Talking ChatBot

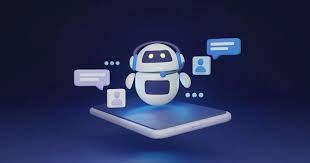
Good evening, everyone. I hope you're all doing well. In today's session, we will delve into the intricacies of the code that powers your chatbot. We will examine its functionalities and discuss how it operates to provide a seamless user experience.
Chatbot:
A chatbot is a computer program that simulates human conversation through text chats or voice commands. Chatbots are an artificial intelligence (AI) feature that can be embedded in any major messaging application. They can be programmed to perform routine tasks based on specific triggers and algorithms. Chatbots search their database of pre-programmed responses for a relevant answer and then send the response back to the user via the user interface. The user can then choose to respond further and the process repeats until the conversation ends.
Python code:
```python import random import string import warnings import numpy as np from sklearn.feature_extraction.text import TfidfVectorizer from sklearn.metrics.pairwise import cosine_similarity import warnings
warnings.filterwarnings('ignore')
import nltk from nltk.stem import WordNetLemmatizer
nltk.download('popular', quiet=True)
uncomment the following only the first time
nltk.download('punkt') # first-time use only
nltk.download('wordnet') # first-time use only
import pyttsx3
Initialize the text-to-speech engine
engine = pyttsx3.init()
Reading in the corpus
with open('txt/chatbot.txt', 'r', encoding='utf8', errors='ignore') as fin: raw = fin.read().lower()
Tokenization
sent_tokens = nltk.sent_tokenize(raw) word_tokens = nltk.word_tokenize(raw)
Preprocessing
lemmer = WordNetLemmatizer()
def LemTokens(tokens): return [lemmer.lemmatize(token) for token in tokens]
remove_punct_dict = dict((ord(punct), None) for punct in string.punctuation)
def LemNormalize(text): return LemTokens(nltk.word_tokenize(text.lower().translate(remove_punct_dict)))
Keyword Matching
GREETING_INPUTS = ("hello", "hi", "greetings", "sup", "what's up", "hey", "morning", "evening") GREETING_RESPONSES = ["hi", "hey", "nods", "hi there", "hello", "I am glad! You are talking to me", "good morning sir, have a good day ", 'good evening sir, i hope you had wonderful day', 'My name is jarvis ur personnel assistant ']
def greeting(sentence): """If user's input is a greeting, return a greeting response""" for word in sentence.split(): if word.lower() in GREETING_INPUTS: return random.choice(GREETING_RESPONSES)
Generating response
def response(user_response): robo_response = '' sent_tokens.append(user_response) TfidfVec = TfidfVectorizer(tokenizer=LemNormalize, stop_words='english') tfidf = TfidfVec.fit_transform(sent_tokens) vals = cosine_similarity(tfidf[-1], tfidf) idx = vals.argsort()[0][-2] flat = vals.flatten() flat.sort() req_tfidf = flat[-2] if (req_tfidf == 0): robo_response = robo_response + "I am sorry! I don't understand you" return robo_response else: robo_response = robo_response + sent_tokens[idx] return robo_response
Speak the introductory message
intro_message = "Hi sir. My name is Jarvis. I will answer your queries about Chatbots. If you want to exit, type Bye!" print("ROBO:", intro_message) engine.say(intro_message) engine.runAndWait()
flag = True
while (flag == True): user_response = input("You: ") user_response = user_response.lower() if (user_response != 'bye'): if (user_response == 'thanks' or user_response == 'thank you'): flag = False response_message = "You are welcome.." print("Jaarvis:", response_message) engine.say(response_message) engine.runAndWait() else: if (greeting(user_response) != None): response_message = greeting(user_response) print("Jarvis:", response_message) engine.say(response_message) engine.runAndWait() else: robo_response = response(user_response) print("Jarvis:", robo_response) engine.say(robo_response) engine.runAndWait() sent_tokens.remove(user_response) else: flag = False response_message = "Bye! take care.." print("Jarvis:", response_message) engine.say(response_message) engine.runAndWait()
Results:


### Explanation:
4. GITHUB LINK: FOR FILES:[https://github.com/SAIKUMAR500/chatbot.git](https://github.com/SAIKUMAR500/chatbot.git)
###
Here's a line-by-line explanation of the provided code:
```python
import random
import string
import warnings
import numpy as np
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
import warnings
warnings.filterwarnings('ignore')
- These lines import necessary libraries and modules including
random
,string
,warnings
,numpy
,TfidfVectorizer
, andcosine_similarity
from scikit-learn. Thewarnings.filterwarnings('ignore')
statement suppresses any warnings that might occur during execution.
import nltk
from nltk.stem import WordNetLemmatizer
nltk.download('popular', quiet=True)
# uncomment the following only the first time
# nltk.download('punkt') # first-time use only
# nltk.download('wordnet') # first-time use only
- These lines import the Natural Language Toolkit (NLTK) and the WordNet Lemmatizer. The
nltk.download
('popular', quiet=True)
statement downloads popular NLTK corpora and resources. The commented lines are for downloading additional NLTK resources if needed.
import pyttsx3
# Initialize the text-to-speech engine
engine = pyttsx3.init()
- These lines import the pyttsx3 library for text-to-speech conversion and initialize the text-to-speech engine.
# Reading in the corpus
with open('txt/chatbot.txt', 'r', encoding='utf8', errors='ignore') as fin:
raw = fin.read().lower()
- This code reads the chatbot corpus from the file 'chatbot.txt' and stores the content in the variable
raw
.
# Tokenization
sent_tokens = nltk.sent_tokenize(raw)
word_tokens = nltk.word_tokenize(raw)
- These lines tokenize the text into sentences (
sent_tokens
) and words (word_tokens
) using NLTK's tokenization functions.
# Preprocessing
lemmer = WordNetLemmatizer()
def LemTokens(tokens):
return [lemmer.lemmatize(token) for token in tokens]
remove_punct_dict = dict((ord(punct), None) for punct in string.punctuation)
def LemNormalize(text):
return LemTokens(nltk.word_tokenize(text.lower().translate(remove_punct_dict)))
- These lines initialize the WordNet Lemmatizer and define functions for lemmatization (
LemTokens
) and normalization (LemNormalize
), which tokenizes, lowercases, removes punctuation, and lemmatizes the text.
# Keyword Matching
GREETING_INPUTS = ("hello", "hi", "greetings", "sup", "what's up", "hey", "morning", "evening")
GREETING_RESPONSES = ["hi", "hey", "*nods*", "hi there", "hello", "I am glad! You are talking to me",
"good morning sir, have a good day ", 'good evening sir, i hope you had wonderful day',
'My name is jarvis ur personnel assistant ']
def greeting(sentence):
"""If user's input is a greeting, return a greeting response"""
for word in sentence.split():
if word.lower() in GREETING_INPUTS:
return random.choice(GREETING_RESPONSES)
- These lines define sets of input greetings (
GREETING_INPUTS
) and corresponding response messages (GREETING_RESPONSES
). Thegreeting
function checks if the user's input contains a greeting word and returns a random greeting response if it does.
# Generating response
def response(user_response):
robo_response = ''
sent_tokens.append(user_response)
TfidfVec = TfidfVectorizer(tokenizer=LemNormalize, stop_words='english')
tfidf = TfidfVec.fit_transform(sent_tokens)
vals = cosine_similarity(tfidf[-1], tfidf)
idx = vals.argsort()[0][-2]
flat = vals.flatten()
flat.sort()
req_tfidf = flat[-2]
if (req_tfidf == 0):
robo_response = robo_response + "I am sorry! I don't understand you"
return robo_response
else:
robo_response = robo_response + sent_tokens[idx]
return robo_response
- This code defines the
response
function, which generates responses to user inputs. It uses TF-IDF vectorization to calculate the similarity between the user input and the chatbot corpus, and selects the most similar sentence as the response.
# Speak the introductory message
intro_message = "Hi sir. My name is Jarvis. I will answer your queries about Chatbots. If you want to exit, type Bye!"
print("ROBO:", intro_message)
engine.say(intro_message)
engine.runAndWait()
- This code speaks out the introductory message using the text-to-speech engine.
flag = True
while (flag == True):
user_response = input("You: ")
user_response = user_response.lower()
if (user_response != 'bye'):
if (user_response == 'thanks' or user_response == 'thank you'):
flag = False
response_message =
That's it guy for today thank you for viewing
Bye
Subscribe to my newsletter
Read articles from Sai Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sai Kumar
Sai Kumar
I am a confident technology seeker who fearlessly explores new ways to engage with technology and pave the way for transformative progress in the world.