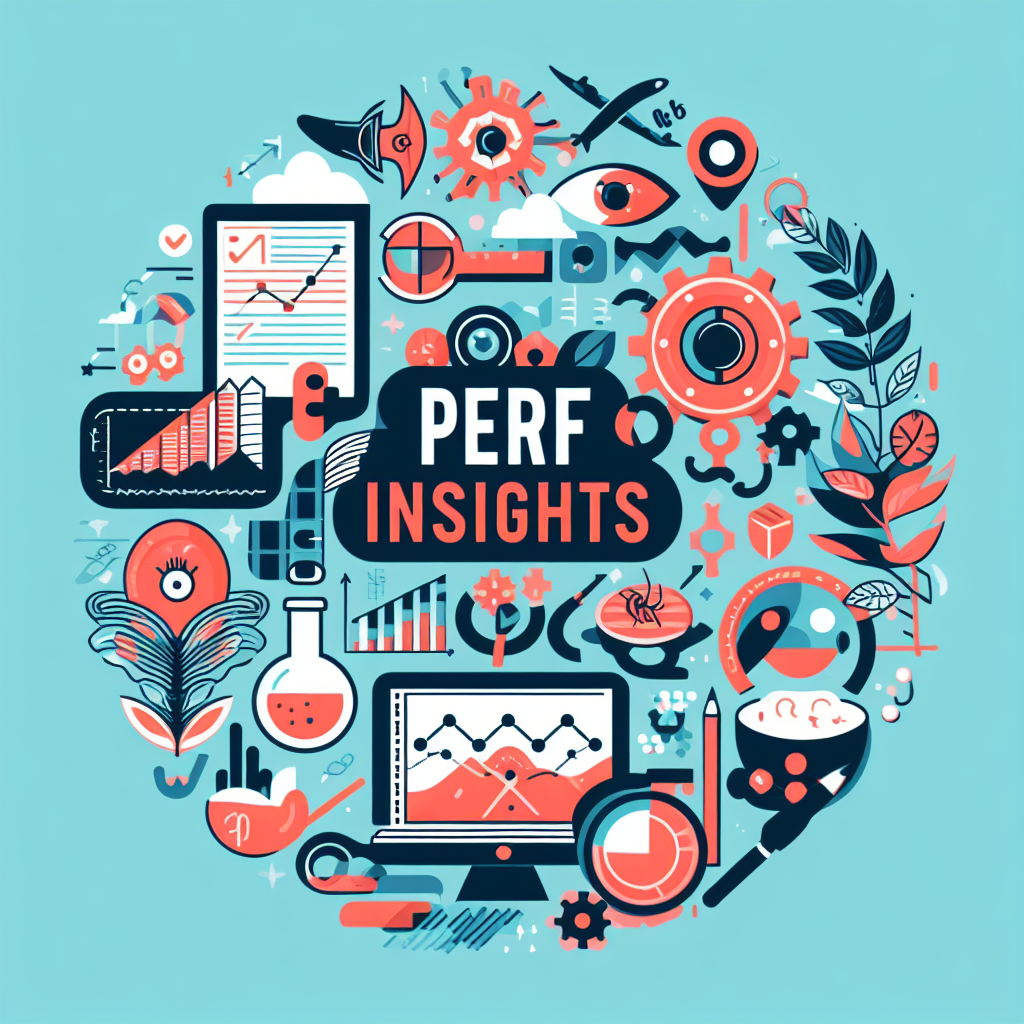
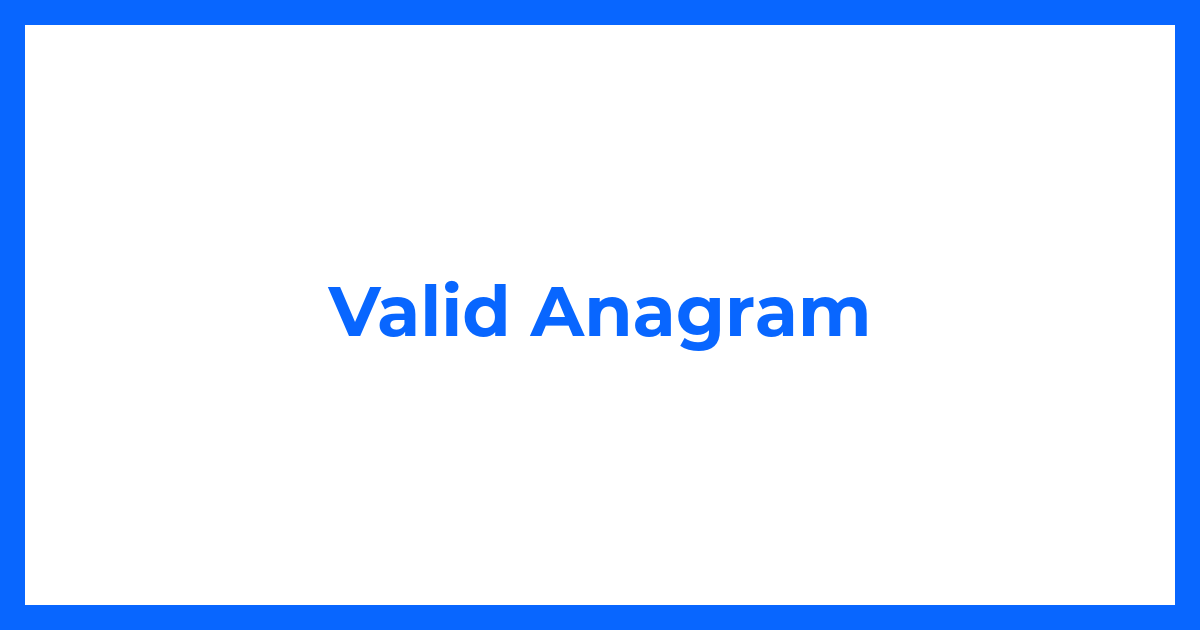
Given two strings s
and t
, return true
if t
is an anagram of s
, and false
otherwise.
An Anagram is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
LeetCode Problem - 242
class Solution {
// Method to check if two strings are anagrams of each other
public boolean isAnagram(String s, String t) {
// If the lengths of the strings are different, they cannot be anagrams
if (s.length() != t.length()) return false;
// Initializing an array to count occurrences of each character
int[] count = new int[26];
// Counting occurrences of characters in string s
for (char c : s.toCharArray()) {
count[c - 'a']++;
}
// Checking occurrences of characters in string t and decrementing counts
for (char c : t.toCharArray()) {
int idx = c - 'a';
count[idx]--;
// Early termination if count becomes negative, indicating an excess character
if (count[idx] < 0) return false;
}
// If all characters are accounted for, return true (strings are anagrams)
return true;
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
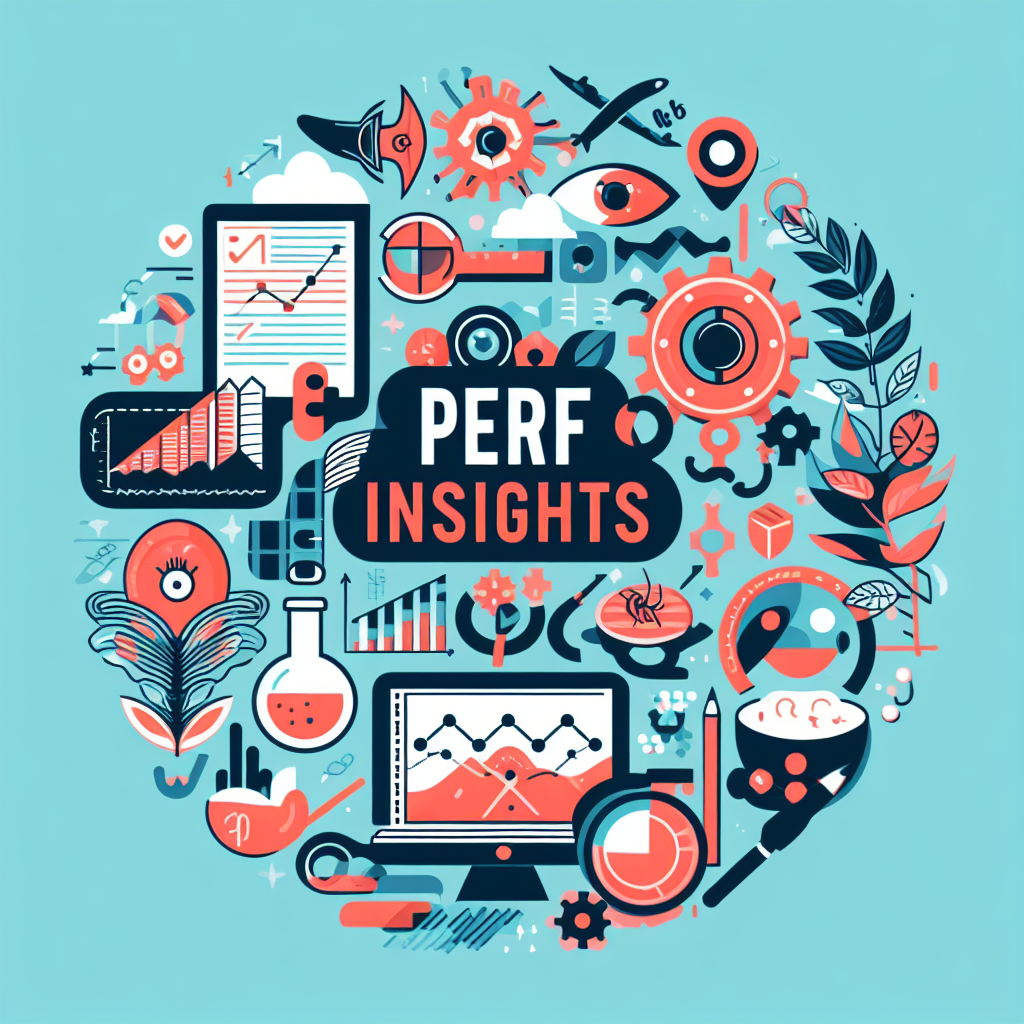
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.